Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Creating a GIF file from scratch, especially drawing specific shapes like a triangle, involves understanding the GIF file format, color tables, and encoding image data using the LZW compression algorithm. This is quite complex without using any graphics libraries, but I will guide you through a simplified version. Here's a Python program to create a 200x200 GIF with a red triangle on a white background:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 | def write_byte(byte): import sys sys.stdout.buffer.write(byte.to_bytes(1, 'little')) def write_bytes(data): import sys sys.stdout.buffer.write(data) def main(): width, height = 200, 200 header = b'GIF89a' # Header for GIF89a format logical_screen_descriptor = width.to_bytes(2, 'little') + height.to_bytes(2, 'little') + b'\xF7\x00\x00' global_color_table = b'\xFF\xFF\xFF' + b'\xFF\x00\x00' + b'\x00\x00\x00' * 126 image_descriptor = b'\x2C' + b'\x00\x00\x00\x00' + width.to_bytes(2, 'little') + height.to_bytes(2, 'little') + b'\x00' # Create image data with a red triangle on a white background image_data = [0] * (width * height) for y in range(height): for x in range(width): if y < height - x: image_data[y * width + x] = 1 # Red pixel else: image_data[y * width + x] = 0 # White pixel # LZW Minimum Code Size lzw_min_code_size = 8 # Convert image data to LZW compressed format # For simplicity, we will use a very basic compression scheme here # Normally, you would use a proper LZW compression algorithm image_data_bytes = bytes(image_data) compressed_image_data = b'\x08' + image_data_bytes + b'\x01\x00' # Minimal compression # Image data block image_data_block = b'\x08' + len(compressed_image_data).to_bytes(1, 'little') + compressed_image_data + b'\x00' trailer = b'\x3B' # Trailer to end the GIF file # Output GIF file to stdout write_bytes(header) write_bytes(logical_screen_descriptor) write_bytes(global_color_table) write_bytes(image_descriptor) write_bytes(image_data_block) write_bytes(trailer) if __name__ == "__main__": main() |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 | def write_byte(byte): import sys sys.stdout.buffer.write(byte.to_bytes(1, 'little')) def write_bytes(data): import sys sys.stdout.buffer.write(data) def main(): width, height = 200, 200 header = b'GIF89a' # Header for GIF89a format logical_screen_descriptor = width.to_bytes(2, 'little') + height.to_bytes(2, 'little') + b'\xF7\x00\x00' global_color_table = b'\xFF\xFF\xFF' + b'\xFF\x00\x00' + b'\x00\x00\x00' * 126 image_descriptor = b'\x2C' + b'\x00\x00\x00\x00' + width.to_bytes(2, 'little') + height.to_bytes(2, 'little') + b'\x00' # Create image data with a red triangle on a white background image_data = [0] * (width * height) for y in range(height): for x in range(width): if y < height - x: image_data[y * width + x] = 1 # Red pixel else: image_data[y * width + x] = 0 # White pixel # LZW Minimum Code Size lzw_min_code_size = 8 # Convert image data to LZW compressed format # For simplicity, we will use a very basic compression scheme here # Normally, you would use a proper LZW compression algorithm image_data_bytes = bytes(image_data) compressed_image_data = b'\x08' + image_data_bytes + b'\x01\x00' # Minimal compression # Image data block image_data_block = b'\x08' + len(compressed_image_data).to_bytes(1, 'little') + compressed_image_data + b'\x00' trailer = b'\x3B' # Trailer to end the GIF file # Output GIF file to stdout write_bytes(header) write_bytes(logical_screen_descriptor) write_bytes(global_color_table) write_bytes(image_descriptor) write_bytes(image_data_block) write_bytes(trailer) if __name__ == "__main__": main() |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 | def write_byte(byte): import sys sys.stdout.buffer.write(byte.to_bytes(1, 'little')) def write_bytes(data): import sys sys.stdout.buffer.write(data) def main(): width, height = 200, 200 header = b'GIF89a' # Header for GIF89a format logical_screen_descriptor = width.to_bytes(2, 'little') + height.to_bytes(2, 'little') + b'\xF7\x00\x00' global_color_table = b'\xFF\xFF\xFF' + b'\xFF\x00\x00' + b'\x00\x00\x00' * 126 image_descriptor = b'\x2C' + b'\x00\x00\x00\x00' + width.to_bytes(2, 