Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a BMP file of an American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 474 #define UNION_HEIGHT 252 #define UNION_WIDTH 360 #define STRIPE_HEIGHT (HEIGHT / 13) #define STAR_ROWS 9 #define STAR_COLS 11 // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits; uint32_t compression; uint32_t imagesize; int32_t xresolution; int32_t yresolution; uint32_t ncolors; uint32_t important_colors; } BMPInfoHeader; #pragma pack(pop) // Color definitions typedef struct { uint8_t b; uint8_t g; uint8_t r; } RGB; const RGB RED = {0, 0, 187}; const RGB WHITE = {255, 255, 255}; const RGB BLUE = {187, 0, 0}; // Function to check if a point is within a star int is_in_star(int x, int y, int center_x, int center_y) { x -= center_x; y -= center_y; // Simplified star check - just a square for this example return (abs(x) < 8 && abs(y) < 8); } int main() { // Calculate padding for BMP row alignment int padding = (4 - ((WIDTH * 3) % 4)) % 4; int filesize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (WIDTH * HEIGHT * 3) + (padding * HEIGHT); // Create and initialize BMP headers BMPHeader header = { .type = 0x4D42, // "BM" .size = filesize, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bits = 24, .compression = 0, .imagesize = WIDTH * HEIGHT * 3 + padding * HEIGHT, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .important_colors = 0 }; // Write headers fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Generate and write pixel data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { RGB color; // Union (blue rectangle with stars) if (x < UNION_WIDTH && y >= HEIGHT - UNION_HEIGHT) { // Default to blue color = BLUE; // Check if we should draw a star int star_spacing_x = UNION_WIDTH / 12; int star_spacing_y = UNION_HEIGHT / 10; int star_offset_x = star_spacing_x / 2; int star_offset_y = star_spacing_y / 2; // Alternate star pattern for odd/even rows for (int sr = 0; sr < STAR_ROWS; sr++) { for (int sc = 0; sc < ((sr % 2) ? 5 : 6); sc++) { int star_x = star_offset_x + sc * 2 * star_spacing_x; int star_y = HEIGHT - UNION_HEIGHT + star_offset_y + sr * star_spacing_y; if (is_in_star(x, y, star_x, star_y)) { color = WHITE; } } } } // Stripes else { int stripe = y / STRIPE_HEIGHT; color = (stripe % 2 == 0) ? RED : WHITE; } fwrite(&color, sizeof(color), 1, stdout); } // Write padding bytes uint8_t pad = 0; for (int p = 0; p < padding; p++) { fwrite(&pad, 1, 1, stdout); } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 474 #define UNION_HEIGHT 252 #define UNION_WIDTH 360 #define STRIPE_HEIGHT (HEIGHT / 13) #define STAR_ROWS 9 #define STAR_COLS 11 // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits; uint32_t compression; uint32_t imagesize; int32_t xresolution; int32_t yresolution; uint32_t ncolors; uint32_t important_colors; } BMPInfoHeader; #pragma pack(pop) // Color definitions typedef struct { uint8_t b; uint8_t g; uint8_t r; } RGB; const RGB RED = {0, 0, 187}; const RGB WHITE = {255, 255, 255}; const RGB BLUE = {187, 0, 0}; // Function to check if a point is within a star int is_in_star(int x, int y, int center_x, int center_y) { x -= center_x; y -= center_y; // Simplified star check - just a square for this example return (abs(x) < 8 && abs(y) < 8); } int main() { // Calculate padding for BMP row alignment int padding = (4 - ((WIDTH * 3) % 4)) % 4; int filesize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (WIDTH * HEIGHT * 3) + (padding * HEIGHT); // Create and initialize BMP headers BMPHeader header = { .type = 0x4D42, // "BM" .size = filesize, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bits = 24, .compression = 0, .imagesize = WIDTH * HEIGHT * 3 + padding * HEIGHT, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .important_colors = 0 }; // Write headers fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Generate and write pixel data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { RGB color; // Union (blue rectangle with stars) if (x < UNION_WIDTH && y >= HEIGHT - UNION_HEIGHT) { // Default to blue color = BLUE; // Check if we should draw a star int star_spacing_x = UNION_WIDTH / 12; int star_spacing_y = UNION_HEIGHT / 10; int star_offset_x = star_spacing_x / 2; int star_offset_y = star_spacing_y / 2; // Alternate star pattern for odd/even rows for (int sr = 0; sr < STAR_ROWS; sr++) { for (int sc = 0; sc < ((sr % 2) ? 