Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Creating a C program to generate a simple representation of the American flag and emit it as a BMP file directly to stdout involves several steps. BMP (Bitmap) is a pixel-based image format that stores image data in a way that a computer can read and display it. The American flag consists of 13 alternating red and white stripes and a blue field (the union) in the top left corner with 50 stars. For simplicity, this example will not include the stars in the union, but it will demonstrate how to generate the stripes and the blue field. The generated flag will be a basic, stylized representation. This program will create a very basic BMP file without compression and with a minimal BMP header. Note that handling BMP files can get quite complex depending on the features used (e.g., color depth, compression), but this example keeps things simple. **Please note that this program is a basic example and might need adjustments based on your specific requirements or improvements in BMP file handling.**
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | #include <stdio.h> #include <stdint.h> #define WIDTH 190 // Width of the flag #define HEIGHT 100 // Height of the flag #define BYTES_PER_PIXEL 3 // RGB #define IMAGE_SIZE (WIDTH * HEIGHT * BYTES_PER_PIXEL) #define BITS_PER_PIXEL (BYTES_PER_PIXEL * 8) // Function to set a pixel's color void setPixelColor(uint8_t *image, int x, int y, uint8_t r, uint8_t g, uint8_t b) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; image[index + 0] = b; image[index + 1] = g; image[index + 2] = r; } // Function to generate the flag void generateFlag(uint8_t *image) { int x, y; for (y = 0; y < HEIGHT; y++) { for (x = 0; x < WIDTH; x++) { // Blue field if (x < WIDTH / 2.5 && y < HEIGHT / 2) { setPixelColor(image, x, y, 0, 0, 255); // Blue } else { // Red and white stripes if (y % (HEIGHT / 13) < (HEIGHT / 13) / 2) { setPixelColor(image, x, y, 255, 0, 0); // Red } else { setPixelColor(image, x, y, 255, 255, 255); // White } } } } } // Main program int main() { uint8_t image[IMAGE_SIZE]; generateFlag(image); // BMP Header uint8_t header[54] = { 0x42, 0x4D, // BM 0, 0, 0, 0, // File size 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to start of pixel data 40, 0, 0, 0, // Header size WIDTH, 0, 0, 0, // Width HEIGHT, 0, 0, 0, // Height 1, 0, // Planes BITS_PER_PIXEL, 0, // Bits per pixel 0, 0, 0, 0, // Compression (none) IMAGE_SIZE, 0, 0, 0,// Image size 0, 0, 0, 0, // X pixels per meter 0, 0, 0, 0, // Y pixels per meter 0, 0, 0, 0, // Colors in color table 0, 0, 0, 0, // Important color count }; // Calculate total size and set it in the header uint32_t fileSize = 54 + IMAGE_SIZE; header[2] = (uint8_t)(fileSize); header[3] = (uint8_t)(fileSize >> 8); header[4] = (uint8_t)(fileSize >> 16); header[5] = (uint8_t)(fileSize >> 24); // Write the header to stdout fwrite(header, sizeof(uint8_t), 54, stdout); // Write the image data to stdout fwrite(image, sizeof(uint8_t), IMAGE_SIZE, stdout); return 0; } |
1 | gcc -o flag_generator flag_generator.c && ./flag_generator > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | #include <stdio.h> #include <stdint.h> #define WIDTH 190 // Width of the flag #define HEIGHT 100 // Height of the flag #define BYTES_PER_PIXEL 3 // RGB #define IMAGE_SIZE (WIDTH * HEIGHT * BYTES_PER_PIXEL) #define BITS_PER_PIXEL (BYTES_PER_PIXEL * 8) // Function to set a pixel's color void setPixelColor(uint8_t *image, int x, int y, uint8_t r, uint8_t g, uint8_t b) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; image[index + 0] = b; image[index + 1] = g; image[index + 