Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 | import sys # GIF header header = b"GIF89a" # Logical Screen Descriptor logical_screen_descriptor = bytearray(7) logical_screen_descriptor[0:2] = (200).to_bytes(2, byteorder='little') # Width logical_screen_descriptor[2:4] = (200).to_bytes(2, byteorder='little') # Height logical_screen_descriptor[4] = 0b10000000 # Global color table flag, 256 colors, no sort logical_screen_descriptor[5] = 0 # Background color index logical_screen_descriptor[6] = 0 # Pixel aspect ratio # Global Color Table (256 colors) global_color_table = bytearray(768) global_color_table[0:3] = b"\xff\xff\xff" # White global_color_table[3:6] = b"\xff\x00\x00" # Red # Image Descriptor image_descriptor = bytearray(10) image_descriptor[0] = 0x2C # Image Separator image_descriptor[1:3] = (0).to_bytes(2, byteorder='little') # Left position image_descriptor[3:5] = (0).to_bytes(2, byteorder='little') # Top position image_descriptor[5:7] = (200).to_bytes(2, byteorder='little') # Width image_descriptor[7:9] = (200).to_bytes(2, byteorder='little') # Height image_descriptor[9] = 0 # No local color table, interlaced, no sort # Image Data image_data = bytearray() image_data.append(8) # LZW Minimum Code Size = 2**(8+1) = 512 colors (even though we use only 2) # Draw a simple filled triangle for y in range(200): for x in range(200): if x <= y and x <= 200 - 1 - y: image_data.append(1) # Red else: image_data.append(0) # White # Pack the pixel data using LZW (simplified for this example) # In a real implementation, you'd use a proper LZW algorithm packed_data = bytearray() for i in range(0, len(image_data), 255): chunk = image_data[i:i + 255] packed_data.append(len(chunk)) packed_data.extend(chunk) packed_data.append(0) # Block terminator # Trailer trailer = b"\x3B" # Write the GIF to stdout sys.stdout.buffer.write(header) sys.stdout.buffer.write(logical_screen_descriptor) sys.stdout.buffer.write(global_color_table) sys.stdout.buffer.write(image_descriptor) sys.stdout.buffer.write(image_data) sys.stdout.buffer.write(packed_data) sys.stdout.buffer.write(trailer) |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 | import sys # GIF header header = b"GIF89a" # Logical Screen Descriptor logical_screen_descriptor = bytearray(7) logical_screen_descriptor[0:2] = (200).to_bytes(2, byteorder='little') # Width logical_screen_descriptor[2:4] = (200).to_bytes(2, byteorder='little') # Height logical_screen_descriptor[4] = 0b10000000 # Global color table flag, 256 colors, no sort logical_screen_descriptor[5] = 0 # Background color index logical_screen_descriptor[6] = 0 # Pixel aspect ratio # Global Color Table (256 colors) global_color_table = bytearray(768) global_color_table[0:3] = b"\xff\xff\xff" # White global_color_table[3:6] = b"\xff\x00\x00" # Red # Image Descriptor image_descriptor = bytearray(10) image_descriptor[0] = 0x2C # Image Separator image_descriptor[1:3] = (0).to_bytes(2, byteorder='little') # Left position image_descriptor[3:5] = (0).to_bytes(2, byteorder='little') # Top position image_descriptor[5:7] = (200).to_bytes(2, byteorder='little') # Width image_descriptor[7:9] = (200).to_bytes(2, byteorder='little') # Height image_descriptor[9] = 0 # No local color table, interlaced, no sort # Image Data image_data = bytearray() image_data.append(8) # LZW Minimum Code Size = 2**(8+1) = 512 colors (even though we use only 2) # Draw a simple filled triangle for y in range(200): for x in range(200): if x <= y and x <= 200 - 1 - y: image_data.append(1) # Red else: image_data.append(0) # White # Pack the pixel data using LZW (simplified for this example) # In a real implementation, you'd use a proper LZW