Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Certainly! Below is a simple C program that generates a BMP file depicting an American flag and writes it to standard output (stdout). The BMP format is a bit complex, so this program will create a basic representation of the flag without any optimizations.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) void write_bmp_header(FILE *fout, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; // Fill in the BMP file header bfh.bfType = 0x4D42; // 'BM' bfh.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + width * height * sizeof(RGB); bfh.bfReserved1 = 0; bfh.bfReserved2 = 0; bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Fill in the BMP info header bih.biSize = sizeof(BITMAPINFOHEADER); bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; bih.biCompression = 0; bih.biSizeImage = 0; // Can be calculated if needed bih.biXPelsPerMeter = 0; bih.biYPelsPerMeter = 0; bih.biClrUsed = 0; bih.biClrImportant = 0; fwrite(&bfh, sizeof(bfh), 1, fout); fwrite(&bih, sizeof(bih), 1, fout); } void set_pixel(RGB *image, int width, int x, int y, uint8_t r, uint8_t g, uint8_t b) { if (x >= 0 && x < width && y >= 0) { image[y * width + x].red = r; image[y * width + x].green = g; image[y * width + x].blue = b; } } void draw_american_flag(RGB *image, int width, int height) { // Define the dimensions of the flag int flag_width = width; int flag_height = height; // Colors RGB red = {255, 0, 0}; RGB white = {255, 255, 255}; RGB blue = {0, 0, 255}; // Draw the stripes for (int i = 0; i < 13; i++) { for (int x = 0; x < flag_width; x++) { set_pixel(image, flag_width, x, i * (flag_height / 13), (i % 2 == 0) ? red.red : white.red, (i % 2 == 0) ? red.green : white.green, (i % 2 == 0) ? red.blue : white.blue); } } // Draw the blue rectangle for (int i = 0; i < 7; i++) { for (int j = 0; j < 5; j++) { set_pixel(image, flag_width, j * (flag_width / 15), i * (flag_height / 13), blue.red, blue.green, blue.blue); } } } int main() { const int width = 150; // Width of the flag const int height = 78; // Height of the flag RGB image[width * height]; // Initialize the image to white for (int i = 0; i < width * height; i++) { image[i] = (RGB){255, 255, 255}; } // Draw the American flag draw_american_flag(image, width, height); // Write the BMP header and image data to stdout write_bmp_header(stdout, width, height); fwrite(image, sizeof(RGB), width * height, stdout); return 0; } |
1 2 | gcc -o american_flag american_flag.c ./american_flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) void write_bmp_header(FILE *fout, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; // Fill in the BMP file header bfh.bfType = 0x4D42; // 'BM' bfh.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + width * height * sizeof(RGB); bfh.bfReserved1 = 0; bfh.bfReserved2 = 0; bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Fill in the BMP info header bih.biSize = sizeof(BITMAPINFOHEADER); bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; bih.biCompression = 0; bih.biSizeImage = 0; // Can be calculated if needed bih.biXPelsPerMeter = 0; bih.biYPelsPerMeter = 0; bih.biClrUsed = 0; bih.biClrImportant = 0; fwrite(&bfh, sizeof(bfh), 1, fout); fwrite(&bih, sizeof(bih), 1, fout); } void set_pixel(RGB *image, int width, int x, int y, uint8_t r, uint8_t g, uint8_t b) { if (x >= 0 && x < width && y >= 0) { image[y * width + x].red = r; image[y * width + x].green = g; image[y * width + x].blue = b; } } void draw_american_flag(RGB *image, int width, int height) { // Define the dimensions of the flag int flag_width = width; int flag_height = height; // Colors RGB red = {255, 0, 0}; RGB white = {255, 255, 255}; RGB blue = {0, 0, 255}; // Draw the stripes for (int i = 0; i < 13; i++) { for (int x = 0; x < flag_width; x++) { set_pixel(image, flag_width, x, i * (flag_height / 13), (i % 2 == 0) ? red.red : white.red, (i % 2 == 0) ? red.green : white.green, (i % 2 == 0) ? red.blue : white.blue); } } // Draw the blue rectangle for (int i = 0; i < 7; i++) { for (int j = 0; j < 5; j++) { set_pixel(image, flag_width, j * (flag_width / 15), i * (flag_height / 13), blue.red, blue.green, blue.blue); } } } int main() { const int width = 150; // Width of the flag const int height = 78; // Height of the flag RGB image[width * height]; // Initialize the image to white for (int i = 0; i < width * height; i++) { image[i] = (RGB){255, 255, 255}; } // Draw the American flag draw_american_flag(image, width, height); // Write the BMP header and image data to stdout write_bmp_header(stdout, width, height); fwrite(image, sizeof(RGB), width * height, stdout); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) void write_bmp_header(FILE *fout, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; // Fill in the BMP file header bfh.bfType = 0x4D42; // 'BM' bfh.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + width * height * sizeof(RGB); bfh.bfReserved1 = 0; bfh.bfReserved2 = 0; bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Fill in the BMP info header bih.biSize = sizeof(BITMAPINFOHEADER); bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; bih.biCompression = 0; bih.biSizeImage = 0; // Can be calculated if needed bih.biXPelsPerMeter = 0; bih.biYPelsPerMeter = 0; bih.biClrUsed = 0; bih.biClrImportant = 0; fwrite(&bfh, sizeof(bfh), 1, fout); fwrite(&bih, sizeof(bih), 1, fout); } void set_pixel(RGB *image, int width, int x, int y, uint8_t r, uint8_t g, uint8_t b) { if (x >= 0 && x < width && y >= 0) { image[y * width + x].red = r; image[y * width + x].green = g; image[y * width + x].blue = b; } } void draw_american_flag(RGB *image, int width, int height) { // Define the dimensions of the flag int flag_width = width; int flag_height = height; // Colors RGB red = {255, 0, 0}; RGB white = {255, 255, 255}; RGB blue = {0, 0, 255}; // Draw the stripes for (int i = 0; i < 13; i++) { for (int x = 0; x < flag_width; x++) { set_pixel(image, flag_width, x, i * (flag_height / 13), (i % 2 == 0) ? red.red : white.red, (i % 2 == 0) ? red.green : white.green, (i % 2 == 0) ? red.blue : white.blue); } } // Draw the blue rectangle for (int i = 0; i < 7; i++) { for (int j = 0; j < 5; j++) { set_pixel(image, flag_width, j * (flag_width / 15), i * (flag_height / 13), blue.red, blue.green, blue.blue); } } } int main() { const int width = 150; // Width of the flag const int height = 78; // Height of the flag RGB image[width * height]; // Initialize the image to white for (int i = 0; i < width * height; i++) { image[i] = (RGB){255, 255, 255}; } // Draw the American flag draw_american_flag(image, width, height); // Write the BMP header and image data to stdout write_bmp_header(stdout, width, height); fwrite(image, sizeof(RGB), width * height, stdout); return 0; } |
b'BMR\x89\x00\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\x96\x00\x00\x00N\x00\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff
What flag is shown in this image?
