Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a simplified American flag in BMP format and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 | #include <stdio.h> #include <stdlib.h> #define WIDTH 400 #define HEIGHT 250 #define BYTES_PER_PIXEL 3 typedef struct { unsigned char r, g, b; } RGB; void write_bmp_header(int width, int height) { int filesize = 54 + width * height * BYTES_PER_PIXEL; putchar('B'); putchar('M'); fwrite(&filesize, 4, 1, stdout); putchar(0); putchar(0); putchar(0); putchar(0); putchar(54); putchar(0); putchar(0); putchar(0); int header_size = 40; fwrite(&header_size, 4, 1, stdout); fwrite(&width, 4, 1, stdout); fwrite(&height, 4, 1, stdout); putchar(1); putchar(0); putchar(24); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); } void write_pixel(RGB pixel) { putchar(pixel.b); putchar(pixel.g); putchar(pixel.r); } int main() { RGB red = {0, 0, 255}; RGB white = {255, 255, 255}; RGB blue = {255, 0, 0}; write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (y < HEIGHT / 2) { if (y < HEIGHT / 13 || (y >= HEIGHT / 13 * 2 && y < HEIGHT / 13 * 3) || (y >= HEIGHT / 13 * 4 && y < HEIGHT / 13 * 5) || (y >= HEIGHT / 13 * 6)) { if (x < WIDTH / 5) write_pixel(blue); else write_pixel(red); } else { if (x < WIDTH / 5) write_pixel(white); else write_pixel(red); } } else { if (y % (HEIGHT / 13) < HEIGHT / 26) write_pixel(white); else write_pixel(red); } } } return 0; } |
1 2 | gcc -o american_flag american_flag.c ./american_flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 | #include <stdio.h> #include <stdlib.h> #define WIDTH 400 #define HEIGHT 250 #define BYTES_PER_PIXEL 3 typedef struct { unsigned char r, g, b; } RGB; void write_bmp_header(int width, int height) { int filesize = 54 + width * height * BYTES_PER_PIXEL; putchar('B'); putchar('M'); fwrite(&filesize, 4, 1, stdout); putchar(0); putchar(0); putchar(0); putchar(0); putchar(54); putchar(0); putchar(0); putchar(0); int header_size = 40; fwrite(&header_size, 4, 1, stdout); fwrite(&width, 4, 1, stdout); fwrite(&height, 4, 1, stdout); putchar(1); putchar(0); putchar(24); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); } void write_pixel(RGB pixel) { putchar(pixel.b); putchar(pixel.g); putchar(pixel.r); } int main() { RGB red = {0, 0, 255}; RGB white = {255, 255, 255}; RGB blue = {255, 0, 0}; write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (y < HEIGHT / 2) { if (y < HEIGHT / 13 || (y >= HEIGHT / 13 * 2 && y < HEIGHT / 13 * 3) || (y >= HEIGHT / 13 * 4 && y < HEIGHT / 13 * 5) || (y >= HEIGHT / 13 * 6)) { if (x < WIDTH / 5) write_pixel(blue); else write_pixel(red); } else { if (x < WIDTH / 5) write_pixel(white); else write_pixel(red); } } else { if (y % (HEIGHT / 13) < HEIGHT / 26) write_pixel(white); else write_pixel(red); } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 | #include <stdio.h> #include <stdlib.h> #define WIDTH 400 #define HEIGHT 250 #define BYTES_PER_PIXEL 3 typedef struct { unsigned char r, g, b; } RGB; void write_bmp_header(int width, int height) { int filesize = 54 + width * height * BYTES_PER_PIXEL; putchar('B'); putchar('M'); fwrite(&filesize, 4, 1, stdout); putchar(0); putchar(0); putchar(0); putchar(0); putchar(54); putchar(0); putchar(0); putchar(0); int header_size = 40; fwrite(&header_size, 4, 1, stdout); fwrite(&width, 4, 1, stdout); fwrite(&height, 4, 1, stdout); putchar(1); putchar(0); putchar(24); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); } void write_pixel(RGB pixel) { putchar(pixel.b); putchar(pixel.g); putchar(pixel.r); } int main() { RGB red = {0, 0, 255}; RGB white = {255, 255, 255}; RGB blue = {255, 0, 0}; write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (y < HEIGHT / 2) { if (y < HEIGHT / 13 || (y >= HEIGHT / 13 * 2 && y < HEIGHT / 13 * 3) || (y >= HEIGHT / 13 * 4 && y < HEIGHT / 13 * 5) || (y >= HEIGHT / 13 * 6)) { if (x < WIDTH / 5) write_pixel(blue); else write_pixel(red); } else { if (x < WIDTH / 5) write_pixel(white); else write_pixel(red); } } else { if (y % (HEIGHT / 13) < HEIGHT / 26) write_pixel(white); else write_pixel(red); } } } return 0; } |
b'BM\x16\x94\x04\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\x90\x01\x00\x00\xfa\x00\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x
What flag is shown in this image?