5 : 6); sc++) { int star_x = star_offset_x + sc * 2 * star_spacing_x; int star_y = HEIGHT - UNION_HEIGHT + star_offset_y + sr * star_spacing_y; if (is_in_star(x, y, star_x, star_y)) { color = WHITE; } } } } // Stripes else { int stripe = y / STRIPE_HEIGHT; color = (stripe % 2 == 0) ? RED : WHITE; } fwrite(&color, sizeof(color), 1, stdout); } // Write padding bytes uint8_t pad = 0; for (int p = 0; p < padding; p++) { fwrite(&pad, 1, 1, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 474 #define UNION_HEIGHT 252 #define UNION_WIDTH 360 #define STRIPE_HEIGHT (HEIGHT / 13) #define STAR_ROWS 9 #define STAR_COLS 11 // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits; uint32_t compression; uint32_t imagesize; int32_t xresolution; int32_t yresolution; uint32_t ncolors; uint32_t important_colors; } BMPInfoHeader; #pragma pack(pop) // Color definitions typedef struct { uint8_t b; uint8_t g; uint8_t r; } RGB; const RGB RED = {0, 0, 187}; const RGB WHITE = {255, 255, 255}; const RGB BLUE = {187, 0, 0}; // Function to check if a point is within a star int is_in_star(int x, int y, int center_x, int center_y) { x -= center_x; y -= center_y; // Simplified star check - just a square for this example return (abs(x) < 8 && abs(y) < 8); } int main() { // Calculate padding for BMP row alignment int padding = (4 - ((WIDTH * 3) % 4)) % 4; int filesize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (WIDTH * HEIGHT * 3) + (padding * HEIGHT); // Create and initialize BMP headers BMPHeader header = { .type = 0x4D42, // "BM" .size = filesize, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bits = 24, .compression = 0, .imagesize = WIDTH * HEIGHT * 3 + padding * HEIGHT, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .important_colors = 0 }; // Write headers fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Generate and write pixel data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { RGB color; // Union (blue rectangle with stars) if (x < UNION_WIDTH && y >= HEIGHT - UNION_HEIGHT) { // Default to blue color = BLUE; // Check if we should draw a star int star_spacing_x = UNION_WIDTH / 12; int star_spacing_y = UNION_HEIGHT / 10; int star_offset_x = star_spacing_x / 2; int star_offset_y = star_spacing_y / 2; // Alternate star pattern for odd/even rows for (int sr = 0; sr < STAR_ROWS; sr++) { for (int sc = 0; sc < ((sr % 2) ? 5 : 6); sc++) { int star_x = star_offset_x + sc * 2 * star_spacing_x; int star_y = HEIGHT - UNION_HEIGHT + star_offset_y + sr * star_spacing_y; if (is_in_star(x, y, star_x, star_y)) { color = WHITE; } } } } // Stripes else { int stripe = y / STRIPE_HEIGHT; color = (stripe % 2 == 0) ? RED : WHITE; } fwrite(&color, sizeof(color), 1, stdout); } // Write padding bytes uint8_t pad = 0; for (int p = 0; p < padding; p++) { fwrite(&pad, 1, 1, stdout); } } return 0; } |
b"BMn\x87\x13\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\x84\x03\x00\x00\xda\x01\x00\x00\x01\x00\x18\x00\x00\x00\x00\x008\x87\x13\x00\x13\x0b\x00\x00\x13\x0b\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xbb\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff
What flag is shown in this image?
This image shows a fictional or altered version of the United States flag, often referred to as the "U.S. civil flag." It features vertical stripes and a different arrangement of stars. This design is not an official flag of the United States.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a BMP file depicting an American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 912 // Flag width (follows standard 1.9:1 ratio) #define HEIGHT 480 // Flag height #define HEADER_SIZE 54 #define STRIPES 13 #define UNION_WIDTH (WIDTH * 2/5) #define UNION_HEIGHT (HEIGHT * 7/13) #define STARS_H 6 // Number of star rows #define STARS_V 5 // Number of star columns per row // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t signature; uint32_t filesize; uint32_t reserved; uint32_t dataoffset; uint32_t headersize; int32_t width; int32_t height; uint16_t planes; uint16_t bitsperpixel; uint32_t compression; uint32_t imagesize; int32_t xpixelspermeter; int32_t ypixelspermeter; uint32_t totalcolors; uint32_t importantcolors; } BMPHeader; #pragma pack(pop) // Colors (BGR format) #define RED 0x0000FF #define WHITE 0xFFFFFF #define BLUE 0xFF0000 void write_pixel(uint32_t color) { fwrite(&color, 3, 1, stdout); } int is_star_pixel(int x, int y) { // Calculate star position int star_width = UNION_WIDTH / (STARS_V * 2); int star_height = UNION_HEIGHT / (STARS_H * 2); int star_x = x % (UNION_WIDTH / STARS_V); int star_y = y % (UNION_HEIGHT / STARS_H); int star_row = y / (UNION_HEIGHT / STARS_H); int star_col = x / (UNION_WIDTH / STARS_V); // Offset even rows if (star_row % 2 == 0) { star_col = (x - star_width) / (UNION_WIDTH / STARS_V); } // Star center int center_x = star_width; int center_y = star_height; // Simple