Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates an American flag in BMP format and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 | #include <stdio.h> #include <stdlib.h> #include <math.h> typedef struct { unsigned char b, g, r; } Pixel; int main() { const int H = 130; // Flag height const int W = (int)(H * 1.9); // Flag width const int stripe_height = H / 13; const int canton_height = 7 * stripe_height; const int canton_width = (int)(0.76 * H); // Initialize star matrix unsigned char **stars = (unsigned char **)malloc(canton_height * sizeof(unsigned char *)); for (int y = 0; y < canton_height; y++) { stars[y] = (unsigned char *)calloc(canton_width, sizeof(unsigned char)); } // Create star pattern for (int row = 0; row < 9; row++) { int num_stars = (row % 2 == 0) ? 6 : 5; double y_pos = (row + 0.5) * canton_height / 9.0; for (int i = 0; i < num_stars; i++) { double x_pos; if (num_stars == 6) { x_pos = (i + 0.5) * canton_width / 6.0; } else { x_pos = (i + 0.5) * canton_width / 5.0; } int sx = (int)round(x_pos); int sy = (int)round(y_pos); // Mark 3x3 area around star center for (int dy = -1; dy <= 1; dy++) { for (int dx = -1; dx <= 1; dx++) { int x = sx + dx; int y = sy + dy; if (x >= 0 && x < canton_width && y >= 0 && y < canton_height) { stars[y][x] = 1; } } } } } // BMP headers const int padding = (4 - (W * 3) % 4) % 4; const int file_size = 54 + (W * 3 + padding) * H; unsigned char file_header[14] = { 'B', 'M', // Signature (unsigned char)(file_size), (unsigned char)(file_size >> 8), (unsigned char)(file_size >> 16), (unsigned char)(file_size >> 24), 0, 0, 0, 0, // Reserved 54, 0, 0, 0 // Data offset }; unsigned char info_header[40] = { 40, 0, 0, 0, // Info header size (unsigned char)(W), (unsigned char)(W >> 8), (unsigned char)(W >> 16), (unsigned char)(W >> 24), (unsigned char)(H), (unsigned char)(H >> 8), (unsigned char)(H >> 16), (unsigned char)(H >> 24), 1, 0, // Planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression 0, 0, 0, 0, // Image size 0, 0, 0, 0, // Pixels/meter 0, 0, 0, 0, // Colors used 0, 0, 0, 0 // Important colors }; fwrite(file_header, 1, 14, stdout); fwrite(info_header, 1, 40, stdout); // Generate pixel data for (int image_y = H - 1; image_y >= 0; image_y--) { for (int x = 0; x < W; x++) { Pixel p; if (x < canton_width && image_y < canton_height) { // Canton area if (stars[image_y][x]) { // White star p.r = p.g = p.b = 255; } else { // Old Glory Blue p.r = 10; p.g = 49; p.b = 97; } } else { // Stripes area int stripe = image_y / stripe_height; if (stripe % 2 == 0) { // Old Glory Red p.r = 179; p.g = 25; p.b = 66; } else { // White p.r = p.g = p.b = 255; } } fwrite(&p, 3, 1, stdout); } // Add row padding for (int p = 0; p < padding; p++) { fputc(0, stdout); } } // Cleanup for (int y = 0; y < canton_height; y++) { free(stars[y]); } free(stars); return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 | #include <stdio.h> #include <stdlib.h> #include <math.h> typedef struct { unsigned char b, g, r; } Pixel; int main() { const int H = 130; // Flag height const int W = (int)(H * 1.9); // Flag width const int stripe_height = H / 13; const int canton_height = 7 * stripe_height; const int canton_width = (int)(0.76 * H); // Initialize star matrix unsigned char **stars = (unsigned char **)malloc(canton_height * sizeof(unsigned char *)); for (int y = 0; y < canton_height; y++) { stars[y] = (unsigned char *)calloc(canton_width, sizeof(unsigned char)); } // Create star pattern for (int row = 0; row < 9; row++) { int num_stars = (row % 2 == 0) ? 6 : 5; double y_pos = (row + 0.5) * canton_height / 9.0; for (int i = 0; i < num_stars; i++) { double x_pos; if (num_stars == 6) { x_pos = (i + 0.5) * canton_width / 6.0; } else { x_pos = (i + 0.5) * canton_width / 5.0; } int sx = (int)round(x_pos); int sy = (int)round(y_pos); // Mark 3x3 area around star center for (int dy = -1; dy <= 1; dy++) { for (int dx = -1; dx <= 1; dx++) { int x = sx + dx; int y = sy + dy; if (x >= 0 && x < canton_width && y >= 0 && y < canton_height) { stars[y][x] = 1; } } } } } // BMP headers const int padding = (4 - (W * 3) % 4) % 4; const int file_size = 54 + (W * 3 + padding) * H; unsigned char file_header[14] = { 'B', 'M', // Signature (unsigned char)(file_size), (unsigned