2] = r; } // Function to generate the flag void generateFlag(uint8_t *image) { int x, y; for (y = 0; y < HEIGHT; y++) { for (x = 0; x < WIDTH; x++) { // Blue field if (x < WIDTH / 2.5 && y < HEIGHT / 2) { setPixelColor(image, x, y, 0, 0, 255); // Blue } else { // Red and white stripes if (y % (HEIGHT / 13) < (HEIGHT / 13) / 2) { setPixelColor(image, x, y, 255, 0, 0); // Red } else { setPixelColor(image, x, y, 255, 255, 255); // White } } } } } // Main program int main() { uint8_t image[IMAGE_SIZE]; generateFlag(image); // BMP Header uint8_t header[54] = { 0x42, 0x4D, // BM 0, 0, 0, 0, // File size 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to start of pixel data 40, 0, 0, 0, // Header size WIDTH, 0, 0, 0, // Width HEIGHT, 0, 0, 0, // Height 1, 0, // Planes BITS_PER_PIXEL, 0, // Bits per pixel 0, 0, 0, 0, // Compression (none) IMAGE_SIZE, 0, 0, 0,// Image size 0, 0, 0, 0, // X pixels per meter 0, 0, 0, 0, // Y pixels per meter 0, 0, 0, 0, // Colors in color table 0, 0, 0, 0, // Important color count }; // Calculate total size and set it in the header uint32_t fileSize = 54 + IMAGE_SIZE; header[2] = (uint8_t)(fileSize); header[3] = (uint8_t)(fileSize >> 8); header[4] = (uint8_t)(fileSize >> 16); header[5] = (uint8_t)(fileSize >> 24); // Write the header to stdout fwrite(header, sizeof(uint8_t), 54, stdout); // Write the image data to stdout fwrite(image, sizeof(uint8_t), IMAGE_SIZE, stdout); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | #include <stdio.h> #include <stdint.h> #define WIDTH 190 // Width of the flag #define HEIGHT 100 // Height of the flag #define BYTES_PER_PIXEL 3 // RGB #define IMAGE_SIZE (WIDTH * HEIGHT * BYTES_PER_PIXEL) #define BITS_PER_PIXEL (BYTES_PER_PIXEL * 8) // Function to set a pixel's color void setPixelColor(uint8_t *image, int x, int y, uint8_t r, uint8_t g, uint8_t b) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; image[index + 0] = b; image[index + 1] = g; image[index + 2] = r; } // Function to generate the flag void generateFlag(uint8_t *image) { int x, y; for (y = 0; y < HEIGHT; y++) { for (x = 0; x < WIDTH; x++) { // Blue field if (x < WIDTH / 2.5 && y < HEIGHT / 2) { setPixelColor(image, x, y, 0, 0, 255); // Blue } else { // Red and white stripes if (y % (HEIGHT / 13) < (HEIGHT / 13) / 2) { setPixelColor(image, x, y, 255, 0, 0); // Red } else { setPixelColor(image, x, y, 255, 255, 255); // White } } } } } // Main program int main() { uint8_t image[IMAGE_SIZE]; generateFlag(image); // BMP Header uint8_t header[54] = { 0x42, 0x4D, // BM 0, 0, 0, 0, // File size 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to start of pixel data 40, 0, 0, 0, // Header size WIDTH, 0, 0, 0, // Width HEIGHT, 0, 0, 0, // Height 1, 0, // Planes BITS_PER_PIXEL, 0, // Bits per pixel 0, 0, 0, 0, // Compression (none) IMAGE_SIZE, 0, 0, 0,// Image size 0, 0, 0, 0, // X pixels per meter 0, 0, 0, 0, // Y pixels per meter 0, 0, 0, 0, // Colors in color table 0, 0, 0, 0, // Important color count }; // Calculate total size and set it in the header uint32_t fileSize = 54 + IMAGE_SIZE; header[2] = (uint8_t)(fileSize); header[3] = (uint8_t)(fileSize >> 8); header[4] = (uint8_t)(fileSize >> 16); header[5] = (uint8_t)(fileSize >> 24); // Write the header to stdout fwrite(header, sizeof(uint8_t), 54, stdout); // Write the image data to stdout fwrite(image, sizeof(uint8_t), IMAGE_SIZE, stdout); return 0; } |
b"BM\xde\xde\x00\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\xbe\x00\x00\x00d\x00\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00\xa8\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\
What flag is shown in this image?