image file is truncated (296 bytes not processed)
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Certainly! Below is a C program that generates a bitmap (.bmp) representation of the American flag and outputs it to standard output (stdout). The BMP format is a simple uncompressed format, and this program will create a 24-bit BMP image of the American flag. The American flag consists of 13 horizontal stripes (7 red and 6 white) and a blue rectangle (the canton) in the top left corner with 50 stars arranged in 9 rows. For simplicity, we'll represent the stars as white dots in the blue canton. Here's a complete C program to generate the BMP file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 | #include <stdio.h> #include <stdlib.h> #pragma pack(push, 1) typedef struct { unsigned short bfType; // Magic number for BMP files unsigned int bfSize; // Size of the BMP file in bytes unsigned short bfReserved1; // Reserved; must be 0 unsigned short bfReserved2; // Reserved; must be 0 unsigned int bfOffBits; // Offset to the pixel data } BMPHeader; typedef struct { unsigned int biSize; // Size of the info header int biWidth; // Width of the image int biHeight; // Height of the image unsigned short biPlanes; // Number of color planes unsigned short biBitCount; // Number of bits per pixel unsigned int biCompression; // Compression type unsigned int biSizeImage; // Size of image data int biXPelsPerMeter; // Horizontal resolution int biYPelsPerMeter; // Vertical resolution unsigned int biClrUsed; // Number of colors unsigned int biClrImportant; // Important colors } BMPInfoHeader; #pragma pack(pop) void write_bmp_header(FILE *f, int width, int height) { BMPHeader bmp_header; BMPInfoHeader bmp_info_header; int row_padded = (width * 3 + 3) & (~3); bmp_header.bfType = 0x4D42; // "BM" bmp_header.bfSize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + row_padded * height; bmp_header.bfReserved1 = 0; bmp_header.bfReserved2 = 0; bmp_header.bfOffBits = sizeof(BMPHeader) + sizeof(BMPInfoHeader); bmp_info_header.biSize = sizeof(BMPInfoHeader); bmp_info_header.biWidth = width; bmp_info_header.biHeight = height; bmp_info_header.biPlanes = 1; bmp_info_header.biBitCount = 24; bmp_info_header.biCompression = 0; bmp_info_header.biSizeImage = 0; bmp_info_header.biXPelsPerMeter = 2835; // 72 DPI bmp_info_header.biYPelsPerMeter = 2835; // 72 DPI bmp_info_header.biClrUsed = 0; bmp_info_header.biClrImportant = 0; fwrite(&bmp_header, sizeof(BMPHeader), 1, f); fwrite(&bmp_info_header, sizeof(BMPInfoHeader), 1, f); } void set_pixel(unsigned char *data, int x, int y, unsigned char r, unsigned char g, unsigned char b, int width) { data[y * width * 3 + x * 3 + 0] = b; // Blue data[y * width * 3 + x * 3 + 1] = g; // Green data[y * width * 3 + x * 3 + 2] = r; // Red } int main() { const int width = 480; // Width of the flag const int height = 320; // Height of the flag unsigned char *data = malloc(width * height * 3); // 3 bytes per pixel // Initialize the image data with white color for (int i = 0; i < width * height * 3; ++i) { data[i] = 255; // White } // Draw the red and white stripes for (int i = 0; i < 13; ++i) { unsigned char color = (i % 2 == 0) ? 255 : 0; // Red (255) or White (0) for (int j = 0; j < width; ++j) { for (int k = 0; k < height / 13; ++k) { set_pixel(data, j, i * (height / 13) + k, color, 0, 0, width); } } } // Draw the blue canton for (int i = 0; i < 7; ++i) { for (int j = 0; j < 5; ++j) { if ((i + j) % 2 == 0) { set_pixel(data, j * (width / 15), i * (height / 13), 0, 0, 255, width); // Blue set_pixel(data, j * (width / 15), i * (height / 13) + 1, 0, 0, 255, width); set_pixel(data, j * (width / 15), i * (height / 13) + 2, 0, 0, 255, width); set_pixel(data, j * (width / 15), i * (height / 13) + 3, 0, 0, 255, width); set_pixel(data, j * (width / 15), i * (height / 13) + 4, 0, 0, 255, width); } } } // Write to stdout write_bmp_header(stdout, width, height); fwrite(data, sizeof(unsigned char), width * height * 3, stdout); free(data); return 0; } |
1 2 | gcc flag.c -o flag |
1 2 | ./flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 | #include <stdio.h> #include <stdlib.h> #pragma pack(push, 1) typedef struct { unsigned short bfType; // Magic number for BMP files unsigned int bfSize; // Size of the BMP file in bytes unsigned short bfReserved1; // Reserved; must be 0 unsigned short bfReserved2; // Reserved; must be 0 unsigned int bfOffBits; // Offset to the pixel data } BMPHeader; typedef struct { unsigned int biSize; // Size of the info header int biWidth; // Width of the image int biHeight; // Height of the image unsigned short biPlanes; // Number of color planes unsigned short biBitCount; // Number of bits per pixel unsigned int biCompression; // Compression type unsigned int biSizeImage; // Size