Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Certainly! Here's a C program that generates a BMP file representing the American flag and writes it to stdout. This program creates a simplified version of the flag with 13 stripes and a blue field with white stars.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 600 #define HEADER_SIZE 54 #define STAR_SIZE 20 // BMP header structure typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width; int32_t height; uint16_t planes; uint16_t bpp; uint32_t compression; uint32_t image_size; int32_t x_resolution; int32_t y_resolution; uint32_t num_colors; uint32_t important_colors; } __attribute__((packed)) BMPHeader; // Function to set a pixel color void set_pixel(uint8_t *image, int x, int y, uint8_t r, uint8_t g, uint8_t b) { int index = (y * WIDTH + x) * 3; image[index] = b; image[index + 1] = g; image[index + 2] = r; } // Function to draw a star void draw_star(uint8_t *image, int center_x, int center_y) { for (int y = -STAR_SIZE/2; y < STAR_SIZE/2; y++) { for (int x = -STAR_SIZE/2; x < STAR_SIZE/2; x++) { if ((abs(x) + abs(y) < STAR_SIZE/2) || (abs(x*2) + abs(y*2) < STAR_SIZE)) { set_pixel(image, center_x + x, center_y + y, 255, 255, 255); } } } } int main() { BMPHeader header = { .type = 0x4D42, .size = HEADER_SIZE + WIDTH * HEIGHT * 3, .reserved1 = 0, .reserved2 = 0, .offset = HEADER_SIZE, .dib_header_size = 40, .width = WIDTH, .height = -HEIGHT, // Negative for top-down image .planes = 1, .bpp = 24, .compression = 0, .image_size = WIDTH * HEIGHT * 3, .x_resolution = 2835, .y_resolution = 2835, .num_colors = 0, .important_colors = 0 }; uint8_t *image = calloc(WIDTH * HEIGHT * 3, 1); if (!image) { fprintf(stderr, "Memory allocation failed\n"); return 1; } // Draw stripes for (int y = 0; y < HEIGHT; y++) { uint8_t r = (y / (HEIGHT / 13)) % 2 == 0 ? 255 : 180; uint8_t g = (y / (HEIGHT / 13)) % 2 == 0 ? 255 : 0; uint8_t b = (y / (HEIGHT / 13)) % 2 == 0 ? 255 : 0; for (int x = 0; x < WIDTH; x++) { set_pixel(image, x, y, r, g, b); } } // Draw blue field for (int y = 0; y < HEIGHT * 7/13; y++) { for (int x = 0; x < WIDTH * 2/5; x++) { set_pixel(image, x, y, 0, 0, 128); } } // Draw stars for (int row = 0; row < 9; row++) { for (int col = 0; col < 11; col++) { if ((row % 2 == 0 && col % 2 == 0) || (row % 2 == 1 && col % 2 == 1)) { int x = col * WIDTH / 22 + WIDTH / 44; int y = row * HEIGHT / 18 + HEIGHT / 26; draw_star(image, x, y); } } } // Write BMP header to stdout fwrite(&header, sizeof(BMPHeader), 1, stdout); // Write image data to stdout fwrite(image, WIDTH * HEIGHT * 3, 1, stdout); free(image); return 0; } |
1 2 | gcc -o american_flag american_flag.c |
1 2 | ./american_flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 600 #define HEADER_SIZE 54 #define STAR_SIZE 20 // BMP header structure typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width; int32_t height; uint16_t planes; uint16_t bpp; uint32_t compression; uint32_t image_size; int32_t x_resolution; int32_t y_resolution; uint32_t num_colors; uint32_t important_colors; } __attribute__((packed)) BMPHeader; // Function to set a pixel color void set_pixel(uint8_t *image, int x, int y, uint8_t r, uint8_t g, uint8_t b) { int index = (y * WIDTH + x) * 3; image[index] = b; image[index + 1] = g; image[index + 2] = r; } // Function to draw a star void draw_star(uint8_t *image, int center_x, int center_y) { for (int y = -STAR_SIZE/2; y < STAR_SIZE/2; y++) { for (int x = -STAR_SIZE/2; x < STAR_SIZE/2; x++) { if ((abs(x) + abs(y) < STAR_SIZE/2) || (abs(x*2) + abs(y*2) < STAR_SIZE)) { set_pixel(image, center_x + x, center_y + y, 255, 255, 255); } } } } int main() { BMPHeader header = { .type = 0x4D42, .size = HEADER_SIZE + WIDTH * HEIGHT * 3, .reserved1 = 0, .reserved2 = 0, .offset = HEADER_SIZE, .dib_header_size = 40, .width = WIDTH, .height = -HEIGHT, // Negative for top-down image .planes = 1, .bpp = 24, .compression = 0, .image_size = WIDTH * HEIGHT * 3, .x_resolution = 2835, .y_resolution = 2835, .num_colors = 0, .important_colors = 0 }; uint8_t *image = calloc(WIDTH * HEIGHT * 3, 1); if (!image) { fprintf(stderr, "Memory allocation failed\n"); return 1; } // Draw stripes for (int y = 0; y < HEIGHT; y++) { uint8_t r = (y / (HEIGHT / 13)) % 2 == 0 ? 