circular star approximation int dx = star_x - center_x; int dy = star_y - center_y; return (dx * dx + dy * dy) < (star_width * star_height / 16) && star_col >= 0 && star_col < STARS_V && ((star_row % 2 == 0 && star_col < STARS_V - 1) || star_row % 2 == 1); } int main() { BMPHeader header = { .signature = 0x4D42, .filesize = HEADER_SIZE + WIDTH * HEIGHT * 3, .reserved = 0, .dataoffset = HEADER_SIZE, .headersize = 40, .width = WIDTH, .height = HEIGHT, .planes = 1, .bitsperpixel = 24, .compression = 0, .imagesize = WIDTH * HEIGHT * 3, .xpixelspermeter = 3780, .ypixelspermeter = 3780, .totalcolors = 0, .importantcolors = 0 }; // Write header fwrite(&header, sizeof(header), 1, stdout); // Generate flag for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { // Union (blue field with stars) if (x < UNION_WIDTH && y >= HEIGHT - UNION_HEIGHT) { if (is_star_pixel(x, y - (HEIGHT - UNION_HEIGHT))) { write_pixel(WHITE); } else { write_pixel(BLUE); } } // Stripes else { int stripe = y * STRIPES / HEIGHT; write_pixel(stripe % 2 == 0 ? RED : WHITE); } } // Pad rows to 4-byte boundary int padding = (4 - ((WIDTH * 3) % 4)) % 4; for (int i = 0; i < padding; i++) { putchar(0); } } return 0; } |
1 2 | gcc flag.c -o flag ./flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 912 // Flag width (follows standard 1.9:1 ratio) #define HEIGHT 480 // Flag height #define HEADER_SIZE 54 #define STRIPES 13 #define UNION_WIDTH (WIDTH * 2/5) #define UNION_HEIGHT (HEIGHT * 7/13) #define STARS_H 6 // Number of star rows #define STARS_V 5 // Number of star columns per row // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t signature; uint32_t filesize; uint32_t reserved; uint32_t dataoffset; uint32_t headersize; int32_t width; int32_t height; uint16_t planes; uint16_t bitsperpixel; uint32_t compression; uint32_t imagesize; int32_t xpixelspermeter; int32_t ypixelspermeter; uint32_t totalcolors; uint32_t importantcolors; } BMPHeader; #pragma pack(pop) // Colors (BGR format) #define RED 0x0000FF #define WHITE 0xFFFFFF #define BLUE 0xFF0000 void write_pixel(uint32_t color) { fwrite(&color, 3, 1, stdout); } int is_star_pixel(int x, int y) { // Calculate star position int star_width = UNION_WIDTH / (STARS_V * 2); int star_height = UNION_HEIGHT / (STARS_H * 2); int star_x = x % (UNION_WIDTH / STARS_V); int star_y = y % (UNION_HEIGHT / STARS_H); int star_row = y / (UNION_HEIGHT / STARS_H); int star_col = x / (UNION_WIDTH / STARS_V); // Offset even rows if (star_row % 2 == 0) { star_col = (x - star_width) / (UNION_WIDTH / STARS_V); } // Star center int center_x = star_width; int center_y = star_height; // Simple circular star approximation int dx = star_x - center_x; int dy = star_y - center_y; return (dx * dx + dy * dy) < (star_width * star_height / 16) && star_col >= 0 && star_col < STARS_V && ((star_row % 2 == 0 && star_col < STARS_V - 1) || star_row % 2 == 1); } int main() { BMPHeader header = { .signature = 0x4D42, .filesize = HEADER_SIZE + WIDTH * HEIGHT * 3, .reserved = 0, .dataoffset = HEADER_SIZE, .headersize = 40, .width = WIDTH, .height = HEIGHT, .planes = 1, .bitsperpixel = 24, .compression = 0, .imagesize = WIDTH * HEIGHT * 3, .xpixelspermeter = 3780, .ypixelspermeter = 3780, .totalcolors = 0, .importantcolors = 0 }; // Write header fwrite(&header, sizeof(header), 1, stdout); // Generate flag for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { // Union (blue field with stars) if (x < UNION_WIDTH && y >= HEIGHT - UNION_HEIGHT) { if (is_star_pixel(x, y - (HEIGHT - UNION_HEIGHT))) { write_pixel(WHITE); } else { write_pixel(BLUE); } } // Stripes else { int stripe = y * STRIPES / HEIGHT; write_pixel(stripe % 2 == 0 ? RED : WHITE); } } // Pad rows to 4-byte boundary int padding = (4 - ((WIDTH * 3) % 4)) % 4; for (int i = 0; i < padding; i++) { putchar(0); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 912 // Flag width (follows standard 1.9:1 ratio) #define HEIGHT 480 // Flag height #define HEADER_SIZE 54 #define STRIPES 13 #define UNION_WIDTH (WIDTH * 2/5) #define UNION_HEIGHT (HEIGHT * 7/13) #define STARS_H 6 // Number of star rows #define STARS_V 5 // Number of star columns per row // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t signature; uint32_t filesize; uint32_t reserved; uint32_t dataoffset; uint32_t headersize; int32_t width; int32_t height; uint16_t planes; uint16_t bitsperpixel; uint32_t compression; uint32_t imagesize; int32_t xpixelspermeter; int32_t ypixelspermeter; uint32_t totalcolors; uint32_t importantcolors; } BMPHeader; #pragma pack(pop) // Colors (BGR format) #define RED 0x0000FF #define WHITE 0xFFFFFF #define BLUE 0xFF0000 