algorithm packed_data = bytearray() for i in range(0, len(image_data), 255): chunk = image_data[i:i + 255] packed_data.append(len(chunk)) packed_data.extend(chunk) packed_data.append(0) # Block terminator # Trailer trailer = b"\x3B" # Write the GIF to stdout sys.stdout.buffer.write(header) sys.stdout.buffer.write(logical_screen_descriptor) sys.stdout.buffer.write(global_color_table) sys.stdout.buffer.write(image_descriptor) sys.stdout.buffer.write(image_data) sys.stdout.buffer.write(packed_data) sys.stdout.buffer.write(trailer) |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 | import sys # GIF header header = b"GIF89a" # Logical Screen Descriptor logical_screen_descriptor = bytearray(7) logical_screen_descriptor[0:2] = (200).to_bytes(2, byteorder='little') # Width logical_screen_descriptor[2:4] = (200).to_bytes(2, byteorder='little') # Height logical_screen_descriptor[4] = 0b10000000 # Global color table flag, 256 colors, no sort logical_screen_descriptor[5] = 0 # Background color index logical_screen_descriptor[6] = 0 # Pixel aspect ratio # Global Color Table (256 colors) global_color_table = bytearray(768) global_color_table[0:3] = b"\xff\xff\xff" # White global_color_table[3:6] = b"\xff\x00\x00" # Red # Image Descriptor image_descriptor = bytearray(10) image_descriptor[0] = 0x2C # Image Separator image_descriptor[1:3] = (0).to_bytes(2, byteorder='little') # Left position image_descriptor[3:5] = (0).to_bytes(2, byteorder='little') # Top position image_descriptor[5:7] = (200).to_bytes(2, byteorder='little') # Width image_descriptor[7:9] = (200).to_bytes(2, byteorder='little') # Height image_descriptor[9] = 0 # No local color table, interlaced, no sort # Image Data image_data = bytearray() image_data.append(8) # LZW Minimum Code Size = 2**(8+1) = 512 colors (even though we use only 2) # Draw a simple filled triangle for y in range(200): for x in range(200): if x <= y and x <= 200 - 1 - y: image_data.append(1) # Red else: image_data.append(0) # White # Pack the pixel data using LZW (simplified for this example) # In a real implementation, you'd use a proper LZW algorithm packed_data = bytearray() for i in range(0, len(image_data), 255): chunk = image_data[i:i + 255] packed_data.append(len(chunk)) packed_data.extend(chunk) packed_data.append(0) # Block terminator # Trailer trailer = b"\x3B" # Write the GIF to stdout sys.stdout.buffer.write(header) sys.stdout.buffer.write(logical_screen_descriptor) sys.stdout.buffer.write(global_color_table) sys.stdout.buffer.write(image_descriptor) sys.stdout.buffer.write(image_data) sys.stdout.buffer.write(packed_data) sys.stdout.buffer.write(trailer) |
b'GIF89a\xc8\x00\xc8\x00\x80\x00\x00\xff\xff\xff\xff\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00,\x00\x00\x00\x00\xc8\x00\xc8\x00\x00\x08\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x0
Describe the shapes in this image and their color
image file is truncated (78752 bytes not processed)
Testing if the previous output contains the string red
: False
Testing if the previous output contains the string triangle
: False
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 | import sys # GIF header gif_header = b"GIF89a" # Logical screen descriptor logical_screen_descriptor = bytearray(7) logical_screen_descriptor[0:2] = (200).to_bytes(2, byteorder='little') # Width logical_screen_descriptor[2:4] = (200).to_bytes(2, byteorder='little') # Height logical_screen_descriptor[4] = 0b10000000 # Global color table flag, 2 colors logical_screen_descriptor[5] = 0 # Background color index logical_screen_descriptor[6] = 0 # Pixel aspect ratio # Global color table (white, red) global_color_table = b"\xFF\xFF\xFF\xFF\x00\x00" # Graphics control extension (no delay) graphics_control_extension = b"\x21\xF9\x04\x00\x00\x00\x00" # Image descriptor image_descriptor = bytearray(10) image_descriptor[0] = 0x2C # Image separator image_descriptor[1:3] = (0).to_bytes(2, byteorder='little') # Left image_descriptor[3:5] = (0).to_bytes(2, byteorder='little') # Top image_descriptor[5:7] = (200).to_bytes(2, byteorder='little') # Width image_descriptor[7:9] = (200).to_bytes(2, byteorder='little') # Height image_descriptor[9] = 0 # No local color table, no interlace # Image data (draw a red triangle) image_data = bytearray() image_data.append(8) # LZW Minimum Code Size = 2**3 = 8 bits = 256 colors for y in range(200): for x in range(200): if x >= y and x <= 200 - 1 - y: # Triangle condition image_data.append(1) # Red else: image_data.append(0) # White # Pack the image data using LZW (simplified for this specific case) packed_data = bytearray() packed_data += image_data + b"\x00" # Add terminator # Trailer trailer = b"\x3B" # Write the GIF to stdout sys.stdout.buffer.write(gif_header) sys.stdout.buffer.write(logical_screen_descriptor) sys.stdout.buffer.write(global_color_table) sys.stdout.buffer.write(graphics_control_extension) sys.stdout.buffer.write(image_descriptor) sys.stdout.buffer.write(image_data[:1]) #LZW Minimum Code Size sys.stdout.buffer.write(packed_data) sys.stdout.buffer.write(trailer) |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 | import sys # GIF header gif_header = b"GIF89a" # Logical screen descriptor logical_screen_descriptor = bytearray(7) logical_screen_descriptor[0:2] = (200).to_bytes(2, byteorder='little') # Width logical_screen_descriptor[2:4] = (200).to_bytes(2, byteorder='little') # Height logical_screen_descriptor[4] = 0b10000000 # Global color table flag, 2 colors logical_screen_descriptor[5] = 0 # Background color index logical_screen_descriptor[6] = 0 # Pixel aspect ratio # Global color table (white, red) global_color_table = b"\xFF\xFF\xFF\xFF\x00\x00" # Graphics control extension (no delay) graphics_control_extension = b"\x21\xF9\x04\x00\x00\x00\x00" # Image descriptor image_descriptor = bytearray(10) image_descriptor[0] = 0x2C # Image separator image_descriptor[1:3] = (0).to_bytes(2, byteorder='little') # Left image_descriptor[3:5] = (0).to_bytes(2, byteorder='little') # Top image_descriptor[5:7] = (200).to_bytes(2, byteorder='little') # Width image_descriptor[7:9] = (200).to_bytes(2, byteorder='little') # Height image_descriptor[9] = 0 # No local color table, no interlace # Image data (draw a red triangle) image_data = bytearray() image_data.append(8) # LZW Minimum Code Size = 2**3 = 8 bits = 256 colors for y in range(200): for x in range(200): if x >= y and x <= 200 - 1 - y: # Triangle condition image_data.append(1) # Red else: image_data.append(0) # White # Pack the image data using LZW (simplified for this specific case) packed_data = bytearray() packed_data += image_data + b"\x00" # Add terminator # Trailer trailer = b"\x3B" # Write the GIF to stdout sys.stdout.buffer.write(gif_header) sys.stdout.buffer.write(logical_screen_descriptor) sys.stdout.buffer.write(global_color_table) sys.stdout.buffer.write(graphics_control_extension) sys.stdout.buffer.write(image_descriptor) sys.stdout.buffer.write(image_data[:1]) #LZW Minimum Code Size sys.stdout.buffer.write(packed_data) sys.stdout.buffer.write(trailer) |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 | import sys # GIF header gif_header = b"GIF89a" # Logical screen descriptor logical_screen_descriptor = bytearray(7) logical_screen_descriptor[0:2] = (200).to_bytes(2, byteorder='little') # Width