255 : 180; uint8_t g = (y / (HEIGHT / 13)) % 2 == 0 ? 255 : 0; uint8_t b = (y / (HEIGHT / 13)) % 2 == 0 ? 255 : 0; for (int x = 0; x < WIDTH; x++) { set_pixel(image, x, y, r, g, b); } } // Draw blue field for (int y = 0; y < HEIGHT * 7/13; y++) { for (int x = 0; x < WIDTH * 2/5; x++) { set_pixel(image, x, y, 0, 0, 128); } } // Draw stars for (int row = 0; row < 9; row++) { for (int col = 0; col < 11; col++) { if ((row % 2 == 0 && col % 2 == 0) || (row % 2 == 1 && col % 2 == 1)) { int x = col * WIDTH / 22 + WIDTH / 44; int y = row * HEIGHT / 18 + HEIGHT / 26; draw_star(image, x, y); } } } // Write BMP header to stdout fwrite(&header, sizeof(BMPHeader), 1, stdout); // Write image data to stdout fwrite(image, WIDTH * HEIGHT * 3, 1, stdout); free(image); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 600 #define HEADER_SIZE 54 #define STAR_SIZE 20 // BMP header structure typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width; int32_t height; uint16_t planes; uint16_t bpp; uint32_t compression; uint32_t image_size; int32_t x_resolution; int32_t y_resolution; uint32_t num_colors; uint32_t important_colors; } __attribute__((packed)) BMPHeader; // Function to set a pixel color void set_pixel(uint8_t *image, int x, int y, uint8_t r, uint8_t g, uint8_t b) { int index = (y * WIDTH + x) * 3; image[index] = b; image[index + 1] = g; image[index + 2] = r; } // Function to draw a star void draw_star(uint8_t *image, int center_x, int center_y) { for (int y = -STAR_SIZE/2; y < STAR_SIZE/2; y++) { for (int x = -STAR_SIZE/2; x < STAR_SIZE/2; x++) { if ((abs(x) + abs(y) < STAR_SIZE/2) || (abs(x*2) + abs(y*2) < STAR_SIZE)) { set_pixel(image, center_x + x, center_y + y, 255, 255, 255); } } } } int main() { BMPHeader header = { .type = 0x4D42, .size = HEADER_SIZE + WIDTH * HEIGHT * 3, .reserved1 = 0, .reserved2 = 0, .offset = HEADER_SIZE, .dib_header_size = 40, .width = WIDTH, .height = -HEIGHT, // Negative for top-down image .planes = 1, .bpp = 24, .compression = 0, .image_size = WIDTH * HEIGHT * 3, .x_resolution = 2835, .y_resolution = 2835, .num_colors = 0, .important_colors = 0 }; uint8_t *image = calloc(WIDTH * HEIGHT * 3, 1); if (!image) { fprintf(stderr, "Memory allocation failed\n"); return 1; } // Draw stripes for (int y = 0; y < HEIGHT; y++) { uint8_t r = (y / (HEIGHT / 13)) % 2 == 0 ? 255 : 180; uint8_t g = (y / (HEIGHT / 13)) % 2 == 0 ? 255 : 0; uint8_t b = (y / (HEIGHT / 13)) % 2 == 0 ? 255 : 0; for (int x = 0; x < WIDTH; x++) { set_pixel(image, x, y, r, g, b); } } // Draw blue field for (int y = 0; y < HEIGHT * 7/13; y++) { for (int x = 0; x < WIDTH * 2/5; x++) { set_pixel(image, x, y, 0, 0, 128); } } // Draw stars for (int row = 0; row < 9; row++) { for (int col = 0; col < 11; col++) { if ((row % 2 == 0 && col % 2 == 0) || (row % 2 == 1 && col % 2 == 1)) { int x = col * WIDTH / 22 + WIDTH / 44; int y = row * HEIGHT / 18 + HEIGHT / 26; draw_star(image, x, y); } } } // Write BMP header to stdout fwrite(&header, sizeof(BMPHeader), 1, stdout); // Write image data to stdout fwrite(image, WIDTH * HEIGHT * 3, 1, stdout); free(image); return 0; } |
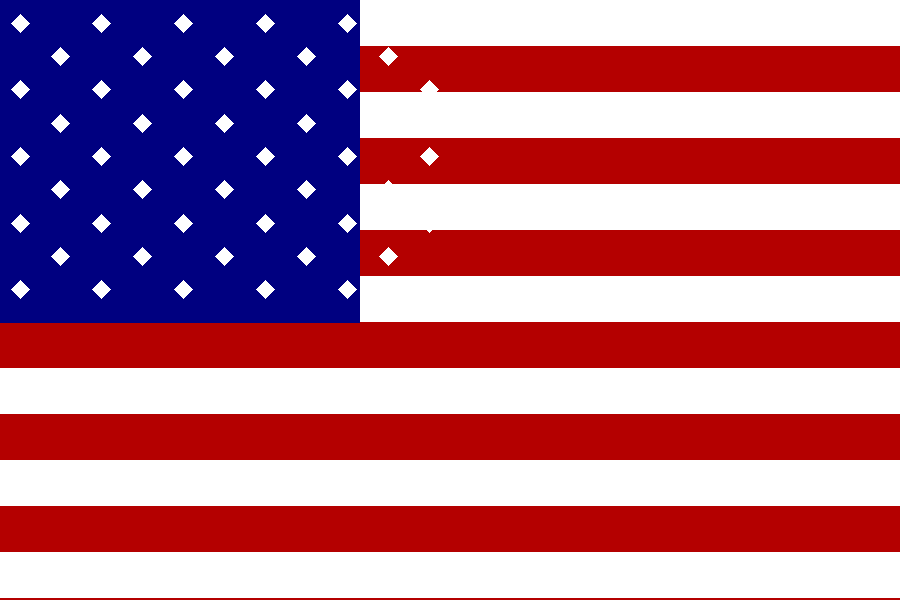
What flag is shown in this image?