'little') + height.to_bytes(2, 'little') + b'\x00' # Create image data with a red triangle on a white background image_data = [0] * (width * height) for y in range(height): for x in range(width): if y < height - x: image_data[y * width + x] = 1 # Red pixel else: image_data[y * width + x] = 0 # White pixel # LZW Minimum Code Size lzw_min_code_size = 8 # Convert image data to LZW compressed format # For simplicity, we will use a very basic compression scheme here # Normally, you would use a proper LZW compression algorithm image_data_bytes = bytes(image_data) compressed_image_data = b'\x08' + image_data_bytes + b'\x01\x00' # Minimal compression # Image data block image_data_block = b'\x08' + len(compressed_image_data).to_bytes(1, 'little') + compressed_image_data + b'\x00' trailer = b'\x3B' # Trailer to end the GIF file # Output GIF file to stdout write_bytes(header) write_bytes(logical_screen_descriptor) write_bytes(global_color_table) write_bytes(image_descriptor) write_bytes(image_data_block) write_bytes(trailer) if __name__ == "__main__": main() |
b'Traceback (most recent call last):\n File "/usr/src/app/main.py", line 50, in <module>\n main()\n File "/usr/src/app/main.py", line 37, in main\n image_data_block = b\'\\x08\' + len(compressed_image_data).to_bytes(1, \'little\') + compressed_image_data + b\'\\x00\'\nOverflowError: int too big to convert\n'
Describe the shapes in this image and their color
cannot identify image file <_io.BytesIO object at 0x7fd702b74e50>
Testing if the previous output contains the string red
: False
Testing if the previous output contains the string triangle
: False
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Sure, here is a Python program that manually constructs a 200x200 GIF file with a red triangle on a white background. The program outputs the GIF file to stdout as specified.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 | import sys def create_gif_header(width, height): header = b'GIF89a' header += width.to_bytes(2, byteorder='little') header += height.to_bytes(2, byteorder='little') header += b'\xF7\x00\x00' # GCT follows for 256 colors, color resolution 8 bits return header def create_global_color_table(): gct = bytearray(3 * 256) gct[0:3] = b'\xFF\xFF\xFF' # Color 0: White gct[3:6] = b'\xFF\x00\x00' # Color 1: Red return gct def create_image_descriptor(x, y, width, height): descriptor = b'\x2C' descriptor += x.to_bytes(2, byteorder='little') descriptor += y.to_bytes(2, byteorder='little') descriptor += width.to_bytes(2, byteorder='little') descriptor += height.to_bytes(2, byteorder='little') descriptor += b'\x00' # No local color table, no interlace return descriptor def create_image_data(width, height): lzw_min_code_size = 8 image_data = bytearray() image_data.append(lzw_min_code_size) # Create the pixel data for the red triangle on a white background data = [] for y in range(height): for x in range(width): # Draw a red triangle if y >= height - x - 1: data.append(1) # Red else: data.append(0) # White # Simple LZW compression would be done here # For simplicity, let's use uncompressed data with GIF block structure data_bytes = bytes(data) sub_block_size = 255 for i in range(0, len(data_bytes), sub_block_size): image_data.append(min(sub_block_size, len(data_bytes) - i)) image_data.extend(data_bytes[i:i + sub_block_size]) image_data.append(0) # End of image data return image_data def create_gif_footer(): return b'\x3B' def main(): width, height = 200, 200 gif_data = bytearray() gif_data.extend(create_gif_header(width, height)) gif_data.extend(create_global_color_table()) gif_data.extend(create_image_descriptor(0, 0, width, height)) gif_data.extend(create_image_data(width, height)) gif_data.extend(create_gif_footer()) sys.stdout.buffer.write(gif_data) if __name__ == '__main__': main() |