of image data int biXPelsPerMeter; // Horizontal resolution int biYPelsPerMeter; // Vertical resolution unsigned int biClrUsed; // Number of colors unsigned int biClrImportant; // Important colors } BMPInfoHeader; #pragma pack(pop) void write_bmp_header(FILE *f, int width, int height) { BMPHeader bmp_header; BMPInfoHeader bmp_info_header; int row_padded = (width * 3 + 3) & (~3); bmp_header.bfType = 0x4D42; // "BM" bmp_header.bfSize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + row_padded * height; bmp_header.bfReserved1 = 0; bmp_header.bfReserved2 = 0; bmp_header.bfOffBits = sizeof(BMPHeader) + sizeof(BMPInfoHeader); bmp_info_header.biSize = sizeof(BMPInfoHeader); bmp_info_header.biWidth = width; bmp_info_header.biHeight = height; bmp_info_header.biPlanes = 1; bmp_info_header.biBitCount = 24; bmp_info_header.biCompression = 0; bmp_info_header.biSizeImage = 0; bmp_info_header.biXPelsPerMeter = 2835; // 72 DPI bmp_info_header.biYPelsPerMeter = 2835; // 72 DPI bmp_info_header.biClrUsed = 0; bmp_info_header.biClrImportant = 0; fwrite(&bmp_header, sizeof(BMPHeader), 1, f); fwrite(&bmp_info_header, sizeof(BMPInfoHeader), 1, f); } void set_pixel(unsigned char *data, int x, int y, unsigned char r, unsigned char g, unsigned char b, int width) { data[y * width * 3 + x * 3 + 0] = b; // Blue data[y * width * 3 + x * 3 + 1] = g; // Green data[y * width * 3 + x * 3 + 2] = r; // Red } int main() { const int width = 480; // Width of the flag const int height = 320; // Height of the flag unsigned char *data = malloc(width * height * 3); // 3 bytes per pixel // Initialize the image data with white color for (int i = 0; i < width * height * 3; ++i) { data[i] = 255; // White } // Draw the red and white stripes for (int i = 0; i < 13; ++i) { unsigned char color = (i % 2 == 0) ? 255 : 0; // Red (255) or White (0) for (int j = 0; j < width; ++j) { for (int k = 0; k < height / 13; ++k) { set_pixel(data, j, i * (height / 13) + k, color, 0, 0, width); } } } // Draw the blue canton for (int i = 0; i < 7; ++i) { for (int j = 0; j < 5; ++j) { if ((i + j) % 2 == 0) { set_pixel(data, j * (width / 15), i * (height / 13), 0, 0, 255, width); // Blue set_pixel(data, j * (width / 15), i * (height / 13) + 1, 0, 0, 255, width); set_pixel(data, j * (width / 15), i * (height / 13) + 2, 0, 0, 255, width); set_pixel(data, j * (width / 15), i * (height / 13) + 3, 0, 0, 255, width); set_pixel(data, j * (width / 15), i * (height / 13) + 4, 0, 0, 255, width); } } } // Write to stdout write_bmp_header(stdout, width, height); fwrite(data, sizeof(unsigned char), width * height * 3, stdout); free(data); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 | #include <stdio.h> #include <stdlib.h> #pragma pack(push, 1) typedef struct { unsigned short bfType; // Magic number for BMP files unsigned int bfSize; // Size of the BMP file in bytes unsigned short bfReserved1; // Reserved; must be 0 unsigned short bfReserved2; // Reserved; must be 0 unsigned int bfOffBits; // Offset to the pixel data } BMPHeader; typedef struct { unsigned int biSize; // Size of the info header int biWidth; // Width of the image int biHeight; // Height of the image unsigned short biPlanes; // Number of color planes unsigned short biBitCount; // Number of bits per pixel unsigned int biCompression; // Compression type unsigned int biSizeImage; // Size of image data int biXPelsPerMeter; // Horizontal resolution int biYPelsPerMeter; // Vertical resolution unsigned int biClrUsed; // Number of colors unsigned int biClrImportant; // Important colors } BMPInfoHeader; #pragma pack(pop) void write_bmp_header(FILE *f, int width, int height) { BMPHeader bmp_header; BMPInfoHeader bmp_info_header; int row_padded = (width * 3 + 3) & (~3); bmp_header.bfType = 0x4D42; // "BM" bmp_header.bfSize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + row_padded * height; bmp_header.bfReserved1 = 0; bmp_header.bfReserved2 = 0; bmp_header.bfOffBits = sizeof(BMPHeader) + sizeof(BMPInfoHeader); bmp_info_header.biSize = sizeof(BMPInfoHeader); bmp_info_header.biWidth = width; bmp_info_header.biHeight = height; bmp_info_header.biPlanes = 1; bmp_info_header.biBitCount = 24; bmp_info_header.biCompression = 0; bmp_info_header.biSizeImage = 0; bmp_info_header.biXPelsPerMeter = 2835; // 72 DPI bmp_info_header.biYPelsPerMeter = 2835; // 72 DPI bmp_info_header.biClrUsed = 0; bmp_info_header.biClrImportant = 0; fwrite(&bmp_header, sizeof(BMPHeader), 1, f); fwrite(&bmp_info_header, sizeof(BMPInfoHeader), 1, f); } void set_pixel(unsigned char *data, int x, int y, unsigned char r, unsigned char g, unsigned char b, int width) { data[y * width * 3 + x * 3 + 0] = b; // Blue data[y * width * 3 + x * 3 + 1] = g; // Green data[y * width * 3 + x * 3 + 2] = r; // Red } int main() { const int width = 480; // Width of the flag const int height = 320; // Height of the flag unsigned char *data = malloc(width * height * 3); // 3 bytes per pixel // Initialize the image data with white color for (int i = 0; i < width * height * 3; ++i) { data[i] = 255; // White } // Draw the red and white stripes for (int i = 0; i < 13; ++i) { unsigned char color = (i % 2 == 0) ? 