image file is truncated (1196 bytes not processed)
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a simplified version of the American flag as a BMP image and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PADDING 3 void write_bmp_header(int width, int height) { printf("BM"); uint32_t filesize = 54 + (width * BYTES_PER_PIXEL + PADDING) * height; printf("%c%c%c%c", (unsigned char)(filesize), (unsigned char)(filesize >> 8), (unsigned char)(filesize >> 16), (unsigned char)(filesize >> 24)); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 54, 0, 0, 0); printf("%c%c%c%c", 40, 0, 0, 0); printf("%c%c%c%c", (unsigned char)(width), (unsigned char)(width >> 8), (unsigned char)(width >> 16), (unsigned char)(width >> 24)); printf("%c%c%c%c", (unsigned char)(height), (unsigned char)(height >> 8), (unsigned char)(height >> 16), (unsigned char)(height >> 24)); printf("%c%c", 1, 0); printf("%c%c", BYTES_PER_PIXEL * 8, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); } void write_pixel(unsigned char r, unsigned char g, unsigned char b) { printf("%c%c%c", b, g, r); } void write_padding(int padding) { for (int i = 0; i < padding; i++) { printf("%c", 0); } } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (y < HEIGHT / 2) { if (x < WIDTH * 2 / 5) { if ((y / 20 + x / 30) % 2 == 0) { write_pixel(255, 255, 255); } else { write_pixel(0, 0, 128); } } else { write_pixel(255, 0, 0); } } else { if (x % (WIDTH / 13) < (WIDTH / 26)) { write_pixel(255, 255, 255); } else { write_pixel(255, 0, 0); } } } write_padding(PADDING); } return 0; } |
1 2 | gcc -o american_flag american_flag.c ./american_flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PADDING 3 void write_bmp_header(int width, int height) { printf("BM"); uint32_t filesize = 54 + (width * BYTES_PER_PIXEL + PADDING) * height; printf("%c%c%c%c", (unsigned char)(filesize), (unsigned char)(filesize >> 8), (unsigned char)(filesize >> 16), (unsigned char)(filesize >> 24)); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 54, 0, 0, 0); printf("%c%c%c%c", 40, 0, 0, 0); printf("%c%c%c%c", (unsigned char)(width), (unsigned char)(width >> 8), (unsigned char)(width >> 16), (unsigned char)(width >> 24)); printf("%c%c%c%c", (unsigned char)(height), (unsigned char)(height >> 8), (unsigned char)(height >> 16), (unsigned char)(height >> 24)); printf("%c%c", 1, 0); printf("%c%c", BYTES_PER_PIXEL * 8, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); } void write_pixel(unsigned char r, unsigned char g, unsigned char b) { printf("%c%c%c", b, g, r); } void write_padding(int padding) { for (int i = 0; i < padding; i++) { printf("%c", 0); } } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (y < HEIGHT / 2) { if (x < WIDTH * 2 / 5) { if ((y / 20 + x / 30) % 2 == 0) { write_pixel(255, 255, 255); } else { write_pixel(0, 0, 128); } } else { write_pixel(255, 0, 0); } } else { if (x % (WIDTH / 13) < (WIDTH / 26)) { write_pixel(255, 255, 255); } else { write_pixel(255, 0, 0); } } } write_padding(PADDING); } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PADDING 3 void write_bmp_header(int width, int height) { printf("BM"); uint32_t filesize = 54 + (width * BYTES_PER_PIXEL + PADDING) * height; printf("%c%c%c%c", (unsigned char)(filesize), (unsigned char)(filesize >> 8), (unsigned char)(filesize >> 16), (unsigned char)(filesize >> 24)); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 54, 0, 0, 0); printf("%c%c%c%c", 40, 0, 0, 0); printf("%c%c%c%c", (unsigned char)(width), (unsigned char)(width >> 8), (unsigned char)(width >> 16), (unsigned char)(width >> 24)); printf("%c%c%c%c", (unsigned char)(height), (unsigned char)(height >> 8), (unsigned char)(height >> 16), (unsigned char)(height >> 24)); printf("%c%c", 1, 0); printf("%c%c", BYTES_PER_PIXEL * 8, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); } void write_pixel(unsigned char r, unsigned char g, unsigned char b) { printf("%c%c%c", b, g, r); } void write_padding(int padding) { for (int i = 0; i < padding; i++) { printf("%c", 0); } } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (y < HEIGHT / 2) { if (x < WIDTH * 2 / 5) { if ((y / 20 + x / 30) % 2 == 0) { write_pixel(255, 255, 255); } else { write_pixel(0, 0, 128); } } else { write_pixel(255, 0, 0); } } else { if (x % (WIDTH / 13) < (WIDTH / 26)) { write_pixel(255, 255, 255); } else { write_pixel(255, 0, 0); } } } write_padding(PADDING); } return 0; } |
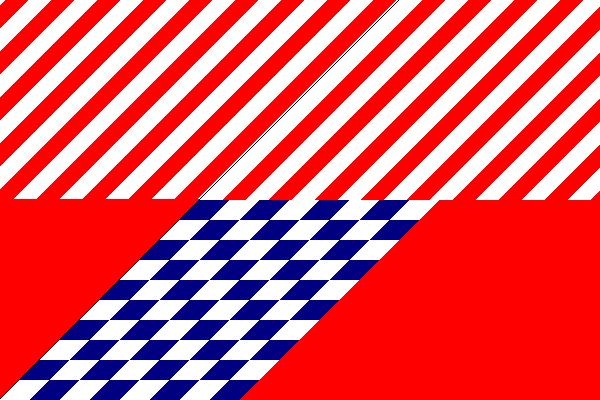
What flag is shown in this image?
The image you've provided appears to be a stylized or altered version of a flag, rather than an official flag of a recognized country or entity. The design features a diagonal bicolor of red and white with a checkered pattern on the lower hoist side and red and white stripes on the upper fly side. This design does not correspond to any widely recognized national flag. It's possible that this is a fictional flag, a flag for a specific organization, or a custom design not associated with any official entity.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a .bmp image of an American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 | #include <stdio.h> #include <stdint.h> #define WIDTH 800 #define HEIGHT 533 #define HEADER_SIZE 54 typedef struct { uint8_t r; uint8_t g; uint8_t b; } Pixel; void write_header(int width, int height) { uint32_t file_size = HEADER_SIZE + width * height * sizeof(Pixel); uint32_t image_size = width * height * sizeof(Pixel); printf("BM"); printf("%c%c%c%c", file_size & 0xff, (file_size >> 8) & 0xff, (file_size >> 16) & 0xff, (file_size >> 24) & 0xff); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 54, 0, 0, 0); printf("%c%c%c%c", 40, 0, 0, 0); printf("%c%c%c%c", width & 0xff, (width >> 8) & 0xff, (width >> 16) & 0xff, (width >> 24) & 0xff); printf("%c%c%c%c", height & 0xff, (height >> 8) & 0xff, (height >> 16) & 0xff, (height >> 24) & 0xff); printf("%c%c", 1, 0); printf("%c%c", 24, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", image_size & 0xff, (image_size >> 8) & 0xff, (image_size >> 16) & 0xff, (image_size >> 24) & 0xff); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); } Pixel get_pixel(int x, int y) { Pixel pixel; if (y < HEIGHT / 13 * 7) { if (x < WIDTH / 2 && y < HEIGHT / 13 * 7 / 2 && ((x / (WIDTH / 26) + y / (HEIGHT / 13)) % 2 == 0)) { pixel.r = 0; pixel.g = 0; pixel.b = 128; } else if (x < WIDTH / 2 && y < HEIGHT / 13 * 7 / 2) { pixel.r = 255; pixel.g = 255; pixel.b = 255; } else if ((y / (HEIGHT / 13)) % 2 == 0) { pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { pixel.r = 191; pixel.g = 10; pixel.b = 48; } } else { pixel.r = 255; pixel.g = 255; pixel.b = 255; } return pixel; } int main() { write_header(WIDTH, HEIGHT); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { Pixel pixel = get_pixel(x, y); putchar(pixel.b); putchar(pixel.g); putchar(pixel.r); } } return 0; } |
