Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Below is one complete solution. This program writes a 24‐bit BMP image of an American flag to standard output. In this example the flag is drawn at a 10:19 ratio (height 130 pixels, width 247 pixels), with 13 horizontal stripes (each 10 pixels tall) and a “union” (the blue rectangle) drawn in the top‐left covering the top 7 stripes in height and about 2⁄5 of the flag’s length (here 99 pixels wide). (On Windows you may need to set stdout into binary mode.) Compile and run (redirecting stdout to a “.bmp” file) to get your BMP file. For example: gcc flag.c –o flag ./flag > flag.bmp Here is the complete code: ------------------------------------------------ /* flag.c – Write a BMP American flag to stdout */ #include <stdio.h> #include <stdlib.h> #ifdef _WIN32 #include <fcntl.h> #include <io.h> #endif int main(void) { /* On Windows, set stdout to binary mode */ #ifdef _WIN32 _setmode(_fileno(stdout), _O_BINARY); #endif /* Dimensions for the flag. We choose: Flag height = 130 pixels (13 stripes × 10 pixels each), Flag width = 247 pixels (to give a 10:19 ratio), Union (blue) covers the top 70 pixels (7 stripes) in height, and 99 pixels in width (~2/5 of 247). */ const int width = 247; const int height = 130; const int stripe_height = 10; const int union_height = 70; const int union_width = 99; /* Each pixel is 3 bytes, and each scanline (row) must be padded to a 4‐byte boundary. The padded row length in bytes is computed as follows: */ const int row_stride = ((width * 3 + 3) / 4) * 4; const int pixel_data_size = row_stride * height; const int file_size = 14 + 40 + pixel_data_size; /* 14‐byte file header + 40‐byte DIB header */ /* --- BMP File Header (14 bytes) --- */ unsigned char bmp_file_header[14] = {0}; bmp_file_header[0] = 'B'; bmp_file_header[1] = 'M'; bmp_file_header[2] = file_size & 0xFF; bmp_file_header[3] = (file_size >> 8) & 0xFF; bmp_file_header[4] = (file_size >> 16) & 0xFF; bmp_file_header[5] = (file_size >> 24) & 0xFF; /* bytes 6–9: reserved (set to 0) */ int pixel_data_offset = 14 + 40; /* header sizes */ bmp_file_header[10] = pixel_data_offset & 0xFF; bmp_file_header[11] = (pixel_data_offset >> 8) & 0xFF; bmp_file_header[12] = (pixel_data_offset >> 16) & 0xFF; bmp_file_header[13] = (pixel_data_offset >> 24) & 0xFF; /* --- DIB Header (BITMAPINFOHEADER, 40 bytes) --- */ unsigned char bmp_info_header[40] = {0}; bmp_info_header[0] = 40; /* header size (40 bytes) */ bmp_info_header[4] = width & 0xFF; bmp_info_header[5] = (width >> 8) & 0xFF; bmp_info_header[6] = (width >> 16) & 0xFF; bmp_info_header[7] = (width >> 24) & 0xFF; bmp_info_header[8] = height & 0xFF; bmp_info_header[9] = (height >> 8) & 0xFF; bmp_info_header[10] = (height >> 16) & 0xFF; bmp_info_header[11] = (height >> 24) & 0xFF; bmp_info_header[12] = 1; /* color planes */ bmp_info_header[13] = 0; bmp_info_header[14] = 24; /* bits per pixel (24-bit BMP) */ bmp_info_header[15] = 0; /* The remainder (compression, image size, etc.) can be left as 0. */ /* Write headers to stdout */ fwrite(bmp_file_header, 1, 14, stdout); fwrite(bmp_info_header, 1, 40, stdout); /* Allocate buffer for one row (scanline) of pixel data */ unsigned char *row = malloc(row_stride); if (!row) { fprintf(stderr, "Memory allocation error\n"); return 1; } /* Draw the flag. Note: In BMP the pixel rows go from bottom to top. We define row_from_top = (height - 1 - y) so that 0 is the top row. The top 7 stripes (rows 0 to 69) get the blue union on the left (x < union_width). The stripes alternate red and white: Even-numbered stripe (from the top) = red, odd-numbered = white. Colors are stored in BGR order (each color as: blue, then green, then red). For simplicity we choose: Red stripe: (B=0, G=0, R=255) White stripe: (B=255, G=255, R=255) Union (blue): (B=255, G=0, R=0) [i.e. pure blue] */ for (int y = 0; y < height; y++) { int row_from_top = height - 1 - y; for (int x = 0; x < width; x++) { unsigned char blue, green, red; /* Determine which stripe this pixel lies in */ int stripe_index = row_from_top / stripe_height; if (stripe_index % 2 == 0) { /* Even stripe: red */ blue = 0; green = 0; red = 255; } else { /* Odd stripe: white */ blue = 255; green = 255; red = 255; } /* Override with union (blue) if we are in the top union area */ if (row_from_top < union_height && x < union_width) { blue = 255; /* Blue pixel: B=255, G=0, R=0 */ green = 0; red = 0; } /* Set pixel at column x in the row buffer: */ int pixel_index = x * 3; row[pixel_index] = blue; row[pixel_index + 1] = green; row[pixel_index + 2] = red; } /* Zero out any padding bytes */ for (int p = width * 3; p < row_stride; p++) row[p] = 0; fwrite(row, 1, row_stride, stdout); } free(row); return 0; } ------------------------------------------------ Explanation of key parts: 1. The BMP file header (14 bytes) and the BITMAPINFOHEADER (40 bytes) are manually built using little-endian encoding. 2. The row_stride is computed so that each row’s total bytes is a multiple of 4 (a BMP requirement). 3. For each pixel the program: – Computes the “row_from_top” (since BMP rows are stored bottom–up). – Determines the stripe number by dividing row_from_top by stripe_height. – Sets the pixel’s color to red or white depending on whether the stripe is even or odd. – If the pixel is in the union region (the top 70 rows and x < 99), the color is set to blue. 4. The program writes the headers followed by each padded scanline. Compile and run this program. When you redirect its output to a file (e.g. flag.bmp) you’ll get a valid BMP image of a stylized American flag.
