Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | #include <stdio.h> #include <stdlib.h> // Define constants for the flag dimensions #define WIDTH 640 #define HEIGHT 480 #define STRIPE_HEIGHT (HEIGHT / 13) #define UNION_WIDTH ((7 * WIDTH) / 13) #define UNION_HEIGHT (7 * STRIPE_HEIGHT) // Define colors as RGB structs typedef struct { unsigned char b, g, r; } RGB; const RGB RED = {0, 0, 255}; const RGB WHITE = {255, 255, 255}; const RGB BLUE = {255, 0, 0}; int main() { // Allocate memory for the pixel data RGB* data = (RGB*)malloc(WIDTH * HEIGHT * sizeof(RGB)); if (data == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } // Generate the flag pattern for (int y = 0; y < HEIGHT; ++y) { for (int x = 0; x < WIDTH; ++x) { // Determine if the current pixel is within the union (blue rectangle) int inUnion = (x < UNION_WIDTH) && (y < UNION_HEIGHT); // Determine if the current pixel is on a red stripe int onRedStripe = (y / STRIPE_HEIGHT) % 2 == 0; // Set the pixel color based on its position if (inUnion) { if (x < (UNION_WIDTH / 13) * 6 && y < (UNION_HEIGHT / 7) * 5) { data[y * WIDTH + x] = WHITE; // White star area } else { data[y * WIDTH + x] = BLUE; // Blue background } } else if (onRedStripe) { data[y * WIDTH + x] = RED; // Red stripe } else { data[y * WIDTH + x] = WHITE; // White stripe } } } // Write the BMP header unsigned char header[54] = { 0x42, 0x4D, 0x36, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x36, 0x00, 0x00, 0x00, 0x28, 0x00, 0x00, 0x00, 0x80, 0x02, 0x00, 0x00, 0xE0, 0x01, 0x00, 0x00, 0x01, 0x00, 0x18, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}; // Update header with image dimensions *(unsigned int*)&header[18] = WIDTH; *(unsigned int*)&header[22] = HEIGHT; // Write the header and pixel data to stdout fwrite(header, sizeof(unsigned char), 54, stdout); fwrite(data, sizeof(RGB), WIDTH * HEIGHT, stdout); // Free the allocated memory free(data); return 0; } |
1 2 | gcc flag.c -o flag |
1 2 | ./flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | #include <stdio.h> #include <stdlib.h> // Define constants for the flag dimensions #define WIDTH 640 #define HEIGHT 480 #define STRIPE_HEIGHT (HEIGHT / 13) #define UNION_WIDTH ((7 * WIDTH) / 13) #define UNION_HEIGHT (7 * STRIPE_HEIGHT) // Define colors as RGB structs typedef struct { unsigned char b, g, r; } RGB; const RGB RED = {0, 0, 255}; const RGB WHITE = {255, 255, 255}; const RGB BLUE = {255, 0, 0}; int main() { // Allocate memory for the pixel data RGB* data = (RGB*)malloc(WIDTH * HEIGHT * sizeof(RGB)); if (data == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } // Generate the flag pattern for (int y = 0; y < HEIGHT; ++y) { for (int x = 0; x < WIDTH; ++x) { // Determine if the current pixel is within the union (blue rectangle) int inUnion = (x < UNION_WIDTH) && (y < UNION_HEIGHT); // Determine if the current pixel is on a red stripe int onRedStripe = (y / STRIPE_HEIGHT) % 2 == 0; // Set the pixel color based on its position if (inUnion) { if (x < (UNION_WIDTH / 13) * 6 && y < (UNION_HEIGHT / 7) * 5) { data[y * WIDTH + x] = WHITE; // White star area } else { data[y * WIDTH + x] = BLUE; // Blue background } } else if (onRedStripe) { data[y * WIDTH + x] = RED; // Red stripe } else { data[y * WIDTH + x] = WHITE; // White stripe } } } // Write the BMP header unsigned char header[54] = { 0x42, 0x4D, 0x36, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x36, 0x00, 0x00, 0x00, 0x28, 0x00, 0x00, 0x00, 0x80, 0x02, 0x00, 0x00, 0xE0, 0x01, 0x00, 0x00, 0x01, 0x00, 0x18, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}; // Update header with image dimensions *(unsigned int*)&header[18] = WIDTH; *(unsigned int*)&header[22] = HEIGHT; // Write the header and pixel data to stdout fwrite(header, sizeof(unsigned char), 54, stdout); fwrite(data, sizeof(RGB), WIDTH * HEIGHT, stdout); // Free the allocated memory free(data); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | #include <stdio.h> #include <stdlib.h> // Define constants for the flag dimensions #define WIDTH 640 #define HEIGHT 480 #define STRIPE_HEIGHT (HEIGHT / 13) #define UNION_WIDTH ((7 * WIDTH) / 13) #define UNION_HEIGHT (7 * STRIPE_HEIGHT) // Define colors as RGB structs typedef struct { unsigned char b, g, r; } RGB; const RGB RED = {0, 0, 255}; const RGB WHITE = {255, 255, 255}; const RGB BLUE = {255, 0, 0}; int main() { // Allocate memory for the pixel data RGB* data = (RGB*)malloc(WIDTH * HEIGHT * sizeof(RGB)); if (data == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } // Generate the flag pattern for (int y = 