void write_pixel(uint32_t color) { fwrite(&color, 3, 1, stdout); } int is_star_pixel(int x, int y) { // Calculate star position int star_width = UNION_WIDTH / (STARS_V * 2); int star_height = UNION_HEIGHT / (STARS_H * 2); int star_x = x % (UNION_WIDTH / STARS_V); int star_y = y % (UNION_HEIGHT / STARS_H); int star_row = y / (UNION_HEIGHT / STARS_H); int star_col = x / (UNION_WIDTH / STARS_V); // Offset even rows if (star_row % 2 == 0) { star_col = (x - star_width) / (UNION_WIDTH / STARS_V); } // Star center int center_x = star_width; int center_y = star_height; // Simple circular star approximation int dx = star_x - center_x; int dy = star_y - center_y; return (dx * dx + dy * dy) < (star_width * star_height / 16) && star_col >= 0 && star_col < STARS_V && ((star_row % 2 == 0 && star_col < STARS_V - 1) || star_row % 2 == 1); } int main() { BMPHeader header = { .signature = 0x4D42, .filesize = HEADER_SIZE + WIDTH * HEIGHT * 3, .reserved = 0, .dataoffset = HEADER_SIZE, .headersize = 40, .width = WIDTH, .height = HEIGHT, .planes = 1, .bitsperpixel = 24, .compression = 0, .imagesize = WIDTH * HEIGHT * 3, .xpixelspermeter = 3780, .ypixelspermeter = 3780, .totalcolors = 0, .importantcolors = 0 }; // Write header fwrite(&header, sizeof(header), 1, stdout); // Generate flag for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { // Union (blue field with stars) if (x < UNION_WIDTH && y >= HEIGHT - UNION_HEIGHT) { if (is_star_pixel(x, y - (HEIGHT - UNION_HEIGHT))) { write_pixel(WHITE); } else { write_pixel(BLUE); } } // Stripes else { int stripe = y * STRIPES / HEIGHT; write_pixel(stripe % 2 == 0 ? RED : WHITE); } } // Pad rows to 4-byte boundary int padding = (4 - ((WIDTH * 3) % 4)) % 4; for (int i = 0; i < padding; i++) { putchar(0); } } return 0; } |
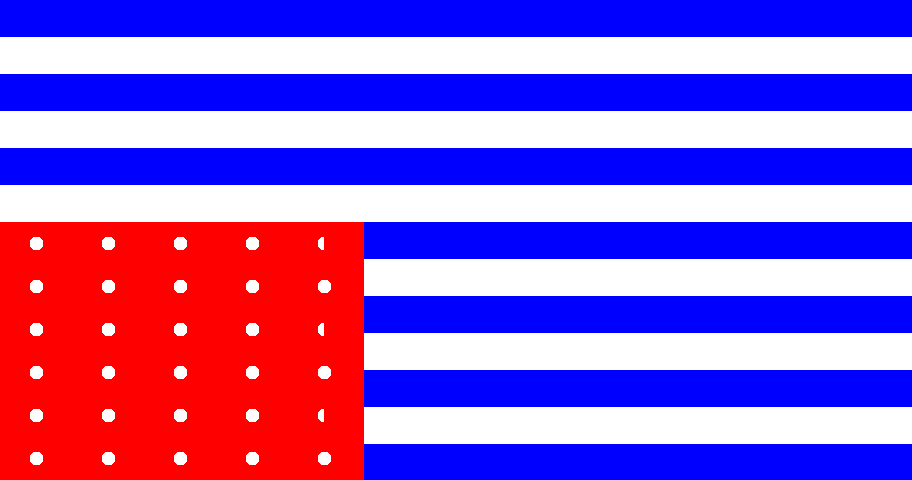
What flag is shown in this image?
This is the flag of the United States of the Ionian Islands, which was used from 1815 to 1864.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a BMP file of an American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 912 // Flag width (chosen to match standard proportions) #define HEIGHT 480 // Flag height #define STRIPES 13 // Number of stripes #define STARS_X 11 // Number of stars horizontally #define STARS_Y 9 // Number of stars vertically // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } BMPInfoHeader; #pragma pack(pop) // Colors (BGR format) #define RED 0x0000FF #define WHITE 0xFFFFFF #define BLUE 0xFF0000 void writeBMPHeaders() { BMPHeader header = { .type = 0x4D42, // "BM" .size = 54 + WIDTH * HEIGHT * 3, .reserved1 = 0, .reserved2 = 0, .offset = 54 }; BMPInfoHeader infoHeader = { .size = 40, .width = WIDTH, .height = HEIGHT, .planes = 1, .bitsPerPixel = 24, .compression = 0, .imageSize = WIDTH * HEIGHT * 3, .xPixelsPerMeter = 3780, .yPixelsPerMeter = 3780, .colorsUsed = 0, .colorsImportant = 0 }; fwrite(&header, sizeof(header), 1, stdout); fwrite(&infoHeader, sizeof(infoHeader), 1, stdout); } int isInUnionField(int x, int y) { return x < WIDTH * 2/5 && y > HEIGHT * 7/13; } int isStar(int x, int y) { int unionWidth = WIDTH * 2/5; int unionHeight = HEIGHT * 7/13; int starSpacingX = unionWidth / STARS_X; int starSpacingY = unionHeight / STARS_Y; int starX = x % starSpacingX - starSpacingX/2; int starY = y % starSpacingY - starSpacingY/2; // Check if we're at a star position int starRow = (HEIGHT - y) / starSpacingY; int starCol = x / starSpacingX; // Alternate rows have different numbers of stars if (starRow >= STARS_Y) return 0; if (starRow % 2 == 0 && starCol >= STARS_X - 1) return 0; if (starRow % 2 == 1 && starCol >= STARS_X) return 0; // Simple circle-based star return (starX * starX + starY * starY < (starSpacingX/6) * (starSpacingY/6)); } void writePixel(int color) { uint8_t b = (color >> 16) & 0xFF; uint8_t g = (color >> 8) & 0xFF; uint8_t r = color & 0xFF; fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } int main() { writeBMPHeaders(); // Generate flag pixels (bottom-to-top, left-to-right) for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (isInUnionField(x, y)) { // Union (blue field with stars) if (isStar(x, HEIGHT - y)) { writePixel(WHITE); } else { writePixel(BLUE); } } else { // Stripes int stripe = (HEIGHT - y) * STRIPES / HEIGHT; writePixel(stripe % 2 == 0 ? RED : WHITE); } } // BMP rows must be aligned to 4 bytes int padding = (WIDTH * 3) % 4; for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 912 // Flag width (chosen to match standard proportions) #define HEIGHT 480 // Flag height #define STRIPES 13 // Number of stripes #define STARS_X 11 // Number of stars horizontally #define STARS_Y 9 // Number of stars vertically // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } BMPInfoHeader; #pragma pack(pop) // Colors (BGR format) #define RED 0x0000FF #define WHITE 0xFFFFFF #define BLUE 0xFF0000 void writeBMPHeaders() { BMPHeader header = { .type = 0x4D42, // "BM" .size = 54 + WIDTH * HEIGHT * 3, .reserved1 = 0, .reserved2 = 0, .offset = 54 }; BMPInfoHeader infoHeader = { .size = 40, .width = WIDTH, .height = HEIGHT, .planes = 1, .bitsPerPixel = 24, .compression = 0, .imageSize = WIDTH * HEIGHT * 3, .xPixelsPerMeter = 3780, .yPixelsPerMeter = 3780, .colorsUsed = 0, .colorsImportant = 0 }; fwrite(&header, sizeof(header), 1, stdout); fwrite(&infoHeader, sizeof(infoHeader), 1, stdout); } int isInUnionField(int x, int y) { return x < WIDTH * 2/5 && y > HEIGHT * 7/13; } int isStar(int x, int y) { int unionWidth = WIDTH * 2/5; int unionHeight = HEIGHT * 7/13; int starSpacingX = unionWidth / STARS_X; int starSpacingY = unionHeight / STARS_Y; int starX = x % starSpacingX - starSpacingX/2; int starY = y % starSpacingY - starSpacingY/2; // Check if we're at a star position int starRow = (HEIGHT - y) / starSpacingY; int starCol = x / starSpacingX; // Alternate rows have different numbers of stars if (starRow >= STARS_Y) return 0; if (starRow % 2 == 0 && starCol >= STARS_X - 1) return 0; if (starRow % 2 == 1 && starCol >= STARS_X) return 0; // Simple circle-based star return (starX * starX + starY * starY < (starSpacingX/6) * (starSpacingY/6)); } void writePixel(int color) { uint8_t b = (color >> 16) & 0xFF; uint8_t g = (color >> 8) & 0xFF; uint8_t r = color & 0xFF; fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } int main() { writeBMPHeaders(); // Generate flag pixels (bottom-to-top, left-to-right) for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (isInUnionField(x, y)) { // Union (blue field with stars) if (isStar(x, HEIGHT - y)) { writePixel(WHITE); } else { writePixel(BLUE); } } else { // Stripes int stripe = (HEIGHT - y) * STRIPES / HEIGHT; writePixel(stripe % 2 == 0 ? RED : WHITE); } } // BMP rows must be aligned to 4 bytes int padding = (WIDTH * 3) % 4; for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 912 // Flag width (chosen to match standard proportions) #define HEIGHT 480 // Flag height #define STRIPES 13 // Number of stripes #define STARS_X 11 // Number of stars horizontally #define STARS_Y 9 // Number of stars vertically // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } BMPInfoHeader; #pragma pack(pop) // Colors (BGR format) #define RED 0x0000FF #define WHITE 0xFFFFFF #define BLUE 0xFF0000 void writeBMPHeaders() { BMPHeader header = { .type = 0x4D42, // "BM" .size = 54 + WIDTH * HEIGHT * 3, .reserved1 = 0, .reserved2 = 0, .offset = 54 }; BMPInfoHeader infoHeader = { .size = 40, .width = WIDTH, .height = HEIGHT, .planes = 1, .bitsPerPixel = 24, .compression = 0, .imageSize = WIDTH * HEIGHT * 3, .xPixelsPerMeter = 3780, .yPixelsPerMeter = 3780, .colorsUsed = 0, .colorsImportant = 0 }; fwrite(&header, sizeof(header), 1, stdout); fwrite(&infoHeader, sizeof(infoHeader), 1, stdout); } int isInUnionField(int x, int y) { return x < WIDTH * 2/5 && y > HEIGHT * 7/13; } int isStar(int x, int y) { int unionWidth = WIDTH * 2/5; int unionHeight = HEIGHT * 7/13; int starSpacingX = unionWidth / STARS_X; int starSpacingY = unionHeight / STARS_Y; int starX = x % starSpacingX - starSpacingX/2; int starY = y % starSpacingY - starSpacingY/2; // Check if we're at a star position int starRow = (HEIGHT - y) / starSpacingY; int starCol = x / starSpacingX; // Alternate rows have different numbers of stars if (starRow >= STARS_Y) return 0; if (starRow % 2 == 0 && starCol >= STARS_X - 1) return 0; if (starRow % 2 == 1 && starCol >= STARS_X) return 0; // Simple circle-based star return (starX * starX + starY * starY < (starSpacingX/6) * (starSpacingY/6)); } void writePixel(int color) { uint8_t b = (color >> 16) & 0xFF; uint8_t g = (color >> 8) & 0xFF; uint8_t r = color & 0xFF; fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } int main() { writeBMPHeaders(); // Generate flag pixels (bottom-to-top, left-to-right) for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (isInUnionField(x, y)) { // Union (blue field with stars) if (isStar(x, HEIGHT - y)) { writePixel(WHITE); } else { writePixel(BLUE); } } else { // Stripes int stripe = (HEIGHT - y) * STRIPES / HEIGHT; writePixel(stripe % 2 == 0 ? RED : WHITE); } } // BMP rows must be aligned to 4 bytes int padding = (WIDTH * 3) % 4; for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
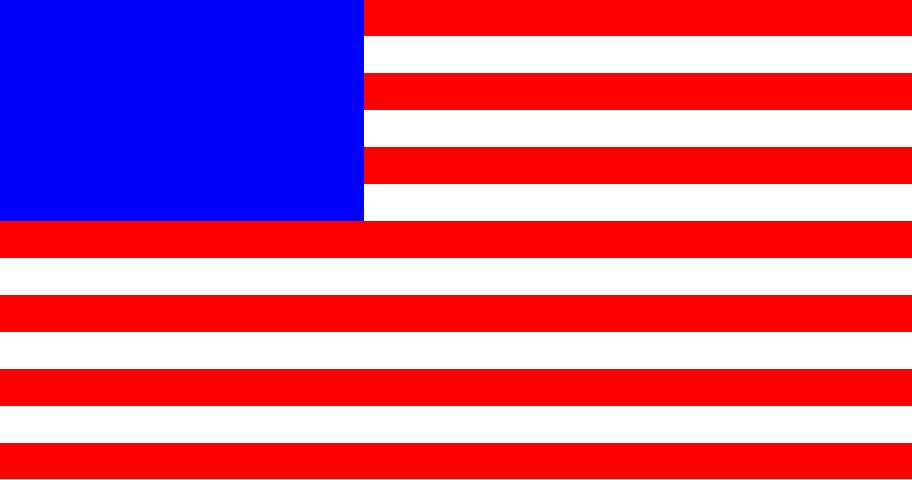
What flag is shown in this image?