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 | /* flag.c – Write a BMP American flag to stdout */ #include <stdio.h> #include <stdlib.h> #ifdef _WIN32 #include <fcntl.h> #include <io.h> #endif int main(void) { /* On Windows, set stdout to binary mode */ #ifdef _WIN32 _setmode(_fileno(stdout), _O_BINARY); #endif /* Dimensions for the flag. We choose: Flag height = 130 pixels (13 stripes × 10 pixels each), Flag width = 247 pixels (to give a 10:19 ratio), Union (blue) covers the top 70 pixels (7 stripes) in height, and 99 pixels in width (~2/5 of 247). */ const int width = 247; const int height = 130; const int stripe_height = 10; const int union_height = 70; const int union_width = 99; /* Each pixel is 3 bytes, and each scanline (row) must be padded to a 4‐byte boundary. The padded row length in bytes is computed as follows: */ const int row_stride = ((width * 3 + 3) / 4) * 4; const int pixel_data_size = row_stride * height; const int file_size = 14 + 40 + pixel_data_size; /* 14‐byte file header + 40‐byte DIB header */ /* --- BMP File Header (14 bytes) --- */ unsigned char bmp_file_header[14] = {0}; bmp_file_header[0] = 'B'; bmp_file_header[1] = 'M'; bmp_file_header[2] = file_size & 0xFF; bmp_file_header[3] = (file_size >> 8) & 0xFF; bmp_file_header[4] = (file_size >> 16) & 0xFF; bmp_file_header[5] = (file_size >> 24) & 0xFF; /* bytes 6–9: reserved (set to 0) */ int pixel_data_offset = 14 + 40; /* header sizes */ bmp_file_header[10] = pixel_data_offset & 0xFF; bmp_file_header[11] = (pixel_data_offset >> 8) & 0xFF; bmp_file_header[12] = (pixel_data_offset >> 16) & 0xFF; bmp_file_header[13] = (pixel_data_offset >> 24) & 0xFF; /* --- DIB Header (BITMAPINFOHEADER, 40 bytes) --- */ unsigned char bmp_info_header[40] = {0}; bmp_info_header[0] = 40; /* header size (40 bytes) */ bmp_info_header[4] = width & 0xFF; bmp_info_header[5] = (width >> 8) & 0xFF; bmp_info_header[6] = (width >> 16) & 0xFF; bmp_info_header[7] = (width >> 24) & 0xFF; bmp_info_header[8] = height & 0xFF; bmp_info_header[9] = (height >> 8) & 0xFF; bmp_info_header[10] = (height >> 16) & 0xFF; bmp_info_header[11] = (height >> 24) & 0xFF; bmp_info_header[12] = 1; /* color planes */ bmp_info_header[13] = 0; bmp_info_header[14] = 24; /* bits per pixel (24-bit BMP) */ bmp_info_header[15] = 0; /* The remainder (compression, image size, etc.) can be left as 0. */ /* Write headers to stdout */ fwrite(bmp_file_header, 1, 14, stdout); fwrite(bmp_info_header, 1, 40, stdout); /* Allocate buffer for one row (scanline) of pixel data */ unsigned char *row = malloc(row_stride); if (!row) { fprintf(stderr, "Memory allocation error\n"); return 1; } /* Draw the flag. Note: In BMP the pixel rows go from bottom to top. We define row_from_top = (height - 1 - y) so that 0 is the top row. The top 7 stripes (rows 0 to 69) get the blue union on the left (x < union_width). The stripes alternate red and white: Even-numbered stripe (from the top) = red, odd-numbered = white. Colors are stored in BGR order (each color as: blue, then green, then red). For simplicity we choose: Red stripe: (B=0, G=0, R=255) White stripe: (B=255, G=255, R=255) Union (blue): (B=255, G=0, R=0) [i.e. pure blue] */ for (int y = 0; y < height; y++) { int row_from_top = height - 1 - y; for (int x = 0; x < width; x++) { unsigned char blue, green, red; /* Determine which stripe this pixel lies in */ int stripe_index = row_from_top / stripe_height; if (stripe_index % 2 == 0) { /* Even stripe: red */ blue = 0; green = 0; red = 255; } else { /* Odd stripe: white */ blue = 255; green = 255; red = 255; } /* Override with union (blue) if we are in the top union area */ if (row_from_top < union_height && x < union_width) { blue = 255; /* Blue pixel: B=255, G=0, R=0 */ green = 0; red = 0; } /* Set pixel at column x in the row buffer: */ int pixel_index = x * 3; row[pixel_index] = blue; row[pixel_index + 1] = green; row[pixel_index + 2] = red; } /* Zero out any padding bytes */ for (int p = width * 3; p < row_stride; p++) row[p] = 0; fwrite(row, 1, row_stride, stdout); } free(row); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 | /* flag.c – Write a BMP American flag to stdout */ #include <stdio.h> #include <stdlib.h> #ifdef _WIN32 #include <fcntl.h> #include <io.h> #endif int main(void) { /* On Windows, set stdout to binary mode */ #ifdef _WIN32 _setmode(_fileno(stdout), _O_BINARY); #endif /* Dimensions for the flag. We choose: Flag height = 130 pixels (13 stripes × 10 pixels each), Flag width = 247 pixels (to give a 10:19 ratio), Union (blue) covers the top 70 pixels (7 stripes) in height, and 99 pixels in width (~2/5 of 247). */ const int width = 247; const int height = 130; const int stripe_height = 10; const int union_height = 70; const int union_width = 99; /* Each pixel is 3 bytes, and each scanline (row) must be padded to a 4‐byte boundary. The padded row length in bytes is computed as follows: */ const int row_stride = ((width * 3 + 3) / 4) * 4; const int pixel_data_size = row_stride * height; const int file_size = 14 + 40 + pixel_data_size; /* 14‐byte file header + 40‐byte DIB header */ /* --- BMP File Header (14 bytes) --- */ unsigned char bmp_file_header[14] = {0}; bmp_file_header[0] = 'B'; bmp_file_header[1] = 'M'; bmp_file_header[2] = file_size & 0xFF; bmp_file_header[3] = (file_size >> 8) & 0xFF; bmp_file_header[4] = (file_size >> 16) & 0xFF; bmp_file_header[5] = (file_size >> 24) & 0xFF; /* bytes 6–9: reserved (set to 0) */ int pixel_data_offset = 14 + 40; /* header sizes */ bmp_file_header[10] = pixel_data_offset & 0xFF; bmp_file_header[11] = (pixel_data_offset >> 8) & 0xFF; bmp_file_header[12] = (pixel_data_offset >> 16) & 0xFF; bmp_file_header[13] = (pixel_data_offset >> 24) & 0xFF; /* --- DIB Header (BITMAPINFOHEADER, 40 bytes) --- */ unsigned char bmp_info_header[40] = {0}; bmp_info_header[0] = 40; /* header size (40 bytes) */ bmp_info_header[4] = width & 0xFF; bmp_info_header[5] = (width >> 8) & 0xFF; bmp_info_header[6] = (width >> 16) & 0xFF; bmp_info_header[7] = (width >> 24) & 0xFF; bmp_info_header[8] = height & 0xFF; bmp_info_header[9] = (height >> 8) & 0xFF; bmp_info_header[10] = (height >> 16) & 0xFF; bmp_info_header[11] = (height >> 24) & 0xFF; bmp_info_header[12] = 1; /* color planes */ bmp_info_header[13] = 0; bmp_info_header[14] = 24; /* bits per pixel (24-bit BMP) */ bmp_info_header[15] = 0; /* The remainder (compression, image size, etc.) can be left as 0. */ /* Write headers to stdout */ fwrite(bmp_file_header, 1, 14, stdout); fwrite(bmp_info_header, 1, 40, stdout); /* Allocate buffer for one row (scanline) of pixel data */ unsigned char *row = malloc(row_stride); if (!row) { fprintf(stderr, "Memory allocation error\n"); return 1; } /* Draw the flag. Note: In BMP the pixel rows go from bottom to top. We define row_from_top = (height - 1 - y) so that 0 is the top row. The top 7 stripes (rows 0 to 69) get the blue union on the left (x < union_width). The stripes alternate red and white: Even-numbered stripe (from the top) = red, odd-numbered = white. Colors are stored in BGR order (each color as: blue, then green, then red). For simplicity we choose: Red stripe: (B=0, G=0, R=255) White stripe: (B=255, G=255, R=255) Union (blue): (B=255, G=0, R=0) [i.e. pure blue] */ for (int y = 0; y < height; y++) { int row_from_top = height - 1 - y; for (int x = 0; x < width; x++) { unsigned char blue, green, red; /* Determine which stripe this pixel lies in */ int stripe_index = row_from_top / stripe_height; if (stripe_index % 2 == 0) { /* Even stripe: red */ blue = 0; green = 0; red = 255; } else { /* Odd stripe: white */ blue = 255; green = 255; red = 255; } /* Override with union (blue) if we are in the top union area */ if (row_from_top < union_height && x < union_width) { blue = 255; /* Blue pixel: B=255, G=0, R=0 */ green = 0; red = 0; } /* Set pixel at column x in the row buffer: */ int pixel_index = x * 3; row[pixel_index] = blue; row[pixel_index + 1] = green; row[pixel_index + 2] = red; } /* Zero out any padding bytes */ for (int p = width * 3; p < row_stride; p++) row[p] = 0; fwrite(row, 1, row_stride, stdout); } free(row); return 0; } |
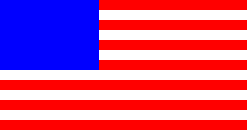
What flag is shown in this image?