The flag shown in the image is a modified version of the flag of the United States. The traditional flag of the United States features 50 stars arranged in a specific pattern on a blue field, representing the 50 states, and 13 horizontal stripes alternating red and white, representing the original 13 colonies. In this modified version, the stars have been replaced with diamond shapes.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a BMP image of an American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 570 #define HEIGHT 300 #define STARS_WIDTH 228 #define STARS_HEIGHT 159 #define STRIPES 13 #define STAR_ROWS 9 #define STAR_COLS 11 // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width_px; int32_t height_px; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size_bytes; int32_t x_resolution_ppm; int32_t y_resolution_ppm; uint32_t num_colors; uint32_t important_colors; } BMPHeader; #pragma pack(pop) // Function to write a pixel void write_pixel(FILE* fp, uint8_t r, uint8_t g, uint8_t b) { fputc(b, fp); fputc(g, fp); fputc(r, fp); } // Function to draw a star void draw_star(uint8_t* image, int x, int y) { for (int dy = -2; dy <= 2; dy++) { for (int dx = -2; dx <= 2; dx++) { if (abs(dx) + abs(dy) <= 2) { int pos = (y + dy) * WIDTH * 3 + (x + dx) * 3; image[pos] = image[pos + 1] = image[pos + 2] = 255; } } } } int main() { BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader), .dib_header_size = 40, .width_px = WIDTH, .height_px = HEIGHT, .num_planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size_bytes = WIDTH * HEIGHT * 3, .x_resolution_ppm = 2835, .y_resolution_ppm = 2835, .num_colors = 0, .important_colors = 0 }; uint8_t* image = calloc(WIDTH * HEIGHT * 3, 1); // Draw stripes for (int y = 0; y < HEIGHT; y++) { uint8_t r = (y / (HEIGHT / STRIPES)) % 2 == 0 ? 178 : 255; uint8_t g = (y / (HEIGHT / STRIPES)) % 2 == 0 ? 34 : 255; uint8_t b = (y / (HEIGHT / STRIPES)) % 2 == 0 ? 52 : 255; for (int x = 0; x < WIDTH; x++) { int pos = y * WIDTH * 3 + x * 3; image[pos] = b; image[pos + 1] = g; image[pos + 2] = r; } } // Draw blue rectangle for (int y = HEIGHT - 1; y >= HEIGHT - STARS_HEIGHT; y--) { for (int x = 0; x < STARS_WIDTH; x++) { int pos = y * WIDTH * 3 + x * 3; image[pos] = 187; image[pos + 1] = 19; image[pos + 2] = 60; } } // Draw stars for (int row = 0; row < STAR_ROWS; row++) { for (int col = 0; col < STAR_COLS; col++) { if ((row % 2 == 0 && col < STAR_COLS) || (row % 2 == 1 && col < STAR_COLS - 1)) { int x = col * (STARS_WIDTH / (STAR_COLS - 1)) + (row % 2 == 1 ? STARS_WIDTH / (STAR_COLS - 1) / 2 : 0); int y = HEIGHT - STARS_HEIGHT + row * (STARS_HEIGHT / (STAR_ROWS - 1)); draw_star(image, x, y); } } } // Write the BMP to stdout fwrite(&header, sizeof(header), 1, stdout); for (int y = HEIGHT - 1; y >= 0; y--) { fwrite(image + y * WIDTH * 3, 1, WIDTH * 3, stdout); } free(image); return 0; } |
1 2 | gcc flag.c -o flag ./flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 | #include <stdio.h> #include <stdint.h> #include <string.h> #include <stdlib.h> #define WIDTH 570 #define HEIGHT 300 #define STARS_WIDTH 228 #define STARS_HEIGHT 159 #define STRIPES 13 #define STAR_ROWS 9 #define STAR_COLS 11 // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width_px; int32_t height_px; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size_bytes; int32_t x_resolution_ppm; int32_t y_resolution_ppm; uint32_t num_colors; uint32_t important_colors; } BMPHeader; #pragma pack(pop) // Function to write a pixel void write_pixel(FILE* fp, uint8_t r, uint8_t g, uint8_t b) { fputc(b, fp); fputc(g, fp); fputc(r, fp); } // Function to draw a star void draw_star(uint8_t* image, int x, int y) { for (int dy = -2; dy <= 2; dy++) { for (int dx = -2; dx <= 2; dx++) { if (abs(dx) + abs(dy) <= 2) { int pos = (y + dy) * WIDTH * 3 + (x + dx) * 3; image[pos] = image[pos + 1] = image[pos + 2] = 255; } } } } int main() { BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader), .dib_header_size = 40, .width_px = WIDTH, .height_px = HEIGHT, .num_planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size_bytes = WIDTH * HEIGHT * 3, .x_resolution_ppm = 2835, .y_resolution_ppm = 2835, .num_colors = 0, .important_colors = 0 }; uint8_t* image = calloc(WIDTH * HEIGHT * 3, 1); // Draw stripes for (int y = 0; y < HEIGHT; y++) { uint8_t r = (y / (HEIGHT / STRIPES)) % 2 == 0 ? 