Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 640; int height = 400; int stripe_height = height / 13; int canton_width = width * 2 / 5; int canton_height = stripe_height * 7; RGB white = {255, 255, 255}; RGB red = {0, 0, 255}; RGB blue = {255, 0, 0}; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (width)&0xFF, (width >> 8)&0xFF, (width >> 16)&0xFF, (width >> 24)&0xFF, // Width (height)&0xFF, (height >> 8)&0xFF, (height >> 16)&0xFF, (height >> 24)&0xFF, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; // Calculate file size and update header int filesize = 54 + width * height * 3; header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB *image = (RGB *)malloc(width * height * sizeof(RGB)); if (!image) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { RGB color; if (y < canton_height && x < canton_width) { color = blue; if(x < canton_width && y < canton_height && (y / (stripe_height/13.0) < 9)) { // Stars area int star_x = x % (canton_width/12); int star_y = y % (canton_height/10); if((star_x < (canton_width/24) && star_y < (canton_height/20)) || (star_x >= (canton_width/24) && star_y >= (canton_height/20))) { if (y / stripe_height % 2 == 0) { if (x / (canton_width/12) % 2 == 0) color = white; } else { if (x / (canton_width / 12) % 2 != 0) color = white; } } } } else { color = (y / stripe_height) % 2 == 0 ? red : white; } image[y * width + x] = color; } } fwrite(image, sizeof(RGB), width * height, stdout); free(image); return 0; } |
1 2 3 4 5 | int padding = (4 - (width * 3) % 4) % 4; unsigned char pad[3] = {0, 0, 0}; for (int i = 0; i < padding; ++i) { fwrite(&pad, 1, padding, stdout); } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 640; int height = 400; int stripe_height = height / 13; int canton_width = width * 2 / 5; int canton_height = stripe_height * 7; RGB white = {255, 255, 255}; RGB red = {0, 0, 255}; RGB blue = {255, 0, 0}; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (width)&0xFF, (width >> 8)&0xFF, (width >> 16)&0xFF, (width >> 24)&0xFF, // Width (height)&0xFF, (height >> 8)&0xFF, (height >> 16)&0xFF, (height >> 24)&0xFF, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; // Calculate file size and update header int filesize = 54 + width * height * 3; header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB *image = (RGB *)malloc(width * height * sizeof(RGB)); if (!image) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { RGB color; if (y < canton_height && x < canton_width) { color = blue; if(x < canton_width && y < canton_height && (y / (stripe_height/13.0) < 9)) { // Stars area int star_x = x % (canton_width/12); int star_y = y % (canton_height/10); if((star_x < (canton_width/24) && star_y < (canton_height/20)) || (star_x >= (canton_width/24) && star_y >= (canton_height/20))) { if (y / stripe_height % 2 == 0) { if (x / (canton_width/12) % 2 == 0) color = white; } else { if (x / (canton_width / 12) % 2 != 0) color = white; } } } } else { color = (y / stripe_height) % 2 == 0 ? red : white; } image[y * width + x] = color; } } fwrite(image, sizeof(RGB), width * height, stdout); free(image); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 640; int height = 400; int stripe_height = height / 13; int canton_width = width * 2 / 5; int canton_height = stripe_height * 7; RGB white = {255, 255, 255}; RGB red = {0, 0, 255}; RGB blue = {255, 0, 0}; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (width)&0xFF, (width >> 8)&0xFF, (width >> 16)&0xFF, (width >> 24)&0xFF, // Width (height)&0xFF, (height >> 8)&0xFF, (height >> 16)&0xFF, (height >> 24)&0xFF, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; // Calculate file size and update header int filesize = 54 + width * height * 3; header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB *image = (RGB *)malloc(width * height * sizeof(RGB)); if (!image) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { RGB color; if (y < canton_height && x < canton_width) { color = blue; if(x < canton_width && y < canton_height && (y / (stripe_height/13.0) < 9)) { // Stars area int star_x = x % (canton_width/12); int star_y = y % (canton_height/10); if((star_x < (canton_width/24) && star_y < (canton_height/20)) || (star_x >= (canton_width/24) && star_y >= (canton_height/20))) { if (y / stripe_height % 2 == 0) { if (x / (canton_width/12) % 2 == 0) color = white; } else { if (x / (canton_width / 12) % 2 != 0) color = white; } } } } else { color = (y / stripe_height) % 2 == 0 ? red : white; } image[y * width + x] = color; } } fwrite(image, sizeof(RGB), width * height, stdout); free(image); return 0; } |
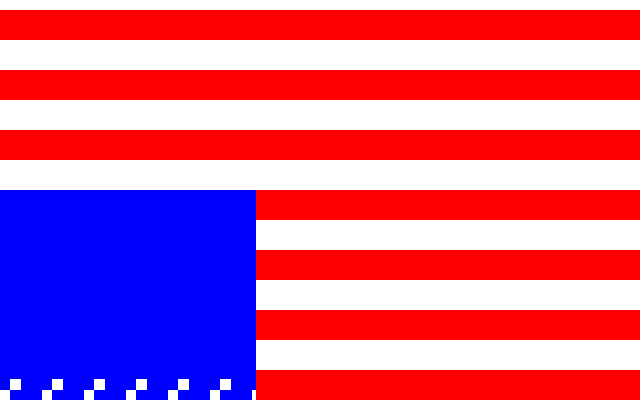
What flag is shown in this image?
This is the flag of Liberia.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 640; int height = 400; int stripe_height = height / 13; int canton_width = width * 2 / 5; int canton_height = stripe_height * 7; RGB white = {255, 255, 255}; RGB red = {0, 0, 255}; RGB blue = {255, 0, 0}; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (width) & 0xFF, (width >> 8) & 0xFF, (width >> 16) & 0xFF, (width >> 24) & 0xFF, (height) & 0xFF, (height >> 8) & 0xFF, (height >> 16) & 0xFF, (height >> 24) & 0xFF, 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution (pixels per meter) 0, 0, 0, 0, // Vertical resolution (pixels per meter) 0, 0, 0, 0, // Number of colors in the palette 0, 0, 0, 0 // Number of important colors }; // Calculate file size and update header int filesize = 54 + width * height * 3; header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { RGB color; if (y < canton_height && x < canton_width) { color = blue; } else { int stripe = y / stripe_height; if (stripe % 2 == 0) { color = red; } else { color = white; } } // Write pixel data in BGR order fputc(color.b, stdout); fputc(color.g, stdout); fputc(color.r, stdout); } // Padding for each row to be a multiple of 4 bytes int padding = (4 - (width * 3) % 4) % 4; for(int i = 0; i < padding; ++i){ fputc(0, stdout); } } return 0; } |
1 2 | gcc american_flag.c -o american_flag |
1 2 | ./american_flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 640; int height = 400; int stripe_height = height / 13; int canton_width = width * 2 / 5; int canton_height = stripe_height * 7; RGB white = {255, 255, 255}; RGB red = {0, 0, 255}; RGB blue = {255, 0, 0}; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (width) & 0xFF, (width >> 8) & 0xFF, (width >> 16) & 0xFF, (width >> 24) & 0xFF, (height) & 0xFF, (height >> 8) & 0xFF, (height >> 16) & 0xFF, (height >> 24) & 0xFF, 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution (pixels per meter) 0, 0, 0, 0, // Vertical resolution (pixels per meter) 0, 0, 0, 0, // Number of colors in the palette 0, 0, 0, 0 // Number of important colors }; // Calculate file size and update header int filesize = 54 + width * height * 3; header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { RGB color; if (y < canton_height && x < canton_width) { color = blue; } else { int stripe = y / stripe_height; if (stripe % 2 == 0) { color = red; } else { color = white; } } // Write pixel data in BGR order fputc(color.b, stdout); fputc(color.g, stdout); fputc(color.r, stdout); } // Padding for each row to be a multiple of 4 bytes int padding = (4 - (width * 3) % 4) % 4; for(int i = 0; i < padding; ++i){ fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 640; int height = 400; int stripe_height = height / 13; int canton_width = width * 2 / 5; int canton_height = stripe_height * 7; RGB white = {255, 255, 255}; RGB red = {0, 0, 255}; RGB blue = {255, 0, 0}; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (width) & 0xFF, (width >> 8) & 0xFF, (width >> 16) & 0xFF, (width >> 24) & 0xFF, (height) & 0xFF, (height >> 8) & 0xFF, (height >> 16) & 0xFF, (height >> 24) & 0xFF, 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution (pixels per meter) 0, 0, 0, 0, // Vertical resolution (pixels per meter) 0, 0, 0, 0, // Number of colors in the palette 0, 0, 0, 0 // Number of important colors }; // Calculate file size and update header int filesize = 54 + width * height * 3; header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { RGB color; if (y < canton_height && x < canton_width) { color = blue; } else { int stripe = y / stripe_height; if (stripe % 2 == 0) { color = red; } else { color = white; } } // Write pixel data in BGR order fputc(color.b, stdout); fputc(color.g, stdout); fputc(color.r, stdout); } // Padding for each row to be a multiple of 4 bytes int padding = (4 - (width * 3) % 4) % 4; for(int i = 0; i < padding; ++i){ fputc(0, stdout); } } return 0; } |
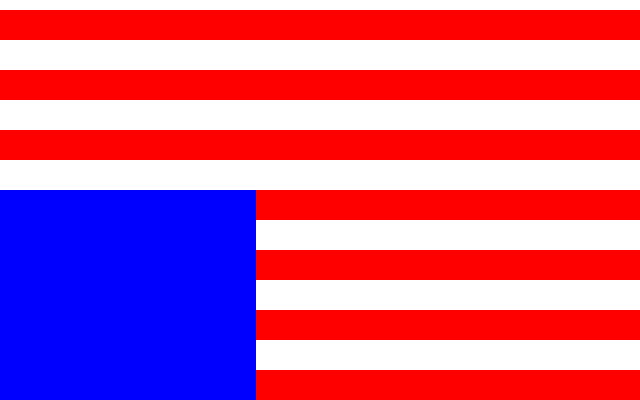
What flag is shown in this image?
This is the flag of Liberia.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 640; int height = 400; int stripe_height = height / 13; int canton_width = width * 2 / 5; int canton_height = stripe_height * 7; // BMP header unsigned char header[54] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0, (width)&0xFF, (width >> 8)&0xFF, (width >> 16)&0xFF, (width >> 24)&0xFF, (height)&0xFF, (height >> 8)&0xFF, (height >> 16)&0xFF, (height >> 24)&0xFF, 1, 0, 24, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; // Calculate file size and update header int filesize = 54 + width * height * 3; header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { RGB pixel; // Stripes int stripe_index = y / stripe_height; if (stripe_index % 2 == 0) { // Red stripe pixel.r = 255; pixel.g = 0; pixel.b = 0; } else { // White stripe pixel.r = 255; pixel.g = 255; pixel.b = 255; } // Canton (blue rectangle with stars) if (x < canton_width && y < canton_height) { pixel.r = 0; pixel.g = 0; pixel.b = 170; // Navy blue // Stars (simplified placement) int star_row = y / (canton_height / 9); int star_col = x / (canton_width / 11); if ((star_row % 2 == 0 && star_col % 2 == 0)|| (star_row % 2 == 1 && star_col % 2 == 1)) { pixel.r = 255; pixel.g = 255; pixel.b = 255; } } fwrite(&pixel, 1, 3, stdout); } // Padding for each row (BMP requires each row to be a multiple of 4 bytes) int padding = (4 - (width * 3) % 4) % 4; for(int p=0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