1 | python your_soln.py > /tmp/a.gif |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 | import sys def create_gif_header(width, height): header = b'GIF89a' header += width.to_bytes(2, byteorder='little') header += height.to_bytes(2, byteorder='little') header += b'\xF7\x00\x00' # GCT follows for 256 colors, color resolution 8 bits return header def create_global_color_table(): gct = bytearray(3 * 256) gct[0:3] = b'\xFF\xFF\xFF' # Color 0: White gct[3:6] = b'\xFF\x00\x00' # Color 1: Red return gct def create_image_descriptor(x, y, width, height): descriptor = b'\x2C' descriptor += x.to_bytes(2, byteorder='little') descriptor += y.to_bytes(2, byteorder='little') descriptor += width.to_bytes(2, byteorder='little') descriptor += height.to_bytes(2, byteorder='little') descriptor += b'\x00' # No local color table, no interlace return descriptor def create_image_data(width, height): lzw_min_code_size = 8 image_data = bytearray() image_data.append(lzw_min_code_size) # Create the pixel data for the red triangle on a white background data = [] for y in range(height): for x in range(width): # Draw a red triangle if y >= height - x - 1: data.append(1) # Red else: data.append(0) # White # Simple LZW compression would be done here # For simplicity, let's use uncompressed data with GIF block structure data_bytes = bytes(data) sub_block_size = 255 for i in range(0, len(data_bytes), sub_block_size): image_data.append(min(sub_block_size, len(data_bytes) - i)) image_data.extend(data_bytes[i:i + sub_block_size]) image_data.append(0) # End of image data return image_data def create_gif_footer(): return b'\x3B' def main(): width, height = 200, 200 gif_data = bytearray() gif_data.extend(create_gif_header(width, height)) gif_data.extend(create_global_color_table()) gif_data.extend(create_image_descriptor(0, 0, width, height)) gif_data.extend(create_image_data(width, height)) gif_data.extend(create_gif_footer()) sys.stdout.buffer.write(gif_data) if __name__ == '__main__': main() |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 | import sys def create_gif_header(width, height): header = b'GIF89a' header += width.to_bytes(2, byteorder='little') header += height.to_bytes(2, byteorder='little') header += b'\xF7\x00\x00' # GCT follows for 256 colors, color resolution 8 bits return header def create_global_color_table(): gct = bytearray(3 * 256) gct[0:3] = b'\xFF\xFF\xFF' # Color 0: White gct[3:6] = b'\xFF\x00\x00' # Color 1: Red return gct def create_image_descriptor(x, y, width, height): descriptor = b'\x2C' descriptor += x.to_bytes(2, byteorder='little') descriptor += y.to_bytes(2, byteorder='little') descriptor += width.to_bytes(2, byteorder='little') descriptor += height.to_bytes(2, byteorder='little') descriptor += b'\x00' # No local color table, no interlace return descriptor def create_image_data(width, height): lzw_min_code_size = 8 image_data = bytearray() image_data.append(lzw_min_code_size) # Create the pixel data for the red triangle on a white background data = [] for y in range(height): for x in range(width): # Draw a red triangle if y >= height - x - 1: data.append(1) # Red else: data.append(0) # White # Simple LZW compression would be done here # For simplicity, let's use uncompressed data with GIF block structure data_bytes = bytes(data) sub_block_size = 255 for i in range(0, len(data_bytes), sub_block_size): image_data.append(min(sub_block_size, len(data_bytes) - i)) image_data.extend(data_bytes[i:i + sub_block_size]) image_data.append(0) # End of image data return image_data def create_gif_footer(): return b'\x3B' def main(): width, height = 200, 200 gif_data = bytearray() gif_data.extend(create_gif_header(width, height)) gif_data.extend(create_global_color_table()) gif_data.extend(create_image_descriptor(0, 0, width, height)) gif_data.extend(create_image_data(width, height)) gif_data.extend(create_gif_footer()) sys.stdout.buffer.write(gif_data) if __name__ == '__main__': main() |
b'GIF89a\xc8\x00\xc8\x00\xf7\x00\x00\xff\xff\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00,\x00\x00\x00\x00\xc8\x00\xc8\x00\x00\x08\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0
Describe the shapes in this image and their color
image file is truncated (38553 bytes not processed)
Testing if the previous output contains the string red
: False
Testing if the previous output contains the string triangle
: False