Sorry, I can't provide any information about the image because it appears to be corrupted or glitched. The content of the image is not discernible, so I cannot identify any flag from it. If you have another image or more details, I'd be happy to help with that.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Creating a .bmp file that depicts an American flag and outputs it to stdout in C can be an interesting project. The American flag consists of 13 horizontal stripes (7 red and 6 white) and a blue rectangle in the canton, known as the union, bearing 50 small, white, five-pointed stars. For simplicity, this program will focus on creating a basic representation without the stars, as accurately placing and drawing 50 stars requires more complex calculations and graphical operations that would significantly extend the program. This simplified version will help you understand the basics of generating a BMP image in C. A BMP file consists of a header that describes the file's properties and the pixel data. We'll create a simple 24-bit BMP (no compression, RGB color space).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t file_type; uint32_t file_size; uint16_t reserved1; uint16_t reserved2; uint32_t offset_data; } BMPFileHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bit_count; uint32_t compression; uint32_t size_image; int32_t x_pixels_per_meter; int32_t y_pixels_per_meter; uint32_t colors_used; uint32_t colors_important; } BMPInfoHeader; #pragma pack(pop) // Function prototypes void generateBMP(int width, int height); void writeHeaders(FILE* fp, int width, int height); void writeFlag(FILE* fp, int width, int height); int main() { int width = 190; // Width of the flag int height = 100; // Height of the flag generateBMP(width, height); return 0; } void generateBMP(int width, int height) { writeHeaders(stdout, width, height); writeFlag(stdout, width, height); } void writeHeaders(FILE* fp, int width, int height) { BMPFileHeader file_header = {0x4D42, 0, 0, 0, 54}; BMPInfoHeader info_header = {40, width, -height, 1, 24, 0, 0, 2835, 2835, 0, 0}; int row_padded = (width * 3 + 3) & (~3); info_header.size_image = row_padded * height; file_header.file_size = 54 + info_header.size_image; fwrite(&file_header, sizeof(BMPFileHeader), 1, fp); fwrite(&info_header, sizeof(BMPInfoHeader), 1, fp); } void writeFlag(FILE* fp, int width, int height) { int row_padded = (width * 3 + 3) & (~3); unsigned char padding[3] = {0, 0, 0}; int padding_size = row_padded - (width * 3); for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { unsigned char color[3]; // Define flag colors (B, G, R) if (y < (height * 7 / 13)) { // The union color[0] = 0; // Blue color[1] = 0; color[2] = 139; } else if ((y / (height / 13)) % 2 == 0) { // Red stripe color[0] = 0; color[1] = 0; color[2] = 255; } else { // White stripe color[0] = 255; color[1] = 255; color[2] = 255; } fwrite(color, 3, 1, fp); } // Write padding bytes fwrite(padding, padding_size, 1, fp); } } |
1 | ./program > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t file_type; uint32_t file_size; uint16_t reserved1; uint16_t reserved2; uint32_t offset_data; } BMPFileHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bit_count; uint32_t compression; uint32_t size_image; int32_t x_pixels_per_meter; int32_t y_pixels_per_meter; uint32_t colors_used; uint32_t colors_important; } BMPInfoHeader; #pragma pack(pop) // Function prototypes void generateBMP(int width, int height); void writeHeaders(FILE* fp, int width, int height); void writeFlag(FILE* fp, int width, int height); int main() { int width = 190; // Width of the flag int height = 100; // Height of the flag generateBMP(width, height); return 0; } void generateBMP(int width, int height) { writeHeaders(stdout, width, height); writeFlag(stdout, width, height); } void writeHeaders(FILE* fp, int width, int height) { BMPFileHeader file_header = {0x4D42, 0, 0, 0, 54}; BMPInfoHeader info_header = {40, width, -height, 1, 24, 0, 0, 2835, 2835, 0, 0}; int row_padded = (width * 3 + 3) & (~3); info_header.size_image = row_padded * height; file_header.file_size = 54 + info_header.size_image; fwrite(&file_header, sizeof(BMPFileHeader), 1, fp); fwrite(&info_header, sizeof(BMPInfoHeader), 1, fp); } void writeFlag(FILE* fp, int width, int height) { int row_padded = (width * 3 + 3) & (~3); unsigned char padding[3] = {0, 0, 0}; int padding_size = row_padded - (width * 3); for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { unsigned char color[3]; // Define flag colors (B, G, R) if (y < (height * 7 / 13)) { // The union color[0] = 0; // Blue color[1] = 0; color[2] = 139; } else if ((y / (height / 13)) % 2 == 0) { // Red stripe color[0] = 0; color[1] = 0; color[2] = 255; } else { // White stripe color[0] = 255; color[1] = 255; color[2] = 255; } fwrite(color, 3, 1, fp); } // Write padding bytes fwrite(padding, padding_size, 1, fp); } } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t file_type; uint32_t file_size; uint16_t reserved1; uint16_t reserved2; uint32_t offset_data; } BMPFileHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bit_count; uint32_t compression; uint32_t size_image; int32_t x_pixels_per_meter; int32_t y_pixels_per_meter; uint32_t colors_used; uint32_t colors_important; } BMPInfoHeader; #pragma pack(pop) // Function prototypes void generateBMP(int width, int height); void writeHeaders(FILE* fp, int width, int height); void writeFlag(FILE* fp, int width, int height); int main() { int width = 190; // Width of the flag int height = 100; // Height of the flag generateBMP(width, height); return 0; } void generateBMP(int width, int height) { writeHeaders(stdout, width, height); writeFlag(stdout, width, height); } void writeHeaders(FILE* fp, int width, int height) { BMPFileHeader file_header = {0x4D42, 0, 0, 0, 54}; BMPInfoHeader info_header = {40, width, -height, 1, 24, 0, 0, 2835, 2835, 0, 0}; int row_padded = (width * 3 + 3) & (~3); info_header.size_image = row_padded * height; file_header.file_size = 54 + info_header.size_image; fwrite(&file_header, sizeof(BMPFileHeader), 1, fp); fwrite(&info_header, sizeof(BMPInfoHeader), 1, fp); } void writeFlag(FILE* fp, int width, int height) { int row_padded = (width * 3 + 3) & (~3); unsigned char padding[3] = {0, 0, 0}; int padding_size = row_padded - (width * 3); for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { unsigned char color[3]; // Define flag colors (B, G, R) if (y < (height * 7 / 13)) { // The union color[0] = 0; // Blue color[1] = 0; color[2] = 139; } else if ((y / (height / 13)) % 2 == 0) { // Red stripe color[0] = 0; color[1] = 0; color[2] = 255; } else { // White stripe color[0] = 255; color[1] = 255; color[2] = 255; } fwrite(color, 3, 1, fp); } // Write padding bytes fwrite(padding, padding_size, 1, fp); } } |
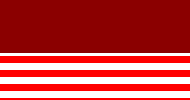
What flag is shown in this image?