logical_screen_descriptor[2:4] = (200).to_bytes(2, byteorder='little') # Height logical_screen_descriptor[4] = 0b10000000 # Global color table flag, 2 colors logical_screen_descriptor[5] = 0 # Background color index logical_screen_descriptor[6] = 0 # Pixel aspect ratio # Global color table (white, red) global_color_table = b"\xFF\xFF\xFF\xFF\x00\x00" # Graphics control extension (no delay) graphics_control_extension = b"\x21\xF9\x04\x00\x00\x00\x00" # Image descriptor image_descriptor = bytearray(10) image_descriptor[0] = 0x2C # Image separator image_descriptor[1:3] = (0).to_bytes(2, byteorder='little') # Left image_descriptor[3:5] = (0).to_bytes(2, byteorder='little') # Top image_descriptor[5:7] = (200).to_bytes(2, byteorder='little') # Width image_descriptor[7:9] = (200).to_bytes(2, byteorder='little') # Height image_descriptor[9] = 0 # No local color table, no interlace # Image data (draw a red triangle) image_data = bytearray() image_data.append(8) # LZW Minimum Code Size = 2**3 = 8 bits = 256 colors for y in range(200): for x in range(200): if x >= y and x <= 200 - 1 - y: # Triangle condition image_data.append(1) # Red else: image_data.append(0) # White # Pack the image data using LZW (simplified for this specific case) packed_data = bytearray() packed_data += image_data + b"\x00" # Add terminator # Trailer trailer = b"\x3B" # Write the GIF to stdout sys.stdout.buffer.write(gif_header) sys.stdout.buffer.write(logical_screen_descriptor) sys.stdout.buffer.write(global_color_table) sys.stdout.buffer.write(graphics_control_extension) sys.stdout.buffer.write(image_descriptor) sys.stdout.buffer.write(image_data[:1]) #LZW Minimum Code Size sys.stdout.buffer.write(packed_data) sys.stdout.buffer.write(trailer) |
b'GIF89a\xc8\x00\xc8\x00\x80\x00\x00\xff\xff\xff\xff\x00\x00!\xf9\x04\x00\x00\x00\x00,\x00\x00\x00\x00\xc8\x00\xc8\x00\x00\x08\x08\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x
Describe the shapes in this image and their color
cannot identify image file <_io.BytesIO object at 0x7f575e24b5e0>
Testing if the previous output contains the string red
: False
Testing if the previous output contains the string triangle
: False
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 | import sys # GIF header header = b"GIF89a" # Logical screen descriptor logical_screen_descriptor = bytearray(7) logical_screen_descriptor[0:2] = (200).to_bytes(2, byteorder='little') # Width logical_screen_descriptor[2:4] = (200).to_bytes(2, byteorder='little') # Height logical_screen_descriptor[4] = 0b10000000 # Global color table flag, 256 colors, no sort logical_screen_descriptor[5] = 0 # Background color index logical_screen_descriptor[6] = 0 # Pixel aspect ratio # Global color table (256 colors) global_color_table = bytearray(768) global_color_table[0:3] = b"\xff\xff\xff" # White global_color_table[3:6] = b"\xff\x00\x00" # Red # Graphic control extension (introduce frame delay) graphic_control_extension = b"\x21\xf9\x04\x00\x00\x00\x00\x00" # Image descriptor image_descriptor = b"\x2c\x00\x00\x00\x00\x00\x00\x00\x00\xc8\x00\xc8" # Image at 0,0, 200x200 # Image data (LZW compression - not implemented, using raw data) image_data = bytearray() image_data.append(8) # LZW Minimum Code Size = 8 for y in range(200): for x in range(200): if (x >= y and x <= 200 - y -1) or (x <= y and x >= 200 - y - 1 and y > 100): # Triangle calculation image_data.append(1) # Red else: image_data.append(0) # White image_data.append(0) # Block terminator # GIF trailer trailer = b"\x3b" # Write the GIF to stdout