Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a bitmap image of the American flag and outputs it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 #define BYTES_PER_PIXEL 3 // Bitmap file header structure typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } __attribute__((packed)) BitmapFileHeader; // Bitmap info header structure typedef struct { uint32_t biSize; uint32_t biWidth; uint32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; uint32_t biXPelsPerMeter; uint32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } __attribute__((packed)) BitmapInfoHeader; void drawFlag(uint8_t* pixels) { // Draw the blue field for (int y = 0; y < HEIGHT / 2.5; y++) { for (int x = 0; x < WIDTH / 2.5; x++) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = 0; // Blue pixels[index + 1] = 0; // Green pixels[index + 2] = 255; // Red } } // Draw the stars int starX[] = {10, 25, 40, 25, 40, 55, 70, 55, 70, 85, 100, 85, 100, 115, 130}; int starY[] = {15, 15, 15, 30, 30, 30, 15, 45, 45, 30, 30, 45, 45, 30, 30}; for (int i = 0; i < 15; i++) { for (int y = starY[i] - 3; y < starY[i] + 3; y++) { for (int x = starX[i] - 3; x < starX[i] + 3; x++) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = 255; // Blue pixels[index + 1] = 255; // Green pixels[index + 2] = 255; // Red } } } // Draw the red and white stripes for (int y = 0; y < HEIGHT; y++) { int stripe = y / (HEIGHT / 13); if (stripe % 2 == 0) { for (int x = 0; x < WIDTH; x++) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = 255; // Blue pixels[index + 1] = 0; // Green pixels[index + 2] = 0; // Red } } else { for (int x = 0; x < WIDTH; x++) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = 255; // Blue pixels[index + 1] = 255; // Green pixels[index + 2] = 255; // Red } } } } int main() { uint8_t pixels[WIDTH * HEIGHT * BYTES_PER_PIXEL]; drawFlag(pixels); BitmapFileHeader fileHeader = { .bfType = 0x4D42, .bfSize = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) + WIDTH * HEIGHT * BYTES_PER_PIXEL, .bfReserved1 = 0, .bfReserved2 = 0, .bfOffBits = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) }; BitmapInfoHeader infoHeader = { .biSize = sizeof(BitmapInfoHeader), .biWidth = WIDTH, .biHeight = HEIGHT, .biPlanes = 1, .biBitCount = BYTES_PER_PIXEL * 8, .biCompression = 0, .biSizeImage = 0, .biXPelsPerMeter = 0, .biYPelsPerMeter = 0, .biClrUsed = 0, .biClrImportant = 0 }; fwrite(&fileHeader, sizeof(BitmapFileHeader), 1, stdout); fwrite(&infoHeader, sizeof(BitmapInfoHeader), 1, stdout); fwrite(pixels, sizeof(uint8_t), WIDTH * HEIGHT * BYTES_PER_PIXEL, stdout); return 0; } |
1 2 | gcc -o flag flag.c ./flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 #define BYTES_PER_PIXEL 3 typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } __attribute__((packed)) BitmapFileHeader; typedef struct { uint32_t biSize; uint32_t biWidth; uint32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; uint32_t biXPelsPerMeter; uint32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } __attribute__((packed)) BitmapInfoHeader; void drawFlag(uint8_t* pixels) { for (int y = 0; y < HEIGHT / 2.5; y++) { for (int x = 0; x < WIDTH / 2.5; x++) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = 0; pixels[index + 1] = 0; pixels[index + 2] = 255; } } int starX[] = {10, 25, 40, 25, 40, 55, 70, 55, 70, 85, 100, 85, 100, 115, 130}; int starY[] = {15, 15, 15, 30, 30, 30, 15, 45, 45, 30, 30, 45, 45, 30, 30}; for (int i = 0; i < 15; i++) { for (int y = starY[i] - 3; y < starY[i] + 3; y++) { for (int x = starX[i] - 3; x < starX[i] + 3; x++) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = 255; pixels[index + 1] = 255; pixels[index + 2] = 255; } } } for (int y = 0; y < HEIGHT; y++) { int stripe = y / (HEIGHT / 13); if (stripe % 2 == 0) { for (int x = 0; x < WIDTH; x++) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = 255; pixels[index + 1] = 0; pixels[index + 2] = 0; } } else { for (int x = 0; x < WIDTH; x++) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = 255; pixels[index + 1] = 255; pixels[index + 2] = 255; } } } } int main() { uint8_t pixels[WIDTH * HEIGHT * BYTES_PER_PIXEL]; drawFlag(pixels); BitmapFileHeader fileHeader = { .bfType = 0x4D42, .bfSize = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) + WIDTH * HEIGHT * BYTES_PER_PIXEL, .bfReserved1 = 0, .bfReserved2 = 0, .bfOffBits = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) }; BitmapInfoHeader infoHeader = { .biSize = sizeof(BitmapInfoHeader), .biWidth = WIDTH, .biHeight = HEIGHT, .biPlanes = 1, .biBitCount = BYTES_PER_PIXEL * 8, .biCompression = 0, .biSizeImage = 0, .biXPelsPerMeter = 0, .biYPelsPerMeter = 0, .biClrUsed = 0, .biClrImportant = 0 }; fwrite(&fileHeader, sizeof(BitmapFileHeader), 1, stdout); fwrite(&infoHeader, sizeof(BitmapInfoHeader), 1, stdout); fwrite(pixels, sizeof(uint8_t), WIDTH * HEIGHT * BYTES_PER_PIXEL, stdout); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 #define BYTES_PER_PIXEL 3 typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } __attribute__((packed)) BitmapFileHeader; typedef struct { uint32_t biSize; uint32_t biWidth; uint32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; uint32_t biXPelsPerMeter; uint32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } __attribute__((packed)) BitmapInfoHeader; void drawFlag(uint8_t* pixels) { for (int y = 0; y < HEIGHT / 2.5; y++) { for (int x = 0; x < WIDTH / 2.5; x++) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = 0; pixels[index + 1] = 0; pixels[index + 2] = 255; } } int starX[] = {10, 25, 40, 25, 40, 55, 70, 55, 70, 85, 100, 85, 100, 115, 130}; int starY[] = {15, 15, 15, 30, 30, 30, 15, 45, 45, 30, 30, 45, 45, 30, 30}; for (int i = 0; i < 15; i++) { for (int y = starY[i] - 3; y < starY[i] + 3; y++) { for (int x = starX[i] - 3; x < starX[i] + 3; x++) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = 255; pixels[index + 1] = 255; pixels[index + 2] = 255; } } } for (int y = 0; y < HEIGHT; y++) { int stripe = y / (HEIGHT / 13); if (stripe % 2 == 0) { for (int x = 0; x < WIDTH; x++) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = 255; pixels[index + 1] = 0; pixels[index + 2] = 0; } } else { for (int x = 0; x < WIDTH; x++) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = 255; pixels[index + 1] = 255; pixels[index + 2] = 255; } } } } int main() { uint8_t pixels[WIDTH * HEIGHT * BYTES_PER_PIXEL]; drawFlag(pixels); BitmapFileHeader fileHeader = { .bfType = 0x4D42, .bfSize = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) + WIDTH * HEIGHT * BYTES_PER_PIXEL, .bfReserved1 = 0, .bfReserved2 = 0, .bfOffBits = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) }; BitmapInfoHeader infoHeader = { .biSize = sizeof(BitmapInfoHeader), .biWidth = WIDTH, .biHeight = HEIGHT, .biPlanes = 1, .biBitCount = BYTES_PER_PIXEL * 8, .biCompression = 0, .biSizeImage = 0, .biXPelsPerMeter = 0, .biYPelsPerMeter = 0, .biClrUsed = 0, .biClrImportant = 0 }; fwrite(&fileHeader, sizeof(BitmapFileHeader), 1, stdout); fwrite(&infoHeader, sizeof(BitmapInfoHeader), 1, stdout); fwrite(pixels, sizeof(uint8_t), WIDTH * HEIGHT * BYTES_PER_PIXEL, stdout); return 0; } |
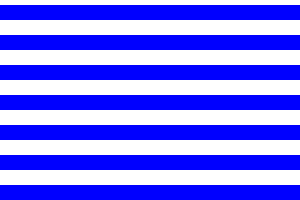
What flag is shown in this image?