1 2 | gcc american_flag.c -o american_flag ./american_flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 640; int height = 400; int stripe_height = height / 13; int canton_width = width * 2 / 5; int canton_height = stripe_height * 7; // BMP header unsigned char header[54] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0, (width)&0xFF, (width >> 8)&0xFF, (width >> 16)&0xFF, (width >> 24)&0xFF, (height)&0xFF, (height >> 8)&0xFF, (height >> 16)&0xFF, (height >> 24)&0xFF, 1, 0, 24, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; // Calculate file size and update header int filesize = 54 + width * height * 3; header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { RGB pixel; // Stripes int stripe_index = y / stripe_height; if (stripe_index % 2 == 0) { // Red stripe pixel.r = 255; pixel.g = 0; pixel.b = 0; } else { // White stripe pixel.r = 255; pixel.g = 255; pixel.b = 255; } // Canton (blue rectangle with stars) if (x < canton_width && y < canton_height) { pixel.r = 0; pixel.g = 0; pixel.b = 170; // Navy blue // Stars (simplified placement) int star_row = y / (canton_height / 9); int star_col = x / (canton_width / 11); if ((star_row % 2 == 0 && star_col % 2 == 0)|| (star_row % 2 == 1 && star_col % 2 == 1)) { pixel.r = 255; pixel.g = 255; pixel.b = 255; } } fwrite(&pixel, 1, 3, stdout); } // Padding for each row (BMP requires each row to be a multiple of 4 bytes) int padding = (4 - (width * 3) % 4) % 4; for(int p=0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 640; int height = 400; int stripe_height = height / 13; int canton_width = width * 2 / 5; int canton_height = stripe_height * 7; // BMP header unsigned char header[54] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0, (width)&0xFF, (width >> 8)&0xFF, (width >> 16)&0xFF, (width >> 24)&0xFF, (height)&0xFF, (height >> 8)&0xFF, (height >> 16)&0xFF, (height >> 24)&0xFF, 1, 0, 24, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; // Calculate file size and update header int filesize = 54 + width * height * 3; header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { RGB pixel; // Stripes int stripe_index = y / stripe_height; if (stripe_index % 2 == 0) { // Red stripe pixel.r = 255; pixel.g = 0; pixel.b = 0; } else { // White stripe pixel.r = 255; pixel.g = 255; pixel.b = 255; } // Canton (blue rectangle with stars) if (x < canton_width && y < canton_height) { pixel.r = 0; pixel.g = 0; pixel.b = 170; // Navy blue // Stars (simplified placement) int star_row = y / (canton_height / 9); int star_col = x / (canton_width / 11); if ((star_row % 2 == 0 && star_col % 2 == 0)|| (star_row % 2 == 1 && star_col % 2 == 1)) { pixel.r = 255; pixel.g = 255; pixel.b = 255; } } fwrite(&pixel, 1, 3, stdout); } // Padding for each row (BMP requires each row to be a multiple of 4 bytes) int padding = (4 - (width * 3) % 4) % 4; for(int p=0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
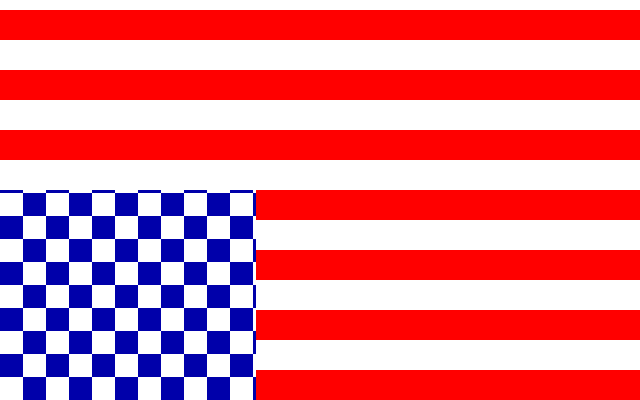
What flag is shown in this image?
