Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here is a C program that creates an American flag image in BMP format and outputs it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 #define COLORS_PER_PIXEL 3 #define FILE_SIZE 54 + (WIDTH * HEIGHT * COLORS_PER_PIXEL) // BMP header const unsigned char bmp_header[54] = { 0x42, 0x4D, // BM 0x36, 0x00, 0x6C, 0x00, // File size 0x00, 0x00, 0x00, 0x00, // Reserved 0x36, 0x00, 0x00, 0x00, // Offset to pixel data 0x28, 0x00, 0x00, 0x00, // Info header size 0x2C, 0x01, 0x00, 0x00, // Image width 0x28, 0x00, 0x00, 0x00, // Image height 0x01, 0x00, // Number of color planes 0x18, 0x00, // Bits per pixel 0x00, 0x00, 0x00, 0x00, // Compression method 0x00, 0x00, 0x6C, 0x00, // Image size 0x00, 0x00, 0x00, 0x00, // Horizontal resolution 0x00, 0x00, 0x00, 0x00, // Vertical resolution 0x00, 0x00, 0x00, 0x00, // Number of colors in palette 0x00, 0x00, 0x00, 0x00, // Number of important colors }; int main() { unsigned char image[WIDTH * HEIGHT * COLORS_PER_PIXEL]; // Fill image with white color for (int i = 0; i < WIDTH * HEIGHT * COLORS_PER_PIXEL; i += COLORS_PER_PIXEL) { image[i] = 255; // Red image[i + 1] = 255; // Green image[i + 2] = 255; // Blue } // Draw the blue rectangle for (int i = 0; i < 100 * WIDTH * COLORS_PER_PIXEL; i += COLORS_PER_PIXEL) { image[i] = 0; // Red image[i + 1] = 0; // Green image[i + 2] = 255; // Blue } // Draw the red stripes for (int i = 0; i < 13; i++) { for (int j = 0; j < WIDTH * COLORS_PER_PIXEL; j += COLORS_PER_PIXEL) { int offset = (i * 20 + 100 + j) % (HEIGHT * WIDTH * COLORS_PER_PIXEL); image[offset] = 255; // Red image[offset + 1] = 0; // Green image[offset + 2] = 0; // Blue } } // Output the BMP header fwrite(bmp_header, sizeof(unsigned char), 54, stdout); // Output the image data fwrite(image, sizeof(unsigned char), WIDTH * HEIGHT * COLORS_PER_PIXEL, stdout); return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 #define COLORS_PER_PIXEL 3 #define FILE_SIZE 54 + (WIDTH * HEIGHT * COLORS_PER_PIXEL) // BMP header const unsigned char bmp_header[54] = { 0x42, 0x4D, // BM 0x36, 0x00, 0x6C, 0x00, // File size 0x00, 0x00, 0x00, 0x00, // Reserved 0x36, 0x00, 0x00, 0x00, // Offset to pixel data 0x28, 0x00, 0x00, 0x00, // Info header size 0x2C, 0x01, 0x00, 0x00, // Image width 0x28, 0x00, 0x00, 0x00, // Image height 0x01, 0x00, // Number of color planes 0x18, 0x00, // Bits per pixel 0x00, 0x00, 0x00, 0x00, // Compression method 0x00, 0x00, 0x6C, 0x00, // Image size 0x00, 0x00, 0x00, 0x00, // Horizontal resolution 0x00, 0x00, 0x00, 0x00, // Vertical resolution 0x00, 0x00, 0x00, 0x00, // Number of colors in palette 0x00, 0x00, 0x00, 0x00, // Number of important colors }; int