1 2 | gcc -o american_flag american_flag.c ./american_flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 | #include <stdio.h> #include <stdint.h> #define WIDTH 800 #define HEIGHT 533 #define HEADER_SIZE 54 typedef struct { uint8_t r; uint8_t g; uint8_t b; } Pixel; void write_header(int width, int height) { uint32_t file_size = HEADER_SIZE + width * height * sizeof(Pixel); uint32_t image_size = width * height * sizeof(Pixel); printf("BM"); printf("%c%c%c%c", file_size & 0xff, (file_size >> 8) & 0xff, (file_size >> 16) & 0xff, (file_size >> 24) & 0xff); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 54, 0, 0, 0); printf("%c%c%c%c", 40, 0, 0, 0); printf("%c%c%c%c", width & 0xff, (width >> 8) & 0xff, (width >> 16) & 0xff, (width >> 24) & 0xff); printf("%c%c%c%c", height & 0xff, (height >> 8) & 0xff, (height >> 16) & 0xff, (height >> 24) & 0xff); printf("%c%c", 1, 0); printf("%c%c", 24, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", image_size & 0xff, (image_size >> 8) & 0xff, (image_size >> 16) & 0xff, (image_size >> 24) & 0xff); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); } Pixel get_pixel(int x, int y) { Pixel pixel; if (y < HEIGHT / 13 * 7) { if (x < WIDTH / 2 && y < HEIGHT / 13 * 7 / 2 && ((x / (WIDTH / 26) + y / (HEIGHT / 13)) % 2 == 0)) { pixel.r = 0; pixel.g = 0; pixel.b = 128; } else if (x < WIDTH / 2 && y < HEIGHT / 13 * 7 / 2) { pixel.r = 255; pixel.g = 255; pixel.b = 255; } else if ((y / (HEIGHT / 13)) % 2 == 0) { pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { pixel.r = 191; pixel.g = 10; pixel.b = 48; } } else { pixel.r = 255; pixel.g = 255; pixel.b = 255; } return pixel; } int main() { write_header(WIDTH, HEIGHT); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { Pixel pixel = get_pixel(x, y); putchar(pixel.b); putchar(pixel.g); putchar(pixel.r); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 | #include <stdio.h> #include <stdint.h> #define WIDTH 800 #define HEIGHT 533 #define HEADER_SIZE 54 typedef struct { uint8_t r; uint8_t g; uint8_t b; } Pixel; void write_header(int width, int height) { uint32_t file_size = HEADER_SIZE + width * height * sizeof(Pixel); uint32_t image_size = width * height * sizeof(Pixel); printf("BM"); printf("%c%c%c%c", file_size & 0xff, (file_size >> 8) & 0xff, (file_size >> 16) & 0xff, (file_size >> 24) & 0xff); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 54, 0, 0, 0); printf("%c%c%c%c", 40, 0, 0, 0); printf("%c%c%c%c", width & 0xff, (width >> 8) & 0xff, (width >> 16) & 0xff, (width >> 24) & 0xff); printf("%c%c%c%c", height & 0xff, (height >> 8) & 0xff, (height >> 16) & 0xff, (height >> 24) & 0xff); printf("%c%c", 1, 0); printf("%c%c", 24, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", image_size & 0xff, (image_size >> 8) & 0xff, (image_size >> 16) & 0xff, (image_size >> 24) & 0xff); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); } Pixel get_pixel(int x, int y) { Pixel pixel; if (y < HEIGHT / 13 * 7) { if (x < WIDTH / 2 && y < HEIGHT / 13 * 7 / 2 && ((x / (WIDTH / 26) + y / (HEIGHT / 13)) % 2 == 0)) { pixel.r = 0; pixel.g = 0; pixel.b = 128; } else if (x < WIDTH / 2 && y < HEIGHT / 13 * 7 / 2) { pixel.r = 255; pixel.g = 255; pixel.b = 255; } else if ((y / (HEIGHT / 13)) % 2 == 0) { pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { pixel.r = 191; pixel.g = 10; pixel.b = 48; } } else { pixel.r = 255; pixel.g = 255; pixel.b = 255; } return pixel; } int main() { write_header(WIDTH, HEIGHT); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { Pixel pixel = get_pixel(x, y); putchar(pixel.b); putchar(pixel.g); putchar(pixel.r); } } return 0; } |
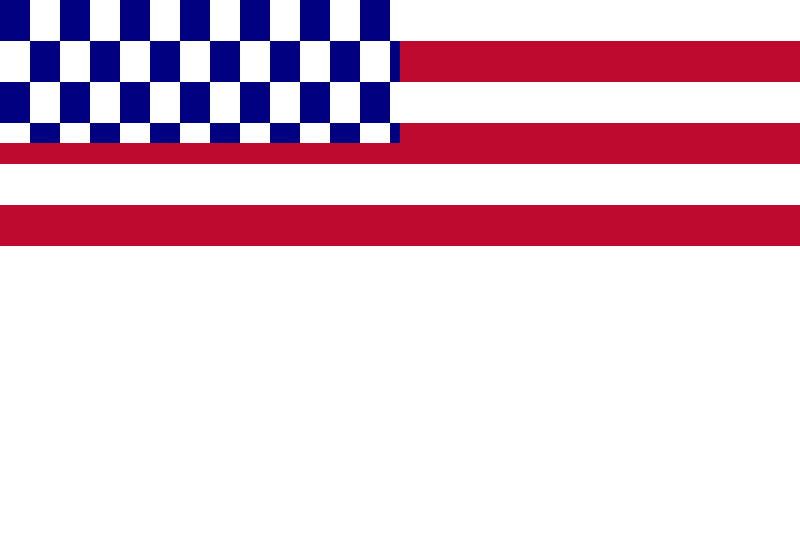
What flag is shown in this image?
The image shows a flag that appears to be a combination of elements from the United States flag and the checkered pattern commonly associated with auto racing flags. However, this is not an official flag of any country or recognized entity. It seems to be a novelty or custom design that merges the iconic red and white stripes of the U.S. flag with a blue and white checkered pattern in the canton area where the stars would normally be.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a simplified depiction of the American flag as a .bmp file and outputs it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 | #include <stdio.h> #include <stdint.h> #define WIDTH 400 #define HEIGHT 200 #define HEADER_SIZE 54 void write_bmp_header(int width, int height) { uint32_t filesize = HEADER_SIZE + (width * height * 3); uint16_t reserved = 0; uint32_t offset = HEADER_SIZE; // BMP header putchar('B'); putchar('M'); fwrite(&filesize, 4, 1, stdout); fwrite(&reserved, 2, 1, stdout); fwrite(&reserved, 2, 1, stdout); fwrite(&offset, 4, 1, stdout); // DIB header (BITMAPINFOHEADER) uint32_t header_size = 40; int32_t width_signed = width; int32_t height_signed = height; uint16_t planes = 1; uint16_t bits_per_pixel = 24; uint32_t compression = 0; uint32_t image_size = 0; int32_t x_pixels_per_meter = 0; int32_t y_pixels_per_meter = 0; uint32_t colors_used = 0; uint32_t important_colors = 0; fwrite(&header_size, 4, 1, stdout); fwrite(&width_signed, 4, 1, stdout); fwrite(&height_signed, 4, 1, stdout); fwrite(&planes, 2, 1, stdout); fwrite(&bits_per_pixel, 2, 1, stdout); fwrite(&compression, 4, 1, stdout); fwrite(&image_size, 4, 1, stdout); fwrite(&x_pixels_per_meter, 4, 1, stdout); fwrite(&y_pixels_per_meter, 4, 1, stdout); fwrite(&colors_used, 4, 1, stdout); fwrite(&important_colors, 4, 1, stdout); } void write_pixel(uint8_t red, uint8_t green, uint8_t blue) { putchar(blue); putchar(green); putchar(red); } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (y < HEIGHT / 2) { if (x < WIDTH / 3) { if ((y / 20) % 2 == 0 && (x / 20) % 2 == 0) { write_pixel(255, 255, 255); // White star } else { write_pixel(0, 0, 128); // Blue background } } else { if ((y / 20) % 2 == 0) { write_pixel(255, 0, 0); // Red stripe } else { write_pixel(255, 255, 255); // White stripe } } } else { if ((y / 20) % 2 == 0) { write_pixel(255, 255, 255); // White stripe } else { write_pixel(255, 0, 0); // Red stripe } } } } return 0; } |