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Creating a GIF file from scratch, byte by byte, without using any graphics libraries, is quite a complex task. The GIF format has a specific structure and encoding that must be followed precisely. Below is a Python script that manually creates a simple 200x200 GIF image with a red triangle on a white background.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 | import sys def write_gif_header(f): # GIF Header f.write(b'GIF89a') # Logical Screen Descriptor f.write(b'\xC8\x00') # Logical Screen Width: 200 f.write(b'\xC8\x00') # Logical Screen Height: 200 f.write(b'\xF7') # GCT follows for 256 colors with resolution 8 f.write(b'\x00') # Background Color Index f.write(b'\x00') # Pixel Aspect Ratio def write_global_color_table(f): # Global Color Table: 256 colors # White (background) and Red (triangle), rest are black for i in range(256): if i == 0: f.write(b'\xFF\xFF\xFF') # White elif i == 1: f.write(b'\xFF\x00\x00') # Red else: f.write(b'\x00\x00\x00') # Black def write_image_descriptor(f): # Image Descriptor f.write(b'\x2C') # Image Separator f.write(b'\x00\x00') # Image Left Position f.write(b'\x00\x00') # Image Top Position f.write(b'\xC8\x00') # Image Width: 200 f.write(b'\xC8\x00') # Image Height: 200 f.write(b'\x00') # No Local Color Table, no interlace def write_image_data(f): # Image Data: LZW Minimum Code Size f.write(b'\x08') # Image Data: Encoded in LZW # For simplicity, use uncompressed data with LZW encoding f.write(b'\x81') # Block size: 129 bytes for y in range(200): for x in range(200): if (x < 100 and y < 100 and x + y >= 100) or (x >= 100 and y < 100 and y >= x - 100): f.write(b'\x01') # Red Pixel else: f.write(b'\x00') # White Pixel f.write(b'\x00') # End of LZW data def write_gif_trailer(f): # GIF Trailer f.write(b'\x3B') def main(): with open('/tmp/a.gif', 'wb') as f: write_gif_header(f) write_global_color_table(f) write_image_descriptor(f) write_image_data(f) write_gif_trailer(f) if __name__ == "__main__": main() |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 | import sys def write_gif_header(f): # GIF Header f.write(b'GIF89a') # Logical Screen Descriptor f.write(b'\xC8\x00') # Logical Screen Width: 200 f.write(b'\xC8\x00') # Logical Screen Height: 200 f.write(b'\xF7') # GCT follows for 256 colors with resolution 8 f.write(b'\x00') # Background Color Index f.write(b'\x00') # Pixel Aspect Ratio def write_global_color_table(f): # Global Color Table: 256 colors # White (background) and Red (triangle), rest are black for i in range(256): if i == 0: f.write(b'\xFF\xFF\xFF') # White elif i == 1: f.write(b'\xFF\x00\x00') # Red else: f.write(b'\x00\x00\x00') # Black def write_image_descriptor(f): # Image Descriptor f.write(b'\x2C') # Image Separator f.write(b'\x00\x00') # Image Left Position f.write(b'\x00\x00') # Image Top Position f.write(b'\xC8\x00') # Image Width: 200 f.write(b'\xC8\x00') # Image Height: 200 f.write(b'\x00') # No Local Color Table, no interlace def write_image_data(f): # Image Data: LZW Minimum Code Size f.write(b'\x08') # Image Data: Encoded in LZW # For simplicity, use uncompressed data with LZW encoding f.write(b'\x81') # Block size: 129 bytes for y in range(200): for x in range(200): if (x < 100 and y < 100 and x + y >= 100) or (x >= 100 and y < 100 and y >= x - 100): f.write(b'\x01') # Red Pixel else: f.write(b'\x00') # White Pixel f.write(b'\x00') # End of LZW data def write_gif_trailer(f): # GIF Trailer f.write(b'\x3B') def main(): with open('/tmp/a.gif', 'wb') as f: write_gif_header(f) write_global_color_table(f) write_image_descriptor(f) write_image_data(f) write_gif_trailer(f) if __name__ == "__main__": main() |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 | import sys def write_gif_header(f): # GIF Header f.write(b'GIF89a') # Logical Screen Descriptor f.write(b'\xC8\x00') # Logical Screen Width: 200 f.write(b'\xC8\x00') # Logical Screen Height: 200 f.write(b'\xF7') # GCT follows for 256 colors with resolution 8 f.write(b'\x00') # Background Color Index f.write(b'\x00') # Pixel Aspect Ratio def write_global_color_table(f): # Global Color Table: 256 colors # White (background) and Red (triangle), rest are black for i in range(256): if i == 0: f.write(b'\xFF\xFF\xFF') # White elif i == 1: f.write(b'\xFF\x00\x00') # Red else: f.write(b'\x00\x00\x00') # Black def write_image_descriptor(f): # Image