Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a bitmap (BMP) image of the American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 | #include <stdio.h> #include <stdint.h> // Color definitions (RGB) #define RED (uint8_t[]){0, 0, 255} #define WHITE (uint8_t[]){255, 255, 255} #define BLUE (uint8_t[]){128, 0, 0} // Flag dimensions #define WIDTH 250 #define HEIGHT 150 #define STRIPES 13 #define STARS_X 10 #define STARS_Y 5 // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; int32_t x_pixels_per_meter; int32_t y_pixels_per_meter; uint32_t colors_used; uint32_t colors_important; } BMPInfoHeader; #pragma pack(pop) // Function to write a pixel void write_pixel(uint8_t* color) { fwrite(color, 1, 3, stdout); } // Function to draw the flag void draw_flag() { // Stripe height int stripe_height = HEIGHT / STRIPES; // Draw stripes for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { uint8_t* color; // Red and white stripes if ((y / stripe_height) % 2 == 0) { color = RED; } else { color = WHITE; } // Blue canton (star field) if (x < WIDTH * 0.4 && y < HEIGHT * (2.0/3.0)) { color = BLUE; // Draw stars int star_width = (WIDTH * 0.4) / STARS_X; int star_height = (HEIGHT * 2.0/3.0) / STARS_Y; if (x % star_width < star_width/2 && y % star_height < star_height/2) { color = WHITE; } } write_pixel(color); } } } int main() { // Calculate padding int padding = (4 - (WIDTH * 3) % 4) % 4; // BMP headers BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (WIDTH * 3 + padding) * HEIGHT, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bits_per_pixel = 24, .compression = 0 }; // Write headers fwrite(&header, sizeof(BMPHeader), 1, stdout); fwrite(&info, sizeof(BMPInfoHeader), 1, stdout); // Draw flag draw_flag(); return 0; } |
1 2 | gcc flag.c -o flag ./flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 | #include <stdio.h> #include <stdint.h> // Color definitions (RGB) #define RED (uint8_t[]){0, 0, 255} #define WHITE (uint8_t[]){255, 255, 255} #define BLUE (uint8_t[]){128, 0, 0} // Flag dimensions #define WIDTH 250 #define HEIGHT 150 #define STRIPES 13 #define STARS_X 10 #define STARS_Y 5 // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; int32_t x_pixels_per_meter; int32_t y_pixels_per_meter; uint32_t colors_used; uint32_t colors_important; } BMPInfoHeader; #pragma pack(pop) // Function to write a pixel void write_pixel(uint8_t* color) { fwrite(color, 1, 3, stdout); } // Function to draw the flag void draw_flag() { // Stripe height int stripe_height = HEIGHT / STRIPES; // Draw stripes for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { uint8_t* color; // Red and white stripes if ((y / stripe_height) % 2 == 0) { color = RED; } else { color = WHITE; } // Blue canton (star field) if (x < WIDTH * 0.4 && y < HEIGHT * (2.0/3.0)) { color = BLUE; // Draw stars int star_width = (WIDTH * 0.4) / STARS_X; int star_height = (HEIGHT * 2.0/3.0) / STARS_Y; if (x % star_width < star_width/2 && y % star_height < star_height/2) { color = WHITE; } } write_pixel(color); } } } int main() { // Calculate padding int padding = (4 - (WIDTH * 3) % 4) % 4; // BMP headers BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (WIDTH * 3 + padding) * HEIGHT, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bits_per_pixel = 24, .compression = 0 }; // Write headers fwrite(&header, sizeof(BMPHeader), 1, stdout); fwrite(&info, sizeof(BMPInfoHeader), 1, stdout); // Draw flag draw_flag(); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 | #include <stdio.h> #include <stdint.h> // Color definitions (RGB) #define RED (uint8_t[]){0, 0, 255} #define WHITE (uint8_t[]){255, 255, 255} #define BLUE (uint8_t[]){128, 0, 0} // Flag dimensions #define WIDTH 250 #define HEIGHT 150 #define STRIPES 13 #define STARS_X 10 #define STARS_Y 5 // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; int32_t x_pixels_per_meter; int32_t y_pixels_per_meter; uint32_t colors_used; uint32_t colors_important; } BMPInfoHeader; #pragma pack(pop) // Function to write a pixel void write_pixel(uint8_t* color) { fwrite(color, 1, 3, stdout); } // Function to draw the flag void draw_flag() { // Stripe height int stripe_height = HEIGHT / STRIPES; // Draw stripes for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { uint8_t* color; // Red and white stripes if ((y / stripe_height) % 2 == 0) { color = RED; } else { color = WHITE; } // Blue canton (star field) if (x < WIDTH * 0.4 && y < HEIGHT * (2.0/3.0)) { color = BLUE; // Draw stars int star_width = (WIDTH * 0.4) / STARS_X; int star_height = (HEIGHT * 2.0/3.0) / STARS_Y; if (x % star_width < star_width/2 && y % star_height < star_height/2) { color = WHITE; } } write_pixel(color); } } } int main() { // Calculate padding int padding = (4 - (WIDTH * 3) % 4) % 4; // BMP headers BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (WIDTH * 3 + padding) * HEIGHT, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bits_per_pixel = 24, .compression = 0 }; // Write headers fwrite(&header, sizeof(BMPHeader), 1, stdout); fwrite(&info, sizeof(BMPInfoHeader), 1, stdout); // Draw flag draw_flag(); return 0; } |
b'BM\xd6\xb8\x01\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\xfa\x00\x00\x00\x96\x00\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x80\x00\x00\x
What flag is shown in this image?
image file is truncated (452 bytes not processed)
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a bitmap (BMP) image of the American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 | #include <stdio.h> #include <stdint.h> // Color definitions (RGB) #define RED (uint32_t)0xFF0000 #define WHITE (uint32_t)0xFFFFFF #define BLUE (uint32_t)0x0000FF // Flag dimensions #define FLAG_WIDTH 250 #define FLAG_HEIGHT 150 #define STRIPE_HEIGHT (FLAG_HEIGHT / 13) void write_bmp_header(int width, int height) { // BMP Header uint16_t bfType = 0x4D42; // "BM" uint32_t bfSize = 54 + width * height * 3; uint16_t bfReserved1 = 0; uint16_t bfReserved2 = 0; uint32_t bfOffBits = 54; // Image Header uint32_t biSize = 40; uint32_t biWidth = width; uint32_t biHeight = height; uint16_t biPlanes = 1; uint16_t biBitCount = 24; uint32_t biCompression = 0; uint32_t biSizeImage = width * height * 3; uint32_t biXPelsPerMeter = 0; uint32_t biYPelsPerMeter = 0; uint32_t biClrUsed = 0; uint32_t biClrImportant = 0; // Write headers fwrite(&bfType, 2, 1, stdout); fwrite(&bfSize, 4, 1, stdout); fwrite(&bfReserved1, 2, 1, stdout); fwrite(&bfReserved2, 2, 1, stdout); fwrite(&bfOffBits, 4, 1, stdout); fwrite(&biSize, 4, 1, stdout); fwrite(&biWidth, 4, 1, stdout); fwrite(&biHeight, 4, 1, stdout); fwrite(&biPlanes, 2, 1, stdout); fwrite(&biBitCount, 2, 1, stdout); fwrite(&biCompression, 4, 1, stdout); fwrite(&biSizeImage, 4, 1, stdout); fwrite(&biXPelsPerMeter, 4, 1, stdout); fwrite(&biYPelsPerMeter, 4, 1, stdout); fwrite(&biClrUsed, 4, 1, stdout); fwrite(&biClrImportant, 4, 1, stdout); } void write_pixel(uint32_t color) { uint8_t r = (color >> 16) & 0xFF; uint8_t g = (color >> 8) & 0xFF; uint8_t b = color & 0xFF; fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } int main() { write_bmp_header(FLAG_WIDTH, FLAG_HEIGHT); // Draw flag for (int y = 0; y < FLAG_HEIGHT; y++) { int stripe = y / STRIPE_HEIGHT; uint32_t stripe_color = (stripe % 2 == 0) ? WHITE : RED; for (int x = 0; x < FLAG_WIDTH; x++) { // Blue canton (star field) if (x < FLAG_WIDTH / 3 && y >= FLAG_HEIGHT * 7/13) { write_pixel(BLUE); } // Draw stripes else if (x < FLAG_WIDTH) { write_pixel(stripe_color); } } } return 0; } |