main() { unsigned char image[WIDTH * HEIGHT * COLORS_PER_PIXEL]; // Fill image with white color for (int i = 0; i < WIDTH * HEIGHT * COLORS_PER_PIXEL; i += COLORS_PER_PIXEL) { image[i] = 255; // Red image[i + 1] = 255; // Green image[i + 2] = 255; // Blue } // Draw the blue rectangle for (int i = 0; i < 100 * WIDTH * COLORS_PER_PIXEL; i += COLORS_PER_PIXEL) { image[i] = 0; // Red image[i + 1] = 0; // Green image[i + 2] = 255; // Blue } // Draw the red stripes for (int i = 0; i < 13; i++) { for (int j = 0; j < WIDTH * COLORS_PER_PIXEL; j += COLORS_PER_PIXEL) { int offset = (i * 20 + 100 + j) % (HEIGHT * WIDTH * COLORS_PER_PIXEL); image[offset] = 255; // Red image[offset + 1] = 0; // Green image[offset + 2] = 0; // Blue } } // Output the BMP header fwrite(bmp_header, sizeof(unsigned char), 54, stdout); // Output the image data fwrite(image, sizeof(unsigned char), WIDTH * HEIGHT * COLORS_PER_PIXEL, stdout); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 #define COLORS_PER_PIXEL 3 #define FILE_SIZE 54 + (WIDTH * HEIGHT * COLORS_PER_PIXEL) // BMP header const unsigned char bmp_header[54] = { 0x42, 0x4D, // BM 0x36, 0x00, 0x6C, 0x00, // File size 0x00, 0x00, 0x00, 0x00, // Reserved 0x36, 0x00, 0x00, 0x00, // Offset to pixel data 0x28, 0x00, 0x00, 0x00, // Info header size 0x2C, 0x01, 0x00, 0x00, // Image width 0x28, 0x00, 0x00, 0x00, // Image height 0x01, 0x00, // Number of color planes 0x18, 0x00, // Bits per pixel 0x00, 0x00, 0x00, 0x00, // Compression method 0x00, 0x00, 0x6C, 0x00, // Image size 0x00, 0x00, 0x00, 0x00, // Horizontal resolution 0x00, 0x00, 0x00, 0x00, // Vertical resolution 0x00, 0x00, 0x00, 0x00, // Number of colors in palette 0x00, 0x00, 0x00, 0x00, // Number of important colors }; int main() { unsigned char image[WIDTH * HEIGHT * COLORS_PER_PIXEL]; // Fill image with white color for (int i = 0; i < WIDTH * HEIGHT * COLORS_PER_PIXEL; i += COLORS_PER_PIXEL) { image[i] = 255; // Red image[i + 1] = 255; // Green image[i + 2] = 255; // Blue } // Draw the blue rectangle for (int i = 0; i < 100 * WIDTH * COLORS_PER_PIXEL; i += COLORS_PER_PIXEL) { image[i] = 0; // Red image[i + 1] = 0; // Green image[i + 2] = 255; // Blue } // Draw the red stripes for (int i = 0; i < 13; i++) { for (int j = 0; j < WIDTH * COLORS_PER_PIXEL; j += COLORS_PER_PIXEL) { int offset = (i * 20 + 100 + j) % (HEIGHT * WIDTH * COLORS_PER_PIXEL); image[offset] = 255; // Red image[offset + 1] = 0; // Green image[offset + 2] = 0; // Blue } } // Output the BMP header fwrite(bmp_header, sizeof(unsigned char), 54, stdout); // Output the image data fwrite(image, sizeof(unsigned char), WIDTH * HEIGHT * COLORS_PER_PIXEL, stdout); return 0; } |

What flag is shown in this image?