This image shows a fictional or altered version of the United States flag. The design resembles the U.S. flag with red and white stripes, but the blue field is solid without stars.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Below is one complete C program that “draws” an American flag into a 24‐bit BMP image and writes its bytes to stdout. (On Windows you may wish to set stdout to binary mode.) In this example the flag is 380×260 pixels with 13 equally tall stripes (each 20 pixels high). The navy “union” covers the top‐left 152×140 pixels (i.e. the upper seven stripes), and over that we “stamp” white “stars” (each a 3×3 block) arranged in 9 staggered rows (6 stars in even rows and 5 in odd rows). You can compile and run this code; for example, redirect its output to a file (e.g. flag.bmp) and then open that file with an image viewer. Here is the complete source code: ----------------------------------------------------------- /************************************************************* * american_flag.c * * This program generates a 24-bit BMP image of an American flag * and writes it to stdout. Compile and run, then redirect stdout * to a file (e.g., flag.bmp) so that you get a valid BMP file. * * Note: On Windows you may need to set stdout to binary mode. ************************************************************/ #include <stdio.h> #include <stdlib.h> #include <math.h> /* Structure for a star's center coordinate */ typedef struct { int x; int y; } Star; int main(void) { /* Image dimensions and flag geometry parameters */ const int width = 380; const int height = 260; const int stripeHeight = 20; /* 13 stripes of 20 pixels each */ const int unionWidth = 152; /* union covers 2/5 of width */ const int unionHeight = 7 * stripeHeight; /* union covers top 7 stripes */ /* Colors in (B, G, R) order for BMP 24-bit pixels */ unsigned char red[3] = {0, 0, 255}; /* red stripe: RGB(255,0,0) */ unsigned char white[3] = {255, 255, 255}; /* white stripe or star */ unsigned char blue[3] = {128, 0, 0}; /* navy blue union: RGB(0,0,128) */ /* Pre-calculate star positions. We follow the traditional layout: 9 rows; rows with index 0,2,4,6,8 get 6 stars; rows with index 1,3,5,7 get 5 stars. We compute the centers within the union rectangle. We use a vertical margin computed as unionHeight/(9+1) so that the first star's center is roughly 1/10 from the top and the last roughly 1/10 from the bottom. */ Star stars[60]; /* at most 9*6 = 54 stars */ int starCount = 0; const int totalStarRows = 9; double vertSpacing = unionHeight / (double)(totalStarRows + 1); /* about 140/10 = 14.0 */ int row; for(row = 0; row < totalStarRows; row++){ int nStars = (row % 2 == 0) ? 6 : 5; /* even rows get 6, odd rows get 5 */ double horizSpacing = unionWidth / (double)(nStars + 1); /* Compute this star row's vertical center (relative to the top of the flag) */ int centerY = (int) ( (row + 1) * vertSpacing + 0.5 ); int col; for(col = 0; col < nStars; col++){ int centerX = (int)( (col + 1) * horizSpacing + 0.5 ); stars[starCount].x = centerX; stars[starCount].y = centerY; starCount++; } } /* Allocate memory for the pixel data. We store the image in top-down order (row 0 = top) in an array. Each pixel will be 3 bytes (B, G, R). */ unsigned char *img = malloc(width * height * 3); if (!img) { fprintf(stderr, "Could not allocate memory for image.