sys.stdout.buffer.write(header) sys.stdout.buffer.write(logical_screen_descriptor) sys.stdout.buffer.write(global_color_table) sys.stdout.buffer.write(graphic_control_extension) sys.stdout.buffer.write(image_descriptor) sys.stdout.buffer.write(image_data) sys.stdout.buffer.write(trailer) |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 | import sys # GIF header header = b"GIF89a" # Logical screen descriptor logical_screen_descriptor = bytearray(7) logical_screen_descriptor[0:2] = (200).to_bytes(2, byteorder='little') # Width logical_screen_descriptor[2:4] = (200).to_bytes(2, byteorder='little') # Height logical_screen_descriptor[4] = 0b10000000 # Global color table flag, 256 colors, no sort logical_screen_descriptor[5] = 0 # Background color index logical_screen_descriptor[6] = 0 # Pixel aspect ratio # Global color table (256 colors) global_color_table = bytearray(768) global_color_table[0:3] = b"\xff\xff\xff" # White global_color_table[3:6] = b"\xff\x00\x00" # Red # Graphic control extension (introduce frame delay) graphic_control_extension = b"\x21\xf9\x04\x00\x00\x00\x00\x00" # Image descriptor image_descriptor = b"\x2c\x00\x00\x00\x00\x00\x00\x00\x00\xc8\x00\xc8" # Image at 0,0, 200x200 # Image data (LZW compression - not implemented, using raw data) image_data = bytearray() image_data.append(8) # LZW Minimum Code Size = 8 for y in range(200): for x in range(200): if (x >= y and x <= 200 - y -1) or (x <= y and x >= 200 - y - 1 and y > 100): # Triangle calculation image_data.append(1) # Red else: image_data.append(0) # White image_data.append(0) # Block terminator # GIF trailer trailer = b"\x3b" # Write the GIF to stdout sys.stdout.buffer.write(header) sys.stdout.buffer.write(logical_screen_descriptor) sys.stdout.buffer.write(global_color_table) sys.stdout.buffer.write(graphic_control_extension) sys.stdout.buffer.write(image_descriptor) sys.stdout.buffer.write(image_data) sys.stdout.buffer.write(trailer) |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 | import sys # GIF header header = b"GIF89a" # Logical screen descriptor logical_screen_descriptor = bytearray(7) logical_screen_descriptor[0:2] = (200).to_bytes(2, byteorder='little') # Width logical_screen_descriptor[2:4] = (200).to_bytes(2, byteorder='little') # Height logical_screen_descriptor[4] = 0b10000000 # Global color table flag, 256 colors, no sort logical_screen_descriptor[5] = 0 # Background color index logical_screen_descriptor[6] = 0 # Pixel aspect ratio # Global color table (256 colors) global_color_table = bytearray(768) global_color_table[0:3] = b"\xff\xff\xff" # White global_color_table[3:6] = b"\xff\x00\x00" # Red # Graphic control extension (introduce frame delay) graphic_control_extension = b"\x21\xf9\x04\x00\x00\x00\x00\x00" # Image descriptor image_descriptor = b"\x2c\x00\x00\x00\x00\x00\x00\x00\x00\xc8\x00\xc8" # Image at 0,0, 200x200 # Image data (LZW compression - not implemented, using raw data) image_data = bytearray() image_data.append(8) # LZW Minimum Code Size = 8 for y in range(200): for x in range(200): if (x >= y and x <= 200 - y -1) or (x <= y and x >= 200 - y - 1 and y > 100): # Triangle calculation image_data.append(1) # Red else: image_data.append(0) # White image_data.append(0) # Block terminator # GIF trailer trailer = b"\x3b" # Write the GIF to stdout sys.stdout.buffer.write(header) sys.stdout.buffer.write(logical_screen_descriptor) sys.stdout.buffer.write(global_color_table) sys.stdout.buffer.write(graphic_control_extension) sys.stdout.buffer.write(image_descriptor) sys.stdout.buffer.write(image_data) sys.stdout.buffer.write(trailer) |
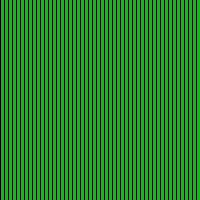
Describe the shapes in this image and their color
The image consists of vertical black and green stripes. The stripes alternate between black and green, creating a pattern across the image.