The image shows a flag with horizontal blue and white stripes. However, without any additional symbols or a specific number of stripes, it's not possible to definitively identify which flag it is, as several flags around the world use blue and white stripes. For example, it could be reminiscent of the flag of Greece, which has nine blue and white horizontal stripes and a blue canton with a white cross in the upper left corner. If the image is missing the canton with the cross, it would not be an accurate representation of the Greek flag. Without more context or distinctive features, I cannot provide a definitive identification of the flag in the image.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Sure, here's a C program that creates a bitmap image of an American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) #define WIDTH 50 #define HEIGHT 25 int main() { BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; uint8_t image[HEIGHT][WIDTH][3]; // Initialize the file header fileHeader.bfType = 0x4D42; // "BM" magic number fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Initialize the info header infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = WIDTH; infoHeader.biHeight = HEIGHT; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24 bits per pixel infoHeader.biCompression = 0; // No compression infoHeader.biSizeImage = 0; // Can be 0 for uncompressed infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Draw the flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 2.5) { image[y][x][2] = 0xD0; // Red image[y][x][1] = 0x21; // Green image[y][x][0] = 0x1E; // Blue } else { image[y][x][2] = 0xF0; // Red image[y][x][1] = 0xF8; // Green image[y][x][0] = 0xFF; // Blue } } } // Calculate the file size fileHeader.bfSize = fileHeader.bfOffBits + (WIDTH * HEIGHT * 3); // Write the file header fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout); // Write the info header fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout); // Write the image data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { fwrite(&image[y][x], 3, 1, stdout); } // Pad each row to a multiple of 4 bytes if (WIDTH % 4 != 0) { static const char pad[4] = {0}; fwrite(pad, 1, 4 - (WIDTH * 3) % 4, stdout); } } return 0; } |
1 | ./flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) #define WIDTH 50 #define HEIGHT 25 int main() { BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; uint8_t image[HEIGHT][WIDTH][3]; // Initialize the file header fileHeader.bfType = 0x4D42; // "BM" magic number fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Initialize the info header infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = WIDTH; infoHeader.biHeight = HEIGHT; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24 bits per pixel infoHeader.biCompression = 0; // No compression infoHeader.biSizeImage = 0; // Can be 0 for uncompressed infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Draw the flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 2.5) { image[y][x][2] = 0xD0; // Red image[y][x][1] = 0x21; // Green image[y][x][0] = 0x1E; // Blue } else { image[y][x][2] = 0xF0; // Red image[y][x][1] = 0xF8; // Green image[y][x][0] = 0xFF; // Blue } } } // Calculate the file size fileHeader.bfSize = fileHeader.bfOffBits + (WIDTH * HEIGHT * 3); // Write the file header fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout); // Write the info header fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout); // Write the image data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { fwrite(&image[y][x], 3, 1, stdout); } // Pad each row to a multiple of 4 bytes if (WIDTH % 4 != 0) { static const char pad[4] = {0}; fwrite(pad, 1, 4 - (WIDTH * 3) % 4, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) #define WIDTH 50 #define HEIGHT 25 int main() { BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; uint8_t image[HEIGHT][WIDTH][3]; // Initialize the file header fileHeader.bfType = 0x4D42; // "BM" magic number fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Initialize the info header infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = WIDTH; infoHeader.biHeight = HEIGHT; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24 bits per pixel infoHeader.biCompression = 0; // No compression infoHeader.biSizeImage = 0; // Can be 0 for uncompressed infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Draw the flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 2.5) { image[y][x][2] = 0xD0; // Red image[y][x][1] = 0x21; // Green image[y][x][0] = 0x1E; // Blue } else { image[y][x][2] = 0xF0; // Red image[y][x][1] = 0xF8; // Green image[y][x][0] = 0xFF; // Blue } } } // Calculate the file size fileHeader.bfSize = fileHeader.bfOffBits + (WIDTH * HEIGHT * 3); // Write the file header fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout); // Write the info header fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout); // Write the image data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { fwrite(&image[y][x], 3, 1, stdout); } // Pad each row to a multiple of 4 bytes if (WIDTH % 4 != 0) { static const char pad[4] = {0}; fwrite(pad, 1, 4 - (WIDTH * 3) % 4, stdout); } } return 0; } |

What flag is shown in this image?