1 2 | gcc -o flag flag.c ./flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 | #include <stdio.h> #include <stdint.h> // Color definitions (RGB) #define RED (uint32_t)0xFF0000 #define WHITE (uint32_t)0xFFFFFF #define BLUE (uint32_t)0x0000FF // Flag dimensions #define FLAG_WIDTH 250 #define FLAG_HEIGHT 150 #define STRIPE_HEIGHT (FLAG_HEIGHT / 13) void write_bmp_header(int width, int height) { // BMP Header uint16_t bfType = 0x4D42; // "BM" uint32_t bfSize = 54 + width * height * 3; uint16_t bfReserved1 = 0; uint16_t bfReserved2 = 0; uint32_t bfOffBits = 54; // Image Header uint32_t biSize = 40; uint32_t biWidth = width; uint32_t biHeight = height; uint16_t biPlanes = 1; uint16_t biBitCount = 24; uint32_t biCompression = 0; uint32_t biSizeImage = width * height * 3; uint32_t biXPelsPerMeter = 0; uint32_t biYPelsPerMeter = 0; uint32_t biClrUsed = 0; uint32_t biClrImportant = 0; // Write headers fwrite(&bfType, 2, 1, stdout); fwrite(&bfSize, 4, 1, stdout); fwrite(&bfReserved1, 2, 1, stdout); fwrite(&bfReserved2, 2, 1, stdout); fwrite(&bfOffBits, 4, 1, stdout); fwrite(&biSize, 4, 1, stdout); fwrite(&biWidth, 4, 1, stdout); fwrite(&biHeight, 4, 1, stdout); fwrite(&biPlanes, 2, 1, stdout); fwrite(&biBitCount, 2, 1, stdout); fwrite(&biCompression, 4, 1, stdout); fwrite(&biSizeImage, 4, 1, stdout); fwrite(&biXPelsPerMeter, 4, 1, stdout); fwrite(&biYPelsPerMeter, 4, 1, stdout); fwrite(&biClrUsed, 4, 1, stdout); fwrite(&biClrImportant, 4, 1, stdout); } void write_pixel(uint32_t color) { uint8_t r = (color >> 16) & 0xFF; uint8_t g = (color >> 8) & 0xFF; uint8_t b = color & 0xFF; fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } int main() { write_bmp_header(FLAG_WIDTH, FLAG_HEIGHT); // Draw flag for (int y = 0; y < FLAG_HEIGHT; y++) { int stripe = y / STRIPE_HEIGHT; uint32_t stripe_color = (stripe % 2 == 0) ? WHITE : RED; for (int x = 0; x < FLAG_WIDTH; x++) { // Blue canton (star field) if (x < FLAG_WIDTH / 3 && y >= FLAG_HEIGHT * 7/13) { write_pixel(BLUE); } // Draw stripes else if (x < FLAG_WIDTH) { write_pixel(stripe_color); } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 | #include <stdio.h> #include <stdint.h> // Color definitions (RGB) #define RED (uint32_t)0xFF0000 #define WHITE (uint32_t)0xFFFFFF #define BLUE (uint32_t)0x0000FF // Flag dimensions #define FLAG_WIDTH 250 #define FLAG_HEIGHT 150 #define STRIPE_HEIGHT (FLAG_HEIGHT / 13) void write_bmp_header(int width, int height) { // BMP Header uint16_t bfType = 0x4D42; // "BM" uint32_t bfSize = 54 + width * height * 3; uint16_t bfReserved1 = 0; uint16_t bfReserved2 = 0; uint32_t bfOffBits = 54; // Image Header uint32_t biSize = 40; uint32_t biWidth = width; uint32_t biHeight = height; uint16_t biPlanes = 1; uint16_t biBitCount = 24; uint32_t biCompression = 0; uint32_t biSizeImage = width * height * 3; uint32_t biXPelsPerMeter = 0; uint32_t biYPelsPerMeter = 0; uint32_t biClrUsed = 0; uint32_t biClrImportant = 0; // Write headers fwrite(&bfType, 2, 1, stdout); fwrite(&bfSize, 4, 1, stdout); fwrite(&bfReserved1, 2, 