178 : 255; uint8_t g = (y / (HEIGHT / STRIPES)) % 2 == 0 ? 34 : 255; uint8_t b = (y / (HEIGHT / STRIPES)) % 2 == 0 ? 52 : 255; for (int x = 0; x < WIDTH; x++) { int pos = y * WIDTH * 3 + x * 3; image[pos] = b; image[pos + 1] = g; image[pos + 2] = r; } } // Draw blue rectangle for (int y = HEIGHT - 1; y >= HEIGHT - STARS_HEIGHT; y--) { for (int x = 0; x < STARS_WIDTH; x++) { int pos = y * WIDTH * 3 + x * 3; image[pos] = 187; image[pos + 1] = 19; image[pos + 2] = 60; } } // Draw stars for (int row = 0; row < STAR_ROWS; row++) { for (int col = 0; col < STAR_COLS; col++) { if ((row % 2 == 0 && col < STAR_COLS) || (row % 2 == 1 && col < STAR_COLS - 1)) { int x = col * (STARS_WIDTH / (STAR_COLS - 1)) + (row % 2 == 1 ? STARS_WIDTH / (STAR_COLS - 1) / 2 : 0); int y = HEIGHT - STARS_HEIGHT + row * (STARS_HEIGHT / (STAR_ROWS - 1)); draw_star(image, x, y); } } } // Write the BMP to stdout fwrite(&header, sizeof(header), 1, stdout); for (int y = HEIGHT - 1; y >= 0; y--) { fwrite(image + y * WIDTH * 3, 1, WIDTH * 3, stdout); } free(image); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 | #include <stdio.h> #include <stdint.h> #include <string.h> #include <stdlib.h> #define WIDTH 570 #define HEIGHT 300 #define STARS_WIDTH 228 #define STARS_HEIGHT 159 #define STRIPES 13 #define STAR_ROWS 9 #define STAR_COLS 11 // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width_px; int32_t height_px; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size_bytes; int32_t x_resolution_ppm; int32_t y_resolution_ppm; uint32_t num_colors; uint32_t important_colors; } BMPHeader; #pragma pack(pop) // Function to write a pixel void write_pixel(FILE* fp, uint8_t r, uint8_t g, uint8_t b) { fputc(b, fp); fputc(g, fp); fputc(r, fp); } // Function to draw a star void draw_star(uint8_t* image, int x, int y) { for (int dy = -2; dy <= 2; dy++) { for (int dx = -2; dx <= 2; dx++) { if (abs(dx) + abs(dy) <= 2) { int pos = (y + dy) * WIDTH * 3 + (x + dx) * 3; image[pos] = image[pos + 1] = image[pos + 2] = 255; } } } } int main() { BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader), .dib_header_size = 40, .width_px = WIDTH, .height_px = HEIGHT, .num_planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size_bytes = WIDTH * HEIGHT * 3, .x_resolution_ppm = 2835, .y_resolution_ppm = 2835, .num_colors = 0, .important_colors = 0 }; uint8_t* image = calloc(WIDTH * HEIGHT * 3, 1); // Draw stripes for (int y = 0; y < HEIGHT; y++) { uint8_t r = (y / (HEIGHT / STRIPES)) % 2 == 0 ? 178 : 255; uint8_t g = (y / (HEIGHT / STRIPES)) % 2 == 0 ? 34 : 255; uint8_t b = (y / (HEIGHT / STRIPES)) % 2 == 0 ? 52 : 255; for (int x = 0; x < WIDTH; x++) { int pos = y * WIDTH * 3 + x * 3; image[pos] = b; image[pos + 1] = g; image[pos + 2] = r; } } // Draw blue rectangle for (int y = HEIGHT - 1; y >= HEIGHT - STARS_HEIGHT; y--) { for (int x = 0; x < STARS_WIDTH; x++) { int pos = y * WIDTH * 3 + x * 3; image[pos] = 187; image[pos + 1] = 19; image[pos + 2] = 60; } } // Draw stars for (int row = 0; row < STAR_ROWS; row++) { for (int col = 0; col < STAR_COLS; col++) { if ((row % 2 == 0 && col < STAR_COLS) || (row % 2 == 1 && col < STAR_COLS - 1)) { int x = col * (STARS_WIDTH / (STAR_COLS - 1)) + (row % 2 == 1 ? STARS_WIDTH / (STAR_COLS - 1) / 2 : 0); int y = HEIGHT - STARS_HEIGHT + row * (STARS_HEIGHT / (STAR_ROWS - 1)); draw_star(image, x, y); } } } // Write the BMP to stdout fwrite(&header, sizeof(header), 1, stdout); for (int y = HEIGHT - 1; y >= 0; y--) { fwrite(image + y * WIDTH * 3, 1, WIDTH * 3, stdout); } free(image); return 0; } |
b'BM\x1e\xd4\x07\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00:\x02\x00\x00,\x01\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00\xe8\xd3\x07\x00\x13\x0b\x00\x00\x13\x0b\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<\xbb\x13<4"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"\xb24"
What flag is shown in this image?