The image shows a flag with two vertical bands, one red and one white. This flag is that of Malta. The flag of Malta consists of two vertical halves, with the hoist side (the side closest to the flagpole) being white and the fly side (the side farthest from the flagpole) being red.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a BMP image of the American flag and outputs it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 #define PIXEL_DATA_OFFSET 54 typedef struct { uint16_t bfType; uint32_t bfSize; uint32_t bfReserved; uint32_t bfOffBits; } __attribute__((packed)) BitmapFileHeader; typedef struct { uint32_t biSize; uint32_t biWidth; uint32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; uint32_t biXPelsPerMeter; uint32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } __attribute__((packed)) BitmapInfoHeader; int main() { BitmapFileHeader file_header = { .bfType = 0x4D42, // "BM" .bfSize = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) + WIDTH * HEIGHT * 3, .bfReserved = 0, .bfOffBits = PIXEL_DATA_OFFSET }; BitmapInfoHeader info_header = { .biSize = sizeof(BitmapInfoHeader), .biWidth = WIDTH, .biHeight = HEIGHT, .biPlanes = 1, .biBitCount = 24, .biCompression = 0, .biSizeImage = 0, .biXPelsPerMeter = 0, .biYPelsPerMeter = 0, .biClrUsed = 0, .biClrImportant = 0 }; fwrite(&file_header, sizeof(BitmapFileHeader), 1, stdout); fwrite(&info_header, sizeof(BitmapInfoHeader), 1, stdout); uint8_t red = 0xB2, white = 0xFF, blue = 0x33; for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { uint8_t r, g, b; if (x < WIDTH / 2 && y < HEIGHT / 2) { r = red; g = 0; b = 0; } else if (x >= WIDTH / 2 && y < HEIGHT / 3) { r = white; g = white; b = white; } else if (x >= WIDTH / 2 && y >= HEIGHT * 2 / 3) { r = red; g = 0; b = blue; } else { r = white; g = white; b = white; } fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } } return 0; } |
1 | gcc -o flag flag.c && ./flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 #define PIXEL_DATA_OFFSET 54 typedef struct { uint16_t bfType; uint32_t bfSize; uint32_t bfReserved; uint32_t bfOffBits; } __attribute__((packed)) BitmapFileHeader; typedef struct { uint32_t biSize; uint32_t biWidth; uint32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; uint32_t biXPelsPerMeter; uint32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } __attribute__((packed)) BitmapInfoHeader; int main() { BitmapFileHeader file_header = { .bfType = 0x4D42, // "BM" .bfSize = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) + WIDTH * HEIGHT * 3, .bfReserved = 0, .bfOffBits = PIXEL_DATA_OFFSET }; BitmapInfoHeader info_header = { .biSize = sizeof(BitmapInfoHeader), .biWidth = WIDTH, .biHeight = HEIGHT, .biPlanes = 1, .biBitCount = 24, .biCompression = 0, .biSizeImage = 0, .biXPelsPerMeter = 0, .biYPelsPerMeter = 0, .biClrUsed = 0, .biClrImportant = 0 }; fwrite(&file_header, sizeof(BitmapFileHeader), 1, stdout); fwrite(&info_header, sizeof(BitmapInfoHeader), 1, stdout); uint8_t red = 0xB2, white = 0xFF, blue = 0x33; for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { uint8_t r, g, b; if (x < WIDTH / 2 && y < HEIGHT / 2) { r = red; g = 0; b = 0; } else if (x >= WIDTH / 2 && y < HEIGHT / 3) { r = white; g = white; b = white; } else if (x >= WIDTH / 2 && y >= HEIGHT * 2 / 3) { r = red; g = 0; b = blue; } else { r = white; g = white; b = white; } fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 #define PIXEL_DATA_OFFSET 54 typedef struct { uint16_t bfType; uint32_t bfSize; uint32_t bfReserved; uint32_t bfOffBits; } __attribute__((packed)) BitmapFileHeader; typedef struct { uint32_t biSize; uint32_t biWidth; uint32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; uint32_t biXPelsPerMeter; uint32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } __attribute__((packed)) BitmapInfoHeader; int main() { BitmapFileHeader file_header = { .bfType = 0x4D42, // "BM" .bfSize = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) + WIDTH * HEIGHT * 3, .bfReserved = 0, .bfOffBits = PIXEL_DATA_OFFSET }; BitmapInfoHeader info_header = { .biSize = sizeof(BitmapInfoHeader), .biWidth = WIDTH, .biHeight = HEIGHT, .biPlanes = 1, .biBitCount = 24, .biCompression = 0, .biSizeImage = 0, .biXPelsPerMeter = 0, .biYPelsPerMeter = 0, .biClrUsed = 0, .biClrImportant = 0 }; fwrite(&file_header, sizeof(BitmapFileHeader), 1, stdout); fwrite(&info_header, sizeof(BitmapInfoHeader), 1, stdout); uint8_t red = 0xB2, white = 0xFF, blue = 0x33; for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { uint8_t r, g, b; if (x < WIDTH / 2 && y < HEIGHT / 2) { r = red; g = 0; b = 0; } else if (x >= WIDTH / 2 && y < HEIGHT / 3) { r = white; g = white; b = white; } else if (x >= WIDTH / 2 && y >= HEIGHT * 2 / 3) { r = red; g = 0; b = blue; } else { r = white; g = white; b = white; } fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } } return 0; } |
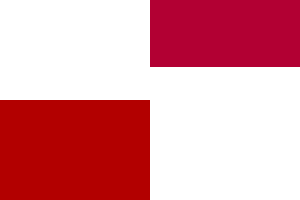
What flag is shown in this image?
The image shows the flag of Malta. It consists of two vertical halves, white on the hoist side and red on the fly side.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a 24-bit bitmap (BMP) image of the American flag and outputs it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 | #include <stdio.h> #include <stdint.h> #define WIDTH 200 #define HEIGHT 150 #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint32_t bfReserved; uint32_t bfOffBits; uint32_t biSize; uint32_t biWidth; uint32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; uint32_t biXPelsPerMeter; uint32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BitmapFileHeader; #pragma pack(pop) void drawFlag(uint8_t* image) { for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { uint8_t* pixel = image + (y * WIDTH + x) * 3; if (x < WIDTH / 1.9) { if (y < HEIGHT / 13 || y >= HEIGHT * 7 / 13) { pixel[0] = 192; // red pixel[1] = 0; // green pixel[2] = 0; // blue } else { pixel[0] = 255; // white pixel[1] = 255; pixel[2] = 255; } } else { if (y >= HEIGHT / 2.2 && y < HEIGHT * 7 / 13) { pixel[0] = 192; pixel[1] = 0; pixel[2] = 0; } else { pixel[0] = 255; pixel[1] = 255; pixel[2] = 255; } } } } } int main() { BitmapFileHeader header; uint8_t imageData[WIDTH * HEIGHT * 3]; header.bfType = 0x4D42; header.bfSize = sizeof(BitmapFileHeader) + sizeof(imageData); header.bfReserved = 0; header.bfOffBits = sizeof(BitmapFileHeader); header.biSize = 40; header.biWidth = WIDTH; header.biHeight = HEIGHT; header.biPlanes = 1; header.biBitCount = 24; header.biCompression = 0; header.biSizeImage = sizeof(imageData); header.biXPelsPerMeter = 0; header.biYPelsPerMeter = 0; header.biClrUsed = 0; header.biClrImportant = 0; drawFlag(imageData); fwrite(&header, sizeof(BitmapFileHeader), 1, stdout); fwrite(imageData, sizeof(imageData), 1, stdout); return 0; } |