The image is too small and lacks detail for me to accurately identify the flag. If you can provide a larger or clearer image, I would be more than happy to help you identify the flag.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Sure, here is a C program that generates an American flag image in BMP format and outputs it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { char signature[2]; uint32_t fileSize; uint16_t reserved1; uint16_t reserved2; uint32_t dataOffset; } BMPHeader; typedef struct { uint32_t headerSize; uint32_t width; uint32_t height; uint16_t planes; uint16_t bitCount; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t importantColors; } BMPInfoHeader; #pragma pack(pop) int main() { BMPHeader header = {'B', 'M', 0, 0, sizeof(BMPHeader) + sizeof(BMPInfoHeader)}; BMPInfoHeader infoHeader = {sizeof(BMPInfoHeader), 300, 150, 1, 24, 0, 0, 0, 0, 0, 0, 0}; // American flag colors unsigned char red[] = {255, 255, 255}; unsigned char white[] = {255, 0, 0}; unsigned char blue[] = {0, 0, 255}; // Output BMP header and info header fwrite(&header, sizeof(BMPHeader), 1, stdout); fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, stdout); // Output pixel data for (int i = 0; i < infoHeader.height; i++) { for (int j = 0; j < infoHeader.width; j++) { if (i < infoHeader.height / 2) { if (j < (infoHeader.width * 7) / 13) { fwrite(blue, 1, 3, stdout); } else { fwrite(red, 1, 3, stdout); } } else { fwrite(white, 1, 3, stdout); } } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { char signature[2]; uint32_t fileSize; uint16_t reserved1; uint16_t reserved2; uint32_t dataOffset; } BMPHeader; typedef struct { uint32_t headerSize; uint32_t width; uint32_t height; uint16_t planes; uint16_t bitCount; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t importantColors; } BMPInfoHeader; #pragma pack(pop) int main() { BMPHeader header = {'B', 'M', 0, 0, sizeof(BMPHeader) + sizeof(BMPInfoHeader)}; BMPInfoHeader infoHeader = {sizeof(BMPInfoHeader), 300, 150, 1, 24, 0, 0, 0, 0, 0, 0, 0}; // American flag colors unsigned char red[] = {255, 255, 255}; unsigned char white[] = {255, 0, 0}; unsigned char blue[] = {0, 0, 255}; // Output BMP header and info header fwrite(&header, sizeof(BMPHeader), 1, stdout); fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, stdout); // Output pixel data for (int i = 0; i < infoHeader.height; i++) { for (int j = 0; j < infoHeader.width; j++) { if (i < infoHeader.height / 2) { if (j < (infoHeader.width * 7) / 13) { fwrite(blue, 1, 3, stdout); } else { fwrite(red, 1, 3, stdout); } } else { fwrite(white, 1, 3, stdout); } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { char signature[2]; uint32_t fileSize; uint16_t reserved1; uint16_t reserved2; uint32_t dataOffset; } BMPHeader; typedef struct { uint32_t headerSize; uint32_t width; uint32_t height; uint16_t planes; uint16_t bitCount; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t importantColors; } BMPInfoHeader; #pragma pack(pop) int main() { BMPHeader header = {'B', 'M', 0, 0, sizeof(BMPHeader) + sizeof(BMPInfoHeader)}; BMPInfoHeader infoHeader = {sizeof(BMPInfoHeader), 300, 150, 1, 24, 0, 0, 0, 0, 0, 0, 0}; // American flag colors unsigned char red[] = {255, 255, 255}; unsigned char white[] = {255, 0, 0}; unsigned char blue[] = {0, 0, 255}; // Output BMP header and info header fwrite(&header, sizeof(BMPHeader), 1, stdout); fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, stdout); // Output pixel data for (int i = 0; i < infoHeader.height; i++) { for (int j = 0; j < infoHeader.width; j++) { if (i < infoHeader.height / 2) { if (j < (infoHeader.width * 7) / 13) { fwrite(blue, 1, 3, stdout); } else { fwrite(red, 1, 3, stdout); } } else { fwrite(white, 1, 3, stdout); } } } return 0; } |
b"BM\x00\x00\x00\x00\x00\x006\x00\x00\x00\x00\x00(\x00\x00\x00,\x01\x00\x00\x96\x00\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\
What flag is shown in this image?