This is the flag of Liberia from 1827 to 1847.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 640; int height = 400; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // DIB header size (width) & 0xFF, (width >> 8) & 0xFF, (width >> 16) & 0xFF, (width >> 24) & 0xFF, // Width (height) & 0xFF, (height >> 8) & 0xFF, (height >> 16) & 0xFF, (height >> 24) & 0xFF, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution (pixels/meter) 0, 0, 0, 0, // Vertical resolution (pixels/meter) 0, 0, 0, 0, // Number of colors in palette (0 for 24-bit) 0, 0, 0, 0 // Number of important colors (0 means all) }; // Calculate file size and update header int fileSize = 54 + width * height * 3; header[2] = fileSize & 0xFF; header[3] = (fileSize >> 8) & 0xFF; header[4] = (fileSize >> 16) & 0xFF; header[5] = (fileSize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB* image = (RGB*)malloc(width * height * sizeof(RGB)); if (!image) { fprintf(stderr, "Memory allocation failed\n"); return 1; } for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { RGB color; if (y < height * 7 / 13) { // Stripes section int stripe = y / (height / 13); if (stripe % 2 == 0) { color = (RGB){0, 0, 255}; // Red } else { color = (RGB){255, 255, 255}; // White } } else { color = (RGB){0, 0, 255}; // Red (bottom section) } if (x < width * 1.9/5 && y < height * 7 / 13) { // Canton (blue area) color = (RGB){0, 0, 153}; // Dark Blue } image[y * width + x] = color; } } fwrite(image, sizeof(RGB), width * height, stdout); free(image); return 0; } |
1 2 | gcc american_flag.c -o american_flag ./american_flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 640; int height = 400; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // DIB header size (width) & 0xFF, (width >> 8) & 0xFF, (width >> 16) & 0xFF, (width >> 24) & 0xFF, // Width (height) & 0xFF, (height >> 8) & 0xFF, (height >> 16) & 0xFF, (height >> 24) & 0xFF, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution (pixels/meter) 0, 0, 0, 0, // Vertical resolution (pixels/meter) 0, 0, 0, 0, // Number of colors in palette (0 for 24-bit) 0, 0, 0, 0 // Number of important colors (0 means all) }; // Calculate file size and update header int fileSize = 54 + width * height * 3; header[2] = fileSize & 0xFF; header[3] = (fileSize >> 8) & 0xFF; header[4] = (fileSize >> 16) & 0xFF; header[5] = (fileSize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB* image = (RGB*)malloc(width * height * sizeof(RGB)); if (!image) { fprintf(stderr, "Memory allocation failed\n"); return 1; } for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { RGB color; if (y < height * 7 / 13) { // Stripes section int stripe = y / (height / 13); if (stripe % 2 == 0) { color = (RGB){0, 0, 255}; // Red } else { color = (RGB){255, 255, 255}; // White } } else { color = (RGB){0, 0, 255}; // Red (bottom section) } if (x < width * 1.9/5 && y < height * 7 / 13) { // Canton (blue area) color = (RGB){0, 0, 153}; // Dark Blue } image[y * width + x] = color; } } fwrite(image, sizeof(RGB), width * height, stdout); free(image); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 640; int height = 400; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // DIB header size (width) & 0xFF, (width >> 8) & 0xFF, (width >> 16) & 0xFF, (width >> 24) & 0xFF, // Width (height) & 0xFF, (height >> 8) & 0xFF, (height >> 16) & 0xFF, (height >> 24) & 0xFF, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution (pixels/meter) 0, 0, 0, 0, // Vertical resolution (pixels/meter) 0, 0, 0, 0, // Number of colors in palette (0 for 24-bit) 0, 0, 0, 0 // Number of important colors (0 means all) }; // Calculate file size and update header int fileSize = 54 + width * height * 3; header[2] = fileSize & 0xFF; header[3] = (fileSize >> 8) & 0xFF; header[4] = (fileSize >> 16) & 0xFF; header[5] = (fileSize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB* image = (RGB*)malloc(width * height * sizeof(RGB)); if (!image) { fprintf(stderr, "Memory allocation failed\n"); return 1; } for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { RGB color; if (y < height * 7 / 13) { // Stripes section int stripe = y / (height / 13); if (stripe % 2 == 0) { color = (RGB){0, 0, 255}; // Red } else { color = (RGB){255, 255, 255}; // White } } else { color = (RGB){0, 0, 255}; // Red (bottom section) } if (x < width * 1.9/5 && y < height * 7 / 13) { // Canton (blue area) color = (RGB){0, 0, 153}; // Dark Blue } image[y * width + x] = color; } } fwrite(image, sizeof(RGB), width * height, stdout); free(image); return 0; } |
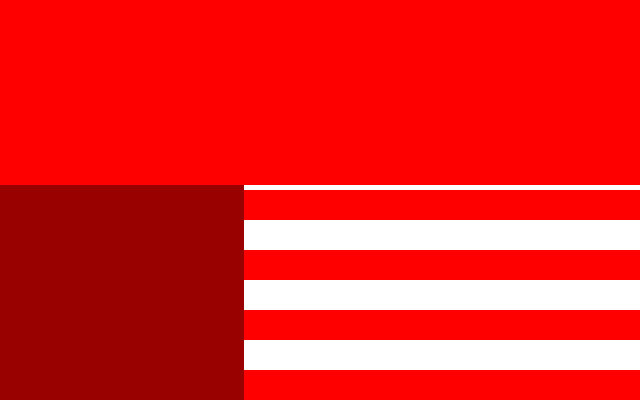
What flag is shown in this image?