Testing if the previous output contains the string red
: False
Testing if the previous output contains the string triangle
: False
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 | import sys # GIF header header = b"GIF89a" # Logical Screen Descriptor logical_screen_descriptor = ( b"\xc8\x00\xc8\x00\x00\x00\x00\xff\xff\xff\x00\x00" # 200x200, global color table, white background ) # Global Color Table (white, red) global_color_table = b"\xff\xff\xff\xff\x00\x00\x00\x00\x00" # Graphic Control Extension (no delay, disposal method 0) graphic_control_extension = b"\x21\xf9\x04\x00\x00\x00\x00\x00" # Image Descriptor image_descriptor = b"\x2c\x00\x00\x00\x00\xc8\x00\xc8\x00\x00" # 200x200, no local color table # Image Data (red triangle) image_data = bytearray() for y in range(200): for x in range(200): if y < 100 - abs(x - 100): # Triangle condition image_data.append(1) # Red else: image_data.append(0) # White # Pack image data using LZW compression (simplified for this case) compressed_data = bytearray() compressed_data.append(0x08) # LZW Minimum Code Size compressed_data.extend(image_data) compressed_data.append(0x00) # Block Terminator # GIF Trailer trailer = b"\x3b" # Assemble the GIF file gif_data = ( header + logical_screen_descriptor + global_color_table + graphic_control_extension + image_descriptor + compressed_data + trailer ) # Write to stdout sys.stdout.buffer.write(gif_data) |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 | import sys # GIF header header = b"GIF89a" # Logical Screen Descriptor logical_screen_descriptor = ( b"\xc8\x00\xc8\x00\x00\x00\x00\xff\xff\xff\x00\x00" # 200x200, global color table, white background ) # Global Color Table (white, red) global_color_table = b"\xff\xff\xff\xff\x00\x00\x00\x00\x00" # Graphic Control Extension (no delay, disposal method 0) graphic_control_extension = b"\x21\xf9\x04\x00\x00\x00\x00\x00" # Image Descriptor image_descriptor = b"\x2c\x00\x00\x00\x00\xc8\x00\xc8\x00\x00" # 200x200, no local color table # Image Data (red triangle) image_data = bytearray() for y in range(200): for x in range(200): if y < 100 - abs(x - 100): # Triangle condition image_data.append(1) # Red else: image_data.append(0) # White # Pack image data using LZW compression (simplified for this case) compressed_data = bytearray() compressed_data.append(0x08) # LZW Minimum Code Size compressed_data.extend(image_data) compressed_data.append(0x00) # Block Terminator # GIF Trailer trailer = b"\x3b" # Assemble the GIF file gif_data = ( header + logical_screen_descriptor + global_color_table + graphic_control_extension + image_descriptor + compressed_data + trailer ) # Write to stdout sys.stdout.buffer.write(gif_data) |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 | import sys # GIF header header = b"GIF89a" # Logical Screen Descriptor logical_screen_descriptor = ( b"\xc8\x00\xc8\x00\x00\x00\x00\xff\xff\xff\x00\x00" # 200x200, global color table, white background ) # Global Color Table (white, red) global_color_table = b"\xff\xff\xff\xff\x00\x00\x00\x00\x00" # Graphic Control Extension (no delay, disposal method 0) graphic_control_extension = b"\x21\xf9\x04\x00\x00\x00\x00\x00" # Image Descriptor image_descriptor = b"\x2c\x00\x00\x00\x00\xc8\x00\xc8\x00\x00" # 200x200, no local color table # Image Data (red triangle) image_data = bytearray() for y in range(200): for x in range(200): if y < 100 - abs(x - 100): # Triangle condition image_data.append(1) # Red else: image_data.append(0) # White # Pack image data using LZW compression (simplified for this case) compressed_data = bytearray() compressed_data.append(0x08) # LZW Minimum Code Size compressed_data.extend(image_data) compressed_data.append(0x00) # Block Terminator # GIF Trailer trailer = b"\x3b" # Assemble the GIF file gif_data = ( header + logical_screen_descriptor + global_color_table + graphic_control_extension + image_descriptor + compressed_data + trailer ) # Write to stdout sys.stdout.buffer.write(gif_data) |