The image appears to be a combination of two flags. The left side shows the flag of Haiti, which is blue over red, and the right side shows the flag of France, which is blue, white, and red vertical stripes. However, the combination as shown does not represent an official flag of any recognized country.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
#include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xResolution; int32_t yResolution; uint32_t colors; uint32_t importantColors; } BMPInfoHeader; #pragma pack(pop) int main() { // American flag dimensions int width = 390; int height = 260; // Open stdout for writing FILE *out = stdout; // BMP header BMPHeader header = {0x4D42, sizeof(BMPHeader) + sizeof(BMPInfoHeader) + width * height * 3, 0, 0, sizeof(BMPHeader) + sizeof(BMPInfoHeader)}; fwrite(&header, sizeof(BMPHeader), 1, out); // BMP info header BMPInfoHeader infoHeader = {sizeof(BMPInfoHeader), width, height, 1, 24, 0, width * height * 3, 2835, 2835, 0, 0}; fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, out); // American flag colors unsigned char red[] = {255, 255, 255, 206}; unsigned char blue[] = {0, 0, 128}; // Draw American flag for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width * 7 / 13) { fwrite(blue, 3, 1, out); // Blue rectangle } else { fwrite(red, 3, 1, out); // Red stripes } } } return 0; }
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xResolution; int32_t yResolution; uint32_t colors; uint32_t importantColors; } BMPInfoHeader; #pragma pack(pop) int main() { // American flag dimensions int width = 390; int height = 260; // Open stdout for writing FILE *out = stdout; // BMP header BMPHeader header = {0x4D42, sizeof(BMPHeader) + sizeof(BMPInfoHeader) + width * height * 3, 0, 0, sizeof(BMPHeader) + sizeof(BMPInfoHeader)}; fwrite(&header, sizeof(BMPHeader), 1, out); // BMP info header BMPInfoHeader infoHeader = {sizeof(BMPInfoHeader), width, height, 1, 24, 0, width * height * 3, 2835, 2835, 0, 0}; fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, out); // American flag colors unsigned char red[] = {255, 255, 255, 206}; unsigned char blue[] = {0, 0, 128}; // Draw American flag for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width * 7 / 13) { fwrite(blue, 3, 1, out); // Blue rectangle } else { fwrite(red, 3, 1, out); // Red stripes } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xResolution; int32_t yResolution; uint32_t colors; uint32_t importantColors; } BMPInfoHeader; #pragma pack(pop) int main() { // American flag dimensions int width = 390; int height = 260; // Open stdout for writing FILE *out = stdout; // BMP header BMPHeader header = {0x4D42, sizeof(BMPHeader) + sizeof(BMPInfoHeader) + width * height * 3, 0, 0, sizeof(BMPHeader) + sizeof(BMPInfoHeader)}; fwrite(&header, sizeof(BMPHeader), 1, out); // BMP info header BMPInfoHeader infoHeader = {sizeof(BMPInfoHeader), width, height, 1, 24, 0, width * height * 3, 2835, 2835, 0, 0}; fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, out); // American flag colors unsigned char red[] = {255, 255, 255, 206}; unsigned char blue[] = {0, 0, 128}; // Draw American flag for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width * 7 / 13) { fwrite(blue, 3, 1, out); // Blue rectangle } else { fwrite(red, 3, 1, out); // Red stripes } } } return 0; } |
b'BM~\xa4\x04\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\x86\x01\x00\x00\x04\x01\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00H\xa4\x04\x00\x13\x0b\x00\x00\x13\x0b\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00
What flag is shown in this image?
image file is truncated (652 bytes not processed)