image file is truncated (1112 bytes not processed)
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a BMP image of an American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 600 // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width_px; int32_t height_px; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size_bytes; int32_t x_resolution_ppm; int32_t y_resolution_ppm; uint32_t num_colors; uint32_t important_colors; } BMPHeader; #pragma pack(pop) void write_bmp_header(BMPHeader* header) { header->type = 0x4D42; // BM header->size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3; header->reserved1 = 0; header->reserved2 = 0; header->offset = sizeof(BMPHeader); header->dib_header_size = 40; header->width_px = WIDTH; header->height_px = HEIGHT; header->num_planes = 1; header->bits_per_pixel = 24; header->compression = 0; header->image_size_bytes = WIDTH * HEIGHT * 3; header->x_resolution_ppm = 2835; header->y_resolution_ppm = 2835; header->num_colors = 0; header->important_colors = 0; } void write_pixel(FILE* stream, uint8_t r, uint8_t g, uint8_t b) { fputc(b, stream); fputc(g, stream); fputc(r, stream); } int main() { BMPHeader header; write_bmp_header(&header); // Write header to stdout fwrite(&header, sizeof(BMPHeader), 1, stdout); // Generate flag image for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH * 2/5 && y >= HEIGHT * 7/13) { // Blue canton write_pixel(stdout, 0, 40, 104); } else if (y % (HEIGHT / 13) < HEIGHT / 26) { // Red stripe write_pixel(stdout, 191, 10, 48); } else { // White stripe write_pixel(stdout, 255, 255, 255); } } } return 0; } |
1 2 | gcc flag.c -o flag ./flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 600 // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width_px; int32_t height_px; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size_bytes; int32_t x_resolution_ppm; int32_t y_resolution_ppm; uint32_t num_colors; uint32_t important_colors; } BMPHeader; #pragma pack(pop) void write_bmp_header(BMPHeader* header) { header->type = 0x4D42; // BM header->size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3; header->reserved1 = 0; header->reserved2 = 0; header->offset = sizeof(BMPHeader); header->dib_header_size = 40; header->width_px = WIDTH; header->height_px = HEIGHT; header->num_planes = 1; header->bits_per_pixel = 24; header->compression = 0; header->image_size_bytes = WIDTH * HEIGHT * 3; header->x_resolution_ppm = 2835; header->y_resolution_ppm = 2835; header->num_colors = 0; header->important_colors = 0; } void write_pixel(FILE* stream, uint8_t r, uint8_t g, uint8_t b) { fputc(b, stream); fputc(g, stream); fputc(r, stream); } int main() { BMPHeader header; write_bmp_header(&header); // Write header to stdout fwrite(&header, sizeof(BMPHeader), 1, stdout); // Generate flag image for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH * 2/5 && y >= HEIGHT * 7/13) { // Blue canton write_pixel(stdout, 0, 40, 104); } else if (y % (HEIGHT / 13) < HEIGHT / 26) { // Red stripe write_pixel(stdout, 191, 10, 48); } else { // White stripe write_pixel(stdout, 255, 255, 255); } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 600 // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width_px; int32_t height_px; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size_bytes; int32_t x_resolution_ppm; int32_t y_resolution_ppm; uint32_t num_colors; uint32_t important_colors; } BMPHeader; #pragma pack(pop) void write_bmp_header(BMPHeader* header) { header->type = 0x4D42; // BM header->size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3; header->reserved1 = 0; header->reserved2 = 0; header->offset = sizeof(BMPHeader); header->dib_header_size = 40; header->width_px = WIDTH; header->height_px = HEIGHT; header->num_planes = 1; header->bits_per_pixel = 24; header->compression = 0; header->image_size_bytes = WIDTH * HEIGHT * 3; header->x_resolution_ppm = 2835; header->y_resolution_ppm = 2835; header->num_colors = 0; header->important_colors = 0; } void write_pixel(FILE* stream, uint8_t r, uint8_t g, uint8_t b) { fputc(b, stream); fputc(g, stream); fputc(r, stream); } int main() { BMPHeader header; write_bmp_header(&header); // Write header to stdout fwrite(&header, sizeof(BMPHeader), 1, stdout); // Generate flag image for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH * 2/5 && y >= HEIGHT * 7/13) { // Blue canton write_pixel(stdout, 0, 40, 104); } else if (y % (HEIGHT / 13) < HEIGHT / 26) { // Red stripe write_pixel(stdout, 191, 10, 48); } else { // White stripe write_pixel(stdout, 255, 255, 255); } } } return 0; } |
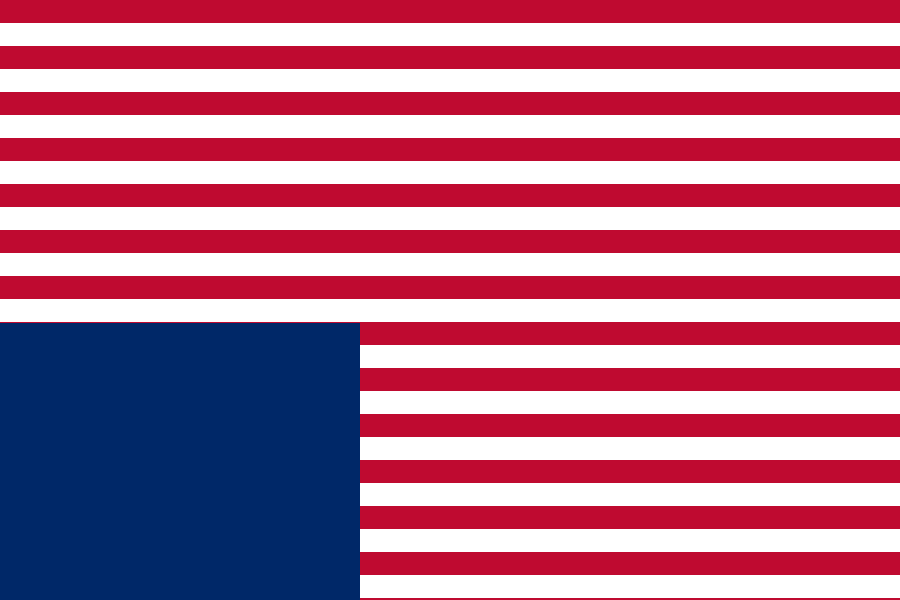
What flag is shown in this image?