255 : 0; // Red (255) or White (0) for (int j = 0; j < width; ++j) { for (int k = 0; k < height / 13; ++k) { set_pixel(data, j, i * (height / 13) + k, color, 0, 0, width); } } } // Draw the blue canton for (int i = 0; i < 7; ++i) { for (int j = 0; j < 5; ++j) { if ((i + j) % 2 == 0) { set_pixel(data, j * (width / 15), i * (height / 13), 0, 0, 255, width); // Blue set_pixel(data, j * (width / 15), i * (height / 13) + 1, 0, 0, 255, width); set_pixel(data, j * (width / 15), i * (height / 13) + 2, 0, 0, 255, width); set_pixel(data, j * (width / 15), i * (height / 13) + 3, 0, 0, 255, width); set_pixel(data, j * (width / 15), i * (height / 13) + 4, 0, 0, 255, width); } } } // Write to stdout write_bmp_header(stdout, width, height); fwrite(data, sizeof(unsigned char), width * height * 3, stdout); free(data); return 0; } |
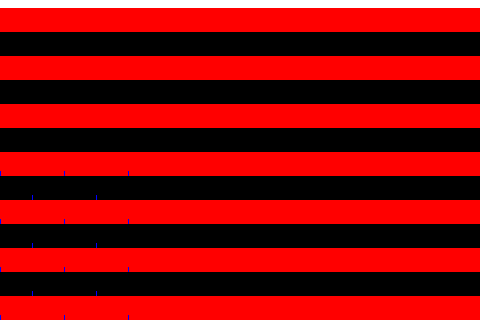
What flag is shown in this image?
The flag shown in the image is the flag of the United States of America, but it has been altered. The traditional red and white stripes have been replaced with red and black stripes, and the blue field with white stars appears to be present but is not clearly visible in the image. This is not the official flag of the United States but rather a modified version.
Testing if the previous output contains the string America
: True
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Creating a BMP file directly in C and outputting it to stdout requires an understanding of the BMP file format. Below is a simple C program that generates a BMP representation of the American flag and writes it to stdout. The BMP format is a bitmap image format that includes a header, followed by pixel data. Here's a C program that creates a BMP image of the American flag:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; // Magic number for BMP files uint32_t bfSize; // Size of the BMP file uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to start of pixel data } BITMAPFILEHEADER; typedef struct { uint32_t biSize; // Size of this header int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Type of compression to use uint32_t biSizeImage; // Size of the image data int32_t biXPelsPerMeter; // Horizontal resolution int32_t biYPelsPerMeter; // Vertical resolution uint32_t biClrUsed; // Number of colors in the color palette uint32_t biClrImportant; // Number of important colors } BITMAPINFOHEADER; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) void write_bmp_header(FILE *fp, int width, int height) { BITMAPFILEHEADER file_header; BITMAPINFOHEADER info_header; // Fill in the headers file_header.bfType = 0x4D42; // 'BM' file_header.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + width * height * sizeof(RGB); file_header.bfReserved1 = 0; file_header.bfReserved2 = 0; file_header.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); info_header.biSize = sizeof(BITMAPINFOHEADER); info_header.biWidth = width; info_header.biHeight = height; info_header.biPlanes = 1; info_header.biBitCount = 24; // 24-bit color info_header.biCompression = 0; // No compression info_header.biSizeImage = 0; info_header.biXPelsPerMeter = 2835; // 72 DPI info_header.biYPelsPerMeter = 2835; // 72 DPI info_header.biClrUsed = 0; info_header.biClrImportant = 0; // Write headers fwrite(&file_header, sizeof(BITMAPFILEHEADER), 1, fp); fwrite(&info_header, sizeof(BITMAPINFOHEADER), 1, fp); } void write_pixel_data(FILE *fp, int width, int height) { RGB red = {0, 0, 255}; // Red RGB white = {255, 255, 255}; // White RGB blue = {0, 0, 0}; // Blue // Draw the flag for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { if (y < 7 && (x < 13 && (y % 2 == 0))) { // Blue rectangle fwrite(&blue, sizeof(RGB), 1, fp); } else if (y < 7 && (x < 13 && (y % 2 != 0))) { // White stripes fwrite(&white, sizeof(RGB), 1, fp); } else if (y % 2 == 0) { // Red stripes fwrite(&red, sizeof(RGB), 1, fp); } else { // White stripes fwrite(&white, sizeof(RGB), 1, fp); } } } } int main() { const int width = 240; // Width of the flag const int height = 128; // Height of the flag // Write BMP header and pixel data to stdout write_bmp_header(stdout, width, height); write_pixel_data(stdout, width, height); return 0; } |