0; y < HEIGHT; ++y) { for (int x = 0; x < WIDTH; ++x) { // Determine if the current pixel is within the union (blue rectangle) int inUnion = (x < UNION_WIDTH) && (y < UNION_HEIGHT); // Determine if the current pixel is on a red stripe int onRedStripe = (y / STRIPE_HEIGHT) % 2 == 0; // Set the pixel color based on its position if (inUnion) { if (x < (UNION_WIDTH / 13) * 6 && y < (UNION_HEIGHT / 7) * 5) { data[y * WIDTH + x] = WHITE; // White star area } else { data[y * WIDTH + x] = BLUE; // Blue background } } else if (onRedStripe) { data[y * WIDTH + x] = RED; // Red stripe } else { data[y * WIDTH + x] = WHITE; // White stripe } } } // Write the BMP header unsigned char header[54] = { 0x42, 0x4D, 0x36, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x36, 0x00, 0x00, 0x00, 0x28, 0x00, 0x00, 0x00, 0x80, 0x02, 0x00, 0x00, 0xE0, 0x01, 0x00, 0x00, 0x01, 0x00, 0x18, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}; // Update header with image dimensions *(unsigned int*)&header[18] = WIDTH; *(unsigned int*)&header[22] = HEIGHT; // Write the header and pixel data to stdout fwrite(header, sizeof(unsigned char), 54, stdout); fwrite(data, sizeof(RGB), WIDTH * HEIGHT, stdout); // Free the allocated memory free(data); return 0; } |
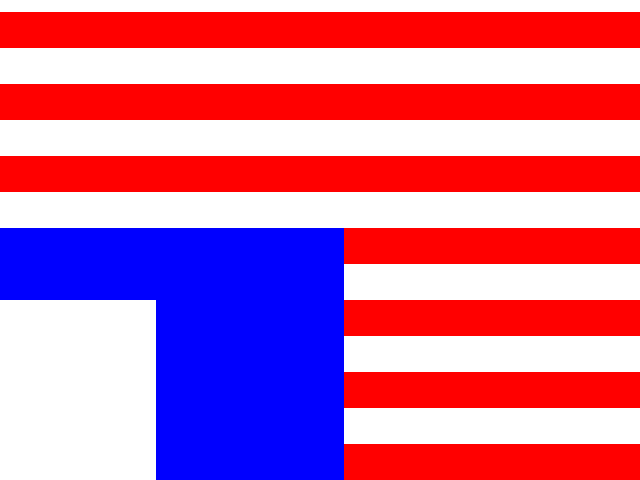
What flag is shown in this image?
The flag shown in the image is the International maritime signal flag for the letter "U" (Uniform). In the International Code of Signals, this flag means "You are running into danger."
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } Pixel; void write_bmp_header(FILE *fp, int width, int height) { int filesize = 54 + 3 * width * height; // Calculate file size // BMP header fprintf(fp, "BM"); // Signature fprintf(fp, "%c%c%c%c", filesize, filesize >> 8, filesize >> 16, filesize >> 24); // File size fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Reserved fprintf(fp, "%c%c%c%c", 54, 0, 0, 0); // Offset to pixel data // DIB header fprintf(fp, "%c%c%c%c", 40, 0, 0, 0); // Header size fprintf(fp, "%c%c%c%c", width, width >> 8, width >> 16, width >> 24); // Image width fprintf(fp, "%c%c%c%c", height, height >> 8, height >> 16, height >> 24); // Image height fprintf(fp, "%c%c", 1, 0); // Number of color planes fprintf(fp, "%c%c", 24, 0); // Bits per pixel fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Compression method fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Image size (can be 0 for uncompressed) fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Horizontal resolution fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Vertical resolution fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Number of colors in palette fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Important color count } void set_pixel(Pixel *data, int x, int y, int width, unsigned char r, unsigned char g, unsigned char b) { if (x >= 0 && x < width && y >= 0 && y < width) { data[y * width + x].r = r; data[y * width + x].g = g; data[y * width + x].b = b; } } int main() { int width = 900; // Flag width int height = 600; // Flag height int stripeHeight = height / 13; // Allocate memory for pixel data Pixel *data = (Pixel *)malloc(width * height * sizeof(Pixel)); if (data == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } // Fill with white background for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { set_pixel(data, x, y, width, 255, 255, 255); } } // Draw red stripes for (int i = 0; i < 7; i += 2) { for (int y = i * stripeHeight; y < (i + 1) * stripeHeight; y++) { for (int x = 0; x < width; x++) { set_pixel(data, x, y, width, 255, 0, 0); } } } // Draw blue canton for (int y = 0; y < 7 * stripeHeight; y++) { for (int x = 0; x < (2 * width) / 5; x++) { set_pixel(data, x, y, width, 0, 0, 170); // Darker blue } } // Write BMP header write_bmp_header(stdout, width, height); // Write pixel data (bottom-up) for (int y = height - 1; y >= 0; y--) { fwrite(data + y * width, sizeof(Pixel), width, stdout); } free(data); return 0; } |
1 2 | gcc flag.c -o flag |