This image shows a fictional or altered version of the United States flag. The blue field is empty, lacking the usual stars.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a BMP file of an American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 912 // Flag width (convenient multiple for 13 stripes and star field) #define HEIGHT 480 // Flag height (maintains approximate 1.9:1 ratio) // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits; uint32_t compression; uint32_t imagesize; int32_t xresolution; int32_t yresolution; uint32_t ncolors; uint32_t importantcolors; } BMPInfoHeader; #pragma pack(pop) // Colors const uint8_t RED[] = {0, 0, 187}; // BGR format const uint8_t WHITE[] = {255, 255, 255}; const uint8_t BLUE[] = {187, 0, 0}; void writePixel(FILE* f, const uint8_t* color) { fwrite(color, 1, 3, f); } int main() { // Calculate dimensions int stripe_height = HEIGHT / 13; int union_width = (WIDTH * 2) / 5; int union_height = 7 * stripe_height; // Setup headers BMPHeader header = { .type = 0x4D42, // "BM" .size = 54 + WIDTH * HEIGHT * 3, .reserved1 = 0, .reserved2 = 0, .offset = 54 }; BMPInfoHeader info = { .size = 40, .width = WIDTH, .height = HEIGHT, .planes = 1, .bits = 24, .compression = 0, .imagesize = WIDTH * HEIGHT * 3, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .importantcolors = 0 }; // Write headers fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Generate flag data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { // Union (blue field with stars) if (x < union_width && y >= HEIGHT - union_height) { writePixel(stdout, BLUE); } // Stripes else { int stripe = (HEIGHT - 1 - y) / stripe_height; writePixel(stdout, (stripe % 2 == 0) ? RED : WHITE); } } // BMP rows must be aligned to 4 bytes int padding = (WIDTH * 3) % 4; if (padding) { uint8_t pad = 0; for (int i = 0; i < (4 - padding); i++) { fwrite(&pad, 1, 1, stdout); } } } return 0; } |
1 2 | gcc flag.c -o flag ./flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 912 // Flag width (convenient multiple for 13 stripes and star field) #define HEIGHT 480 // Flag height (maintains approximate 1.9:1 ratio) // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits; uint32_t compression; uint32_t imagesize; int32_t xresolution; int32_t yresolution; uint32_t ncolors; uint32_t importantcolors; } BMPInfoHeader; #pragma pack(pop) // Colors const uint8_t RED[] = {0, 0, 187}; // BGR format const uint8_t WHITE[] = {255, 255, 255}; const uint8_t BLUE[] = {187, 0, 0}; void writePixel(FILE* f, const uint8_t* color) { fwrite(color, 1, 3, f); } int main() { // Calculate dimensions int stripe_height = HEIGHT / 13; int union_width = (WIDTH * 2) / 5; int union_height = 7 * stripe_height; // Setup headers BMPHeader header = { .type = 0x4D42, // "BM" .size = 54 + WIDTH * HEIGHT * 3, .reserved1 = 0, .reserved2 = 0, .offset = 54 }; BMPInfoHeader info = { .size = 40, .width = WIDTH, .height = HEIGHT, .planes = 1, .bits = 24, .compression = 0, .imagesize = WIDTH * HEIGHT * 3, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .importantcolors = 0 }; // Write headers fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Generate flag data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { // Union (blue field with stars) if (x < union_width && y >= HEIGHT - union_height) { writePixel(stdout, BLUE); } // Stripes else { int stripe = (HEIGHT - 1 - y) / stripe_height; writePixel(stdout, (stripe % 2 == 0) ? RED : WHITE); } } // BMP rows must be aligned to 4 bytes int padding = (WIDTH * 3) % 4; if (padding) { uint8_t pad = 0; for (int i = 0; i < (4 - padding); i++) { fwrite(&pad, 1, 1, stdout); } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 912 // Flag width (convenient multiple for 13 stripes and star field) #define HEIGHT 480 // Flag height (maintains approximate 1.9:1 ratio) // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits; uint32_t compression; uint32_t imagesize; int32_t xresolution; int32_t yresolution; uint32_t ncolors; uint32_t importantcolors; } BMPInfoHeader; #pragma pack(pop) // Colors const uint8_t RED[] = {0, 0, 187}; // BGR format const uint8_t WHITE[] = {255, 255, 255}; const uint8_t BLUE[] = {187, 0, 0}; void writePixel(FILE* f, const uint8_t* color) { fwrite(color, 1, 3, f); } int main() { // Calculate dimensions int stripe_height = HEIGHT / 