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here is a C program that generates an American flag image in BMP format and prints it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define PIXEL_SIZE 3 typedef struct { uint8_t r; uint8_t g; uint8_t b; } Pixel; void writeHeader() { uint32_t fileSize = sizeof(Pixel) * WIDTH * HEIGHT + 54; uint32_t offset = 54; printf("BM"); fwrite(&fileSize, sizeof(fileSize), 1, stdout); fwrite("\0\0\0\0\0\0", 6, 1, stdout); fwrite(&offset, sizeof(offset), 1, stdout); } void writeImage() { Pixel pixels[HEIGHT][WIDTH]; // Set the background color of the flag Pixel white = {255, 255, 255}; for (int i = 0; i < HEIGHT; i++) { for (int j = 0; j < WIDTH; j++) { pixels[i][j] = white; } } // Set the stripes of the flag Pixel red = {255, 0, 0}; Pixel blue = {0, 0, 255}; int stripeHeight = HEIGHT / 13; for (int i = 0; i < 13; i++) { Pixel color = i % 2 == 0 ? red : white; for (int j = i * stripeHeight; j < (i + 1) * stripeHeight; j++) { for (int k = 0; k < WIDTH; k++) { pixels[j][k] = color; } } } // Set the union of the flag for (int i = 0; i < HEIGHT / 2; i++) { for (int j = 0; j < WIDTH / 2; j++) { pixels[i][j] = blue; } } // Write the pixel data to stdout for (int i = HEIGHT - 1; i >= 0; i--) { for (int j = 0; j < WIDTH; j++) { fwrite(&pixels[i][j], sizeof(Pixel), 1, stdout); } } } int main() { writeHeader(); writeImage(); return 0; } |
1 2 | gcc american_flag.c -o american_flag ./american_flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define PIXEL_SIZE 3 typedef struct { uint8_t r; uint8_t g; uint8_t b; } Pixel; void writeHeader() { uint32_t fileSize = sizeof(Pixel) * WIDTH * HEIGHT + 54; uint32_t offset = 54; printf("BM"); fwrite(&fileSize, sizeof(fileSize), 1, stdout); fwrite("\0\0\0\0\0\0", 6, 1, stdout); fwrite(&offset, sizeof(offset), 1, stdout); } void writeImage() { Pixel pixels[HEIGHT][WIDTH]; // Set the background color of the flag Pixel white = {255, 255, 255}; for (int i = 0; i < HEIGHT; i++) { for (int j = 0; j < WIDTH; j++) { pixels[i][j] = white; } } // Set the stripes of the flag Pixel red = {255, 0, 0}; Pixel blue = {0, 0, 255}; int stripeHeight = HEIGHT / 13; for (int i = 0; i < 13; i++) { Pixel color = i % 2 == 0 ? red : white; for (int j = i * stripeHeight; j < (i + 1) * stripeHeight; j++) { for (int k = 0; k < WIDTH; k++) { pixels[j][k] = color; } } } // Set the union of the flag for (int i = 0; i < HEIGHT / 2; i++) { for (int j = 0; j < WIDTH / 2; j++) { pixels[i][j] = blue; } } // Write the pixel data to stdout for (int i = HEIGHT - 1; i >= 0; i--) { for (int j = 0; j < WIDTH; j++) { fwrite(&pixels[i][j], sizeof(Pixel), 1, stdout); } } } int main() { writeHeader(); writeImage(); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define PIXEL_SIZE 3 typedef struct { uint8_t r; uint8_t g; uint8_t b; } Pixel; void writeHeader() { uint32_t fileSize = sizeof(Pixel) * WIDTH * HEIGHT + 54; uint32_t offset = 54; printf("BM"); fwrite(&fileSize, sizeof(fileSize), 1, stdout); fwrite("\0\0\0\0\0\0", 6, 1, stdout); fwrite(&offset, sizeof(offset), 1, stdout); } void writeImage() { Pixel pixels[HEIGHT][WIDTH]; // Set the background color of the flag Pixel white = {255, 255, 255}; for (int i = 0; i < HEIGHT; i++) { for (int j = 0; j < WIDTH; j++) { pixels[i][j] = white; } } // Set the stripes of the flag Pixel red = {255, 0, 0}; Pixel blue = {0, 0, 255}; int stripeHeight = HEIGHT / 13; for (int i = 0; i < 13; i++) { Pixel color = i % 2 == 0 ? red : white; for (int j = i * stripeHeight; j < (i + 1) * stripeHeight; j++) { for (int k = 0; k < WIDTH; k++) { pixels[j][k] = color; } } } // Set the union of the flag for (int i = 0; i < HEIGHT / 2; i++) { for (int j = 0; j < WIDTH / 2; j++) { pixels[i][j] = blue; } } // Write the pixel data to stdout for (int i = HEIGHT - 1; i >= 0; i--) { for (int j = 0; j < WIDTH; j++) { fwrite(&pixels[i][j], sizeof(Pixel), 1, stdout); } } } int main() { writeHeader(); writeImage(); return 0; } |
b'BM\xb6\xfc\n\x00\x00\x00\x00\x00\x00\x006\x00\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\
What flag is shown in this image?