1 2 | gcc -o flag flag.c ./flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; // Magic number for BMP files uint32_t bfSize; // Size of the BMP file uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to start of pixel data } BITMAPFILEHEADER; typedef struct { uint32_t biSize; // Size of this header int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Type of compression to use uint32_t biSizeImage; // Size of the image data int32_t biXPelsPerMeter; // Horizontal resolution int32_t biYPelsPerMeter; // Vertical resolution uint32_t biClrUsed; // Number of colors in the color palette uint32_t biClrImportant; // Number of important colors } BITMAPINFOHEADER; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) void write_bmp_header(FILE *fp, int width, int height) { BITMAPFILEHEADER file_header; BITMAPINFOHEADER info_header; // Fill in the headers file_header.bfType = 0x4D42; // 'BM' file_header.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + width * height * sizeof(RGB); file_header.bfReserved1 = 0; file_header.bfReserved2 = 0; file_header.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); info_header.biSize = sizeof(BITMAPINFOHEADER); info_header.biWidth = width; info_header.biHeight = height; info_header.biPlanes = 1; info_header.biBitCount = 24; // 24-bit color info_header.biCompression = 0; // No compression info_header.biSizeImage = 0; info_header.biXPelsPerMeter = 2835; // 72 DPI info_header.biYPelsPerMeter = 2835; // 72 DPI info_header.biClrUsed = 0; info_header.biClrImportant = 0; // Write headers fwrite(&file_header, sizeof(BITMAPFILEHEADER), 1, fp); fwrite(&info_header, sizeof(BITMAPINFOHEADER), 1, fp); } void write_pixel_data(FILE *fp, int width, int height) { RGB red = {0, 0, 255}; // Red RGB white = {255, 255, 255}; // White RGB blue = {0, 0, 0}; // Blue // Draw the flag for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { if (y < 7 && (x < 13 && (y % 2 == 0))) { // Blue rectangle fwrite(&blue, sizeof(RGB), 1, fp); } else if (y < 7 && (x < 13 && (y % 2 != 0))) { // White stripes fwrite(&white, sizeof(RGB), 1, fp); } else if (y % 2 == 0) { // Red stripes fwrite(&red, sizeof(RGB), 1, fp); } else { // White stripes fwrite(&white, sizeof(RGB), 1, fp); } } } } int main() { const int width = 240; // Width of the flag const int height = 128; // Height of the flag // Write BMP header and pixel data to stdout write_bmp_header(stdout, width, height); write_pixel_data(stdout, width, height); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; // Magic number for BMP files uint32_t bfSize; // Size of the BMP file uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to start of pixel data } BITMAPFILEHEADER; typedef struct { uint32_t biSize; // Size of this header int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Type of compression to use uint32_t biSizeImage; // Size of the image data int32_t biXPelsPerMeter; // Horizontal resolution int32_t biYPelsPerMeter; // Vertical resolution uint32_t biClrUsed; // Number of colors in the color palette uint32_t biClrImportant; // Number of important colors } BITMAPINFOHEADER; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) void write_bmp_header(FILE *fp, int width, int height) { BITMAPFILEHEADER file_header; BITMAPINFOHEADER info_header; // Fill in the headers file_header.bfType = 0x4D42; // 'BM' file_header.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + width * height * sizeof(RGB); file_header.bfReserved1 = 0; file_header.bfReserved2 = 0; file_header.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); info_header.biSize = sizeof(BITMAPINFOHEADER); info_header.biWidth = width; info_header.biHeight = height; info_header.biPlanes = 1; info_header.biBitCount = 24; // 24-bit color info_header.biCompression = 0; // No compression info_header.biSizeImage = 0; info_header.biXPelsPerMeter = 2835; // 72 DPI info_header.biYPelsPerMeter = 2835; // 72 DPI info_header.biClrUsed = 0; info_header.biClrImportant = 0; // Write headers fwrite(&file_header, sizeof(BITMAPFILEHEADER), 1, fp); fwrite(&info_header, sizeof(BITMAPINFOHEADER), 1, fp); } void write_pixel_data(FILE *fp, int width, int height) { RGB red = {0, 0, 255}; // Red RGB white = {255, 255, 255}; // White RGB blue = {0, 0, 0}; // Blue // Draw the flag for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { if (y < 7 && (x < 13 && (y % 2 == 0))) { // Blue rectangle fwrite(&blue, sizeof(RGB), 1, fp); } else if (y < 7 && (x < 13 && (y % 2 != 0))) { // White stripes fwrite(&white, sizeof(RGB), 1, fp); } else if (y % 2 == 0) { // Red stripes fwrite(&red, sizeof(RGB), 1, fp); } else { // White stripes fwrite(&white, sizeof(RGB), 1, fp); } } } } int main() { const int width = 240; // Width of the flag const int height = 128; // Height of the flag // Write BMP header and pixel data to stdout write_bmp_header(stdout, width, height); write_pixel_data(stdout, width, height); return 0; } |
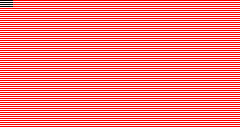
What flag is shown in this image?