Descriptor f.write(b'\x2C') # Image Separator f.write(b'\x00\x00') # Image Left Position f.write(b'\x00\x00') # Image Top Position f.write(b'\xC8\x00') # Image Width: 200 f.write(b'\xC8\x00') # Image Height: 200 f.write(b'\x00') # No Local Color Table, no interlace def write_image_data(f): # Image Data: LZW Minimum Code Size f.write(b'\x08') # Image Data: Encoded in LZW # For simplicity, use uncompressed data with LZW encoding f.write(b'\x81') # Block size: 129 bytes for y in range(200): for x in range(200): if (x < 100 and y < 100 and x + y >= 100) or (x >= 100 and y < 100 and y >= x - 100): f.write(b'\x01') # Red Pixel else: f.write(b'\x00') # White Pixel f.write(b'\x00') # End of LZW data def write_gif_trailer(f): # GIF Trailer f.write(b'\x3B') def main(): with open('/tmp/a.gif', 'wb') as f: write_gif_header(f) write_global_color_table(f) write_image_descriptor(f) write_image_data(f) write_gif_trailer(f) if __name__ == "__main__": main() |
b''
Describe the shapes in this image and their color
cannot identify image file <_io.BytesIO object at 0x7f6f0da08b30>
Testing if the previous output contains the string red
: False
Testing if the previous output contains the string triangle
: False
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Creating a GIF file from scratch manually involves understanding the GIF file format, which includes the header, logical screen descriptor, global color table, image descriptor, image data, and trailer. Below is a Python program that creates a 200x200 GIF image with a red triangle on a white background and writes the output to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | import sys def write_gif_header(): return b'GIF89a' def write_logical_screen_descriptor(width, height): packed_field = 0b11110001 # Global Color Table Flag set to 1, Color Resolution set to 111, Sort Flag set to 0, Size of Global Color Table set to 001 background_color_index = 0 pixel_aspect_ratio = 0 return width.to_bytes(2, 'little') + height.to_bytes(2, 'little') + bytes([packed_field, background_color_index, pixel_aspect_ratio]) def write_global_color_table(): # White and Red return b'\xFF\xFF\xFF' + b'\xFF\x00\x00' + b'\x00' * (3 * 2**1 - 3 * 2) def write_image_descriptor(): image_separator = b'\x2C' image_left = (0).to_bytes(2, 'little') image_top = (0).to_bytes(2, 'little') image_width = (200).to_bytes(2, 'little') image_height = (200).to_bytes(2, 'little') packed_field = b'\x00' return image_separator + image_left + image_top + image_width + image_height + packed_field def write_image_data(): lzw_minimum_code_size = b'\x02' image_data = b'\x04' + b'\x01\x00\x01\x00' + b'\x00' return lzw_minimum_code_size + image_data def write_gif_trailer(): return b'\x3B' def create_red_triangle_image(): width, height = 200, 200 pixels = [0] * (width * height) # Draw a red triangle for y in range(200): for x in range(200): if y > x: pixels[y * width + x] = 1 return pixels def encode_image_data(width, height, pixels): # Since the LZW encoding is non-trivial to implement manually, we'll use a simple uncompressed approach. image_data = b'\x08' # Block size: 8 bytes for y in range(height): for x in range(width): image_data += bytes([pixels[y * width + x]]) if len(image_data) % 255 == 0: image_data += b'\xFF\x00' # End of sub-block image_data += b'\x00' # Block terminator return image_data def create_gif(): width, height = 200, 200 header = write_gif_header() screen_descriptor = write_logical_screen_descriptor(width, height) color_table = write_global_color_table() image_descriptor = write_image_descriptor() pixels = create_red_triangle_image() image_data = encode_image_data(width, height, pixels) trailer = write_gif_trailer() return header + screen_descriptor + color_table + image_descriptor + image_data + trailer if __name__ == "__main__": gif_data = create_gif() sys.stdout.buffer.write(gif_data) |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | import sys def write_gif_header(): return b'GIF89a' def write_logical_screen_descriptor(width, height): packed_field = 0b11110001 # Global Color Table Flag set to 1, Color Resolution set to 111, Sort Flag set to 0, Size of Global Color Table set to 001 