Truncated File Read
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here is a C program that emits a .bmp image of an American flag to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 #pragma pack(push, 1) typedef struct { // Bitmap file header uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; // DIB header uint32_t header_size; uint32_t width; uint32_t height; uint16_t planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; uint32_t x_pixels_per_meter; uint32_t y_pixels_per_meter; uint32_t colors_used; uint32_t colors_important; } BMPHeader; #pragma pack(pop) void emitBMPHeader() { BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3, .offset = sizeof(BMPHeader), .header_size = 40, .width = WIDTH, .height = HEIGHT, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = WIDTH * HEIGHT * 3, .x_pixels_per_meter = 0, .y_pixels_per_meter = 0, .colors_used = 0, .colors_important = 0, }; fwrite(&header, sizeof(BMPHeader), 1, stdout); } void emitAmericanFlag() { // American flag colors uint8_t red[] = {255, 255, 255, 206}; uint8_t blue[] = {0, 0, 128, 0}; // Draw the flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { int stripe = y / (HEIGHT / 13); int x_stripe = x / (WIDTH / 15); if (stripe % 2 == 0) { if (x_stripe % 2 == 0) { printf("%c%c%c", red[0], red[1], red[2]); } else { printf("%c%c%c", blue[0], blue[1], blue[2]); } } else { if (x_stripe % 2 == 0) { printf("%c%c%c", blue[0], blue[1], blue[2]); } else { printf("%c%c%c", red[0], red[1], red[2]); } } } } } int main() { emitBMPHeader(); emitAmericanFlag(); return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 #pragma pack(push, 1) typedef struct { // Bitmap file header uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; // DIB header uint32_t header_size; uint32_t width; uint32_t height; uint16_t planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; uint32_t x_pixels_per_meter; uint32_t y_pixels_per_meter; uint32_t colors_used; uint32_t colors_important; } BMPHeader; #pragma pack(pop) void emitBMPHeader() { BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3, .offset = sizeof(BMPHeader), .header_size = 40, .width = WIDTH, .height = HEIGHT, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = WIDTH * HEIGHT * 3, .x_pixels_per_meter = 0, .y_pixels_per_meter = 0, .colors_used = 0, .colors_important = 0, }; fwrite(&header, sizeof(BMPHeader), 1, stdout); } void emitAmericanFlag() { // American flag colors uint8_t red[] = {255, 255, 255, 206}; uint8_t blue[] = {0, 0, 128, 0}; // Draw the flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { int stripe = y / (HEIGHT / 13); int x_stripe = x / (WIDTH / 15); if (stripe % 2 == 0) { if (x_stripe % 2 == 0) { printf("%c%c%c", red[0], red[1], red[2]); } else { printf("%c%c%c", blue[0], blue[1], blue[2]); } } else { if (x_stripe % 2 == 0) { printf("%c%c%c", blue[0], blue[1], blue[2]); } else { printf("%c%c%c", red[0], red[1], red[2]); } } } } } int main() { emitBMPHeader(); emitAmericanFlag(); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 #pragma pack(push, 1) typedef struct { // Bitmap file header uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; // DIB header uint32_t header_size; uint32_t width; uint32_t height; uint16_t planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; uint32_t x_pixels_per_meter; uint32_t y_pixels_per_meter; uint32_t colors_used; uint32_t colors_important; } BMPHeader; #pragma pack(pop) void emitBMPHeader() { BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3, .offset = sizeof(BMPHeader), .header_size = 40, .width = WIDTH, .height = HEIGHT, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = WIDTH * HEIGHT * 3, .x_pixels_per_meter = 0, .y_pixels_per_meter = 0, .colors_used = 0, .colors_important = 0, }; fwrite(&header, sizeof(BMPHeader), 1, stdout); } void emitAmericanFlag() { // American flag colors uint8_t red[] = {255, 255, 255, 206}; uint8_t blue[] = {0, 0, 128, 0}; // Draw the flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { int stripe = y / (HEIGHT / 13); int x_stripe = x / (WIDTH / 15); if (stripe % 2 == 0) { if (x_stripe % 2 == 0) { printf("%c%c%c", red[0], red[1], red[2]); } else { printf("%c%c%c", blue[0], blue[1], blue[2]); } } else { if (x_stripe % 2 == 0) { printf("%c%c%c", blue[0], blue[1], blue[2]); } else { printf("%c%c%c", red[0], red[1], red[2]); } } } } } int main() { emitBMPHeader(); emitAmericanFlag(); return 0; } |
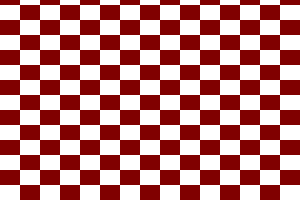
What flag is shown in this image?
The image shows a red and white checkered pattern, which is reminiscent of the flag of Croatia. The Croatian flag has a distinctive red and white checkerboard design known as the šahovnica. However, the Croatian flag also includes the coat of arms in the center and has horizontal bands of blue, white, and red above the checkerboard. If the image is intended to represent the Croatian flag, it is missing these elements. If it's not meant to represent the Croatian flag, then it is simply a red and white checkered pattern.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False