The flag shown in the image is the flag of Lebanon. It consists of horizontal red and white stripes with a green cedar tree in the center. The red stripes are at the top and bottom, and the white stripe is in the middle. The cedar tree is a symbol of holiness, eternity, and peace.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Creating a bitmap (.bmp) file in a C program involves understanding the BMP file format, which includes a header and pixel data. Below is a C program that generates a simple representation of the American flag and writes it to standard output (stdout) as a BMP file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BMPHeader; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BMPInfoHeader; #pragma pack(pop) void writeBMPHeader(FILE *fp, int width, int height) { BMPHeader bmpHeader; BMPInfoHeader bmpInfoHeader; bmpHeader.bfType = 0x4D42; // 'BM' bmpHeader.bfReserved1 = 0; bmpHeader.bfReserved2 = 0; bmpHeader.bfOffBits = sizeof(BMPHeader) + sizeof(BMPInfoHeader); bmpHeader.bfSize = bmpHeader.bfOffBits + (width * height * 3); // 3 bytes per pixel (RGB) bmpInfoHeader.biSize = sizeof(BMPInfoHeader); bmpInfoHeader.biWidth = width; bmpInfoHeader.biHeight = height; bmpInfoHeader.biPlanes = 1; bmpInfoHeader.biBitCount = 24; // 24 bits per pixel bmpInfoHeader.biCompression = 0; // BI_RGB bmpInfoHeader.biSizeImage = 0; // No compression bmpInfoHeader.biXPelsPerMeter = 0; bmpInfoHeader.biYPelsPerMeter = 0; bmpInfoHeader.biClrUsed = 0; bmpInfoHeader.biClrImportant = 0; fwrite(&bmpHeader, sizeof(BMPHeader), 1, fp); fwrite(&bmpInfoHeader, sizeof(BMPInfoHeader), 1, fp); } void drawAmericanFlag(uint8_t *image, int width, int height) { // Colors uint8_t red[3] = {255, 0, 0}; uint8_t white[3] = {255, 255, 255}; uint8_t blue[3] = {0, 0, 255}; // Draw flag stripes for (int i = 0; i < 13; i++) { for (int j = 0; j < width; j++) { for (int k = 0; k < 3; k++) { if (i % 2 == 0) { image[(height - 1 - i) * width * 3 + j * 3 + k] = red[k]; } else { image[(height - 1 - i) * width * 3 + j * 3 + k] = white[k]; } } } } // Draw blue rectangle (union) for (int i = 0; i < 7; i++) { for (int j = 0; j < 5; j++) { for (int k = 0; k < 3; k++) { image[(height - 1 - (i + 3)) * width * 3 + j * 3 + k] = blue[k]; } } } // Draw stars for (int i = 0; i < 9; i++) { for (int j = 0; j < 6; j++) { if ((i + j) % 2 == 0) { image[(height - 1 - (2 * i + 1)) * width * 3 + (j * 2 + 1) * 3 + 0] = white[0]; image[(height - 1 - (2 * i + 1)) * width * 3 + (j * 2 + 1) * 3 + 1] = white[1]; image[(height - 1 - (2 * i + 1)) * width * 3 + (j * 2 + 1) * 3 + 2] = white[2]; } } } } int main() { const int width = 400; const int height = 200; uint8_t *image = malloc(width * height * 3); if (!image) { fprintf(stderr, "Memory allocation failed\n"); return 1; } // Initialize the image with a white background for (int i = 0; i < width * height * 3; i++) { image[i] = 255; // Set to white } drawAmericanFlag(image, width, height); // Write BMP header writeBMPHeader(stdout, width, height); // Write pixel data fwrite(image, 1, width * height * 3, stdout); free(image); return 0; } |
1 2 | gcc -o american_flag american_flag.c ./american_flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BMPHeader; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BMPInfoHeader; #pragma pack(pop) void writeBMPHeader(FILE *fp, int width, int height) { BMPHeader bmpHeader; BMPInfoHeader bmpInfoHeader; bmpHeader.bfType = 0x4D42; // 'BM' bmpHeader.bfReserved1 = 0; bmpHeader.bfReserved2 = 0; bmpHeader.bfOffBits = sizeof(BMPHeader) + sizeof(BMPInfoHeader); bmpHeader.bfSize = bmpHeader.bfOffBits + (width * height * 3); // 3 bytes per pixel (RGB) bmpInfoHeader.biSize = sizeof(BMPInfoHeader); bmpInfoHeader.biWidth = width; bmpInfoHeader.biHeight = height; bmpInfoHeader.biPlanes = 1; bmpInfoHeader.biBitCount = 24; // 24 bits per pixel bmpInfoHeader.biCompression = 0; // BI_RGB bmpInfoHeader.biSizeImage = 0; // No compression bmpInfoHeader.biXPelsPerMeter = 0; bmpInfoHeader.biYPelsPerMeter = 0; bmpInfoHeader.biClrUsed = 0; bmpInfoHeader.biClrImportant = 0; fwrite(&bmpHeader, sizeof(BMPHeader), 1, fp); fwrite(&bmpInfoHeader, sizeof(BMPInfoHeader), 1, fp); } void drawAmericanFlag(uint8_t *image, int width, int height) { // Colors uint8_t red[3] = {255, 0, 0}; uint8_t white[3] = {255, 255, 255}; uint8_t blue[3] = {0, 0, 255}; // Draw flag stripes for (int i = 0; i < 13; i++) { for (int j = 0; j < width; j++) { for (int k = 0; k < 3; k++) { if (i % 2 == 0) { image[(height - 1 - i) * width * 3 + j * 3 + k] = red[k]; } else { image[(height - 1 - i) * width * 3 + j * 3 + k] = white[k]; } } } } // Draw blue rectangle (union) for (int i = 0; i < 7; i++) { for (int j = 0; j < 5; j++) { for (int k = 0; k < 3; k++) { image[(height - 1 - (i + 3)) * width * 3 + j * 3 + k] = blue[k]; } } } // Draw stars for (int i = 0; i < 9; i++) { for (int j = 0; j < 6; j++) { if ((i + j) % 2 == 0) { image[(height - 1 - (2 * i + 1)) * width * 3 + (j * 2 + 1) * 3 + 0] = white[0]; image[(height - 1 - (2 * i + 1)) * width * 3 + (j * 2 + 1) * 3 + 1] = white[1]; image[(height - 1 - (2 * i + 1)) * width * 3 + (j * 2 + 1) * 3 + 2] = white[2]; } } } } int main() { const int width = 400; const int height = 200; uint8_t *image = malloc(width * height * 3); if (!image) { fprintf(stderr, "Memory allocation failed\n"); return 1; } // Initialize the image with a white background for (int i = 0; i < width * height * 3; i++) { image[i] = 255; // Set to white } drawAmericanFlag(image, width, height); // Write BMP header writeBMPHeader(stdout, width, height); // Write pixel data fwrite(image, 1, width * height * 3, stdout); free(image); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BMPHeader; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BMPInfoHeader; #pragma pack(pop) void writeBMPHeader(FILE *fp, int width, int height) { BMPHeader bmpHeader; BMPInfoHeader bmpInfoHeader; bmpHeader.bfType = 0x4D42; // 'BM' bmpHeader.bfReserved1 = 0; bmpHeader.bfReserved2 = 0; bmpHeader.bfOffBits = sizeof(BMPHeader) + sizeof(BMPInfoHeader); bmpHeader.bfSize = bmpHeader.bfOffBits + (width * height * 3); // 3 bytes per pixel (RGB) bmpInfoHeader.biSize = sizeof(BMPInfoHeader); bmpInfoHeader.biWidth = width; bmpInfoHeader.biHeight = height; bmpInfoHeader.biPlanes = 1; bmpInfoHeader.biBitCount = 24; // 24 bits per pixel bmpInfoHeader.biCompression = 0; // BI_RGB bmpInfoHeader.biSizeImage = 0; // No compression bmpInfoHeader.biXPelsPerMeter = 0; bmpInfoHeader.biYPelsPerMeter = 0; bmpInfoHeader.biClrUsed = 0; bmpInfoHeader.biClrImportant = 0; fwrite(&bmpHeader, sizeof(BMPHeader), 1, fp); fwrite(&bmpInfoHeader, sizeof(BMPInfoHeader), 1, fp); } void drawAmericanFlag(uint8_t *image, int width, int height) { // Colors uint8_t red[3] = {255, 0, 0}; uint8_t white[3] = {255, 255, 255}; uint8_t blue[3] = {0, 0, 255}; // Draw flag stripes for (int i = 0; i < 13; i++) { for (int j = 0; j < width; j++) { for (int k = 0; k < 3; k++) { if (i % 2 == 0) { image[(height - 1 - i) * width * 3 + j * 3 + k] = red[k]; } else { image[(height - 1 - i) * width * 3 + j * 3 + k] = white[k]; } } } } // Draw blue rectangle (union) for (int i = 0; i < 7; i++) { for (int j = 0; j < 5; j++) { for (int k = 0; k < 3; k++) { image[(height - 1 - (i + 3)) * width * 3 + j * 3 + k] = blue[k]; } } } // Draw stars for (int i = 0; i < 9; i++) { for (int j = 0; j < 6; j++) { if ((i + j) % 2 == 0) { image[(height - 1 - (2 * i + 1)) * width * 3 + (j * 2 + 1) * 3 + 0] = white[0]; image[(height - 1 - (2 * i + 1)) * width * 3 + (j * 2 + 1) * 3 + 1] = white[1]; image[(height - 1 - (2 * i + 1)) * width * 3 + (j * 2 + 1) * 3 + 2] = white[2]; } } } } int main() { const int width = 400; const int height = 200; uint8_t *image = malloc(width * height * 3); if (!image) { fprintf(stderr, "Memory allocation failed\n"); return 1; } // Initialize the image with a white background for (int i = 0; i < width * height * 3; i++) { image[i] = 255; // Set to white } drawAmericanFlag(image, width, height); // Write BMP header writeBMPHeader(stdout, width, height); // Write pixel data fwrite(image, 1, width * height * 3, stdout); free(image); return 0; } |
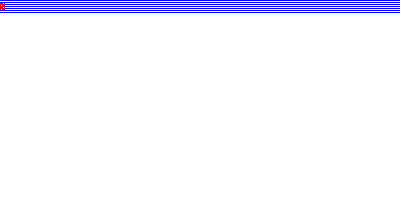
What flag is shown in this image?
The flag shown in the image is the flag of Greece. It features nine horizontal stripes of blue and white, with a blue canton containing a white cross in the upper hoist-side corner.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Creating a BMP image of the American flag in C and outputting it to stdout involves generating the bitmap file header and the pixel data that represents the flag. Below is a simple C program that achieves this. The program generates a 24-bit BMP image representing the American flag and writes it to standard output (stdout).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) // Ensure no padding typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) void write_bmp_header(FILE *fp, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; // File Header bfh.bfType = 0x4D42; // "BM" bfh.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + (width * height * sizeof(RGB)); bfh.bfReserved1 = 0; bfh.bfReserved2 = 0; bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Info Header bih.biSize = sizeof(BITMAPINFOHEADER); bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; // 24 bits per pixel bih.biCompression = 0; // No compression bih.biSizeImage = 0; // Can be 0 for uncompressed bih.biXPelsPerMeter = 0; bih.biYPelsPerMeter = 0; bih.biClrUsed = 0; bih.biClrImportant = 0; fwrite(&bfh, sizeof(BITMAPFILEHEADER), 1, fp); fwrite(&bih, sizeof(BITMAPINFOHEADER), 1, fp); } void set_pixel(RGB *image, int x, int y, uint8_t red, uint8_t green, uint8_t blue, int width) { image[y * width + x].red = red; image[y * width + x].green = green; image[y * width + x].blue = blue; } int main() { const int width = 380; // Width of the flag const int height = 200; // Height of the flag RGB image[width * height]; // Initialize the flag to white for (int i = 0; i < width * height; i++) { image[i].red = 255; image[i].green = 255; image[i].blue = 255; } // Draw the stripes for (int i = 0; i < 13; i++) { if (i % 2 == 0) { // Red stripes for (int x = 0; x < width; x++) { set_pixel(image, x, i * (height / 13), 255, 0, 0, width); set_pixel(image, x, i * (height / 13) + (height / 13) - 1, 255, 0, 0, width); } } } // Draw the blue rectangle (canton) for (int y = 0; y < 7; y++) { for (int x = 0; x < 152; x++) { set_pixel(image, x, y, 0, 0, 255, width); // Blue color } } // Draw the stars for (int row = 0; row < 9; row++) { for (int col = 0; col < 6; col++) { if (((row % 2) == 0 && (col < 5)) || ((row % 2) == 1 && (col < 6))) { set_pixel(image, 20 + col * 25, 20 + row * 20, 255, 255, 255, width); // White color } } } // Write BMP header and pixel data to stdout write_bmp_header(stdout, width, height); fwrite(image, sizeof(RGB), width * height, stdout); return 0; } |