1, stdout); fwrite(&bfReserved2, 2, 1, stdout); fwrite(&bfOffBits, 4, 1, stdout); fwrite(&biSize, 4, 1, stdout); fwrite(&biWidth, 4, 1, stdout); fwrite(&biHeight, 4, 1, stdout); fwrite(&biPlanes, 2, 1, stdout); fwrite(&biBitCount, 2, 1, stdout); fwrite(&biCompression, 4, 1, stdout); fwrite(&biSizeImage, 4, 1, stdout); fwrite(&biXPelsPerMeter, 4, 1, stdout); fwrite(&biYPelsPerMeter, 4, 1, stdout); fwrite(&biClrUsed, 4, 1, stdout); fwrite(&biClrImportant, 4, 1, stdout); } void write_pixel(uint32_t color) { uint8_t r = (color >> 16) & 0xFF; uint8_t g = (color >> 8) & 0xFF; uint8_t b = color & 0xFF; fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } int main() { write_bmp_header(FLAG_WIDTH, FLAG_HEIGHT); // Draw flag for (int y = 0; y < FLAG_HEIGHT; y++) { int stripe = y / STRIPE_HEIGHT; uint32_t stripe_color = (stripe % 2 == 0) ? WHITE : RED; for (int x = 0; x < FLAG_WIDTH; x++) { // Blue canton (star field) if (x < FLAG_WIDTH / 3 && y >= FLAG_HEIGHT * 7/13) { write_pixel(BLUE); } // Draw stripes else if (x < FLAG_WIDTH) { write_pixel(stripe_color); } } } return 0; } |
b'BM\xaa\xb7\x01\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\xfa\x00\x00\x00\x96\x00\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00t\xb7\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\
What flag is shown in this image?
image file is truncated (452 bytes not processed)
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a BMP image of the American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 | #include <stdio.h> #include <stdint.h> // Bitmap file header structure typedef struct { uint16_t type; // Magic identifier uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data } __attribute__((packed)) BMPHeader; // DIB header structure typedef struct { uint32_t dib_header_size; // DIB header size int32_t width; // Width of image int32_t height; // Height of image uint16_t planes; // Color planes uint16_t bits_per_pixel; // Bits per pixel uint32_t compression; // Compression type uint32_t image_size; // Image size in bytes int32_t x_pixels_per_meter; // X pixels per meter int32_t y_pixels_per_meter; // Y pixels per meter uint32_t colors_used; // Number of colors uint32_t important_colors; // Important colors } __attribute__((packed)) DIBHeader; // Flag dimensions #define FLAG_WIDTH 250 #define FLAG_HEIGHT 150 #define STRIPE_HEIGHT (FLAG_HEIGHT / 13) // Colors #define RED 0xFF0000 #define WHITE 0xFFFFFF #define BLUE 0x0000FF void write_pixel(uint32_t color) { uint8_t b = color & 0xFF; uint8_t g = (color >> 8) & 0xFF; uint8_t r = (color >> 16) & 0xFF; putchar(b); putchar(g); putchar(r); } int main() { // Calculate padding int padding = (4 - (FLAG_WIDTH * 3) % 4) % 4; // Total file size uint32_t file_size = sizeof(BMPHeader) + sizeof(DIBHeader) + (FLAG_WIDTH * FLAG_HEIGHT * 3) + (padding * FLAG_HEIGHT); // Create BMP header BMPHeader bmp_header = { .type = 0x4D42, // 'BM' .size = file_size, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(DIBHeader) }; // Create DIB header DIBHeader dib_header = { .dib_header_size = sizeof(DIBHeader), .width = FLAG_WIDTH, .height = FLAG_HEIGHT, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = 0, .x_pixels_per_meter = 0, .y_pixels_per_meter = 0, .colors_used = 0, .important_colors = 0 }; // Write headers fwrite(&bmp_header, sizeof(BMPHeader), 1, stdout); fwrite(&dib_header, sizeof(DIBHeader), 1, stdout); // Draw flag for (int y = 0; y < FLAG_HEIGHT; y++) { for (int x = 0; x < FLAG_WIDTH; x++) { // Blue canton if (x < FLAG_WIDTH * 0.4 && y >= FLAG_HEIGHT * 0.5) { write_pixel(BLUE); continue; } // Stripes int stripe = y / STRIPE_HEIGHT; if (stripe % 2 == 0) { write_pixel(RED); } else { write_pixel(WHITE); } } // Add padding for (int p = 0; p < padding; p++) { putchar(0); } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 | #include <stdio.h> #include <stdint.h> // Bitmap file header structure typedef struct { uint16_t type; // Magic identifier uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data } __attribute__((packed)) BMPHeader; // DIB header structure typedef struct { uint32_t dib_header_size; // DIB header size int32_t width; // Width of image int32_t height; // Height of image uint16_t planes; // Color planes uint16_t bits_per_pixel; // Bits per pixel uint32_t compression; // Compression type uint32_t image_size; // Image size in bytes int32_t x_pixels_per_meter; // X pixels per meter int32_t y_pixels_per_meter; // Y pixels per meter uint32_t colors_used; // Number of colors uint32_t important_colors; // Important colors } __attribute__((packed)) DIBHeader; // Flag dimensions #define FLAG_WIDTH 250 #define FLAG_HEIGHT 150 #define STRIPE_HEIGHT (FLAG_HEIGHT / 13) // Colors #define RED 0xFF0000 #define WHITE 0xFFFFFF #define BLUE 0x0000FF void write_pixel(uint32_t color) { uint8_t b = color & 0xFF; uint8_t g = (color >> 8) & 0xFF; uint8_t r = (color >> 16) & 0xFF; putchar(b); putchar(g); putchar(r); } int main() { // Calculate padding int padding = (4 - (FLAG_WIDTH * 3) % 4) % 4; // Total file size uint32_t file_size = sizeof(BMPHeader) + sizeof(DIBHeader) + (FLAG_WIDTH * FLAG_HEIGHT * 3) + (padding * FLAG_HEIGHT); // Create BMP header BMPHeader bmp_header = { .type = 0x4D42, // 'BM' .size = file_size, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(DIBHeader) }; // Create DIB header DIBHeader dib_header = { .dib_header_size = sizeof(DIBHeader), .width = FLAG_WIDTH, .height = FLAG_HEIGHT, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = 0, .x_pixels_per_meter = 0, .y_pixels_per_meter = 0, .colors_used = 0, .important_colors = 0 }; // Write headers fwrite(&bmp_header, sizeof(BMPHeader), 1, stdout); fwrite(&dib_header, sizeof(DIBHeader), 1, stdout); // Draw flag for (int y = 0; y < FLAG_HEIGHT; y++) { for (int x = 0; x < FLAG_WIDTH; x++) { // Blue canton if (x < FLAG_WIDTH * 0.4 && y >= FLAG_HEIGHT * 0.5) { write_pixel(BLUE); continue; } // Stripes int stripe = y / STRIPE_HEIGHT; if (stripe % 2 == 0) { write_pixel(RED); } else { write_pixel(WHITE); } } // Add padding for (int p = 0; p < padding; p++) { putchar(0); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 | #include <stdio.h> #include <stdint.h> // Bitmap file header structure typedef struct { uint16_t type; // Magic identifier uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data } __attribute__((packed)) BMPHeader; // DIB header structure typedef struct { uint32_t dib_header_size; // DIB header size int32_t width; // Width of image int32_t height; // Height of image uint16_t planes; // Color planes uint16_t bits_per_pixel; // Bits per pixel uint32_t compression; // Compression type uint32_t image_size; // Image size in bytes int32_t x_pixels_per_meter; // X pixels per meter int32_t y_pixels_per_meter; // Y pixels per meter uint32_t colors_used; // Number of colors uint32_t important_colors; // Important colors } __attribute__((packed)) DIBHeader; // Flag dimensions #define FLAG_WIDTH 250 #define FLAG_HEIGHT 150 #define STRIPE_HEIGHT (FLAG_HEIGHT / 13) // Colors #define