The flag shown in the image is the flag of Liberia. The flag features eleven horizontal stripes alternating red and white, with a blue square in the upper left corner. The blue square contains a single white star. The design is similar to the flag of the United States, reflecting Liberia's historical ties to the United States.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a simple representation of the American flag as a BMP file and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 912 #define HEIGHT 480 // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width_px; int32_t height_px; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size_bytes; int32_t x_resolution_ppm; int32_t y_resolution_ppm; uint32_t num_colors; uint32_t important_colors; } BMPHeader; #pragma pack(pop) // Function to set a pixel color void set_pixel(uint8_t* image, int x, int y, uint8_t r, uint8_t g, uint8_t b) { int index = (y * WIDTH + x) * 3; image[index] = b; image[index + 1] = g; image[index + 2] = r; } int main() { BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3, .offset = sizeof(BMPHeader), .dib_header_size = 40, .width_px = WIDTH, .height_px = HEIGHT, .num_planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size_bytes = WIDTH * HEIGHT * 3, .x_resolution_ppm = 2835, .y_resolution_ppm = 2835, .num_colors = 0, .important_colors = 0 }; uint8_t* image = calloc(WIDTH * HEIGHT * 3, 1); // Draw stripes for (int y = 0; y < HEIGHT; y++) { uint8_t r, g, b; if (y % 74 < 37) { r = 178; g = 34; b = 52; // Red stripe } else { r = 255; g = 255; b = 255; // White stripe } for (int x = 0; x < WIDTH; x++) { set_pixel(image, x, y, r, g, b); } } // Draw blue canton for (int y = HEIGHT - 1; y >= HEIGHT - 259; y--) { for (int x = 0; x < 364; x++) { set_pixel(image, x, y, 60, 59, 110); } } // Draw stars (simplified as white dots) for (int row = 0; row < 9; row++) { for (int col = 0; col < 11; col++) { if (row % 2 == 0 && col == 10) continue; int x = 16 + col * 30; int y = HEIGHT - 14 - row * 28; for (int dy = -2; dy <= 2; dy++) { for (int dx = -2; dx <= 2; dx++) { set_pixel(image, x + dx, y + dy, 255, 255, 255); } } } } // Write BMP header and image data to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(image, WIDTH * HEIGHT * 3, 1, stdout); free(image); return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 912 #define HEIGHT 480 // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width_px; int32_t height_px; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size_bytes; int32_t x_resolution_ppm; int32_t y_resolution_ppm; uint32_t num_colors; uint32_t important_colors; } BMPHeader; #pragma pack(pop) // Function to set a pixel color void set_pixel(uint8_t* image, int x, int y, uint8_t r, uint8_t g, uint8_t b) { int index = (y * WIDTH + x) * 3; image[index] = b; image[index + 1] = g; image[index + 2] = r; } int main() { BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3, .offset = sizeof(BMPHeader), .dib_header_size = 40, .width_px = WIDTH, .height_px = HEIGHT, .num_planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size_bytes = WIDTH * HEIGHT * 3, .x_resolution_ppm = 2835, .y_resolution_ppm = 2835, .num_colors = 0, .important_colors = 0 }; uint8_t* image = calloc(WIDTH * HEIGHT * 3, 1); // Draw stripes for (int y = 0; y < HEIGHT; y++) { uint8_t r, g, b; if (y % 74 < 37) { r = 178; g = 34; b = 52; // Red stripe } else { r = 255; g = 255; b = 255; // White stripe } for (int x = 0; x < WIDTH; x++) { set_pixel(image, x, y, r, g, b); } } // Draw blue canton for (int y = HEIGHT - 1; y >= HEIGHT - 259; y--) { for (int x = 0; x < 364; x++) { set_pixel(image, x, y, 60, 59, 110); } } // Draw stars (simplified as white dots) for (int row = 0; row < 9; row++) { for (int col = 0; col < 11; col++) { if (row % 2 == 0 && col == 10) continue; int x = 16 + col * 30; int y = HEIGHT - 14 - row * 28; for (int dy = -2; dy <= 2; dy++) { for (int dx = -2; dx <= 2; dx++) { set_pixel(image, x + dx, y + dy, 255, 255, 255); } } } } // Write BMP header and image data to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(image, WIDTH * HEIGHT * 3, 1, stdout); free(image); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 912 #define HEIGHT 480 // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width_px; int32_t height_px; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size_bytes; int32_t x_resolution_ppm; int32_t y_resolution_ppm; uint32_t num_colors; uint32_t important_colors; } BMPHeader; #pragma pack(pop) // Function to set a pixel color void set_pixel(uint8_t* image, int x, int y, uint8_t r, uint8_t g, uint8_t b) { int index = (y * WIDTH + x) * 3; image[index] = b; image[index + 1] = g; image[index + 2] = r; } int main() { BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3, .offset = sizeof(BMPHeader), .dib_header_size = 40, .width_px = WIDTH, .height_px = HEIGHT, .num_planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size_bytes = WIDTH * HEIGHT * 3, .x_resolution_ppm = 2835, .y_resolution_ppm = 2835, .num_colors = 0, .important_colors = 0 }; uint8_t* image = calloc(WIDTH * HEIGHT * 3, 1); // Draw stripes for (int y = 0; y < HEIGHT; y++) { uint8_t r, g, b; if (y % 74 < 37) { r = 178; g = 34; b = 52; // Red stripe } else { r = 255; g = 255; b = 255; // White stripe } for (int x = 0; x < WIDTH; x++) { set_pixel(image, x, y, r, g, b); } } // Draw blue canton for (int y = HEIGHT - 1; y >= HEIGHT - 259; y--) { for (int x = 0; x < 364; x++) { set_pixel(image, x, y, 60, 59, 110); } } // Draw stars (simplified as white dots) for (int row = 0; row < 9; row++) { for (int col = 0; col < 11; col++) { if (row % 2 == 0 && col == 10) continue; int x = 16 + col * 30; int y = HEIGHT - 14 - row * 28; for (int dy = -2; dy <= 2; dy++) { for (int dx = -2; dx <= 2; dx++) { set_pixel(image, x + dx, y + dy, 255, 255, 255); } } } } // Write BMP header and image data to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(image, WIDTH * HEIGHT * 3, 1, stdout); free(image); return 0; } |
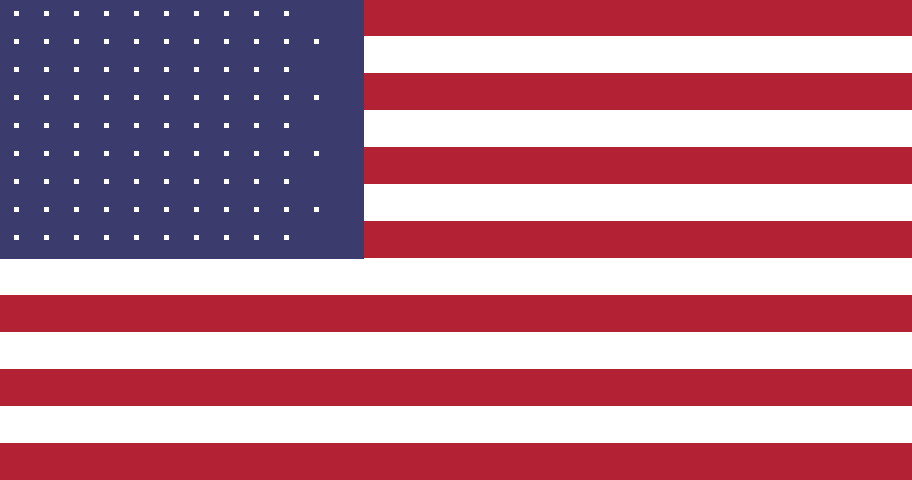
What flag is shown in this image?