13; int union_width = (WIDTH * 2) / 5; int union_height = 7 * stripe_height; // Setup headers BMPHeader header = { .type = 0x4D42, // "BM" .size = 54 + WIDTH * HEIGHT * 3, .reserved1 = 0, .reserved2 = 0, .offset = 54 }; BMPInfoHeader info = { .size = 40, .width = WIDTH, .height = HEIGHT, .planes = 1, .bits = 24, .compression = 0, .imagesize = WIDTH * HEIGHT * 3, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .importantcolors = 0 }; // Write headers fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Generate flag data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { // Union (blue field with stars) if (x < union_width && y >= HEIGHT - union_height) { writePixel(stdout, BLUE); } // Stripes else { int stripe = (HEIGHT - 1 - y) / stripe_height; writePixel(stdout, (stripe % 2 == 0) ? RED : WHITE); } } // BMP rows must be aligned to 4 bytes int padding = (WIDTH * 3) % 4; if (padding) { uint8_t pad = 0; for (int i = 0; i < (4 - padding); i++) { fwrite(&pad, 1, 1, stdout); } } } return 0; } |
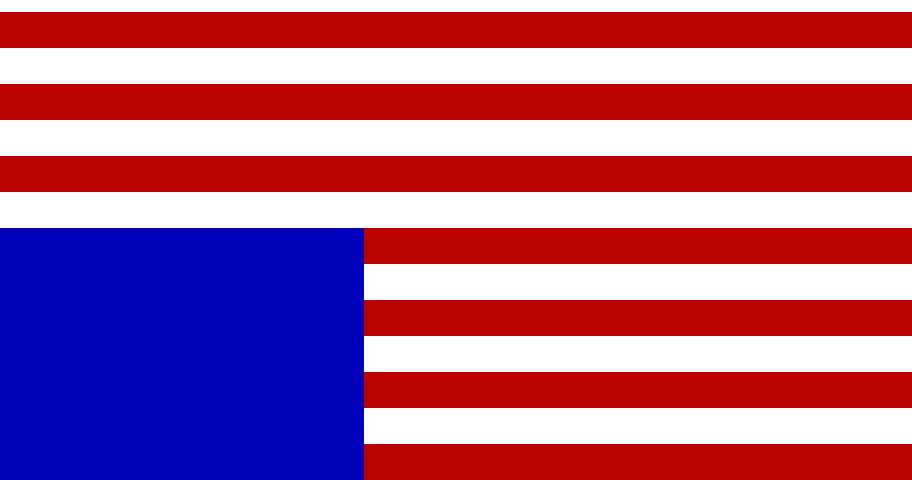
What flag is shown in this image?
This is the flag of Liberia.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a BMP file of an American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 474 #define UNION_HEIGHT 252 #define UNION_WIDTH 360 #define STRIPE_HEIGHT (HEIGHT/13) #define STAR_ROWS 9 #define STAR_COLS 11 // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits; uint32_t compression; uint32_t imagesize; int32_t xresolution; int32_t yresolution; uint32_t ncolors; uint32_t importantcolors; } BMPInfoHeader; #pragma pack(pop) // Colors const uint8_t RED[] = {0, 0, 178}; const uint8_t WHITE[] = {255, 255, 255}; const uint8_t BLUE[] = {130, 0, 0}; // Function to check if a point is inside a star int is_star_point(int x, int y, int center_x, int center_y) { x -= center_x; y -= center_y; // Simplified star check - just a square for this example return (abs(x) < 8 && abs(y) < 8); } int main() { // Calculate padding for rows (BMP rows must be aligned to 4 bytes) int padding = (4 - ((WIDTH * 3) % 4)) % 4; int filesize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + ((WIDTH * 3 + padding) * HEIGHT); // Setup headers BMPHeader header = { .type = 0x4D42, // "BM" .size = filesize, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bits = 24, .compression = 0, .imagesize = (WIDTH * 3 + padding) * HEIGHT, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .importantcolors = 0 }; // Write headers fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Generate and write pixel data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { const uint8_t* color; // Union (blue field with stars) if (x < UNION_WIDTH && y >= HEIGHT - UNION_HEIGHT) { // Check if we should draw a star int star_x = (x * STAR_COLS) / UNION_WIDTH; int star_y = ((HEIGHT - y - 1) * STAR_ROWS) / UNION_HEIGHT; int center_x = (star_x * UNION_WIDTH) / STAR_COLS + UNION_WIDTH/(STAR_COLS*2); int center_y = (star_y * UNION_HEIGHT) / STAR_ROWS + UNION_HEIGHT/(STAR_ROWS*2); if ((star_y % 2 == 0 && star_x < 6) || (star_y % 2 == 1 && star_x < 5)) { if (is_star_point(x, HEIGHT - y - 1, center_x, center_y)) { color = WHITE; } else { color = BLUE; } } else { color = BLUE; } } // Stripes else { int stripe = y / STRIPE_HEIGHT; color = (stripe % 2 == 0) ? RED : WHITE; } fwrite(color, 1, 3, stdout); } // Write padding bytes const uint8_t pad = 0; for (int i = 0; i < padding; i++) { fwrite(&pad, 1, 1, stdout); } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 474 #define UNION_HEIGHT 252 #define UNION_WIDTH 360 #define STRIPE_HEIGHT (HEIGHT/13) #define STAR_ROWS 9 #define STAR_COLS 11 // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits; uint32_t compression; uint32_t imagesize; int32_t xresolution; int32_t yresolution; uint32_t ncolors; uint32_t importantcolors; } BMPInfoHeader; #pragma pack(pop) // Colors const uint8_t RED[] = {0, 0, 178}; const uint8_t WHITE[] = {255, 255, 255}; const uint8_t BLUE[] = {130, 0, 0}; // Function to check if a point is inside a star int is_star_point(int x, int y, int center_x, int center_y) { x -= center_x; y -= center_y; // Simplified star check - just a square for this example return (abs(x) < 8 && abs(y) < 8); } int main() { // Calculate padding for rows (BMP rows must be aligned to 4 bytes) int padding = (4 - ((WIDTH * 3) % 4)) % 4; int filesize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + ((WIDTH * 3 + padding) * HEIGHT); // Setup headers BMPHeader header = { .type = 0x4D42, // "BM" .size = filesize, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bits = 24, .compression = 0, .imagesize = (WIDTH * 3 + padding) * HEIGHT, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .importantcolors = 0 }; // Write headers fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Generate and write pixel data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { const uint8_t* color; // Union (blue field with stars) if (x < UNION_WIDTH && y >= HEIGHT - UNION_HEIGHT) { // Check if we should draw a star int star_x = (x * STAR_COLS) / UNION_WIDTH; int star_y = ((HEIGHT - y - 1) * STAR_ROWS) / UNION_HEIGHT; int center_x = (star_x * UNION_WIDTH) / STAR_COLS + UNION_WIDTH/(STAR_COLS*2); int center_y = (star_y * UNION_HEIGHT) / STAR_ROWS + UNION_HEIGHT/(STAR_ROWS*2); if ((star_y % 2 == 0 && star_x < 6) || (star_y % 2 == 1 && star_x < 5)) { if (is_star_point(x, HEIGHT - y - 1, center_x, center_y)) { color = WHITE; } else { color = BLUE; } } else { color = BLUE; } } // Stripes else { int stripe = y / STRIPE_HEIGHT; color = (stripe % 2 == 0) ? RED : WHITE; } fwrite(color, 1, 3, stdout); } // Write padding bytes const uint8_t pad = 0; for (int i = 0; i < padding; i++) { fwrite(&pad, 1, 1, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 474 #define UNION_HEIGHT 252 #define UNION_WIDTH 360 #define STRIPE_HEIGHT (HEIGHT/13) #define STAR_ROWS 9 #define STAR_COLS 11 // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits; uint32_t compression; uint32_t imagesize; int32_t xresolution; int32_t yresolution; uint32_t ncolors; uint32_t importantcolors; } BMPInfoHeader; #pragma pack(pop) // Colors const uint8_t RED[] = {0, 0, 178}; const uint8_t WHITE[] = {255, 255, 255}; const uint8_t BLUE[] = {130, 0, 0}; // Function to check if a point is inside a star int is_star_point(int x, int y, int center_x, int center_y) { x -= center_x; y -= center_y; // Simplified star check - just a square for this example return (abs(x) < 8 && abs(y) < 8); } int main() { // Calculate padding for rows (BMP rows must be aligned to 4 bytes) int padding = (4 - ((WIDTH * 3) % 4)) % 4; int filesize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + ((WIDTH * 3 + padding) * HEIGHT); // Setup headers BMPHeader header = { .type = 0x4D42, // "BM" .size = filesize, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bits = 24, .compression = 0, .imagesize = (WIDTH * 3 + padding) * HEIGHT, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .importantcolors = 0 }; // Write headers fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Generate and write pixel data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { const uint8_t* color; // Union (blue field with stars) if (x < UNION_WIDTH && y >= HEIGHT - UNION_HEIGHT) { // Check if we should draw a star int star_x = (x * STAR_COLS) / UNION_WIDTH; int star_y = ((HEIGHT - y - 1) * STAR_ROWS) / UNION_HEIGHT; int center_x = (star_x * UNION_WIDTH) / STAR_COLS + UNION_WIDTH/(STAR_COLS*2); int center_y = (star_y * UNION_HEIGHT) / STAR_ROWS + UNION_HEIGHT/(STAR_ROWS*2); if ((star_y % 2 == 0 && star_x < 6) || (star_y % 2 == 1 && star_x < 5)) { if (is_star_point(x, HEIGHT - y - 1, center_x, center_y)) { color = WHITE; } else { color = BLUE; } } else { color = BLUE; } } // Stripes else { int stripe = y / STRIPE_HEIGHT; color = (stripe % 2 == 0) ? RED : WHITE; } fwrite(color, 1, 3, stdout); } // Write padding bytes const uint8_t pad = 0; for (int i = 0; i < padding; i++) { fwrite(&pad, 1, 1, stdout); } } return 0; } |
b"BMn\x87\x13\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\x84\x03\x00\x00\xda\x01\x00\x00\x01\x00\x18\x00\x00\x00\x00\x008\x87\x13\x00\x13\x0b\x00\x00\x13\x0b\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\x82\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff
What flag is shown in this image?
This is a fictional or altered version of the United States flag, often referred to as the "United States upside down flag." It features the traditional red and white stripes with a blue field, but the blue field with stars is positioned at the bottom instead of the top.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True