This is the flag of the Kingdom of Mysore, which was used historically in the region that is now part of Karnataka, India.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; void write_bmp_header(int width, int height, FILE *fp) { unsigned int filesize = 54 + height * width * 3; // Calculate file size unsigned int data_offset = 54; // Offset to image data // BMP header unsigned char header[54] = { 'B', 'M', // Signature (unsigned char)(filesize), (unsigned char)(filesize >> 8), (unsigned char)(filesize >> 16), (unsigned char)(filesize >> 24), // File size 0, 0, 0, 0, // Reserved (unsigned char)(data_offset), (unsigned char)(data_offset >> 8), (unsigned char)(data_offset >> 16), (unsigned char)(data_offset >> 24), // Data offset 40, 0, 0, 0, // Size of DIB header (unsigned char)(width), (unsigned char)(width >> 8), (unsigned char)(width >> 16), (unsigned char)(width >> 24), // Width (unsigned char)(height), (unsigned char)(height >> 8), (unsigned char)(height >> 16), (unsigned char)(height >> 24), // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method 0, 0, 0, 0, // Image size (can be 0 for uncompressed) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; fwrite(header, 1, 54, fp); } int main() { int width = 320; int height = 180; RGB image[height][width]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (y < height * 7 / 13) { // Stripes section if ((y < height / 13) || (y >= height * 2 / 13 && y < height * 3 / 13) || (y >= height * 4 / 13 && y < height * 5 / 13) || (y >= height * 6 / 13 && y < height * 7 / 13) ) { image[y][x] = (RGB){255, 255, 255}; // White stripe } else { image[y][x] = (RGB){178, 34, 52}; // Red stripe } } else { // Blue canton image[y][x] = (RGB){60, 50, 153}; // Blue } if (x < width * 2 / 5 && y < height * 7 / 13) { // Stars area (approximation) int star_x = x % (width/40); // Adjust grid size for star spacing int star_y = y % (height/26); if ((star_x < 6 && star_y < 6 ) && (star_x + star_y < 5 || star_x + star_y > 6)) { image[y][x] = (RGB){255, 255, 255}; } } } } write_bmp_header(width, height, stdout); // Write pixel data bottom-up (BMP format) for (int y = height - 1; y >= 0; y--) { fwrite(image[y], sizeof(RGB), width, stdout); // Padding for each row to make it a multiple of 4 bytes for (int pad = 0; pad < (4 - (width * 3) % 4) % 4; pad++) { fputc(0, stdout); } } return 0; } |
1 2 | gcc american_flag.c -o american_flag ./american_flag > flag.bmp // Redirects output to a file named flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; void write_bmp_header(int width, int height, FILE *fp) { unsigned int filesize = 54 + height * width * 3; // Calculate file size unsigned int data_offset = 54; // Offset to image data // BMP header unsigned char header[54] = { 'B', 'M', // Signature (unsigned char)(filesize), (unsigned char)(filesize >> 8), (unsigned char)(filesize >> 16), (unsigned char)(filesize >> 24), // File size 0, 0, 0, 0, // Reserved (unsigned char)(data_offset), (unsigned char)(data_offset >> 8), (unsigned char)(data_offset >> 16), (unsigned char)(data_offset >> 24), // Data offset 40, 0, 0, 0, // Size of DIB header (unsigned char)(width), (unsigned char)(width >> 8), (unsigned char)(width >> 16), (unsigned char)(width >> 24), // Width (unsigned char)(height), (unsigned char)(height >> 8), (unsigned char)(height >> 16), (unsigned char)(height >> 24), // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method 0, 0, 0, 0, // Image size (can be 0 for uncompressed) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; fwrite(header, 