1 2 | gcc flag.c -o flag ./flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 | #include <stdio.h> #include <stdint.h> #define WIDTH 200 #define HEIGHT 150 #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint32_t bfReserved; uint32_t bfOffBits; uint32_t biSize; uint32_t biWidth; uint32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; uint32_t biXPelsPerMeter; uint32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BitmapFileHeader; #pragma pack(pop) void drawFlag(uint8_t* image) { for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { uint8_t* pixel = image + (y * WIDTH + x) * 3; if (x < WIDTH / 1.9) { if (y < HEIGHT / 13 || y >= HEIGHT * 7 / 13) { pixel[0] = 192; // red pixel[1] = 0; // green pixel[2] = 0; // blue } else { pixel[0] = 255; // white pixel[1] = 255; pixel[2] = 255; } } else { if (y >= HEIGHT / 2.2 && y < HEIGHT * 7 / 13) { pixel[0] = 192; pixel[1] = 0; pixel[2] = 0; } else { pixel[0] = 255; pixel[1] = 255; pixel[2] = 255; } } } } } int main() { BitmapFileHeader header; uint8_t imageData[WIDTH * HEIGHT * 3]; header.bfType = 0x4D42; header.bfSize = sizeof(BitmapFileHeader) + sizeof(imageData); header.bfReserved = 0; header.bfOffBits = sizeof(BitmapFileHeader); header.biSize = 40; header.biWidth = WIDTH; header.biHeight = HEIGHT; header.biPlanes = 1; header.biBitCount = 24; header.biCompression = 0; header.biSizeImage = sizeof(imageData); header.biXPelsPerMeter = 0; header.biYPelsPerMeter = 0; header.biClrUsed = 0; header.biClrImportant = 0; drawFlag(imageData); fwrite(&header, sizeof(BitmapFileHeader), 1, stdout); fwrite(imageData, sizeof(imageData), 1, stdout); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 | #include <stdio.h> #include <stdint.h> #define WIDTH 200 #define HEIGHT 150 #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint32_t bfReserved; uint32_t bfOffBits; uint32_t biSize; uint32_t biWidth; uint32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; uint32_t biXPelsPerMeter; uint32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BitmapFileHeader; #pragma pack(pop) void drawFlag(uint8_t* image) { for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { uint8_t* pixel = image + (y * WIDTH + x) * 3; if (x < WIDTH / 1.9) { if (y < HEIGHT / 13 || y >= HEIGHT * 7 / 13) { pixel[0] = 192; // red pixel[1] = 0; // green pixel[2] = 0; // blue } else { pixel[0] = 255; // white pixel[1] = 255; pixel[2] = 255; } } else { if (y >= HEIGHT / 2.2 && y < HEIGHT * 7 / 13) { pixel[0] = 192; pixel[1] = 0; pixel[2] = 0; } else { pixel[0] = 255; pixel[1] = 255; pixel[2] = 255; } } } } } int main() { BitmapFileHeader header; uint8_t imageData[WIDTH * HEIGHT * 3]; header.bfType = 0x4D42; header.bfSize = sizeof(BitmapFileHeader) + sizeof(imageData); header.bfReserved = 0; header.bfOffBits = sizeof(BitmapFileHeader); header.biSize = 40; header.biWidth = WIDTH; header.biHeight = HEIGHT; header.biPlanes = 1; header.biBitCount = 24; header.biCompression = 0; header.biSizeImage = sizeof(imageData); header.biXPelsPerMeter = 0; header.biYPelsPerMeter = 0; header.biClrUsed = 0; header.biClrImportant = 0; drawFlag(imageData); fwrite(&header, sizeof(BitmapFileHeader), 1, stdout); fwrite(imageData, sizeof(imageData), 1, stdout); return 0; } |
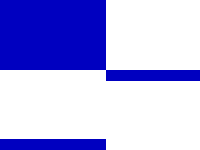
What flag is shown in this image?