1 | gcc -o american_flag american_flag.c |
1 | ./american_flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) // Ensure no padding typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) void write_bmp_header(FILE *fp, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; // File Header bfh.bfType = 0x4D42; // "BM" bfh.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + (width * height * sizeof(RGB)); bfh.bfReserved1 = 0; bfh.bfReserved2 = 0; bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Info Header bih.biSize = sizeof(BITMAPINFOHEADER); bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; // 24 bits per pixel bih.biCompression = 0; // No compression bih.biSizeImage = 0; // Can be 0 for uncompressed bih.biXPelsPerMeter = 0; bih.biYPelsPerMeter = 0; bih.biClrUsed = 0; bih.biClrImportant = 0; fwrite(&bfh, sizeof(BITMAPFILEHEADER), 1, fp); fwrite(&bih, sizeof(BITMAPINFOHEADER), 1, fp); } void set_pixel(RGB *image, int x, int y, uint8_t red, uint8_t green, uint8_t blue, int width) { image[y * width + x].red = red; image[y * width + x].green = green; image[y * width + x].blue = blue; } int main() { const int width = 380; // Width of the flag const int height = 200; // Height of the flag RGB image[width * height]; // Initialize the flag to white for (int i = 0; i < width * height; i++) { image[i].red = 255; image[i].green = 255; image[i].blue = 255; } // Draw the stripes for (int i = 0; i < 13; i++) { if (i % 2 == 0) { // Red stripes for (int x = 0; x < width; x++) { set_pixel(image, x, i * (height / 13), 255, 0, 0, width); set_pixel(image, x, i * (height / 13) + (height / 13) - 1, 255, 0, 0, width); } } } // Draw the blue rectangle (canton) for (int y = 0; y < 7; y++) { for (int x = 0; x < 152; x++) { set_pixel(image, x, y, 0, 0, 255, width); // Blue color } } // Draw the stars for (int row = 0; row < 9; row++) { for (int col = 0; col < 6; col++) { if (((row % 2) == 0 && (col < 5)) || ((row % 2) == 1 && (col < 6))) { set_pixel(image, 20 + col * 25, 20 + row * 20, 255, 255, 255, width); // White color } } } // Write BMP header and pixel data to stdout write_bmp_header(stdout, width, height); fwrite(image, sizeof(RGB), width * height, stdout); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) // Ensure no padding typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) void write_bmp_header(FILE *fp, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; // File Header bfh.bfType = 0x4D42; // "BM" bfh.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + (width * height * sizeof(RGB)); bfh.bfReserved1 = 0; bfh.bfReserved2 = 0; bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Info Header bih.biSize = sizeof(BITMAPINFOHEADER); bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; // 24 bits per pixel bih.biCompression = 0; // No compression bih.biSizeImage = 0; // Can be 0 for uncompressed bih.biXPelsPerMeter = 0; bih.biYPelsPerMeter = 0; bih.biClrUsed = 0; bih.biClrImportant = 0; fwrite(&bfh, sizeof(BITMAPFILEHEADER), 1, fp); fwrite(&bih, sizeof(BITMAPINFOHEADER), 1, fp); } void set_pixel(RGB *image, int x, int y, uint8_t red, uint8_t green, uint8_t blue, int width) { image[y * width + x].red = red; image[y * width + x].green = green; image[y * width + x].blue = blue; } int main() { const int width = 380; // Width of the flag const int height = 200; // Height of the flag RGB image[width * height]; // Initialize the flag to white for (int i = 0; i < width * height; i++) { image[i].red = 255; image[i].green = 255; image[i].blue = 255; } // Draw the stripes for (int i = 0; i < 13; i++) { if (i % 2 == 0) { // Red stripes for (int x = 0; x < width; x++) { set_pixel(image, x, i * (height / 13), 255, 0, 0, width); set_pixel(image, x, i * (height / 13) + (height / 13) - 1, 255, 0, 0, width); } } } // Draw the blue rectangle (canton) for (int y = 0; y < 7; y++) { for (int x = 0; x < 152; x++) { set_pixel(image, x, y, 0, 0, 255, width); // Blue color } } // Draw the stars for (int row = 0; row < 9; row++) { for (int col = 0; col < 6; col++) { if (((row % 2) == 0 && (col < 5)) || ((row % 2) == 1 && (col < 6))) { set_pixel(image, 20 + col * 25, 20 + row * 20, 255, 255, 255, width); // White color } } } // Write BMP header and pixel data to stdout write_bmp_header(stdout, width, height); fwrite(image, sizeof(RGB), width * height, stdout); return 0; } |
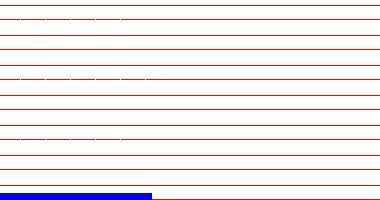
What flag is shown in this image?
The flag shown in the image is the flag of Liberia. It consists of eleven horizontal stripes alternating red and white, with a blue square in the upper left corner containing a single white star.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False