\n"); return 1; } /* Fill the image. For each pixel we decide its base color by stripes. The stripes run the full width. Then if the pixel lies within the union (upper left) we override with blue. Finally, if the pixel lies in the union region and falls in a 3x3 block around one of the star centers, we set it to white. (Note that for simplicity stars are drawn as square patches of 3 pixels per side.) */ int y, x; for(y = 0; y < height; y++){ /* Determine stripe index by starting from the top (row 0) */ int stripe = y / stripeHeight; unsigned char *baseColor = (stripe % 2 == 0) ? red : white; for(x = 0; x < width; x++){ /* Compute pointer to pixel in our image array */ int idx = (y * width + x) * 3; /* Set the pixel to the stripe color */ img[idx] = baseColor[0]; img[idx+1] = baseColor[1]; img[idx+2] = baseColor[2]; /* If the pixel is in the union region, override with blue */ if (x < unionWidth && y < unionHeight) { img[idx] = blue[0]; img[idx+1] = blue[1]; img[idx+2] = blue[2]; /* Check if we should draw a star: if (x,y) is within 1 pixel of any star center, then color that pixel white. (This draws a 3x3 white square.) */ int s; for(s = 0; s < starCount; s++){ if (abs(x - stars[s].x) <= 1 && abs(y - stars[s].y) <= 1) { img[idx] = white[0]; img[idx+1] = white[1]; img[idx+2] = white[2]; break; /* No need to check further stars */ } } } } } /* Prepare BMP header. A 24-bit BMP file header is 14 bytes plus a 40-byte DIB header = 54 bytes total. Rows must be padded to a multiple of 4 bytes. */ int rowSizeBytes = (width * 3); int paddingSize = (4 - (rowSizeBytes % 4)) % 4; int dataSize = (rowSizeBytes + paddingSize) * height; int fileSize = 54 + dataSize; unsigned char header[54] = {0}; /* BMP signature */ header[0] = 'B'; header[1] = 'M'; /* File size (little-endian) */ header[2] = (unsigned char)( fileSize & 0xFF ); header[3] = (unsigned char)((fileSize >> 8) & 0xFF ); header[4] = (unsigned char)((fileSize >> 16)& 0xFF ); header[5] = (unsigned char)((fileSize >> 24)& 0xFF ); /* Reserved fields (2 bytes each) are 0 */ /* Pixel data offset: 54 */ header[10] = 54; /* DIB header size: 40 bytes */ header[14] = 40; /* Image width (little-endian) */ header[18] = (unsigned char)( width & 0xFF ); header[19] = (unsigned char)((width >> 8) & 0xFF ); header[20] = (unsigned char)((width >> 16)& 0xFF ); header[21] = (unsigned char)((width >> 24)& 0xFF ); /* Image height (little-endian) */ header[22] = (unsigned char)( height & 0xFF ); header[23] = (unsigned char)((height >> 8) & 0xFF ); header[24] = (unsigned char)((height >> 16)& 0xFF ); header[25] = (unsigned char)((height >> 24)& 0xFF ); /* Planes: must be 1 */ header[26] = 1; /* Bits per pixel: 24 */ header[28] = 24; /* Compression (0 = none) */ /* Image size (dataSize) */ header[34] = (unsigned char)( dataSize & 0xFF ); header[35] = (unsigned char)((dataSize >> 8) & 0xFF ); header[36] = (unsigned char)((dataSize >> 16)& 0xFF ); header[37] = (unsigned char)((dataSize >> 24)& 0xFF ); /* The rest of the header fields can remain 0 (resolution, colors used, etc.) */ /* Write header to stdout */ if (fwrite(header, 1, 54, stdout) != 54) { fprintf(stderr, "Error writing BMP header.\n"); free(img); return 1; } /* Write pixel data. BMP files store pixel rows bottom-up. For each row, also write the required padding bytes (which are zero). */ int rowIndex; unsigned char padding[3] = {0, 0, 0}; /* up to 3 bytes of padding needed */ for (rowIndex = height - 1; rowIndex >= 0; rowIndex--) { /* Write one row (without padding) */ if (fwrite(&img[rowIndex * width * 3], 1, width * 3, stdout) != width * 3) { fprintf(stderr, "Error writing BMP pixel data.\n"); free(img); return 1; } /* Write row padding */ if (paddingSize > 0) { if (fwrite(padding, 1, paddingSize, stdout) != paddingSize) { fprintf(stderr, "Error writing BMP row padding.\n"); free(img); return 1; } } } free(img); return 0; } ----------------------------------------------------------- Compilation and usage (Linux/Unix example): $ gcc american_flag.c -lm -o american_flag $ ./american_flag > flag.bmp Then open flag.bmp in an image viewer to see the generated American flag. Notes: 1. On Windows, use _setmode(_fileno(stdout), _O_BINARY) from <fcntl.h> and <io.h> so that stdout is in binary mode. 2. The flag drawing here is a simple rendition. You can tweak the sizes and the star‐drawing code to refine the look. This program meets the requirements: it produces a valid BMP file depicting an American flag to stdout. Enjoy!