RED 0xFF0000 #define WHITE 0xFFFFFF #define BLUE 0x0000FF void write_pixel(uint32_t color) { uint8_t b = color & 0xFF; uint8_t g = (color >> 8) & 0xFF; uint8_t r = (color >> 16) & 0xFF; putchar(b); putchar(g); putchar(r); } int main() { // Calculate padding int padding = (4 - (FLAG_WIDTH * 3) % 4) % 4; // Total file size uint32_t file_size = sizeof(BMPHeader) + sizeof(DIBHeader) + (FLAG_WIDTH * FLAG_HEIGHT * 3) + (padding * FLAG_HEIGHT); // Create BMP header BMPHeader bmp_header = { .type = 0x4D42, // 'BM' .size = file_size, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(DIBHeader) }; // Create DIB header DIBHeader dib_header = { .dib_header_size = sizeof(DIBHeader), .width = FLAG_WIDTH, .height = FLAG_HEIGHT, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = 0, .x_pixels_per_meter = 0, .y_pixels_per_meter = 0, .colors_used = 0, .important_colors = 0 }; // Write headers fwrite(&bmp_header, sizeof(BMPHeader), 1, stdout); fwrite(&dib_header, sizeof(DIBHeader), 1, stdout); // Draw flag for (int y = 0; y < FLAG_HEIGHT; y++) { for (int x = 0; x < FLAG_WIDTH; x++) { // Blue canton if (x < FLAG_WIDTH * 0.4 && y >= FLAG_HEIGHT * 0.5) { write_pixel(BLUE); continue; } // Stripes int stripe = y / STRIPE_HEIGHT; if (stripe % 2 == 0) { write_pixel(RED); } else { write_pixel(WHITE); } } // Add padding for (int p = 0; p < padding; p++) { putchar(0); } } return 0; } |
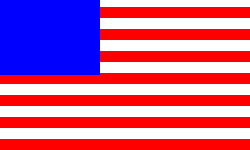
What flag is shown in this image?
This is the Grand Union Flag, also known as the Continental Colors. It was the first national flag of the United States, used from 1775 to 1777.
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: True
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a bitmap (BMP) image of the American flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdint.h> // Color definitions (in BGR format for BMP) #define RED (uint32_t)0x0000FF #define WHITE (uint32_t)0xFFFFFF #define BLUE (uint32_t)0x800000 #define WIDTH 250 #define HEIGHT 150 // BMP Header structures typedef struct { uint16_t type; // Magic identifier uint32_t size; // File size in bytes uint16_t reserved1; uint16_t reserved2; uint32_t offset; // Offset to image data } __attribute__((packed)) BMPHeader; typedef struct { uint32_t size; // Header size int32_t width; // Image width int32_t height; // Image height uint16_t planes; // Color planes uint16_t bits; // Bits per pixel uint32_t compression; // Compression type uint32_t imagesize; // Image size in bytes int32_t xresolution; // Pixels per meter (X) int32_t yresolution; // Pixels per meter (Y) uint32_t ncolors; // Number of colors uint32_t importantcolors; // Important colors } __attribute__((packed)) BMPInfoHeader; void write_pixel(uint32_t color) { fputc((color & 0xFF), stdout); // Blue fputc(((color >> 8) & 0xFF), stdout); // Green fputc(((color >> 16) & 0xFF), stdout);// Red } int main() { BMPHeader bmpHeader = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (WIDTH * HEIGHT * 3), .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader bmpInfoHeader = { .size = sizeof(BMPInfoHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bits = 24, .compression = 0, .imagesize = WIDTH * HEIGHT * 3, .xresolution = 0, .yresolution = 0, .ncolors = 0, .importantcolors = 0 }; // Write headers fwrite(&bmpHeader, sizeof(BMPHeader), 1, stdout); fwrite(&bmpInfoHeader, sizeof(BMPInfoHeader), 1, stdout); // Draw flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { // Blue canton (stars) if (x < WIDTH * 0.4 && y > HEIGHT * 0.6) { if ((x % 2 == 0 && y % 2 == 0) || (x % 2 == 1 && y % 2 == 1)) { write_pixel(WHITE); // Stars } else { write_pixel(BLUE); // Blue background } } // Red and white stripes else { int stripe = y / (HEIGHT / 13); if (stripe % 2 == 0) { write_pixel(RED); // Red stripes } else { write_pixel(WHITE); // White stripes } } } } return 0; } |