background_color_index = 0 pixel_aspect_ratio = 0 return width.to_bytes(2, 'little') + height.to_bytes(2, 'little') + bytes([packed_field, background_color_index, pixel_aspect_ratio]) def write_global_color_table(): # White and Red return b'\xFF\xFF\xFF' + b'\xFF\x00\x00' + b'\x00' * (3 * 2**1 - 3 * 2) def write_image_descriptor(): image_separator = b'\x2C' image_left = (0).to_bytes(2, 'little') image_top = (0).to_bytes(2, 'little') image_width = (200).to_bytes(2, 'little') image_height = (200).to_bytes(2, 'little') packed_field = b'\x00' return image_separator + image_left + image_top + image_width + image_height + packed_field def write_image_data(): lzw_minimum_code_size = b'\x02' image_data = b'\x04' + b'\x01\x00\x01\x00' + b'\x00' return lzw_minimum_code_size + image_data def write_gif_trailer(): return b'\x3B' def create_red_triangle_image(): width, height = 200, 200 pixels = [0] * (width * height) # Draw a red triangle for y in range(200): for x in range(200): if y > x: pixels[y * width + x] = 1 return pixels def encode_image_data(width, height, pixels): # Since the LZW encoding is non-trivial to implement manually, we'll use a simple uncompressed approach. image_data = b'\x08' # Block size: 8 bytes for y in range(height): for x in range(width): image_data += bytes([pixels[y * width + x]]) if len(image_data) % 255 == 0: image_data += b'\xFF\x00' # End of sub-block image_data += b'\x00' # Block terminator return image_data def create_gif(): width, height = 200, 200 header = write_gif_header() screen_descriptor = write_logical_screen_descriptor(width, height) color_table = write_global_color_table() image_descriptor = write_image_descriptor() pixels = create_red_triangle_image() image_data = encode_image_data(width, height, pixels) trailer = write_gif_trailer() return header + screen_descriptor + color_table + image_descriptor + image_data + trailer if __name__ == "__main__": gif_data = create_gif() sys.stdout.buffer.write(gif_data) |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | import sys def write_gif_header(): return b'GIF89a' def write_logical_screen_descriptor(width, height): packed_field = 0b11110001 # Global Color Table Flag set to 1, Color Resolution set to 111, Sort Flag set to 0, Size of Global Color Table set to 001 background_color_index = 0 pixel_aspect_ratio = 0 return width.to_bytes(2, 'little') + height.to_bytes(2, 'little') + bytes([packed_field, background_color_index, pixel_aspect_ratio]) def write_global_color_table(): # White and Red return b'\xFF\xFF\xFF' + b'\xFF\x00\x00' + b'\x00' * (3 * 2**1 - 3 * 2) def write_image_descriptor(): image_separator = b'\x2C' image_left = (0).to_bytes(2, 'little') image_top = (0).to_bytes(2, 'little') image_width = (200).to_bytes(2, 'little') image_height = (200).to_bytes(2, 'little') packed_field = b'\x00' return image_separator + image_left + image_top + image_width + image_height + packed_field def write_image_data(): lzw_minimum_code_size = b'\x02' image_data = b'\x04' + b'\x01\x00\x01\x00' + b'\x00' return lzw_minimum_code_size + image_data def write_gif_trailer(): return b'\x3B' def create_red_triangle_image(): width, height = 200, 200 pixels = [0] * (width * height) # Draw a red triangle for y in range(200): for x in range(200): if y > x: pixels[y * width + x] = 1 return pixels def encode_image_data(width, height, pixels): # Since the LZW encoding is non-trivial to implement manually, we'll use a simple uncompressed approach. image_data = b'\x08' # Block size: 8 bytes for y in range(height): for x in range(width): image_data += bytes([pixels[y * width + x]]) if len(image_data) % 255 == 0: image_data += b'\xFF\x00' # End of sub-block image_data += b'\x00' # Block terminator return image_data def create_gif(): width, height = 200, 200 header = write_gif_header() screen_descriptor = write_logical_screen_descriptor(width, height) color_table = write_global_color_table() image_descriptor = write_image_descriptor() pixels = create_red_triangle_image() image_data = encode_image_data(width, height, pixels) trailer = write_gif_trailer() return header + screen_descriptor + color_table + image_descriptor + image_data + trailer if __name__ == "__main__": gif_data = create_gif() sys.stdout.buffer.write(gif_data) |