The flag shown in the image is a modified version of the flag of the United States. The traditional flag of the United States features 50 stars in the blue field, representing the 50 states, arranged in nine rows of alternating five and six stars. The flag in the image, however, has a different arrangement of stars.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a simple representation of the American flag as a BMP file and outputs it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 600 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // BMP info header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } __attribute__((packed)) BMPInfoHeader; // RGB color typedef struct { uint8_t b; uint8_t g; uint8_t r; } __attribute__((packed)) RGB; // Function to set pixel color void setPixel(RGB *image, int x, int y, RGB color) { image[y * WIDTH + x] = color; } int main() { BMPHeader header = {0x4D42, 54 + 3 * WIDTH * HEIGHT, 0, 0, 54}; BMPInfoHeader infoHeader = {40, WIDTH, HEIGHT, 1, 24, 0, 0, 2835, 2835, 0, 0}; RGB *image = calloc(WIDTH * HEIGHT, sizeof(RGB)); RGB red = {0, 0, 178}; RGB white = {255, 255, 255}; RGB blue = {178, 0, 0}; // Draw stripes for (int y = 0; y < HEIGHT; y++) { RGB color = (y / (HEIGHT / 13)) % 2 == 0 ? red : white; for (int x = 0; x < WIDTH; x++) { setPixel(image, x, y, color); } } // Draw blue rectangle for (int y = 0; y < HEIGHT * 7 / 13; y++) { for (int x = 0; x < WIDTH * 2 / 5; x++) { setPixel(image, x, y, blue); } } // Draw stars (simplified as white dots) for (int row = 0; row < 9; row++) { for (int col = 0; col < 11; col++) { if ((row % 2 == 0 && col % 2 == 0) || (row % 2 == 1 && col % 2 == 1)) { int x = col * (WIDTH * 2 / 5) / 11 + (WIDTH * 2 / 5) / 22; int y = row * (HEIGHT * 7 / 13) / 9 + (HEIGHT * 7 / 13) / 18; setPixel(image, x, y, white); } } } // Write BMP headers and image data to stdout fwrite(&header, sizeof(BMPHeader), 1, stdout); fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, stdout); fwrite(image, sizeof(RGB), WIDTH * HEIGHT, stdout); free(image); return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 600 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // BMP info header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } __attribute__((packed)) BMPInfoHeader; // RGB color typedef struct { uint8_t b; uint8_t g; uint8_t r; } __attribute__((packed)) RGB; // Function to set pixel color void setPixel(RGB *image, int x, int y, RGB color) { image[y * WIDTH + x] = color; } int main() { BMPHeader header = {0x4D42, 54 + 3 * WIDTH * HEIGHT, 0, 0, 54}; BMPInfoHeader infoHeader = {40, WIDTH, HEIGHT, 1, 24, 0, 0, 2835, 2835, 0, 0}; RGB *image = calloc(WIDTH * HEIGHT, sizeof(RGB)); RGB red = {0, 0, 178}; RGB white = {255, 255, 255}; RGB blue = {178, 0, 0}; // Draw stripes for (int y = 0; y < HEIGHT; y++) { RGB color = (y / (HEIGHT / 13)) % 2 == 0 ? red : white; for (int x = 0; x < WIDTH; x++) { setPixel(image, x, y, color); } } // Draw blue rectangle for (int y = 0; y < HEIGHT * 7 / 13; y++) { for (int x = 0; x < WIDTH * 2 / 5; x++) { setPixel(image, x, y, blue); } } // Draw stars (simplified as white dots) for (int row = 0; row < 9; row++) { for (int col = 0; col < 11; col++) { if ((row % 2 == 0 && col % 2 == 0) || (row % 2 == 1 && col % 2 == 1)) { int x = col * (WIDTH * 2 / 5) / 11 + (WIDTH * 2 / 5) / 22; int y = row * (HEIGHT * 7 / 13) / 9 + (HEIGHT * 7 / 13) / 18; setPixel(image, x, y, white); } } } // Write BMP headers and image data to stdout fwrite(&header, sizeof(BMPHeader), 1, stdout); fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, stdout); fwrite(image, sizeof(RGB), WIDTH * HEIGHT, stdout); free(image); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 900 #define HEIGHT 600 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // BMP info header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } __attribute__((packed)) BMPInfoHeader; // RGB color typedef struct { uint8_t b; uint8_t g; uint8_t r; } __attribute__((packed)) RGB; // Function to set pixel color void setPixel(RGB *image, int x, int y, RGB color) { image[y * WIDTH + x] = color; } int main() { BMPHeader header = {0x4D42, 54 + 3 * WIDTH * HEIGHT, 0, 0, 54}; BMPInfoHeader infoHeader = {40, WIDTH, HEIGHT, 1, 24, 0, 0, 2835, 2835, 0, 0}; RGB *image = calloc(WIDTH * HEIGHT, sizeof(RGB)); RGB red = {0, 0, 178}; RGB white = {255, 255, 255}; RGB blue = {178, 0, 0}; // Draw stripes for (int y = 0; y < HEIGHT; y++) { RGB color = (y / (HEIGHT / 13)) % 2 == 0 ? red : white; for (int x = 0; x < WIDTH; x++) { setPixel(image, x, y, color); } } // Draw blue rectangle for (int y = 0; y < HEIGHT * 7 / 13; y++) { for (int x = 0; x < WIDTH * 2 / 5; x++) { setPixel(image, x, y, blue); } } // Draw stars (simplified as white dots) for (int row = 0; row < 9; row++) { for (int col = 0; col < 11; col++) { if ((row % 2 == 0 && col % 2 == 0) || (row % 2 == 1 && col % 2 == 1)) { int x = col * (WIDTH * 2 / 5) / 11 + (WIDTH * 2 / 5) / 22; int y = row * (HEIGHT * 7 / 13) / 9 + (HEIGHT * 7 / 13) / 18; setPixel(image, x, y, white); } } } // Write BMP headers and image data to stdout fwrite(&header, sizeof(BMPHeader), 1, stdout); fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, stdout); fwrite(image, sizeof(RGB), WIDTH * HEIGHT, stdout); free(image); return 0; } |
b"BMV\xb8\x18\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\x84\x03\x00\x00X\x02\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00\x00\x00\x00\x00\x13\x0b\x00\x00\x13\x0b\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00\xb2\x00\x00
What flag is shown in this image?
The image shows an upside-down version of the flag of the United States. The flag consists of 13 horizontal stripes alternating red and white, with a blue rectangle in the canton (top left corner) containing 50 small, white, five-pointed stars arranged in nine offset horizontal rows. In this image, the flag is displayed upside down, which is a signal of distress.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True