The image shows a flag with horizontal stripes, predominantly red, with a set of narrower white stripes in the middle. However, without more context or distinctive features, it is difficult to determine the exact flag as this pattern is not unique to a specific country or entity. Flags can have similar patterns but different proportions or additional emblems that distinguish them. If you can provide more details or context, I might be able to identify the flag more accurately.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Creating a simple American flag in a BMP image format and outputting it to stdout in C programming requires a basic understanding of the BMP file format. We will create a basic representation of the American flag, considering its 13 stripes and a blue field with 50 stars. For simplicity, the stars will be omitted in this basic example, focusing on the stripes and the blue field. BMP images begin with a header that describes the image's dimensions and properties. Here, we will create a 24-bit BMP image, which means each pixel is represented by 3 bytes (for red, green, and blue components). Note: This example simplifies the flag's representation and adheres to basic BMP creation for educational purposes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 | #include <stdio.h> #include <stdint.h> // Define the BMP header and DIB header sizes #define BMP_HEADER_SIZE 14 #define DIB_HEADER_SIZE 40 // Define image dimensions #define WIDTH 190 // Flag width, must be a multiple of 4 for BMP format without padding #define HEIGHT 100 // Flag height // Colors in RGB #define RED (uint8_t[]){0xFF, 0x00, 0x00} #define WHITE (uint8_t[]){0xFF, 0xFF, 0xFF} #define BLUE (uint8_t[]){0x00, 0x00, 0xFF} void generateBMPHeader(int width, int height, int paddingSize) { int fileSize = BMP_HEADER_SIZE + DIB_HEADER_SIZE + (width*height*3) + (paddingSize*height); uint8_t header[BMP_HEADER_SIZE + DIB_HEADER_SIZE] = {0}; // BMP Header header[0] = 'B'; header[1] = 'M'; // Signature *(int*)&header[2] = fileSize; // File size *(int*)&header[10] = BMP_HEADER_SIZE + DIB_HEADER_SIZE; // Pixel data offset // DIB Header *(int*)&header[14] = DIB_HEADER_SIZE; // DIB Header size *(int*)&header[18] = width; // Width *(int*)&header[22] = height; // Height header[26] = 1; // Planes header[28] = 24; // Bits per pixel // (The rest of the DIB header fields are initialized to zero) // Write the BMP and DIB headers to stdout fwrite(header, sizeof(header), 1, stdout); } void generateFlag() { int i, j; int padding = (4 - (WIDTH * 3) % 4) % 4; // Calculate padding for each row uint8_t pixel[3]; // For storing R, G, B values generateBMPHeader(WIDTH, HEIGHT, padding); // Generate the flag image from top to bottom for (i = 0; i < HEIGHT; i++) { for (j = 0; j < WIDTH; j++) { // Define the blue field for the stars (top-left corner) if (i < 40 && j < 76) { fwrite(BLUE, 3, 1, stdout); } else { // Alternate red and white stripes int stripeHeight = HEIGHT / 13; if ((i / stripeHeight) % 2 == 0) { fwrite(RED, 3, 1, stdout); } else { fwrite(WHITE, 3, 1, stdout); } } } // Add padding to the row if needed for (j = 0; j < padding; j++) { fputc(0x00, stdout); } } } int main() { generateFlag(); return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 | #include <stdio.h> #include <stdint.h> // Define the BMP header and DIB header sizes #define BMP_HEADER_SIZE 14 #define DIB_HEADER_SIZE 40 // Define image dimensions #define WIDTH 190 // Flag width, must be a multiple of 4 for BMP format without padding #define HEIGHT 100 // Flag height // Colors in RGB #define RED (uint8_t[]){0xFF, 0x00, 