1 2 | gcc flag.c -o flag ./flag > american_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdint.h> // Color definitions (in BGR format for BMP) #define RED (uint32_t)0x0000FF #define WHITE (uint32_t)0xFFFFFF #define BLUE (uint32_t)0x800000 #define WIDTH 250 #define HEIGHT 150 // BMP Header structures typedef struct { uint16_t type; // Magic identifier uint32_t size; // File size in bytes uint16_t reserved1; uint16_t reserved2; uint32_t offset; // Offset to image data } __attribute__((packed)) BMPHeader; typedef struct { uint32_t size; // Header size int32_t width; // Image width int32_t height; // Image height uint16_t planes; // Color planes uint16_t bits; // Bits per pixel uint32_t compression; // Compression type uint32_t imagesize; // Image size in bytes int32_t xresolution; // Pixels per meter (X) int32_t yresolution; // Pixels per meter (Y) uint32_t ncolors; // Number of colors uint32_t importantcolors; // Important colors } __attribute__((packed)) BMPInfoHeader; void write_pixel(uint32_t color) { fputc((color & 0xFF), stdout); // Blue fputc(((color >> 8) & 0xFF), stdout); // Green fputc(((color >> 16) & 0xFF), stdout);// Red } int main() { BMPHeader bmpHeader = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (WIDTH * HEIGHT * 3), .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader bmpInfoHeader = { .size = sizeof(BMPInfoHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bits = 24, .compression = 0, .imagesize = WIDTH * HEIGHT * 3, .xresolution = 0, .yresolution = 0, .ncolors = 0, .importantcolors = 0 }; // Write headers fwrite(&bmpHeader, sizeof(BMPHeader), 1, stdout); fwrite(&bmpInfoHeader, sizeof(BMPInfoHeader), 1, stdout); // Draw flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { // Blue canton (stars) if (x < WIDTH * 0.4 && y > HEIGHT * 0.6) { if ((x % 2 == 0 && y % 2 == 0) || (x % 2 == 1 && y % 2 == 1)) { write_pixel(WHITE); // Stars } else { write_pixel(BLUE); // Blue background } } // Red and white stripes else { int stripe = y / (HEIGHT / 13); if (stripe % 2 == 0) { write_pixel(RED); // Red stripes } else { write_pixel(WHITE); // White stripes } } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdint.h> // Color definitions (in BGR format for BMP) #define RED (uint32_t)0x0000FF #define WHITE (uint32_t)0xFFFFFF #define BLUE (uint32_t)0x800000 #define WIDTH 250 #define HEIGHT 150 // BMP Header structures typedef struct { uint16_t type; // Magic identifier uint32_t size; // File size in bytes uint16_t reserved1; uint16_t reserved2; uint32_t offset; // Offset to image data } __attribute__((packed)) BMPHeader; typedef struct { uint32_t size; // Header size int32_t width; // Image width int32_t height; // Image height uint16_t planes; // Color planes uint16_t bits; // Bits per pixel uint32_t compression; // Compression type uint32_t imagesize; // Image size in bytes int32_t xresolution; // Pixels per meter (X) int32_t yresolution; // Pixels per meter (Y) uint32_t ncolors; // Number of colors uint32_t importantcolors; // Important colors } __attribute__((packed)) BMPInfoHeader; void write_pixel(uint32_t color) { fputc((color & 0xFF), stdout); // Blue fputc(((color >> 8) & 0xFF), stdout); // Green fputc(((color >> 16) & 0xFF), stdout);// Red } int main() { BMPHeader bmpHeader = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (WIDTH * HEIGHT * 3), .