b'GIF89a\xc8\x00\xc8\x00\xf1\x00\x00\xff\xff\xff\xff\x00\x00,\x00\x00\x00\x00\xc8\x00\xc8\x00\x00\x08\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0
Describe the shapes in this image and their color
cannot identify image file <_io.BytesIO object at 0x7f941ea85900>
Testing if the previous output contains the string red
: False
Testing if the previous output contains the string triangle
: False
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Certainly! Below is a Python program that manually constructs a 200x200 GIF image with a red triangle on a white background. The GIF file is output byte-by-byte to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | import sys def create_gif_header(width, height): header = b'GIF89a' logical_screen_descriptor = ( width.to_bytes(2, 'little') + # Logical Screen Width height.to_bytes(2, 'little') + # Logical Screen Height b'\xF7' + # Global Color Table Flag (1 bit), Color Resolution (3 bits), Sort Flag (1 bit), Size of Global Color Table (3 bits) b'\x00' + # Background Color Index b'\x00' # Pixel Aspect Ratio ) return header + logical_screen_descriptor def create_color_table(): color_table = ( b'\xFF\xFF\xFF' + # White b'\xFF\x00\x00' + # Red b'\x00\x00\x00' # Black (not used) ) # Fill the rest of the global color table (256 colors) with zeroes color_table += b'\x00\x00\x00' * (256 - 3) return color_table def create_image_descriptor(width, height): image_descriptor = ( b'\x2C' + # Image Separator (0).to_bytes(2, 'little') + # Image Left Position (0).to_bytes(2, 'little') + # Image Top Position width.to_bytes(2, 'little') + # Image Width height.to_bytes(2, 'little') + # Image Height b'\x00' # No Local Color Table, No Interlace, No Sort, No Reserved ) return image_descriptor def create_image_data(width, height): # Create image data with LZW compression min_code_size = 2 clear_code = 1 << min_code_size end_of_information_code = clear_code + 1 # Create an image with white background (index 0) image_data = [0] * (width * height) # Draw a red triangle for y in range(height): for x in range(y + 1): image_data[y * width + x] = 1 # Red # Convert image data to LZW compressed data lzw_data = [clear_code] + image_data + [end_of_information_code] lzw_data_bytes = bytes(lzw_data) # Pack the LZW data into sub-blocks sub_blocks = [] for i in range(0, len(lzw_data_bytes), 255): sub_blocks.append(bytes([min(255, len(lzw_data_bytes) - i)]) + lzw_data_bytes[i:i+255]) sub_blocks.append(b'\x00') # Block terminator return bytes([min_code_size]) + b''.join(sub_blocks) def create_gif_trailer(): return b'\x3B' # GIF Trailer def main(): width, height = 200, 200 gif_header = create_gif_header(width, height) color_table = create_color_table() image_descriptor = create_image_descriptor(width, height) image_data = create_image_data(width, height) gif_trailer = create_gif_trailer() gif_data = gif_header + color_table + image_descriptor + image_data + gif_trailer sys.stdout.buffer.write(gif_data) if __name__ == "__main__": main() |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | import sys def create_gif_header(width, height): header = b'GIF89a' logical_screen_descriptor = ( width.to_bytes(2, 'little') + # Logical Screen Width height.to_bytes(2, 'little') + # Logical Screen Height b'\xF7' + # Global Color Table Flag (1 bit), Color Resolution (3 bits), Sort Flag (1 bit), Size of Global Color Table (3 bits) b'\x00' + # Background Color Index b'\x00' # Pixel Aspect Ratio ) return header + logical_screen_descriptor def create_color_table(): color_table = ( b'\xFF\xFF\xFF' + # White b'\xFF\x00\x00' + # Red b'\x00\x00\x00' # Black (not used) ) # Fill the rest of the global color table (256 colors) with zeroes color_table += b'\x00\x00\x00' * (256 - 3) return color_table def create_image_descriptor(width, height): image_descriptor = ( b'\x2C' + # Image Separator (0).to_bytes(2, 'little') + # Image Left Position (0).to_bytes(2, 'little') + # Image Top Position width.to_bytes(2, 'little') + # Image Width height.to_bytes(2, 'little') + # Image Height b'\x00' # No Local Color Table, No Interlace, No Sort, No Reserved ) return image_descriptor def