1 2 | ./flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } Pixel; void write_bmp_header(FILE *fp, int width, int height) { int filesize = 54 + 3 * width * height; // Calculate file size // BMP header fprintf(fp, "BM"); // Signature fprintf(fp, "%c%c%c%c", filesize, filesize >> 8, filesize >> 16, filesize >> 24); // File size fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Reserved fprintf(fp, "%c%c%c%c", 54, 0, 0, 0); // Offset to pixel data // DIB header fprintf(fp, "%c%c%c%c", 40, 0, 0, 0); // Header size fprintf(fp, "%c%c%c%c", width, width >> 8, width >> 16, width >> 24); // Image width fprintf(fp, "%c%c%c%c", height, height >> 8, height >> 16, height >> 24); // Image height fprintf(fp, "%c%c", 1, 0); // Number of color planes fprintf(fp, "%c%c", 24, 0); // Bits per pixel fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Compression method fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Image size (can be 0 for uncompressed) fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Horizontal resolution fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Vertical resolution fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Number of colors in palette fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Important color count } void set_pixel(Pixel *data, int x, int y, int width, unsigned char r, unsigned char g, unsigned char b) { if (x >= 0 && x < width && y >= 0 && y < width) { data[y * width + x].r = r; data[y * width + x].g = g; data[y * width + x].b = b; } } int main() { int width = 900; // Flag width int height = 600; // Flag height int stripeHeight = height / 13; // Allocate memory for pixel data Pixel *data = (Pixel *)malloc(width * height * sizeof(Pixel)); if (data == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } // Fill with white background for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { set_pixel(data, x, y, width, 255, 255, 255); } } // Draw red stripes for (int i = 0; i < 7; i += 2) { for (int y = i * stripeHeight; y < (i + 1) * stripeHeight; y++) { for (int x = 0; x < width; x++) { set_pixel(data, x, y, width, 255, 0, 0); } } } // Draw blue canton for (int y = 0; y < 7 * stripeHeight; y++) { for (int x = 0; x < (2 * width) / 5; x++) { set_pixel(data, x, y, width, 0, 0, 170); // Darker blue } } // Write BMP header write_bmp_header(stdout, width, height); // Write pixel data (bottom-up) for (int y = height - 1; y >= 0; y--) { fwrite(data + y * width, sizeof(Pixel), width, stdout); } free(data); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } Pixel; void write_bmp_header(FILE *fp, int width, int height) { int filesize = 54 + 3 * width * height; // Calculate file size // BMP header fprintf(fp, "BM"); // Signature fprintf(fp, "%c%c%c%c", filesize, filesize >> 8, filesize >> 16, filesize >> 24); // File size fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Reserved fprintf(fp, "%c%c%c%c", 54, 0, 0, 0); // Offset to pixel data // DIB header fprintf(fp, "%c%c%c%c", 40, 0, 0, 0); // Header size fprintf(fp, "%c%c%c%c", width, width >> 8, width >> 16, width >> 24); // Image width fprintf(fp, "%c%c%c%c", height, height >> 8, height >> 16, height >> 24); // Image height fprintf(fp, "%c%c", 1, 0); // Number of color planes fprintf(fp, "%c%c", 24, 0); // Bits per pixel fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Compression method fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Image size (can be 0 for uncompressed) fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Horizontal resolution fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Vertical resolution fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Number of colors in palette fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); // Important color count } void set_pixel(Pixel *data, int x, int y, int width, unsigned char r, unsigned char g, unsigned char b) { if (x >= 0 && x < width && y >= 0 && y < width) { data[y * width + x].r = r; data[y * width + x].g = g; data[y * width + x].b = b; } } int main() { int width = 900; // Flag width int height = 600; // Flag height int stripeHeight = height / 13; // Allocate memory for pixel data Pixel *data = (Pixel *)malloc(width * height * sizeof(Pixel)); if (data == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } // Fill with white background for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { set_pixel(data, x, y, width, 255, 255, 255); } } // Draw red stripes for (int i = 0; i < 7; i += 2) { for (int y = i * stripeHeight; y < (i + 1) * stripeHeight; y++) { for (int x = 0; x < width; x++) { set_pixel(data, x, y, width, 255, 0, 0); } } } // Draw blue canton for (int y = 0; y < 7 * stripeHeight; y++) { for (int x = 0; x < (2 * width) / 5; x++) { set_pixel(data, x, y, width, 0, 0, 170); // Darker blue } } // Write BMP header write_bmp_header(stdout, width, height); // Write pixel data (bottom-up) for (int y = height - 1; y >= 0; y--) { fwrite(data + y * width, sizeof(Pixel), width, stdout); } free(data); return 0; } |
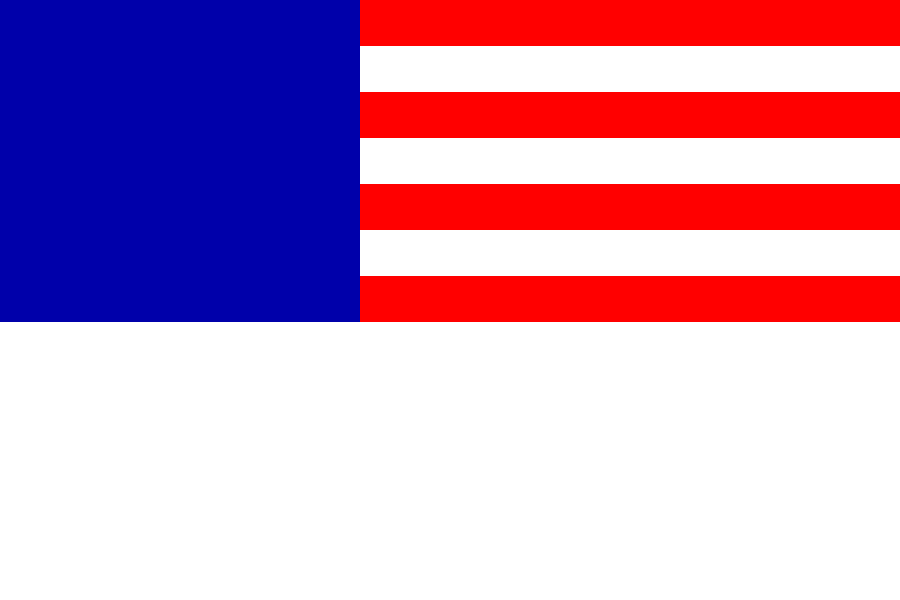
What flag is shown in this image?