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 | /************************************************************* * american_flag.c * * This program generates a 24-bit BMP image of an American flag * and writes it to stdout. Compile and run, then redirect stdout * to a file (e.g., flag.bmp) so that you get a valid BMP file. * * Note: On Windows you may need to set stdout to binary mode. ************************************************************/ #include <stdio.h> #include <stdlib.h> #include <math.h> /* Structure for a star's center coordinate */ typedef struct { int x; int y; } Star; int main(void) { /* Image dimensions and flag geometry parameters */ const int width = 380; const int height = 260; const int stripeHeight = 20; /* 13 stripes of 20 pixels each */ const int unionWidth = 152; /* union covers 2/5 of width */ const int unionHeight = 7 * stripeHeight; /* union covers top 7 stripes */ /* Colors in (B, G, R) order for BMP 24-bit pixels */ unsigned char red[3] = {0, 0, 255}; /* red stripe: RGB(255,0,0) */ unsigned char white[3] = {255, 255, 255}; /* white stripe or star */ unsigned char blue[3] = {128, 0, 0}; /* navy blue union: RGB(0,0,128) */ /* Pre-calculate star positions. We follow the traditional layout: 9 rows; rows with index 0,2,4,6,8 get 6 stars; rows with index 1,3,5,7 get 5 stars. We compute the centers within the union rectangle. We use a vertical margin computed as unionHeight/(9+1) so that the first star's center is roughly 1/10 from the top and the last roughly 1/10 from the bottom. */ Star stars[60]; /* at most 9*6 = 54 stars */ int starCount = 0; const int totalStarRows = 9; double vertSpacing = unionHeight / (double)(totalStarRows + 1); /* about 140/10 = 14.0 */ int row; for(row = 0; row < totalStarRows; row++){ int nStars = (row % 2 == 0) ? 6 : 5; /* even rows get 6, odd rows get 5 */ double horizSpacing = unionWidth / (double)(nStars + 1); /* Compute this star row's vertical center (relative to the top of the flag) */ int centerY = (int) ( (row + 1) * vertSpacing + 0.5 ); int col; for(col = 0; col < nStars; col++){ int centerX = (int)( (col + 1) * horizSpacing + 0.5 ); stars[starCount].x = centerX; stars[starCount].y = centerY; starCount++; } } /* Allocate memory for the pixel data. We store the image in top-down order (row 0 = top) in an array. Each pixel will be 3 bytes (B, G, R). */ unsigned char *img = malloc(width * height * 3); if (!img) { fprintf(stderr, "Could not allocate memory for image.\n"); return 1; } /* Fill the image. For each pixel we decide its base color by stripes. The stripes run the full width. Then if the pixel lies within the union (upper left) we override with blue. Finally, if the pixel lies in the union region and falls in a 3x3 block around one of the star centers, we set it to white. (Note that for simplicity stars are drawn as square patches of 3 pixels per side.) */ int y, x; for(y = 0; y < height; y++){ /* Determine stripe index by starting from the top (row 0) */ int stripe = y / stripeHeight; unsigned char *baseColor = (stripe % 2 == 0) ? red : white; for(x = 0; x < width; x++){ /* Compute pointer to pixel in our image array */ int idx = (y * width + x) * 3; /* Set the pixel to the stripe color */ img[idx] = baseColor[0]; img[idx+1] = baseColor[1]; img[idx+2] = baseColor[2]; /* If the pixel is in the union region, override with blue */ if (x < unionWidth && y < unionHeight) { img[idx] = blue[0]; img[idx+1] = blue[1]; img[idx+2] = blue[2]; /* Check if we should draw a star: if (x,y) is within 1 pixel of any star center, then color that pixel white. (This draws a 3x3 white square.) */ int s; for(s = 0; s < starCount; s++){ if (abs(x - stars[s].x) <= 1 && abs(y - stars[s].y) <= 1) { img[idx] = white[0]; img[idx+1] = white[1]; img[idx+2] = white[2]; break; /* No need to check further stars */ } } } } } /* Prepare BMP header. A 24-bit BMP file header is 14 bytes plus a 40-byte DIB header = 54 bytes total. Rows must be padded to a multiple of 4 bytes. */ int rowSizeBytes = (width * 3); int paddingSize = (4 - (rowSizeBytes % 4)) % 4; int dataSize = (rowSizeBytes + paddingSize) * height; int fileSize = 54 + dataSize; unsigned char header[54] = {0}; /* BMP signature */ header[0] = 'B'; header[1] = 'M'; /* File size (little-endian) */ header[2] = (unsigned char)( fileSize & 0xFF ); header[3] = (unsigned