1 2 | gcc -o flag flag.c ./flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 | #include <stdio.h> #include <stdint.h> #define WIDTH 400 #define HEIGHT 200 #define HEADER_SIZE 54 void write_bmp_header(int width, int height) { uint32_t filesize = HEADER_SIZE + (width * height * 3); uint16_t reserved = 0; uint32_t offset = HEADER_SIZE; // BMP header putchar('B'); putchar('M'); fwrite(&filesize, 4, 1, stdout); fwrite(&reserved, 2, 1, stdout); fwrite(&reserved, 2, 1, stdout); fwrite(&offset, 4, 1, stdout); // DIB header (BITMAPINFOHEADER) uint32_t header_size = 40; int32_t width_signed = width; int32_t height_signed = height; uint16_t planes = 1; uint16_t bits_per_pixel = 24; uint32_t compression = 0; uint32_t image_size = 0; int32_t x_pixels_per_meter = 0; int32_t y_pixels_per_meter = 0; uint32_t colors_used = 0; uint32_t important_colors = 0; fwrite(&header_size, 4, 1, stdout); fwrite(&width_signed, 4, 1, stdout); fwrite(&height_signed, 4, 1, stdout); fwrite(&planes, 2, 1, stdout); fwrite(&bits_per_pixel, 2, 1, stdout); fwrite(&compression, 4, 1, stdout); fwrite(&image_size, 4, 1, stdout); fwrite(&x_pixels_per_meter, 4, 1, stdout); fwrite(&y_pixels_per_meter, 4, 1, stdout); fwrite(&colors_used, 4, 1, stdout); fwrite(&important_colors, 4, 1, stdout); } void write_pixel(uint8_t red, uint8_t green, uint8_t blue) { putchar(blue); putchar(green); putchar(red); } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (y < HEIGHT / 2) { if (x < WIDTH / 3) { if ((y / 20) % 2 == 0 && (x / 20) % 2 == 0) { write_pixel(255, 255, 255); // White star } else { write_pixel(0, 0, 128); // Blue background } } else { if ((y / 20) % 2 == 0) { write_pixel(255, 0, 0); // Red stripe } else { write_pixel(255, 255, 255); // White stripe } } } else { if ((y / 20) % 2 == 0) { write_pixel(255, 255, 255); // White stripe } else { write_pixel(255, 0, 0); // Red stripe } } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 | #include <stdio.h> #include <stdint.h> #define WIDTH 400 #define HEIGHT 200 #define HEADER_SIZE 54 void write_bmp_header(int width, int height) { uint32_t filesize = HEADER_SIZE + (width * height * 3); uint16_t reserved = 0; uint32_t offset = HEADER_SIZE; // BMP header putchar('B'); putchar('M'); fwrite(&filesize, 4, 1, stdout); fwrite(&reserved, 2, 1, stdout); fwrite(&reserved, 2, 1, stdout); fwrite(&offset, 4, 1, stdout); // DIB header (BITMAPINFOHEADER) uint32_t header_size = 40; int32_t width_signed = width; int32_t height_signed = height; uint16_t planes = 1; uint16_t bits_per_pixel = 24; uint32_t compression = 0; uint32_t image_size = 0; int32_t x_pixels_per_meter = 0; int32_t y_pixels_per_meter = 0; uint32_t colors_used = 0; uint32_t important_colors = 0; fwrite(&header_size, 4, 1, stdout); fwrite(&width_signed, 4, 1, stdout); fwrite(&height_signed, 4, 1, stdout); fwrite(&planes, 2, 1, stdout); fwrite(&bits_per_pixel, 2, 1, stdout); fwrite(&compression, 4, 1, stdout); fwrite(&image_size, 4, 1, stdout); fwrite(&x_pixels_per_meter, 