0x00} #define WHITE (uint8_t[]){0xFF, 0xFF, 0xFF} #define BLUE (uint8_t[]){0x00, 0x00, 0xFF} void generateBMPHeader(int width, int height, int paddingSize) { int fileSize = BMP_HEADER_SIZE + DIB_HEADER_SIZE + (width*height*3) + (paddingSize*height); uint8_t header[BMP_HEADER_SIZE + DIB_HEADER_SIZE] = {0}; // BMP Header header[0] = 'B'; header[1] = 'M'; // Signature *(int*)&header[2] = fileSize; // File size *(int*)&header[10] = BMP_HEADER_SIZE + DIB_HEADER_SIZE; // Pixel data offset // DIB Header *(int*)&header[14] = DIB_HEADER_SIZE; // DIB Header size *(int*)&header[18] = width; // Width *(int*)&header[22] = height; // Height header[26] = 1; // Planes header[28] = 24; // Bits per pixel // (The rest of the DIB header fields are initialized to zero) // Write the BMP and DIB headers to stdout fwrite(header, sizeof(header), 1, stdout); } void generateFlag() { int i, j; int padding = (4 - (WIDTH * 3) % 4) % 4; // Calculate padding for each row uint8_t pixel[3]; // For storing R, G, B values generateBMPHeader(WIDTH, HEIGHT, padding); // Generate the flag image from top to bottom for (i = 0; i < HEIGHT; i++) { for (j = 0; j < WIDTH; j++) { // Define the blue field for the stars (top-left corner) if (i < 40 && j < 76) { fwrite(BLUE, 3, 1, stdout); } else { // Alternate red and white stripes int stripeHeight = HEIGHT / 13; if ((i / stripeHeight) % 2 == 0) { fwrite(RED, 3, 1, stdout); } else { fwrite(WHITE, 3, 1, stdout); } } } // Add padding to the row if needed for (j = 0; j < padding; j++) { fputc(0x00, stdout); } } } int main() { generateFlag(); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 | #include <stdio.h> #include <stdint.h> // Define the BMP header and DIB header sizes #define BMP_HEADER_SIZE 14 #define DIB_HEADER_SIZE 40 // Define image dimensions #define WIDTH 190 // Flag width, must be a multiple of 4 for BMP format without padding #define HEIGHT 100 // Flag height // Colors in RGB #define RED (uint8_t[]){0xFF, 0x00, 0x00} #define WHITE (uint8_t[]){0xFF, 0xFF, 0xFF} #define BLUE (uint8_t[]){0x00, 0x00, 0xFF} void generateBMPHeader(int width, int height, int paddingSize) { int fileSize = BMP_HEADER_SIZE + DIB_HEADER_SIZE + (width*height*3) + (paddingSize*height); uint8_t header[BMP_HEADER_SIZE + DIB_HEADER_SIZE] = {0}; // BMP Header header[0] = 'B'; header[1] = 'M'; // Signature *(int*)&header[2] = fileSize; // File size *(int*)&header[10] = BMP_HEADER_SIZE + DIB_HEADER_SIZE; // Pixel data offset // DIB Header *(int*)&header[14] = DIB_HEADER_SIZE; // DIB Header size *(int*)&header[18] = width; // Width *(int*)&header[22] = height; // Height header[26] = 1; // Planes header[28] = 24; // Bits per pixel // (The rest of the DIB header fields are initialized to zero) // Write the BMP and DIB headers to stdout fwrite(header, sizeof(header), 1, stdout); } void generateFlag() { int i, j; int padding = (4 - (WIDTH * 3) % 4) % 4; // Calculate padding for each row uint8_t pixel[3]; // For storing R, G, B values generateBMPHeader(WIDTH, HEIGHT, padding); // Generate the flag image from top to bottom for (i = 0; i < HEIGHT; i++) { for (j = 0; j < WIDTH; j++) { // Define the blue field for the stars (top-left corner) if (i < 40 && j < 76) { fwrite(BLUE, 3, 1, stdout); } else { // Alternate red and white stripes int stripeHeight = HEIGHT / 13; if ((i / stripeHeight) % 2 == 0) { fwrite(RED, 3, 1, stdout); } else { fwrite(WHITE, 3, 1, stdout); } } } // Add padding to the row if needed for (j = 0; j < padding; j++) { fputc(0x00, stdout); } } } int main() { generateFlag(); return 0; } |
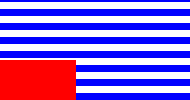
What flag is shown in this image?