char)((fileSize >> 8) & 0xFF ); header[4] = (unsigned char)((fileSize >> 16)& 0xFF ); header[5] = (unsigned char)((fileSize >> 24)& 0xFF ); /* Reserved fields (2 bytes each) are 0 */ /* Pixel data offset: 54 */ header[10] = 54; /* DIB header size: 40 bytes */ header[14] = 40; /* Image width (little-endian) */ header[18] = (unsigned char)( width & 0xFF ); header[19] = (unsigned char)((width >> 8) & 0xFF ); header[20] = (unsigned char)((width >> 16)& 0xFF ); header[21] = (unsigned char)((width >> 24)& 0xFF ); /* Image height (little-endian) */ header[22] = (unsigned char)( height & 0xFF ); header[23] = (unsigned char)((height >> 8) & 0xFF ); header[24] = (unsigned char)((height >> 16)& 0xFF ); header[25] = (unsigned char)((height >> 24)& 0xFF ); /* Planes: must be 1 */ header[26] = 1; /* Bits per pixel: 24 */ header[28] = 24; /* Compression (0 = none) */ /* Image size (dataSize) */ header[34] = (unsigned char)( dataSize & 0xFF ); header[35] = (unsigned char)((dataSize >> 8) & 0xFF ); header[36] = (unsigned char)((dataSize >> 16)& 0xFF ); header[37] = (unsigned char)((dataSize >> 24)& 0xFF ); /* The rest of the header fields can remain 0 (resolution, colors used, etc.) */ /* Write header to stdout */ if (fwrite(header, 1, 54, stdout) != 54) { fprintf(stderr, "Error writing BMP header.\n"); free(img); return 1; } /* Write pixel data. BMP files store pixel rows bottom-up. For each row, also write the required padding bytes (which are zero). */ int rowIndex; unsigned char padding[3] = {0, 0, 0}; /* up to 3 bytes of padding needed */ for (rowIndex = height - 1; rowIndex >= 0; rowIndex--) { /* Write one row (without padding) */ if (fwrite(&img[rowIndex * width * 3], 1, width * 3, stdout) != width * 3) { fprintf(stderr, "Error writing BMP pixel data.\n"); free(img); return 1; } /* Write row padding */ if (paddingSize > 0) { if (fwrite(padding, 1, paddingSize, stdout) != paddingSize) { fprintf(stderr, "Error writing BMP row padding.\n"); free(img); return 1; } } } free(img); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 | /************************************************************* * american_flag.c * * This program generates a 24-bit BMP image of an American flag * and writes it to stdout. Compile and run, then redirect stdout * to a file (e.g., flag.bmp) so that you get a valid BMP file. * * Note: On Windows you may need to set stdout to binary mode. ************************************************************/ #include <stdio.h> #include <stdlib.h> #include <math.h> /* Structure for a star's center coordinate */ typedef struct { int x; int y; } Star; int main(void) { /* Image dimensions and flag geometry parameters */ const int width = 380; const int height = 260; const int stripeHeight = 20; /* 13 stripes of 20 pixels each */ const int unionWidth = 152; /* union covers 2/5 of width */ const int unionHeight = 7 * stripeHeight; /* union covers top 7 stripes */ /* Colors in (B, G, R) order for BMP 24-bit pixels */ unsigned char red[3] = {0, 0, 255}; /* red stripe: RGB(255,0,0) */ unsigned char white[3] = {255, 255, 255}; /* white stripe or star */ unsigned char blue[3] = {128, 0, 0}; /* navy blue union: RGB(0,0,128) */ /* Pre-calculate star positions. We follow the traditional layout: 9 rows; rows with index 0,2,4,6,8 get 6 stars; rows with index 1,3,5,7 get 5 stars. We compute the centers within the union rectangle. We use a vertical margin computed as unionHeight/(9+1) so that the first star's center is roughly 1/10 from the top and the last roughly 1/10 from the bottom. */ Star stars[60]; /* at most 9*6 = 54 stars */ int starCount = 0; const int totalStarRows = 9; double vertSpacing = unionHeight / (double)(totalStarRows + 1); /* about 140/10 = 14.0 */ int row; for(row = 0; row < totalStarRows; row++){ int nStars = (row % 2 == 0) ? 6 : 5; /* even rows get 6, odd rows get 5 */ double horizSpacing = unionWidth / (double)(nStars + 1); /* Compute this star row's vertical center (relative to the top of the flag) */ int centerY = (int) ( (row + 1) * vertSpacing + 0.5 ); int col; for(col = 0; col < nStars; col++){ int centerX = (int)( (col + 1) * horizSpacing + 0.5 ); stars[starCount].x = centerX; stars[starCount].y = centerY; starCount++; } } /* Allocate memory for the pixel data. We store the image in top-down order (row 0 = top) in an array. Each pixel will be 3 bytes (B, G, R). */ unsigned char *img = malloc(width * height * 3); if (!img) { fprintf(stderr, "Could not allocate memory for image.