4, 1, stdout); fwrite(&y_pixels_per_meter, 4, 1, stdout); fwrite(&colors_used, 4, 1, stdout); fwrite(&important_colors, 4, 1, stdout); } void write_pixel(uint8_t red, uint8_t green, uint8_t blue) { putchar(blue); putchar(green); putchar(red); } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (y < HEIGHT / 2) { if (x < WIDTH / 3) { if ((y / 20) % 2 == 0 && (x / 20) % 2 == 0) { write_pixel(255, 255, 255); // White star } else { write_pixel(0, 0, 128); // Blue background } } else { if ((y / 20) % 2 == 0) { write_pixel(255, 0, 0); // Red stripe } else { write_pixel(255, 255, 255); // White stripe } } } else { if ((y / 20) % 2 == 0) { write_pixel(255, 255, 255); // White stripe } else { write_pixel(255, 0, 0); // Red stripe } } } } return 0; } |
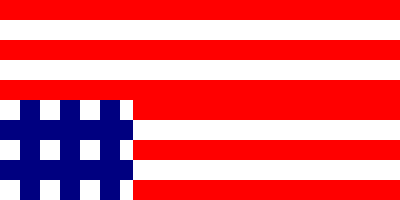
What flag is shown in this image?
The image shows the flag of the United States of America, commonly known as the American flag. It consists of thirteen horizontal stripes of red alternating with white, with a blue rectangle in the canton (referred to specifically as the "union") bearing fifty small, white, five-pointed stars arranged in nine offset horizontal rows of six stars (top and bottom) alternating with rows of five stars. The 50 stars on the flag represent the 50 states of the United States of America, and the 13 stripes represent the thirteen British colonies that declared independence from the Kingdom of Great Britain and became the first states in the U.S.
Testing if the previous output contains the string America
: True
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a BMP image of the American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 | #include <stdio.h> #include <stdint.h> #define WIDTH 800 #define HEIGHT 400 #define HEADER_SIZE 54 typedef struct { uint8_t r; uint8_t g; uint8_t b; } Pixel; void write_header(int width, int height) { uint8_t header[HEADER_SIZE] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 24, 0 }; uint32_t filesize = HEADER_SIZE + width * height * sizeof(Pixel); header[2] = (uint8_t)(filesize); header[3] = (uint8_t)(filesize >> 8); header[4] = (uint8_t)(filesize >> 16); header[5] = (uint8_t)(filesize >> 24); header[18] = (uint8_t)(width); header[19] = (uint8_t)(width >> 8); header[20] = (uint8_t)(width >> 16); header[21] = (uint8_t)(width >> 24); header[22] = (uint8_t)(height); header[23] = (uint8_t)(height >> 8); header[24] = (uint8_t)(height >> 16); header[25] = (uint8_t)(height >> 24); fwrite(header, 1, HEADER_SIZE, stdout); } Pixel get_pixel(int x, int y) { if (y < HEIGHT / 2) { if (x < WIDTH / 2.5) { if ((x / (WIDTH / 25) + y / (HEIGHT / 13)) % 2 == 0) { return (Pixel){255, 255, 255}; } else { return (Pixel){0, 0, 128}; } } else { return (Pixel){255, 0, 0}; } } else { if (x < WIDTH / 2.5) { return (Pixel){255, 255, 255}; } else { return (Pixel){255, 0, 0}; } } } int main() { write_header(WIDTH, HEIGHT); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { Pixel pixel = get_pixel(x, y); fwrite(&pixel, sizeof(Pixel), 1, stdout); } } return 0; } |