The flag shown in the image is the flag of Liberia. The flag features eleven horizontal stripes alternating red and white, with a blue square in the upper left corner. The blue square contains a single white star. The design is similar to the flag of the United States, reflecting Liberia's historical ties to the United States.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { // Image dimensions int width = 960; int height = 540; // Stripe height int stripeHeight = height / 13; // Union dimensions int unionWidth = (int)(width * 2 / 5); int unionHeight = 7 * stripeHeight; // Star dimensions int starDiameter = stripeHeight / 2; int starSpacingX = (int)(starDiameter * 1.5); int starSpacingY = (int)(starDiameter * 1.4); // Calculate padding for BMP file format int padding = (4 - (width * 3) % 4) % 4; // BMP file header printf("BM"); unsigned int fileSize = 54 + (width * 3 + padding) * height; fwrite(&fileSize, sizeof(fileSize), 1, stdout); unsigned int reserved = 0; fwrite(&reserved, sizeof(reserved), 1, stdout); unsigned int dataOffset = 54; fwrite(&dataOffset, sizeof(dataOffset), 1, stdout); // BMP info header unsigned int headerSize = 40; fwrite(&headerSize, sizeof(headerSize), 1, stdout); fwrite(&width, sizeof(width), 1, stdout); fwrite(&height, sizeof(height), 1, stdout); unsigned short planes = 1; fwrite(&planes, sizeof(planes), 1, stdout); unsigned short bitsPerPixel = 24; fwrite(&bitsPerPixel, sizeof(bitsPerPixel), 1, stdout); unsigned int compression = 0; fwrite(&compression, sizeof(compression), 1, stdout); unsigned int imageSize = (width * 3 + padding) * height; fwrite(&imageSize, sizeof(imageSize), 1, stdout); int horizontalResolution = 2835; // Pixels per meter fwrite(&horizontalResolution, sizeof(horizontalResolution), 1, stdout); fwrite(&horizontalResolution, sizeof(horizontalResolution), 1, stdout); unsigned int colorsUsed = 0; fwrite(&colorsUsed, sizeof(colorsUsed), 1, stdout); unsigned int importantColors = 0; fwrite(&importantColors, sizeof(importantColors), 1, stdout); // Define flag colors RGB red = {0, 0, 255}; RGB white = {255, 255, 255}; RGB blue = {255, 0, 0}; // Generate flag image for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { RGB color; // Determine stripe color if (y / stripeHeight % 2 == 0) { color = red; } else { color = white; } // Draw union if (x < unionWidth && y < unionHeight) { // Draw blue background color = blue; // Draw stars int starRow = y / starSpacingY; int starCol = x / starSpacingX; if ((starRow % 2 == 0 && starCol % 2 == 0) || (starRow % 2 != 0 && starCol % 2 != 0)) { int centerX = starCol * starSpacingX + starDiameter / 2; int centerY = starRow * starSpacingY + starDiameter / 2; if ((x - centerX) * (x - centerX) + (y - centerY) * (y - centerY) <= starDiameter * starDiameter / 4) { color = white; } } } // Write pixel to stdout fwrite(&color, sizeof(color), 1, stdout); } // Add padding bytes for (int p = 0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
1 2 | gcc american_flag.c -o american_flag |
1 2 | ./american_flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { // Image dimensions int width = 960; int height = 540; // Stripe height int stripeHeight = height / 13; // Union dimensions int unionWidth = (int)(width * 2 / 5); int unionHeight = 7 * stripeHeight; // Star dimensions int starDiameter = stripeHeight / 2; int starSpacingX = (int)(starDiameter * 1.5); int starSpacingY = (int)(starDiameter * 1.4); // Calculate padding for BMP file format int padding = (4 - (width * 3) % 4) % 4; // BMP file header printf("BM"); unsigned int fileSize = 54 + (width * 3 + padding) * height; fwrite(&fileSize, sizeof(fileSize), 1, stdout); unsigned int reserved = 0; fwrite(&reserved, sizeof(reserved), 1, stdout); unsigned int dataOffset = 54; fwrite(&dataOffset, sizeof(dataOffset), 1, stdout); // BMP info header unsigned int headerSize = 40; fwrite(&headerSize, sizeof(headerSize), 1, stdout); fwrite(&width, sizeof(width), 1, stdout); fwrite(&height, sizeof(height), 1, stdout); unsigned short planes = 1; fwrite(&planes, sizeof(planes), 1, stdout); unsigned short bitsPerPixel = 24; fwrite(&bitsPerPixel, sizeof(bitsPerPixel), 1, stdout); unsigned