1, 54, fp); } int main() { int width = 320; int height = 180; RGB image[height][width]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (y < height * 7 / 13) { // Stripes section if ((y < height / 13) || (y >= height * 2 / 13 && y < height * 3 / 13) || (y >= height * 4 / 13 && y < height * 5 / 13) || (y >= height * 6 / 13 && y < height * 7 / 13) ) { image[y][x] = (RGB){255, 255, 255}; // White stripe } else { image[y][x] = (RGB){178, 34, 52}; // Red stripe } } else { // Blue canton image[y][x] = (RGB){60, 50, 153}; // Blue } if (x < width * 2 / 5 && y < height * 7 / 13) { // Stars area (approximation) int star_x = x % (width/40); // Adjust grid size for star spacing int star_y = y % (height/26); if ((star_x < 6 && star_y < 6 ) && (star_x + star_y < 5 || star_x + star_y > 6)) { image[y][x] = (RGB){255, 255, 255}; } } } } write_bmp_header(width, height, stdout); // Write pixel data bottom-up (BMP format) for (int y = height - 1; y >= 0; y--) { fwrite(image[y], sizeof(RGB), width, stdout); // Padding for each row to make it a multiple of 4 bytes for (int pad = 0; pad < (4 - (width * 3) % 4) % 4; pad++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; void write_bmp_header(int width, int height, FILE *fp) { unsigned int filesize = 54 + height * width * 3; // Calculate file size unsigned int data_offset = 54; // Offset to image data // BMP header unsigned char header[54] = { 'B', 'M', // Signature (unsigned char)(filesize), (unsigned char)(filesize >> 8), (unsigned char)(filesize >> 16), (unsigned char)(filesize >> 24), // File size 0, 0, 0, 0, // Reserved (unsigned char)(data_offset), (unsigned char)(data_offset >> 8), (unsigned char)(data_offset >> 16), (unsigned char)(data_offset >> 24), // Data offset 40, 0, 0, 0, // Size of DIB header (unsigned char)(width), (unsigned char)(width >> 8), (unsigned char)(width >> 16), (unsigned char)(width >> 24), // Width (unsigned char)(height), (unsigned char)(height >> 8), (unsigned char)(height >> 16), (unsigned char)(height >> 24), // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method 0, 0, 0, 0, // Image size (can be 0 for uncompressed) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; fwrite(header, 1, 54, fp); } int main() { int width = 320; int height = 180; RGB image[height][width]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (y < height * 7 / 13) { // Stripes section if ((y < height / 13) || (y >= height * 2 / 13 && y < height * 3 / 13) || (y >= height * 4 / 13 && y < height * 5 / 13) || (y >= height * 6 / 13 && y < height * 7 / 13) ) { image[y][x] = (RGB){255, 255, 255}; // White stripe } else { image[y][x] = (RGB){178, 34, 52}; // Red stripe } } else { // Blue canton image[y][x] = (RGB){60, 50, 153}; // Blue } if (x < width * 2 / 5 && y < height * 7 / 13) { // Stars area (approximation) int star_x = x % (width/40); // Adjust grid size for star spacing int star_y = y % (height/26); if ((star_x < 6 && star_y < 6 ) && (star_x + star_y < 5 || star_x + star_y > 6)) { image[y][x] = (RGB){255, 255, 255}; } } } } write_bmp_header(width, height, stdout); // Write pixel data bottom-up (BMP format) for (int y = height - 1; y >= 0; y--) { fwrite(image[y], sizeof(RGB), width, stdout); // Padding for each row to make it a multiple of 4 bytes for (int pad = 0; pad < (4 - (width * 3) % 4) % 4; pad++) { fputc(0, stdout); } } return 0; } |
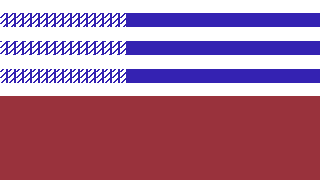
What flag is shown in this image?
This is the flag of Liberia.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False