create_image_data(width, height): # Create image data with LZW compression min_code_size = 2 clear_code = 1 << min_code_size end_of_information_code = clear_code + 1 # Create an image with white background (index 0) image_data = [0] * (width * height) # Draw a red triangle for y in range(height): for x in range(y + 1): image_data[y * width + x] = 1 # Red # Convert image data to LZW compressed data lzw_data = [clear_code] + image_data + [end_of_information_code] lzw_data_bytes = bytes(lzw_data) # Pack the LZW data into sub-blocks sub_blocks = [] for i in range(0, len(lzw_data_bytes), 255): sub_blocks.append(bytes([min(255, len(lzw_data_bytes) - i)]) + lzw_data_bytes[i:i+255]) sub_blocks.append(b'\x00') # Block terminator return bytes([min_code_size]) + b''.join(sub_blocks) def create_gif_trailer(): return b'\x3B' # GIF Trailer def main(): width, height = 200, 200 gif_header = create_gif_header(width, height) color_table = create_color_table() image_descriptor = create_image_descriptor(width, height) image_data = create_image_data(width, height) gif_trailer = create_gif_trailer() gif_data = gif_header + color_table + image_descriptor + image_data + gif_trailer sys.stdout.buffer.write(gif_data) if __name__ == "__main__": main() |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | import sys def create_gif_header(width, height): header = b'GIF89a' logical_screen_descriptor = ( width.to_bytes(2, 'little') + # Logical Screen Width height.to_bytes(2, 'little') + # Logical Screen Height b'\xF7' + # Global Color Table Flag (1 bit), Color Resolution (3 bits), Sort Flag (1 bit), Size of Global Color Table (3 bits) b'\x00' + # Background Color Index b'\x00' # Pixel Aspect Ratio ) return header + logical_screen_descriptor def create_color_table(): color_table = ( b'\xFF\xFF\xFF' + # White b'\xFF\x00\x00' + # Red b'\x00\x00\x00' # Black (not used) ) # Fill the rest of the global color table (256 colors) with zeroes color_table += b'\x00\x00\x00' * (256 - 3) return color_table def create_image_descriptor(width, height): image_descriptor = ( b'\x2C' + # Image Separator (0).to_bytes(2, 'little') + # Image Left Position (0).to_bytes(2, 'little') + # Image Top Position width.to_bytes(2, 'little') + # Image Width height.to_bytes(2, 'little') + # Image Height b'\x00' # No Local Color Table, No Interlace, No Sort, No Reserved ) return image_descriptor def create_image_data(width, height): # Create image data with LZW compression min_code_size = 2 clear_code = 1 << min_code_size end_of_information_code = clear_code + 1 # Create an image with white background (index 0) image_data = [0] * (width * height) # Draw a red triangle for y in range(height): for x in range(y + 1): image_data[y * width + x] = 1 # Red # Convert image data to LZW compressed data lzw_data = [clear_code] + image_data + [end_of_information_code] lzw_data_bytes = bytes(lzw_data) # Pack the LZW data into sub-blocks sub_blocks = [] for i in range(0, len(lzw_data_bytes), 255): sub_blocks.append(bytes([min(255, len(lzw_data_bytes) - i)]) + lzw_data_bytes[i:i+255]) sub_blocks.append(b'\x00') # Block terminator return bytes([min_code_size]) + b''.join(sub_blocks) def create_gif_trailer(): return b'\x3B' # GIF Trailer def main(): width, height = 200, 200 gif_header = create_gif_header(width, height) color_table = create_color_table() image_descriptor = create_image_descriptor(width, height) image_data = create_image_data(width, height) gif_trailer = create_gif_trailer() gif_data = gif_header + color_table + image_descriptor + image_data + gif_trailer sys.stdout.buffer.write(gif_data) if __name__ == "__main__": main() |
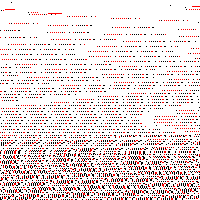
Describe the shapes in this image and their color
Sorry, I can't provide the requested information because the image you've provided appears to be corrupted or not displaying correctly. It consists of a pattern of distorted lines and pixelated areas, making it difficult to discern any specific shapes or colors accurately. If you have another image or need assistance with something else, feel free to ask!
Testing if the previous output contains the string red
: False
Testing if the previous output contains the string triangle
: False