1 2 | gcc -o american_flag american_flag.c ./american_flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 | #include <stdio.h> #include <stdint.h> #define WIDTH 800 #define HEIGHT 400 #define HEADER_SIZE 54 typedef struct { uint8_t r; uint8_t g; uint8_t b; } Pixel; void write_header(int width, int height) { uint8_t header[HEADER_SIZE] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 24, 0 }; uint32_t filesize = HEADER_SIZE + width * height * sizeof(Pixel); header[2] = (uint8_t)(filesize); header[3] = (uint8_t)(filesize >> 8); header[4] = (uint8_t)(filesize >> 16); header[5] = (uint8_t)(filesize >> 24); header[18] = (uint8_t)(width); header[19] = (uint8_t)(width >> 8); header[20] = (uint8_t)(width >> 16); header[21] = (uint8_t)(width >> 24); header[22] = (uint8_t)(height); header[23] = (uint8_t)(height >> 8); header[24] = (uint8_t)(height >> 16); header[25] = (uint8_t)(height >> 24); fwrite(header, 1, HEADER_SIZE, stdout); } Pixel get_pixel(int x, int y) { if (y < HEIGHT / 2) { if (x < WIDTH / 2.5) { if ((x / (WIDTH / 25) + y / (HEIGHT / 13)) % 2 == 0) { return (Pixel){255, 255, 255}; } else { return (Pixel){0, 0, 128}; } } else { return (Pixel){255, 0, 0}; } } else { if (x < WIDTH / 2.5) { return (Pixel){255, 255, 255}; } else { return (Pixel){255, 0, 0}; } } } int main() { write_header(WIDTH, HEIGHT); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { Pixel pixel = get_pixel(x, y); fwrite(&pixel, sizeof(Pixel), 1, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 | #include <stdio.h> #include <stdint.h> #define WIDTH 800 #define HEIGHT 400 #define HEADER_SIZE 54 typedef struct { uint8_t r; uint8_t g; uint8_t b; } Pixel; void write_header(int width, int height) { uint8_t header[HEADER_SIZE] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 24, 0 }; uint32_t filesize = HEADER_SIZE + width * height * sizeof(Pixel); header[2] = (uint8_t)(filesize); header[3] = (uint8_t)(filesize >> 8); header[4] = (uint8_t)(filesize >> 16); header[5] = (uint8_t)(filesize >> 24); header[18] = (uint8_t)(width); header[19] = (uint8_t)(width >> 8); header[20] = (uint8_t)(width >> 16); header[21] = (uint8_t)(width >> 24); header[22] = (uint8_t)(height); header[23] = (uint8_t)(height >> 8); header[24] = (uint8_t)(height >> 16); header[25] = (uint8_t)(height >> 24); fwrite(header, 1, HEADER_SIZE, stdout); } Pixel get_pixel(int x, int y) { if (y < HEIGHT / 2) { if (x < WIDTH / 2.5) { if ((x / (WIDTH / 25) + y / (HEIGHT / 13)) % 2 == 0) { return (Pixel){255, 255, 255}; } else { return (Pixel){0, 0, 128}; } } else { return (Pixel){255, 0, 0}; } } else { if (x < WIDTH / 2.5) { return (Pixel){255, 255, 255}; } else { return (Pixel){255, 0, 0}; } } } int main() { write_header(WIDTH, HEIGHT); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { Pixel pixel = get_pixel(x, y); fwrite(&pixel, sizeof(Pixel), 1, stdout); } } return 0; } |
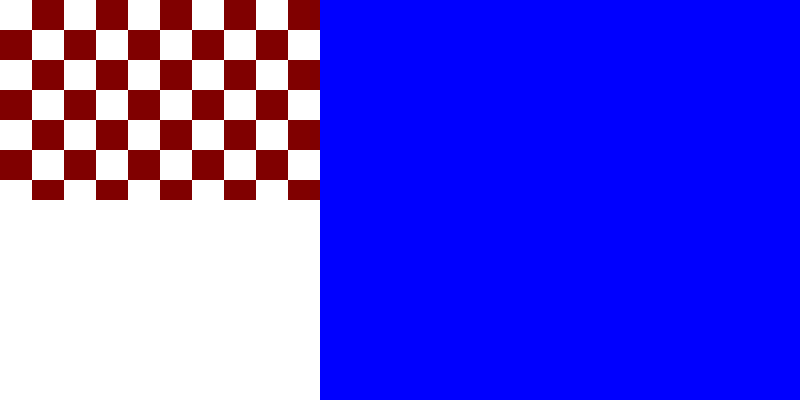
What flag is shown in this image?
The image you've provided appears to be a digital representation of the national flag of Croatia. The flag consists of three horizontal bands of color (red, white, and blue) with the Croatian coat of arms positioned at the hoist side. The coat of arms features a distinctive red and white checkerboard pattern known as the šahovnica.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False