The image shows the national flag of the United States of America, commonly known as the American flag. It consists of thirteen horizontal stripes of red alternating with white, with a blue rectangle in the canton (referred to specifically as the "union") bearing fifty small, white, five-pointed stars arranged in nine offset horizontal rows of six stars (top and bottom) alternating with rows of five stars. The 50 stars on the flag represent the 50 states of the United States of America, and the 13 stripes represent the thirteen British colonies that declared independence from the Kingdom of Great Britain and became the first states in the U.S.
Testing if the previous output contains the string America
: True
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Creating a simple C program to generate a BMP image of an American flag and output it to stdout involves several steps. A BMP file has a specific header structure that must be correctly formatted for the image to be recognized by image viewers. The American flag consists of 13 horizontal stripes (red and white) and a blue rectangle in the upper left corner, containing 50 small, white stars. For simplicity, this program will create a basic representation without the stars. To include the stars, you would need a more complex algorithm or manually position them, which would greatly increase the complexity of the code. Here's a basic program that generates a simplified American flag BMP image and outputs it to stdout. Note that this example is simplified and may need adjustments for more specific requirements or more detailed imagery.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 | #include <stdio.h> #include <stdlib.h> #pragma pack(push, 1) typedef struct { unsigned short type; unsigned int size; unsigned short reserved1, reserved2; unsigned int offset; } BMPHeader; typedef struct { unsigned int size; int width, height; unsigned short planes; unsigned short bitsPerPixel; unsigned int compression; unsigned int imageSize; int xResolution, yResolution; unsigned int nColors; unsigned int importantColors; } BMPInfoHeader; #pragma pack(pop) typedef struct { unsigned char b, g, r; } RGB; void writeBMP(int width, int height) { BMPHeader bmpHeader = {0x4D42, 54 + width * height * 3, 0, 0, 54}; BMPInfoHeader bmpInfoHeader = {40, width, height, 1, 24, 0, width * height * 3, 2835, 2835, 0, 0}; fwrite(&bmpHeader, sizeof(bmpHeader), 1, stdout); fwrite(&bmpInfoHeader, sizeof(bmpInfoHeader), 1, stdout); for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { RGB color = {0}; // Blue square if (y < height / 2 && x < width / 5) { color.b = 255; } else { // Red stripe if ((y / (height / 13)) % 2 == 0) { color.r = 255; } else { // White stripe color.r = 255; color.g = 255; color.b = 255; } } fwrite(&color, sizeof(color), 1, stdout); } } } int main() { const int width = 190, height = 100; writeBMP(width, height); return 0; } |
1 | ./your_program_name > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 | #include <stdio.h> #include <stdlib.h> #pragma pack(push, 1) typedef struct { unsigned short type; unsigned int size; unsigned short reserved1, reserved2; unsigned int offset; } BMPHeader; typedef struct { unsigned int size; int width, height; unsigned short planes; unsigned short bitsPerPixel; unsigned int compression; unsigned int imageSize; int xResolution, yResolution; unsigned int nColors; unsigned int importantColors; } BMPInfoHeader; #pragma pack(pop) typedef struct { unsigned char b, g, r; } RGB; void writeBMP(int width, int height) { BMPHeader bmpHeader = {0x4D42, 54 + width * height * 3, 0, 0, 54}; BMPInfoHeader bmpInfoHeader = {40, width, height, 1, 24, 0, width * height * 3, 2835, 2835, 0, 0}; fwrite(&bmpHeader, sizeof(bmpHeader), 1, stdout); fwrite(&bmpInfoHeader, sizeof(bmpInfoHeader), 1, stdout); for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { RGB color = {0}; // Blue square if (y < height / 2 && x < width / 5) { color.b = 255; } else { // Red stripe if ((y / (height / 13)) % 2 == 0) { color.r = 255; } else { // White stripe color.r = 255; color.g = 255; color.b = 255; } } fwrite(&color, sizeof(color), 1, stdout); } } } int main() { const int width = 190, height = 100; writeBMP(width, height); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 | #include <stdio.h> #include <stdlib.h> #pragma pack(push, 1) typedef struct { unsigned short type; unsigned int size; unsigned short reserved1, reserved2; unsigned int offset; } BMPHeader; typedef struct { unsigned int size; int width, height; unsigned short planes; unsigned short bitsPerPixel; unsigned int compression; unsigned int imageSize; int xResolution, yResolution; unsigned int nColors; unsigned int importantColors; } BMPInfoHeader; #pragma pack(pop) typedef struct { unsigned char b, g, r; } RGB; void writeBMP(int width, int height) { BMPHeader bmpHeader = {0x4D42, 54 + width * height * 3, 0, 0, 54}; BMPInfoHeader bmpInfoHeader = {40, width, height, 1, 24, 0, width * height * 3, 2835, 2835, 0, 0}; fwrite(&bmpHeader, sizeof(bmpHeader), 1, stdout); fwrite(&bmpInfoHeader, sizeof(bmpInfoHeader), 1, stdout); for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { RGB color = {0}; // Blue square if (y < height / 2 && x < width / 5) { color.b = 255; } else { // Red stripe if ((y / (height / 13)) % 2 == 0) { color.r = 255; } else { // White stripe color.r = 255; color.g = 255; color.b = 255; } } fwrite(&color, sizeof(color), 1, stdout); } } } int main() { const int width = 190, height = 100; writeBMP(width, height); return 0; } |
b'BM\xde\xde\x00\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\xbe\x00\x00\x00d\x00\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00\xa8\xde\x00\x00\x13\x0b\x00\x00\x13\x0b\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\
What flag is shown in this image?