char)(file_size >> 8), (unsigned char)(file_size >> 16), (unsigned char)(file_size >> 24), 0, 0, 0, 0, // Reserved 54, 0, 0, 0 // Data offset }; unsigned char info_header[40] = { 40, 0, 0, 0, // Info header size (unsigned char)(W), (unsigned char)(W >> 8), (unsigned char)(W >> 16), (unsigned char)(W >> 24), (unsigned char)(H), (unsigned char)(H >> 8), (unsigned char)(H >> 16), (unsigned char)(H >> 24), 1, 0, // Planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression 0, 0, 0, 0, // Image size 0, 0, 0, 0, // Pixels/meter 0, 0, 0, 0, // Colors used 0, 0, 0, 0 // Important colors }; fwrite(file_header, 1, 14, stdout); fwrite(info_header, 1, 40, stdout); // Generate pixel data for (int image_y = H - 1; image_y >= 0; image_y--) { for (int x = 0; x < W; x++) { Pixel p; if (x < canton_width && image_y < canton_height) { // Canton area if (stars[image_y][x]) { // White star p.r = p.g = p.b = 255; } else { // Old Glory Blue p.r = 10; p.g = 49; p.b = 97; } } else { // Stripes area int stripe = image_y / stripe_height; if (stripe % 2 == 0) { // Old Glory Red p.r = 179; p.g = 25; p.b = 66; } else { // White p.r = p.g = p.b = 255; } } fwrite(&p, 3, 1, stdout); } // Add row padding for (int p = 0; p < padding; p++) { fputc(0, stdout); } } // Cleanup for (int y = 0; y < canton_height; y++) { free(stars[y]); } free(stars); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 | #include <stdio.h> #include <stdlib.h> #include <math.h> typedef struct { unsigned char b, g, r; } Pixel; int main() { const int H = 130; // Flag height const int W = (int)(H * 1.9); // Flag width const int stripe_height = H / 13; const int canton_height = 7 * stripe_height; const int canton_width = (int)(0.76 * H); // Initialize star matrix unsigned char **stars = (unsigned char **)malloc(canton_height * sizeof(unsigned char *)); for (int y = 0; y < canton_height; y++) { stars[y] = (unsigned char *)calloc(canton_width, sizeof(unsigned char)); } // Create star pattern for (int row = 0; row < 9; row++) { int num_stars = (row % 2 == 0) ? 6 : 5; double y_pos = (row + 0.5) * canton_height / 9.0; for (int i = 0; i < num_stars; i++) { double x_pos; if (num_stars == 6) { x_pos = (i + 0.5) * canton_width / 6.0; } else { x_pos = (i + 0.5) * canton_width / 5.0; } int sx = (int)round(x_pos); int sy = (int)round(y_pos); // Mark 3x3 area around star center for (int dy = -1; dy <= 1; dy++) { for (int dx = -1; dx <= 1; dx++) { int x = sx + dx; int y = sy + dy; if (x >= 0 && x < canton_width && y >= 0 && y < canton_height) { stars[y][x] = 1; } } } } } // BMP headers const int padding = (4 - (W * 3) % 4) % 4; const int file_size = 54 + (W * 3 + padding) * H; unsigned char file_header[14] = { 'B', 'M', // Signature (unsigned char)(file_size), (unsigned char)(file_size >> 8), (unsigned char)(file_size >> 16), (unsigned char)(file_size >> 24), 0, 0, 0, 0, // Reserved 54, 0, 0, 0 // Data offset }; unsigned char info_header[40] = { 40, 0, 0, 0, // Info header size (unsigned char)(W), (unsigned char)(W >> 8), (unsigned char)(W >> 16), (unsigned char)(W >> 24), (unsigned char)(H), (unsigned char)(H >> 8), (unsigned char)(H >> 16), (unsigned char)(H >> 24), 1, 0, // Planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression 0, 0, 0, 0, // Image size 0, 0, 0, 0, // Pixels/meter 0, 0, 0, 0, // Colors used 0, 0, 0, 0 // Important colors }; fwrite(file_header, 1, 14, stdout); fwrite(info_header, 1, 40, stdout); // Generate pixel data for (int image_y = H - 1; image_y >= 0; image_y--) { for (int x = 0; x < W; x++) { Pixel p; if (x < canton_width && image_y < canton_height) { // Canton area if (stars[image_y][x]) { // White star p.r = p.g = p.b = 255; } else { // Old Glory Blue p.r = 10; p.g = 49; p.b = 97; } } else { // Stripes area int stripe = image_y / stripe_height; if (stripe % 2 == 0) { // Old Glory Red p.r = 179; p.g = 25; p.b = 66; } else { // White p.r = p.g = p.b = 255; } } fwrite(&p, 3, 1, stdout); } // Add row padding for (int p = 0; p < padding; p++) { fputc(0, stdout); } } // Cleanup for (int y = 0; y < canton_height; y++) { free(stars[y]); } free(stars); return 0; } |
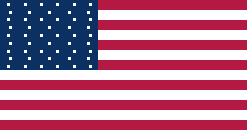
What flag is shown in this image?
This is the flag of the United States.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True