int compression = 0; fwrite(&compression, sizeof(compression), 1, stdout); unsigned int imageSize = (width * 3 + padding) * height; fwrite(&imageSize, sizeof(imageSize), 1, stdout); int horizontalResolution = 2835; // Pixels per meter fwrite(&horizontalResolution, sizeof(horizontalResolution), 1, stdout); fwrite(&horizontalResolution, sizeof(horizontalResolution), 1, stdout); unsigned int colorsUsed = 0; fwrite(&colorsUsed, sizeof(colorsUsed), 1, stdout); unsigned int importantColors = 0; fwrite(&importantColors, sizeof(importantColors), 1, stdout); // Define flag colors RGB red = {0, 0, 255}; RGB white = {255, 255, 255}; RGB blue = {255, 0, 0}; // Generate flag image for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { RGB color; // Determine stripe color if (y / stripeHeight % 2 == 0) { color = red; } else { color = white; } // Draw union if (x < unionWidth && y < unionHeight) { // Draw blue background color = blue; // Draw stars int starRow = y / starSpacingY; int starCol = x / starSpacingX; if ((starRow % 2 == 0 && starCol % 2 == 0) || (starRow % 2 != 0 && starCol % 2 != 0)) { int centerX = starCol * starSpacingX + starDiameter / 2; int centerY = starRow * starSpacingY + starDiameter / 2; if ((x - centerX) * (x - centerX) + (y - centerY) * (y - centerY) <= starDiameter * starDiameter / 4) { color = white; } } } // Write pixel to stdout fwrite(&color, sizeof(color), 1, stdout); } // Add padding bytes for (int p = 0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { // Image dimensions int width = 960; int height = 540; // Stripe height int stripeHeight = height / 13; // Union dimensions int unionWidth = (int)(width * 2 / 5); int unionHeight = 7 * stripeHeight; // Star dimensions int starDiameter = stripeHeight / 2; int starSpacingX = (int)(starDiameter * 1.5); int starSpacingY = (int)(starDiameter * 1.4); // Calculate padding for BMP file format int padding = (4 - (width * 3) % 4) % 4; // BMP file header printf("BM"); unsigned int fileSize = 54 + (width * 3 + padding) * height; fwrite(&fileSize, sizeof(fileSize), 1, stdout); unsigned int reserved = 0; fwrite(&reserved, sizeof(reserved), 1, stdout); unsigned int dataOffset = 54; fwrite(&dataOffset, sizeof(dataOffset), 1, stdout); // BMP info header unsigned int headerSize = 40; fwrite(&headerSize, sizeof(headerSize), 1, stdout); fwrite(&width, sizeof(width), 1, stdout); fwrite(&height, sizeof(height), 1, stdout); unsigned short planes = 1; fwrite(&planes, sizeof(planes), 1, stdout); unsigned short bitsPerPixel = 24; fwrite(&bitsPerPixel, sizeof(bitsPerPixel), 1, stdout); unsigned int compression = 0; fwrite(&compression, sizeof(compression), 1, stdout); unsigned int imageSize = (width * 3 + padding) * height; fwrite(&imageSize, sizeof(imageSize), 1, stdout); int horizontalResolution = 2835; // Pixels per meter fwrite(&horizontalResolution, sizeof(horizontalResolution), 1, stdout); fwrite(&horizontalResolution, sizeof(horizontalResolution), 1, stdout); unsigned int colorsUsed = 0; fwrite(&colorsUsed, sizeof(colorsUsed), 1, stdout); unsigned int importantColors = 0; fwrite(&importantColors, sizeof(importantColors), 1, stdout); // Define flag colors RGB red = {0, 0, 255}; RGB white = {255, 255, 255}; RGB blue = {255, 0, 0}; // Generate flag image for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { RGB color; // Determine stripe color if (y / stripeHeight % 2 == 0) { color = red; } else { color = white; } // Draw union if (x < unionWidth && y < unionHeight) { // Draw blue background color = blue; // Draw stars int starRow = y / starSpacingY; int starCol = x / starSpacingX; if ((starRow % 2 == 0 && starCol % 2 == 0) || (starRow % 2 != 0 && starCol % 2 != 0)) { int centerX = starCol * starSpacingX + starDiameter / 2; int centerY = starRow * starSpacingY + starDiameter / 2; if ((x - centerX) * (x - centerX) + (y - centerY) * (y - centerY) <= starDiameter * starDiameter / 4) { color = white; } } } // Write pixel to stdout fwrite(&color, sizeof(color), 1, stdout); } // Add padding bytes for (int p = 0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
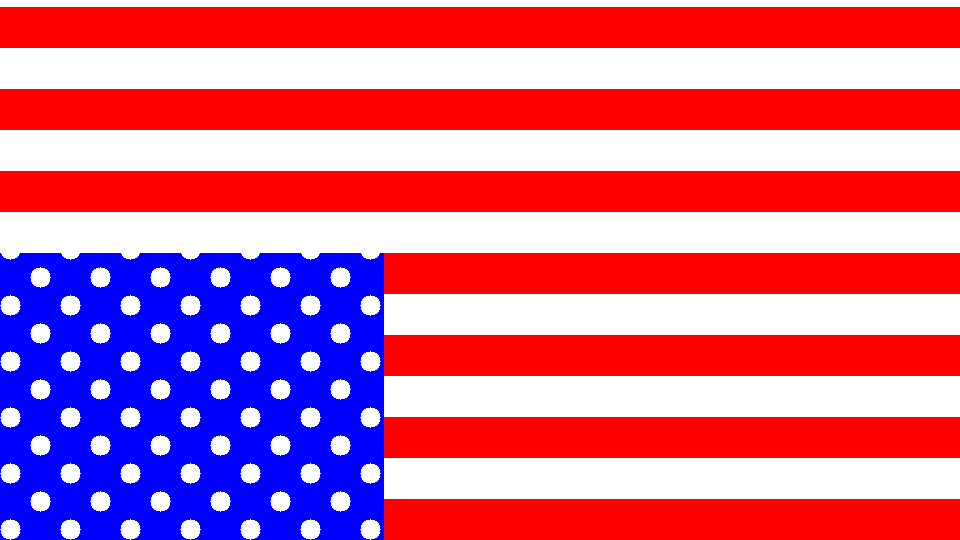
What flag is shown in this image?