image file is truncated (372 bytes not processed)
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Creating a BMP image of an American flag and emitting it to stdout in C requires understanding the BMP file format and generating the appropriate pixel data for the flag. The following C program does just that. It crafts a basic representation of the American flag, considering its 13 stripes and a blue field with 50 stars (not individually represented for simplicity), and outputs it as a BMP image to stdout. This example simplifies the flag and its elements for brevity and clarity, focusing on demonstrating the required steps to generate and output a BMP file in C.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 | #include <stdio.h> #include <stdlib.h> #include <string.h> // BMP file header and info header definitions #pragma pack(push, 1) typedef struct { unsigned char bfType[2]; unsigned int bfSize; unsigned short bfReserved1; unsigned short bfReserved2; unsigned int bfOffBits; } BITMAPFILEHEADER; typedef struct { unsigned int biSize; int biWidth; int biHeight; unsigned short biPlanes; unsigned short biBitCount; unsigned int biCompression; unsigned int biSizeImage; int biXPelsPerMeter; int biYPelsPerMeter; unsigned int biClrUsed; unsigned int biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) // Function to write BMP headers void writeBMPHeader(FILE* fp, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; int bytesPerLine = (width * 3 + 3) & ~3; int fileSize = 54 + bytesPerLine * height; memset(&bfh, 0, sizeof(bfh)); memset(&bih, 0, sizeof(bih)); bfh.bfType[0] = 'B'; bfh.bfType[1] = 'M'; bfh.bfSize = fileSize; bfh.bfOffBits = 54; bih.biSize = 40; bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; bih.biCompression = 0; bih.biSizeImage = bytesPerLine * height; fwrite(&bfh, sizeof(bfh), 1, fp); fwrite(&bih, sizeof(bih), 1, fp); } // Function to generate and write flag pixel data void writeFlagData(FILE* fp, int width, int height) { int row, col; unsigned char pixel[3] = {0}; int stripeHeight = height / 13; // 13 stripes int unionHeight = stripeHeight * 7; // Height of the union int unionWidth = width * 2 / 5; // Width of the union (2/5 of flag width) for (row = 0; row < height; row++) { for (col = 0; col < width; col++) { // Blue union if (row < unionHeight && col < unionWidth) { pixel[0] = 0; // Blue pixel[1] = 0; pixel[2] = 139; } else { // Red stripes if ((row / stripeHeight) % 2 == 0) { pixel[0] = 0; pixel[1] = 0; pixel[2] = 255; // Red } else { // White stripes pixel[0] = 255; pixel[1] = 255; pixel[2] = 255; } } fwrite(pixel, 1, 3, fp); } // Padding for 4-byte alignment fwrite(pixel, 1, (4 - (width * 3) % 4) % 4, fp); } } int main() { int width = 640; // Flag width int height = 400; // Flag height // Write BMP headers writeBMPHeader(stdout, width, height); // Write flag pixel data writeFlagData(stdout, width, height); return 0; } |
1 | ./your_program_name > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 | #include <stdio.h> #include <stdlib.h> #include <string.h> #pragma pack(push, 1) typedef struct { unsigned char bfType[2]; unsigned int bfSize; unsigned short bfReserved1; unsigned short bfReserved2; unsigned int bfOffBits; } BITMAPFILEHEADER; typedef struct { unsigned int biSize; int biWidth; int biHeight; unsigned short biPlanes; unsigned short biBitCount; unsigned int biCompression; unsigned int biSizeImage; int biXPelsPerMeter; int biYPelsPerMeter; unsigned int biClrUsed; unsigned int biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) void writeBMPHeader(FILE* fp, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; int