\n"); return 1; } /* Fill the image. For each pixel we decide its base color by stripes. The stripes run the full width. Then if the pixel lies within the union (upper left) we override with blue. Finally, if the pixel lies in the union region and falls in a 3x3 block around one of the star centers, we set it to white. (Note that for simplicity stars are drawn as square patches of 3 pixels per side.) */ int y, x; for(y = 0; y < height; y++){ /* Determine stripe index by starting from the top (row 0) */ int stripe = y / stripeHeight; unsigned char *baseColor = (stripe % 2 == 0) ? red : white; for(x = 0; x < width; x++){ /* Compute pointer to pixel in our image array */ int idx = (y * width + x) * 3; /* Set the pixel to the stripe color */ img[idx] = baseColor[0]; img[idx+1] = baseColor[1]; img[idx+2] = baseColor[2]; /* If the pixel is in the union region, override with blue */ if (x < unionWidth && y < unionHeight) { img[idx] = blue[0]; img[idx+1] = blue[1]; img[idx+2] = blue[2]; /* Check if we should draw a star: if (x,y) is within 1 pixel of any star center, then color that pixel white. (This draws a 3x3 white square.) */ int s; for(s = 0; s < starCount; s++){ if (abs(x - stars[s].x) <= 1 && abs(y - stars[s].y) <= 1) { img[idx] = white[0]; img[idx+1] = white[1]; img[idx+2] = white[2]; break; /* No need to check further stars */ } } } } } /* Prepare BMP header. A 24-bit BMP file header is 14 bytes plus a 40-byte DIB header = 54 bytes total. Rows must be padded to a multiple of 4 bytes. */ int rowSizeBytes = (width * 3); int paddingSize = (4 - (rowSizeBytes % 4)) % 4; int dataSize = (rowSizeBytes + paddingSize) * height; int fileSize = 54 + dataSize; unsigned char header[54] = {0}; /* BMP signature */ header[0] = 'B'; header[1] = 'M'; /* File size (little-endian) */ header[2] = (unsigned char)( fileSize & 0xFF ); header[3] = (unsigned char)((fileSize >> 8) & 0xFF ); header[4] = (unsigned char)((fileSize >> 16)& 0xFF ); header[5] = (unsigned char)((fileSize >> 24)& 0xFF ); /* Reserved fields (2 bytes each) are 0 */ /* Pixel data offset: 54 */ header[10] = 54; /* DIB header size: 40 bytes */ header[14] = 40; /* Image width (little-endian) */ header[18] = (unsigned char)( width & 0xFF ); header[19] = (unsigned char)((width >> 8) & 0xFF ); header[20] = (unsigned char)((width >> 16)& 0xFF ); header[21] = (unsigned char)((width >> 24)& 0xFF ); /* Image height (little-endian) */ header[22] = (unsigned char)( height & 0xFF ); header[23] = (unsigned char)((height >> 8) & 0xFF ); header[24] = (unsigned char)((height >> 16)& 0xFF ); header[25] = (unsigned char)((height >> 24)& 0xFF ); /* Planes: must be 1 */ header[26] = 1; /* Bits per pixel: 24 */ header[28] = 24; /* Compression (0 = none) */ /* Image size (dataSize) */ header[34] = (unsigned char)( dataSize & 0xFF ); header[35] = (unsigned char)((dataSize >> 8) & 0xFF ); header[36] = (unsigned char)((dataSize >> 16)& 0xFF ); header[37] = (unsigned char)((dataSize >> 24)& 0xFF ); /* The rest of the header fields can remain 0 (resolution, colors used, etc.) */ /* Write header to stdout */ if (fwrite(header, 1, 54, stdout) != 54) { fprintf(stderr, "Error writing BMP header.\n"); free(img); return 1; } /* Write pixel data. BMP files store pixel rows bottom-up. For each row, also write the required padding bytes (which are zero). */ int rowIndex; unsigned char padding[3] = {0, 0, 0}; /* up to 3 bytes of padding needed */ for (rowIndex = height - 1; rowIndex >= 0; rowIndex--) { /* Write one row (without padding) */ if (fwrite(&img[rowIndex * width * 3], 1, width * 3, stdout) != width * 3) { fprintf(stderr, "Error writing BMP pixel data.\n"); free(img); return 1; } /* Write row padding */ if (paddingSize > 0) { if (fwrite(padding, 1, paddingSize, stdout) != paddingSize) { fprintf(stderr, "Error writing BMP row padding.\n"); free(img); return 1; } } } free(img); return 0; } |
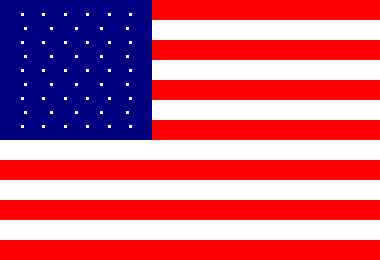
What flag is shown in this image?
This is a fictional or altered version of the United States flag. The stars are arranged in a grid pattern, which is not how they are arranged on the actual U.S. flag.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True