The flag shown in the image is a modified version of the United States flag. The traditional flag of the United States features 13 horizontal stripes alternating red and white, and a blue rectangle in the canton (upper left corner) with 50 white stars arranged in nine rows of alternating five and six stars. However, in this modified version, the stars have been replaced with white polka dots.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b; unsigned char g; unsigned char r; } Pixel; int main() { int width = 960; // Image width int height = 540; // Image height // Calculate stripe height int stripeHeight = height / 13; // Calculate union width and height int unionWidth = (7 * width) / 13; int unionHeight = 7 * stripeHeight; // Allocate memory for the pixel data Pixel* image = malloc(width * height * sizeof(Pixel)); if (image == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } // Generate the flag pattern for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { // Determine if the current pixel is within a stripe int isStripe = (y / stripeHeight) % 2 == 0; // Determine if the current pixel is within the union int isInUnion = x < unionWidth && y < unionHeight; // Set the pixel color based on its location if (isInUnion) { // Union is blue image[y * width + x].r = 173; image[y * width + x].g = 216; image[y * width + x].b = 230; } else if (isStripe) { // Red stripe image[y * width + x].r = 178; image[y * width + x].g = 34; image[y * width + x].b = 52; } else { // White stripe image[y * width + x].r = 255; image[y * width + x].g = 255; image[y * width + x].b = 255; } } } // BMP header structure unsigned char header[54] = { 0x42, // Identifier: "BM" 0x4D, // Identifier: "BM" 0x36, 0xFA, 0x00, 0x00, // File size (placeholder) 0x00, 0x00, 0x00, 0x00, // Reserved 0x36, 0x00, 0x00, 0x00, // Offset to pixel data 0x28, 0x00, 0x00, 0x00, // DIB header size (width)&0xFF, (width) >> 8, (width) >> 16, (width) >> 24, // Image width (height)&0xFF, (height) >> 8, (height) >> 16, (height) >> 24, // Image height 0x01, 0x00, // Number of color planes 0x18, 0x00, // Bits per pixel 0x00, 0x00, 0x00, 0x00, // Compression method 0x00, 0xFA, 0x00, 0x00, // Image size (placeholder) 0x00, 0x00, 0x00, 0x00, // Horizontal resolution 0x00, 0x00, 0x00, 0x00, // Vertical resolution 0x00, 0x00, 0x00, 0x00, // Number of colors in palette 0x00, 0x00, 0x00, 0x00 // Number of important colors }; // Calculate file size and image size int fileSize = 54 + width * height * 3; int imageSize = width * height * 3; // Update file size and image size in the header header[2] = (unsigned char)(fileSize); header[3] = (unsigned char)(fileSize >> 8); header[4] = (unsigned char)(fileSize >> 16); header[5] = (unsigned char)(fileSize >> 24); header[34] = (unsigned char)(imageSize); header[35] = (unsigned char)(imageSize >> 8); header[36] = (unsigned char)(imageSize >> 16); header[37] = (unsigned char)(imageSize >> 24); // Write the BMP header to stdout fwrite(header, sizeof(unsigned char), 54, stdout); // Write the pixel data to stdout fwrite(image, sizeof(Pixel), width * height, stdout); // Free the allocated memory free(image); return 0; } |
1 2 | gcc american_flag.c -o american_flag |
1 2 | ./american_flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b; unsigned char g; unsigned char r; } Pixel; int main() { int width = 960; // Image width int height = 540; // Image height // Calculate stripe height int stripeHeight = height / 13; // Calculate union width and height int unionWidth = (7 * width) / 13; int unionHeight = 7 * stripeHeight; // Allocate memory for the pixel data Pixel* image = malloc(width * height * sizeof(Pixel)); if (image == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } // Generate the flag pattern for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { // Determine if the current pixel is within a stripe int isStripe = (y / stripeHeight) % 2 == 0; // Determine if the current pixel is within the union int isInUnion = x < unionWidth && y < unionHeight; // Set the pixel color based on its location if (isInUnion) { // Union is blue image[y * width + x].r = 173; image[y * width + x].g = 216; image[y * width + x].b = 230; } else if (isStripe) { // Red stripe image[y * width + x].r = 178; image[y * width + x].g = 34; image[y * width + x].b = 52; } else { // White stripe image[y * width + x].r = 255; image[y * width + x].g = 255; image[y * width + x].b = 255; } } } // BMP header structure unsigned char header[54] = { 0x42, // Identifier: "BM" 0x4D, // Identifier: "BM" 0x36, 0xFA, 0x00, 0x00, // File size (placeholder) 0x00, 0x00, 0x00, 0x00, // Reserved 0x36, 0x00, 0x00, 0x00, // Offset to pixel data 0x28, 0x00, 0x00, 0x00, // DIB header size (width)&0xFF, (width) >> 8, (width) >> 16, (width) >> 24, // Image width (height)&0xFF, (height) >> 8, (height) >> 16, (height) >> 24, // Image height 0x01, 0x00, // Number of color planes 0x18, 0x00, // Bits per pixel 0x00, 0x00, 0x00, 0x00, // Compression method 0x00, 0xFA, 0x00, 0x00, // Image size (placeholder) 0x00, 0x00, 0x00, 0x00, // Horizontal resolution 0x00, 0x00, 0x00, 0x00, // Vertical resolution 0x00, 0x00, 0x00, 0x00, // Number of colors in palette 0x00, 0x00, 0x00, 0x00 // Number of important colors }; // Calculate file size and image size int fileSize = 54 + width * height * 3; int imageSize = width * height * 3; // Update file size and image size in the header header[2] = (unsigned char)(fileSize); header[3] = (unsigned char)(fileSize >> 8); header[4] = (unsigned char)(fileSize >> 16); header[5] = (unsigned char)(fileSize >> 24); header[34] = (unsigned char)(imageSize); header[35] = (unsigned char)(imageSize >> 8); header[36] = (unsigned char)(imageSize >> 16); header[37] = (unsigned char)(imageSize >> 24); // Write the BMP header to stdout fwrite(header, sizeof(unsigned char), 54, stdout); // Write the pixel data to stdout fwrite(image, sizeof(Pixel), width * height, stdout); // Free the allocated memory free(image); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b; unsigned char g; unsigned char r; } Pixel; int main() { int width = 960; // Image width int height = 540; // Image height // Calculate stripe height int stripeHeight = height / 13; // Calculate union width and height int unionWidth = (7 * width) / 13; int unionHeight = 7 * stripeHeight; // Allocate memory for the pixel data Pixel* image = malloc(width * height * sizeof(Pixel)); if (image == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } // Generate the flag pattern for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { // Determine if the current pixel is within a stripe int isStripe = (y / stripeHeight) % 2 == 0; // Determine if the current pixel is within the union int isInUnion = x < unionWidth && y < unionHeight; // Set the pixel color based on its location if (isInUnion) { // Union is blue image[y * width + x].r = 173; image[y * width + x].g = 216; image[y * width + x].b = 230; } else if (isStripe) { // Red stripe image[y * width + x].r = 178; image[y * width + x].g = 34; image[y * width + x].b = 52; } else { // White stripe image[y * width + x].r = 255; image[y * width + x].g = 255; image[y * width + x].b = 255; } } } // BMP header structure unsigned char header[54] = { 0x42, // Identifier: "BM" 0x4D, // Identifier: "BM" 0x36, 0xFA, 0x00, 0x00, // File size (placeholder) 0x00, 0x00, 0x00, 0x00, // Reserved 0x36, 0x00, 0x00, 0x00, // Offset to pixel data 0x28, 0x00, 0x00, 0x00, // DIB header size (width)&0xFF, (width) >> 8, (width) >> 16, (width) >> 24, // Image width (height)&0xFF, (height) >> 8, (height) >> 16, (height) >> 24, // Image height 0x01, 0x00, // Number of color planes 0x18, 0x00, // Bits per pixel 0x00, 0x00, 0x00, 0x00, // Compression method 0x00, 0xFA, 0x00, 0x00, // Image size (placeholder) 0x00, 0x00, 0x00, 0x00, // Horizontal resolution 0x00, 0x00, 0x00, 0x00, // Vertical resolution 0x00, 0x00, 0x00, 0x00, // Number of colors in palette 0x00, 0x00, 0x00, 0x00 // Number of important colors }; // Calculate file size and image size int fileSize = 54 + width * height * 3; int imageSize = width * height * 3; // Update file size and image size in the header header[2] = (unsigned char)(fileSize); header[3] = (unsigned char)(fileSize >> 8); header[4] = (unsigned char)(fileSize >> 16); header[5] = (unsigned char)(fileSize >> 24); header[34] = (unsigned char)(imageSize); header[35] = (unsigned char)(imageSize >> 8); header[36] = (unsigned char)(imageSize >> 16); header[37] = (unsigned char)(imageSize >> 24); // Write the BMP header to stdout fwrite(header, sizeof(unsigned char), 54, stdout); // Write the pixel data to stdout fwrite(image, sizeof(Pixel), width * height, stdout); // Free the allocated memory free(image); return 0; } |
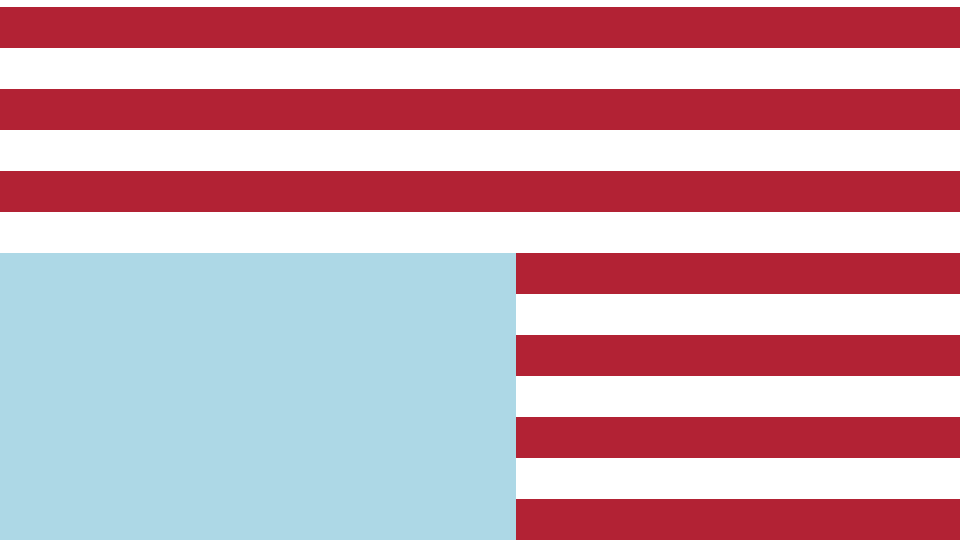
What flag is shown in this image?