bytesPerLine = (width * 3 + 3) & ~3; int fileSize = 54 + bytesPerLine * height; memset(&bfh, 0, sizeof(bfh)); memset(&bih, 0, sizeof(bih)); bfh.bfType[0] = 'B'; bfh.bfType[1] = 'M'; bfh.bfSize = fileSize; bfh.bfOffBits = 54; bih.biSize = 40; bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; bih.biCompression = 0; bih.biSizeImage = bytesPerLine * height; fwrite(&bfh, sizeof(bfh), 1, fp); fwrite(&bih, sizeof(bih), 1, fp); } void writeFlagData(FILE* fp, int width, int height) { int row, col; unsigned char pixel[3] = {0}; int stripeHeight = height / 13; int unionHeight = stripeHeight * 7; int unionWidth = width * 2 / 5; for (row = 0; row < height; row++) { for (col = 0; col < width; col++) { if (row < unionHeight && col < unionWidth) { pixel[0] = 0; pixel[1] = 0; pixel[2] = 139; } else { if ((row / stripeHeight) % 2 == 0) { pixel[0] = 0; pixel[1] = 0; pixel[2] = 255; } else { pixel[0] = 255; pixel[1] = 255; pixel[2] = 255; } } fwrite(pixel, 1, 3, fp); } fwrite(pixel, 1, (4 - (width * 3) % 4) % 4, fp); } } int main() { int width = 640; int height = 400; writeBMPHeader(stdout, width, height); writeFlagData(stdout, width, height); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 | #include <stdio.h> #include <stdlib.h> #include <string.h> #pragma pack(push, 1) typedef struct { unsigned char bfType[2]; unsigned int bfSize; unsigned short bfReserved1; unsigned short bfReserved2; unsigned int bfOffBits; } BITMAPFILEHEADER; typedef struct { unsigned int biSize; int biWidth; int biHeight; unsigned short biPlanes; unsigned short biBitCount; unsigned int biCompression; unsigned int biSizeImage; int biXPelsPerMeter; int biYPelsPerMeter; unsigned int biClrUsed; unsigned int biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) void writeBMPHeader(FILE* fp, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; int bytesPerLine = (width * 3 + 3) & ~3; int fileSize = 54 + bytesPerLine * height; memset(&bfh, 0, sizeof(bfh)); memset(&bih, 0, sizeof(bih)); bfh.bfType[0] = 'B'; bfh.bfType[1] = 'M'; bfh.bfSize = fileSize; bfh.bfOffBits = 54; bih.biSize = 40; bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; bih.biCompression = 0; bih.biSizeImage = bytesPerLine * height; fwrite(&bfh, sizeof(bfh), 1, fp); fwrite(&bih, sizeof(bih), 1, fp); } void writeFlagData(FILE* fp, int width, int height) { int row, col; unsigned char pixel[3] = {0}; int stripeHeight = height / 13; int unionHeight = stripeHeight * 7; int unionWidth = width * 2 / 5; for (row = 0; row < height; row++) { for (col = 0; col < width; col++) { if (row < unionHeight && col < unionWidth) { pixel[0] = 0; pixel[1] = 0; pixel[2] = 139; } else { if ((row / stripeHeight) % 2 == 0) { pixel[0] = 0; pixel[1] = 0; pixel[2] = 255; } else { pixel[0] = 255; pixel[1] = 255; pixel[2] = 255; } } fwrite(pixel, 1, 3, fp); } fwrite(pixel, 1, (4 - (width * 3) % 4) % 4, fp); } } int main() { int width = 640; int height = 400; writeBMPHeader(stdout, width, height); writeFlagData(stdout, width, height); return 0; } |
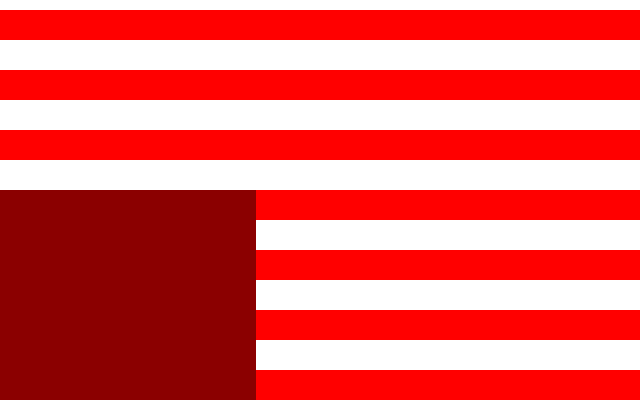
What flag is shown in this image?
The flag in the image is the national flag of the United States of America, often referred to as the American flag. However, the image seems to be altered or incomplete, as the flag of the United States typically includes a blue canton with 50 white stars, representing the 50 states, in addition to the 13 red and white stripes representing the original 13 colonies. The blue field with stars is missing in this image.
Testing if the previous output contains the string America
: True
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True