Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Creating a C program that generates a BMP image of the American flag and writes it to stdout involves several steps. Below is a simplified version of such a program. The program generates a 24-bit BMP image with the correct dimensions and colors for the American flag.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 | #include <stdio.h> #include <stdint.h> #include <string.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) #define WIDTH 950 #define HEIGHT 500 #define STRIPE_HEIGHT (HEIGHT / 13) #define STAR_FIELD_WIDTH (WIDTH * 2 / 5) #define STAR_FIELD_HEIGHT (7 * STRIPE_HEIGHT) void write_bmp_header(FILE *f, int width, int height) { BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; uint32_t rowSize = (width * 3 + 3) & (~3); uint32_t imageSize = rowSize * height; fileHeader.bfType = 0x4D42; fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + imageSize; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; infoHeader.biCompression = 0; infoHeader.biSizeImage = imageSize; infoHeader.biXPelsPerMeter = 2835; infoHeader.biYPelsPerMeter = 2835; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; fwrite(&fileHeader, sizeof(fileHeader), 1, f); fwrite(&infoHeader, sizeof(infoHeader), 1, f); } void write_pixel(FILE *f, uint8_t r, uint8_t g, uint8_t b) { fwrite(&b, 1, 1, f); fwrite(&g, 1, 1, f); fwrite(&r, 1, 1, f); } void draw_stripe(FILE *f, int y, int is_red) { for (int x = 0; x < WIDTH; x++) { if (is_red) { write_pixel(f, 178, 34, 52); // Red stripe } else { write_pixel(f, 255, 255, 255); // White stripe } } } void draw_star_field(FILE *f) { for (int y = 0; y < STAR_FIELD_HEIGHT; y++) { for (int x = 0; x < STAR_FIELD_WIDTH; x++) { if ((x / 32 + y / 27) % 2 == 0) { write_pixel(f, 0, 0, 0); // Blue field } else { write_pixel(f, 255, 255, 255); // White star } } } } int main() { FILE *f = stdout; write_bmp_header(f, WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { int stripe_index = y / STRIPE_HEIGHT; if (stripe_index < 7) { if (y < STAR_FIELD_HEIGHT) { draw_star_field(f); } else { draw_stripe(f, y, stripe_index % 2 == 0); } } else { draw_stripe(f, y, stripe_index % 2 == 0); } } return 0; } |
1 2 | gcc -o flag flag.c ./flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 | #include <stdio.h> #include <stdint.h> #include <string.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) #define WIDTH 950 #define HEIGHT 500 #define STRIPE_HEIGHT (HEIGHT / 13) #define STAR_FIELD_WIDTH (WIDTH * 2 / 5) #define STAR_FIELD_HEIGHT (7 * STRIPE_HEIGHT) void write_bmp_header(FILE *f, int width, int height) { BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; uint32_t rowSize = (width * 3 + 3) & (~3); uint32_t imageSize = rowSize * height; fileHeader.bfType = 0x4D42; fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + imageSize; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; infoHeader.biCompression = 0; infoHeader.biSizeImage = imageSize; infoHeader.biXPelsPerMeter = 2835; infoHeader.biYPelsPerMeter = 2835; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; fwrite(&fileHeader, sizeof(fileHeader), 