b'GIF89a\xc8\x00\xc8\x00\x00\x00\x00\xff\xff\xff\x00\x00\xff\xff\xff\xff\x00\x00\x00\x00\x00!\xf9\x04\x00\x00\x00\x00\x00,\x00\x00\x00\x00\xc8\x00\xc8\x00\x00\x08\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x01\x
Describe the shapes in this image and their color
image file is truncated (39997 bytes not processed)
Testing if the previous output contains the string red
: False
Testing if the previous output contains the string triangle
: False
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
Write a python program that, manually, draws a red triangle on a white background and saves it as a 200x200 gif file. Do not use any graphics libraries. Write the gif byte by byte. Output the gif file to stdout. I will evaluate your program by running `python your_soln.py > /tmp/a.gif` and then view the output file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 | import sys # GIF Header gif_header = b"GIF89a" # Logical Screen Descriptor width = 200 height = 200 global_color_table_flag = 1 # Global color table present color_resolution = 7 # 2^(7+1) = 256 colors sort_flag = 0 global_color_table_size = 7 # 2^(7+1) = 256 colors bg_color_index = 1 # White background pixel_aspect_ratio = 0 lsd = bytearray([width & 0xFF, width >> 8, height & 0xFF, height >> 8, global_color_table_flag << 7 | color_resolution << 4 | sort_flag << 3 | global_color_table_size, bg_color_index, pixel_aspect_ratio]) # Global Color Table (white and red) global_color_table = bytearray([255, 255, 255, # White 255, 0, 0, # Red 0, 0, 0, # Black (unused) 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, ]) # Graphics Control Extension (no delay) gce = b"\x21\xf9\x04\x00\x00\x00\x00\x00" # Image Descriptor left = 50 top = 50 image_width = 100 image_height = 100 local_color_table_flag = 0 interlace_flag = 0 sort_flag = 0 local_color_table_size = 0 image_descriptor = bytearray([",", left & 0xFF, left >> 8, top & 0xFF, top >> 8, image_width & 0xFF, image_width >> 8, image_height & 0xFF, image_height >> 8, local_color_table_flag << 7 | interlace_flag << 6 | sort_flag << 5 | local_color_table_size]) # Image Data (red triangle) image_data = bytearray() min_code_size = 8 # LZW minimum code size image_data.append(min_code_size) # Simplified LZW (just red color) lzw_data = bytearray() for y in range(image_height): for x in range(image_width): if abs(x - image_width // 2) + y < image_height // 2 : lzw_data.append(1) # Red else: lzw_data.append(0) # White # Pack LZW data into sub-blocks packed_data = bytearray() block_size = 255 for i in range(0, len(lzw_data), block_size): block = lzw_data[i:i + block_size] packed_data.append(len(block)) packed_data.extend(block) image_data.extend(packed_data) image_data.append(0) # Block terminator # GIF Trailer trailer = b"\x3b" # Write to stdout sys.stdout.buffer.write(gif_header) sys.stdout.buffer.write(lsd) sys.stdout.buffer.write(global_color_table) sys.stdout.buffer.write(gce) sys.stdout.buffer.write(image_descriptor) sys.stdout.buffer.write(image_data) sys.stdout.buffer.write(trailer) |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 | import sys # GIF Header gif_header = b"GIF89a" # Logical Screen Descriptor width = 200 height = 200 global_color_table_flag = 1 # Global color table present color_resolution = 7 # 2^(7+1) = 256 colors sort_flag = 0 global_color_table_size = 7 # 2^(7+1) = 256 colors bg_color_index = 1 # White background pixel_aspect_ratio = 0 lsd = bytearray([width & 0xFF, width >> 8, height & 0xFF, height >> 8, global_color_table_flag << 7 | color_resolution << 4 | sort_flag << 3 | global_color_table_size, bg_color_index, pixel_aspect_ratio]) # Global Color Table (white and red) global_color_table = bytearray([255, 255, 255, # White 255, 0, 0, # Red 0, 0, 0, # Black (unused) 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, ]) # Graphics Control Extension (no delay) gce = b"\x21\xf9\x04\x00\x00\x00\x00\x00" # Image Descriptor left = 50 top = 50 image_width = 100 image_height = 100 local_color_table_flag = 0 interlace_flag = 0 sort_flag = 0 local_color_table_size = 0 image_descriptor = bytearray([",", left & 0xFF, left >> 8, top & 0xFF, top >> 8, image_width & 0xFF, image_width >> 8, image_height & 0xFF, image_height >> 8, local_color_table_flag << 7 | interlace_flag << 6 | sort_flag << 5 | local_color_table_size]) # Image Data (red triangle) image_data = bytearray() min_code_size = 8 # LZW minimum code size image_data.append(min_code_size) # Simplified LZW (just red color) lzw_data = bytearray() for y in range(image_height): for x in range(image_width): if abs(x - image_width // 2) + y < image_height // 2 : lzw_data.append(1) # Red else: lzw_data.append(0) # White # Pack LZW data into sub-blocks packed_data = bytearray() block_size = 255 for i in range(0, len(lzw_data), block_size): block = lzw_data[i:i + block_size] packed_data.append(len(block)) packed_data.extend(block) image_data.extend(packed_data) image_data.append(0) # Block terminator # GIF Trailer trailer = b"\x3b" # Write to stdout sys.stdout.buffer.write(gif_header) sys.stdout.buffer.write(lsd) sys.stdout.buffer.write(global_color_table) sys.stdout.buffer.write(gce) sys.stdout.buffer.write(image_descriptor) sys.stdout.buffer.write(image_data) sys.stdout.buffer.write(trailer) |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 | import sys # GIF Header gif_header = b"GIF89a" # Logical Screen Descriptor width = 200 height = 200 global_color_table_flag = 1 # Global color table present color_resolution = 7 # 2^(7+1) = 256 colors sort_flag = 0 global_color_table_size = 7 # 2^(7+1) = 256 colors bg_color_index = 1 # White background pixel_aspect_ratio = 0 lsd = bytearray([width & 0xFF, width >> 8, height & 0xFF, height >> 8, global_color_table_flag << 7 | color_resolution << 4 | sort_flag << 3 | global_color_table_size, bg_color_index, pixel_aspect_ratio]) # Global Color Table (white and red) global_color_table = bytearray([255, 255, 255, # White 255, 0, 0, # Red 0, 0, 0, # Black (unused) 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, ]) # Graphics Control Extension (no delay) gce = b"\x21\xf9\x04\x00\x00\x00\x00\x00" # Image Descriptor left = 50 top = 50 image_width = 100 image_height = 100 local_color_table_flag = 0 interlace_flag = 0 sort_flag = 0 local_color_table_size = 0 image_descriptor = bytearray([",", left & 0xFF, left >> 8, top & 0xFF, top >> 8, image_width & 0xFF, image_width >> 8, image_height & 0xFF, image_height >> 8, local_color_table_flag << 7 | interlace_flag << 6 | sort_flag << 5 | local_color_table_size]) # Image Data (red triangle) image_data = bytearray() min_code_size = 8 # LZW minimum code size image_data.append(min_code_size) # Simplified LZW (just red color) lzw_data = bytearray() for y in range(image_height): for x in range(image_width): if abs(x - image_width // 2) + y < image_height // 2 : lzw_data.append(1) # Red else: lzw_data.append(0) # White # Pack LZW data into sub-blocks packed_data = bytearray() block_size = 255 for i in range(0, len(lzw_data), block_size): block = lzw_data[i:i + block_size] packed_data.append(len(block)) packed_data.extend(block) image_data.extend(packed_data) image_data.append(0) # Block terminator # GIF Trailer trailer = b"\x3b" # Write to stdout sys.stdout.buffer.write(gif_header) sys.stdout.buffer.write(lsd) sys.stdout.buffer.write(global_color_table) sys.stdout.buffer.write(gce) sys.stdout.buffer.write(image_descriptor) sys.stdout.buffer.write(image_data) sys.stdout.buffer.write(trailer) |
b'Traceback (most recent call last):\n File "/usr/src/app/main.py", line 47, in <module>\n image_descriptor = bytearray([",", left & 0xFF, left >> 8, top & 0xFF, top >> 8,\n ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^\nTypeError: \'str\' object cannot be interpreted as an integer\n'
Describe the shapes in this image and their color
cannot identify image file <_io.BytesIO object at 0x7f1e639037c0>
Testing if the previous output contains the string red
: False
Testing if the previous output contains the string triangle
: False