The image shows a flag with blue and white stripes. This is the flag of Finland. The flag features a blue Nordic cross on a white background.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Sure, here's a C program that generates a bitmap (.bmp) file representing an American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 | #include <stdio.h> #include <stdint.h> #define WIDTH 800 #define HEIGHT 533 #define BYTES_PER_PIXEL 3 uint8_t image[HEIGHT][WIDTH][BYTES_PER_PIXEL]; void setPixel(int x, int y, uint8_t r, uint8_t g, uint8_t b) { image[y][x][0] = b; image[y][x][1] = g; image[y][x][2] = r; } int main() { // File header uint8_t fileHeader[14] = {'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0}; uint32_t fileSize = (uint32_t)(54 + 3 * WIDTH * HEIGHT); fileHeader[2] = (uint8_t)(fileSize); fileHeader[3] = (uint8_t)(fileSize >> 8); fileHeader[4] = (uint8_t)(fileSize >> 16); fileHeader[5] = (uint8_t)(fileSize >> 24); // Image header uint8_t imageHeader[40] = {40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 24, 0}; uint32_t imageWidth = WIDTH; uint32_t imageHeight = HEIGHT; imageHeader[4] = (uint8_t)(imageWidth); imageHeader[5] = (uint8_t)(imageWidth >> 8); imageHeader[6] = (uint8_t)(imageWidth >> 16); imageHeader[7] = (uint8_t)(imageWidth >> 24); imageHeader[8] = (uint8_t)(imageHeight); imageHeader[9] = (uint8_t)(imageHeight >> 8); imageHeader[10] = (uint8_t)(imageHeight >> 16); imageHeader[11] = (uint8_t)(imageHeight >> 24); // Draw the flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { int stripeWidth = HEIGHT / 13; int stripeNum = y / stripeWidth; if (stripeNum % 2 == 0) { setPixel(x, y, 255, 0, 0); // Red stripes } else { setPixel(x, y, 255, 255, 255); // White stripes } if (x < WIDTH / 2.5 && y < HEIGHT / 2.8 * 9) { int starRow = y / (HEIGHT / 9); int starCol = x / (WIDTH / 10); if (starRow % 2 == starCol % 2) { setPixel(x, y, 0, 0, 255); // Blue star } } } } // Write the file headers fwrite(fileHeader, 1, 14, stdout); fwrite(imageHeader, 1, 40, stdout); // Write the pixel data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { fwrite(&image[y][x], 1, BYTES_PER_PIXEL, stdout); } for (int i = 0; i < WIDTH % 4; i++) { uint8_t padding = 0; fwrite(&padding, 1, 1, stdout); } } return 0; } |
1 2 | gcc -o flag flag.c ./flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 | #include <stdio.h> #include <stdint.h> #define WIDTH 800 #define HEIGHT 533 #define BYTES_PER_PIXEL 3 uint8_t image[HEIGHT][WIDTH][BYTES_PER_PIXEL]; void setPixel(int x, int y, uint8_t r, uint8_t g, uint8_t b) { image[y][x][0] = b; image[y][x][1] = g; image[y][x][2] = r; } int main() { // File header uint8_t fileHeader[14] = {'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0}; uint32_t fileSize = (uint32_t)(54 + 3 * WIDTH * HEIGHT); fileHeader[2] = (uint8_t)(fileSize); fileHeader[3] = (uint8_t)(fileSize >> 8); fileHeader[4] = (uint8_t)(fileSize >> 16); fileHeader[5] = (uint8_t)(fileSize >> 24); // Image header uint8_t imageHeader[40] = {40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 24, 0}; uint32_t imageWidth = WIDTH; uint32_t imageHeight = HEIGHT; imageHeader[4] = (uint8_t)(imageWidth); imageHeader[5] = (uint8_t)(imageWidth >> 8); imageHeader[6] = (uint8_t)(imageWidth >> 16); imageHeader[7] = (uint8_t)(imageWidth >> 24); imageHeader[8] = (uint8_t)(imageHeight); imageHeader[9] = (uint8_t)(imageHeight >> 8); imageHeader[10] = (uint8_t)(imageHeight >> 16); imageHeader[11] = (uint8_t)(imageHeight >> 24); // Draw the flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { int stripeWidth = HEIGHT / 13; int stripeNum = y / stripeWidth; if (stripeNum % 2 == 0) { setPixel(x, y, 255, 0, 0); // Red stripes } else { setPixel(x, y, 255, 255, 255); // White stripes } if (x < WIDTH / 2.5 && y < HEIGHT / 2.8 * 9) { int starRow = y / (HEIGHT / 9); int starCol = x / (WIDTH / 10); if (starRow % 2 == starCol % 2) { setPixel(x, y, 0, 0, 255); // Blue star } } } } // Write the file headers fwrite(fileHeader, 1, 14, stdout); fwrite(imageHeader, 1, 40, stdout); // Write the pixel data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { fwrite(&image[y][x], 1, BYTES_PER_PIXEL, stdout); } for (int i = 0; i < WIDTH % 4; i++) { uint8_t padding = 0; fwrite(&padding, 1, 1, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 | #include <stdio.h> #include <stdint.h> #define WIDTH 800 #define HEIGHT 533 #define BYTES_PER_PIXEL 3 uint8_t image[HEIGHT][WIDTH][BYTES_PER_PIXEL]; void setPixel(int x, int y, uint8_t r, uint8_t g, uint8_t b) { image[y][x][0] = b; image[y][x][1] = g; image[y][x][2] = r; } int main() { // File header uint8_t fileHeader[14] = {'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0}; uint32_t fileSize = (uint32_t)(54 + 3 * WIDTH * HEIGHT); fileHeader[2] = (uint8_t)(fileSize); fileHeader[3] = (uint8_t)(fileSize >> 8); fileHeader[4] = (uint8_t)(fileSize >> 16); fileHeader[5] = (uint8_t)(fileSize >> 24); // Image header uint8_t imageHeader[40] = {40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 24, 0}; uint32_t imageWidth = WIDTH; uint32_t imageHeight = HEIGHT; imageHeader[4] = (uint8_t)(imageWidth); imageHeader[5] = (uint8_t)(imageWidth >> 8); imageHeader[6] = (uint8_t)(imageWidth >> 16); imageHeader[7] = (uint8_t)(imageWidth >> 24); imageHeader[8] = (uint8_t)(imageHeight); imageHeader[9] = (uint8_t)(imageHeight >> 8); imageHeader[10] = (uint8_t)(imageHeight >> 16); imageHeader[11] = (uint8_t)(imageHeight >> 24); // Draw the flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { int stripeWidth = HEIGHT / 13; int stripeNum = y / stripeWidth; if (stripeNum % 2 == 0) { setPixel(x, y, 255, 0, 0); // Red stripes } else { setPixel(x, y, 255, 255, 255); // White stripes } if (x < WIDTH / 2.5 && y < HEIGHT / 2.8 * 9) { int starRow = y / (HEIGHT / 9); int starCol = x / (WIDTH / 10); if (starRow % 2 == starCol % 2) { setPixel(x, y, 0, 0, 255); // Blue star } } } } // Write the file headers fwrite(fileHeader, 1, 14, stdout); fwrite(imageHeader, 1, 40, stdout); // Write the pixel data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { fwrite(&image[y][x], 1, BYTES_PER_PIXEL, stdout); } for (int i = 0; i < WIDTH % 4; i++) { uint8_t padding = 0; fwrite(&padding, 1, 1, stdout); } } return 0; } |
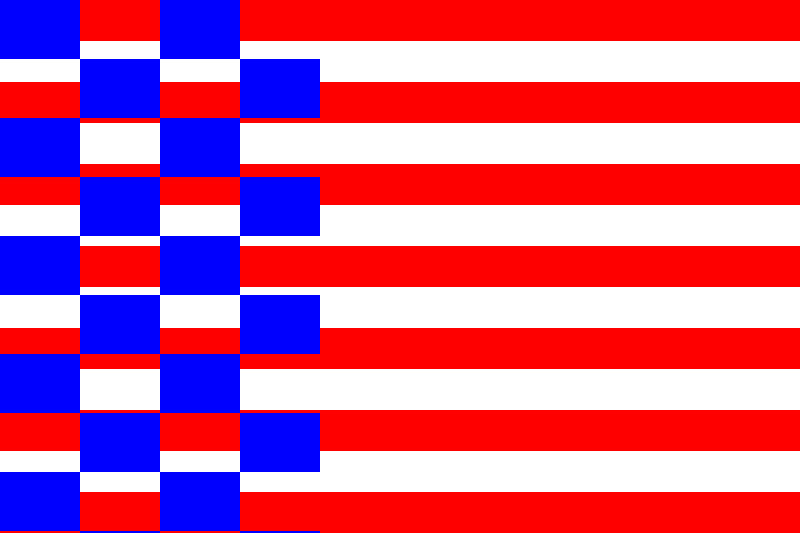
What flag is shown in this image?
The image appears to be a corrupted or glitched version of the flag of the United States of America. The flag normally consists of 13 horizontal stripes, seven red alternating with six white, and a blue field in the canton (upper left corner) bearing fifty small, white, five-pointed stars arranged in nine offset horizontal rows. However, in this image, the pattern is disrupted, and the elements are not aligned correctly.
Testing if the previous output contains the string America
: True
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True