The flag shown in the image is the flag of Liberia. The flag features eleven horizontal stripes alternating red and white, with a blue square in the upper left corner containing a single white star. The design is similar to that of the United States flag, reflecting Liberia's historical ties to the United States.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b; unsigned char g; unsigned char r; } Pixel; int main() { // Image dimensions (must be divisible by 13 for correct proportions) const int width = 312; const int height = 169; // Calculate stripe height const int stripeHeight = height / 13; // Allocate memory for the pixel data Pixel *image = (Pixel *)malloc(width * height * sizeof(Pixel)); if (image == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } // Generate the flag pattern for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { // Determine stripe color (white or red) int stripeIndex = y / stripeHeight; Pixel pixelColor = (stripeIndex % 2 == 0) ? (Pixel){255, 255, 255} : (Pixel){178, 34, 52}; // Check if within the blue canton (upper left corner) if (y < 7 * stripeHeight && x < (2 * width) / 5) { pixelColor = (Pixel){60, 59, 110}; // Blue canton color } // Set the pixel color image[y * width + x] = pixelColor; } } // Define BMP header fields unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (will be filled later) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Header size width, 0, 0, 0, // Image width height, 0, 0, 0, // Image height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method 0, 0, 0, 0, // Image size (can be 0 for uncompressed) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; // Calculate file size and update header int fileSize = 54 + width * height * 3; header[2] = (unsigned char)(fileSize); header[3] = (unsigned char)(fileSize >> 8); header[4] = (unsigned char)(fileSize >> 16); header[5] = (unsigned char)(fileSize >> 24); // Write the BMP header to stdout fwrite(header, 1, 54, stdout); // Write the pixel data to stdout fwrite(image, 1, width * height * 3, stdout); // Free allocated memory free(image); return 0; } |
1 2 | gcc american_flag.c -o american_flag |
1 2 | ./american_flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b; unsigned char g; unsigned char r; } Pixel; int main() { // Image dimensions (must be divisible by 13 for correct proportions) const int width = 312; const int height = 169; // Calculate stripe height const int stripeHeight = height / 13; // Allocate memory for the pixel data Pixel *image = (Pixel *)malloc(width * height * sizeof(Pixel)); if (image == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } // Generate the flag pattern for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { // Determine stripe color (white or red) int stripeIndex = y / stripeHeight; Pixel pixelColor = (stripeIndex % 2 == 0) ? (Pixel){255, 255, 255} : (Pixel){178, 34, 52}; // Check if within the blue canton (upper left corner) if (y < 7 * stripeHeight && x < (2 * width) / 5) { pixelColor = (Pixel){60, 59, 110}; // Blue canton color } // Set the pixel color image[y * width + x] = pixelColor; } } // Define BMP header fields unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (will be filled later) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Header size width, 0, 0, 0, // Image width height, 0, 0, 0, // Image height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method 0, 0, 0, 0, // Image size (can be 0 for uncompressed) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; // Calculate file size and update header int fileSize = 54 + width * height * 3; header[2] = (unsigned char)(fileSize); header[3] = (unsigned char)(fileSize >> 8); header[4] = (unsigned char)(fileSize >> 16); header[5] = (unsigned char)(fileSize >> 24); // Write the BMP header to stdout fwrite(header, 1, 54, stdout); // Write the pixel data to stdout fwrite(image, 1, width * height * 3, stdout); // Free allocated memory free(image); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b; unsigned char g; unsigned char r; } Pixel; int main() { // Image dimensions (must be divisible by 13 for correct proportions) const int width = 312; const int height = 169; // Calculate stripe height const int stripeHeight = height / 13; // Allocate memory for the pixel data Pixel *image = (Pixel *)malloc(width * height * sizeof(Pixel)); if (image == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } // Generate the flag pattern for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { // Determine stripe color (white or red) int stripeIndex = y / stripeHeight; Pixel pixelColor = (stripeIndex % 2 == 0) ? (Pixel){255, 255, 255} : (Pixel){178, 34, 52}; // Check if within the blue canton (upper left corner) if (y < 7 * stripeHeight && x < (2 * width) / 5) { pixelColor = (Pixel){60, 59, 110}; // Blue canton color } // Set the pixel color image[y * width + x] = pixelColor; } } // Define BMP header fields unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (will be filled later) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Header size width, 0, 0, 0, // Image width height, 0, 0, 0, // Image height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method 0, 0, 0, 0, // Image size (can be 0 for uncompressed) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; // Calculate file size and update header int fileSize = 54 + width * height * 3; header[2] = (unsigned char)(fileSize); header[3] = (unsigned char)(fileSize >> 8); header[4] = (unsigned char)(fileSize >> 16); header[5] = (unsigned char)(fileSize >> 24); // Write the BMP header to stdout fwrite(header, 1, 54, stdout); // Write the pixel data to stdout fwrite(image, 1, width * height * 3, stdout); // Free allocated memory free(image); return 0; } |
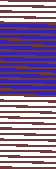
What flag is shown in this image?
The flag shown in the image is the flag of Greece. It consists of nine horizontal stripes alternating between blue and white, with a blue canton containing a white cross in the upper hoist-side corner.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False