1, f); fwrite(&infoHeader, sizeof(infoHeader), 1, f); } void write_pixel(FILE *f, uint8_t r, uint8_t g, uint8_t b) { fwrite(&b, 1, 1, f); fwrite(&g, 1, 1, f); fwrite(&r, 1, 1, f); } void draw_stripe(FILE *f, int y, int is_red) { for (int x = 0; x < WIDTH; x++) { if (is_red) { write_pixel(f, 178, 34, 52); // Red stripe } else { write_pixel(f, 255, 255, 255); // White stripe } } } void draw_star_field(FILE *f) { for (int y = 0; y < STAR_FIELD_HEIGHT; y++) { for (int x = 0; x < STAR_FIELD_WIDTH; x++) { if ((x / 32 + y / 27) % 2 == 0) { write_pixel(f, 0, 0, 0); // Blue field } else { write_pixel(f, 255, 255, 255); // White star } } } } int main() { FILE *f = stdout; write_bmp_header(f, WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { int stripe_index = y / STRIPE_HEIGHT; if (stripe_index < 7) { if (y < STAR_FIELD_HEIGHT) { draw_star_field(f); } else { draw_stripe(f, y, stripe_index % 2 == 0); } } else { draw_stripe(f, y, stripe_index % 2 == 0); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 | #include <stdio.h> #include <stdint.h> #include <string.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) #define WIDTH 950 #define HEIGHT 500 #define STRIPE_HEIGHT (HEIGHT / 13) #define STAR_FIELD_WIDTH (WIDTH * 2 / 5) #define STAR_FIELD_HEIGHT (7 * STRIPE_HEIGHT) void write_bmp_header(FILE *f, int width, int height) { BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; uint32_t rowSize = (width * 3 + 3) & (~3); uint32_t imageSize = rowSize * height; fileHeader.bfType = 0x4D42; fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + imageSize; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; infoHeader.biCompression = 0; infoHeader.biSizeImage = imageSize; infoHeader.biXPelsPerMeter = 2835; infoHeader.biYPelsPerMeter = 2835; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; fwrite(&fileHeader, sizeof(fileHeader), 1, f); fwrite(&infoHeader, sizeof(infoHeader), 1, f); } void write_pixel(FILE *f, uint8_t r, uint8_t g, uint8_t b) { fwrite(&b, 1, 1, f); fwrite(&g, 1, 1, f); fwrite(&r, 1, 1, f); } void draw_stripe(FILE *f, int y, int is_red) { for (int x = 0; x < WIDTH; x++) { if (is_red) { write_pixel(f, 178, 34, 52); // Red stripe } else { write_pixel(f, 255, 255, 255); // White stripe } } } void draw_star_field(FILE *f) { for (int y = 0; y < STAR_FIELD_HEIGHT; y++) { for (int x = 0; x < STAR_FIELD_WIDTH; x++) { if ((x / 32 + y / 27) % 2 == 0) { write_pixel(f, 0, 0, 0); // Blue field } else { write_pixel(f, 255, 255, 255); // White star } } } } int main() { FILE *f = stdout; write_bmp_header(f, WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { int stripe_index = y / STRIPE_HEIGHT; if (stripe_index < 7) { if (y < STAR_FIELD_HEIGHT) { draw_star_field(f); } else { draw_stripe(f, y, stripe_index % 2 == 0); } } else { draw_stripe(f, y, stripe_index % 2 == 0); } } return 0; } |
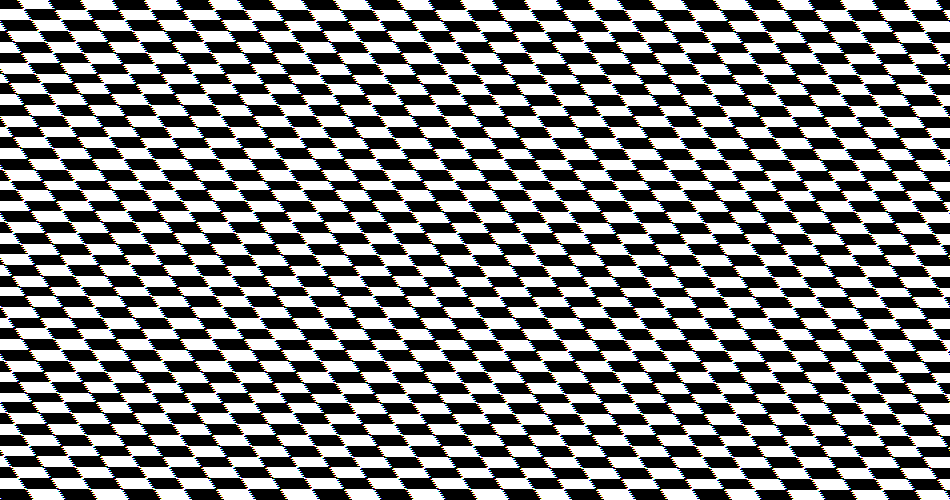
What flag is shown in this image?
This image shows a black and white checkered pattern, often associated with a racing flag used to signal the end of a race.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False