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader bmpInfoHeader = { .size = sizeof(BMPInfoHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bits = 24, .compression = 0, .imagesize = WIDTH * HEIGHT * 3, .xresolution = 0, .yresolution = 0, .ncolors = 0, .importantcolors = 0 }; // Write headers fwrite(&bmpHeader, sizeof(BMPHeader), 1, stdout); fwrite(&bmpInfoHeader, sizeof(BMPInfoHeader), 1, stdout); // Draw flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { // Blue canton (stars) if (x < WIDTH * 0.4 && y > HEIGHT * 0.6) { if ((x % 2 == 0 && y % 2 == 0) || (x % 2 == 1 && y % 2 == 1)) { write_pixel(WHITE); // Stars } else { write_pixel(BLUE); // Blue background } } // Red and white stripes else { int stripe = y / (HEIGHT / 13); if (stripe % 2 == 0) { write_pixel(RED); // Red stripes } else { write_pixel(WHITE); // White stripes } } } } return 0; } |
b'BM\xaa\xb7\x01\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\xfa\x00\x00\x00\x96\x00\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00t\xb7\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\
What flag is shown in this image?
image file is truncated (452 bytes not processed)
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an american flag to stdout.
Here's a C program that generates a bitmap (BMP) image of the American flag and writes it to standard output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 | #include <stdio.h> #include <stdint.h> // BMP Header structures #pragma pack(push, 1) typedef struct { uint16_t signature; uint32_t filesize; uint16_t reserved1; uint16_t reserved2; uint32_t data_offset; } BMPHeader; typedef struct { uint32_t header_size; int32_t width; int32_t height; uint16_t planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; int32_t x_pixels_per_meter; int32_t y_pixels_per_meter; uint32_t colors_used; uint32_t important_colors; } BMPInfoHeader; #pragma pack(pop) // Flag dimensions #define FLAG_WIDTH 250 #define FLAG_HEIGHT 150 #define STRIPE_HEIGHT (FLAG_HEIGHT / 13) #define UNION_WIDTH (FLAG_WIDTH * 2/5) #define UNION_HEIGHT (STRIPE_HEIGHT * 7) // Colors #define RED (uint8_t[]){0, 0, 255} #define WHITE (uint8_t[]){255, 255, 255} #define BLUE (uint8_t[]){128, 0, 0} void write_pixel(uint8_t* color) { fwrite(color, 1, 3, stdout); } int main() { // Create BMP headers BMPHeader bmp_header = { .signature = 0x4D42, .filesize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (FLAG_WIDTH * FLAG_HEIGHT * 3), .data_offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader bmp_info = { .header_size = sizeof(BMPInfoHeader), .width = FLAG_WIDTH, .height = FLAG_HEIGHT, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = FLAG_WIDTH * FLAG_HEIGHT * 3 }; // Write headers fwrite(&bmp_header, sizeof(BMPHeader), 1, stdout); fwrite(&bmp_info, sizeof(BMPInfoHeader), 1, stdout); // Draw flag for (int y = 0; y < FLAG_HEIGHT; y++) { for (int x = 0; x < FLAG_WIDTH; x++) { // Blue union if (x < UNION_WIDTH && y < UNION_HEIGHT) { write_pixel(BLUE); } // Red and white stripes else { int stripe = y / STRIPE_HEIGHT; if (stripe % 2 == 0) { write_pixel(RED); } else { write_pixel(WHITE); } } } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 | #include <stdio.h> #include <stdint.h> // BMP Header structures #pragma pack(push, 1) typedef struct { uint16_t signature; uint32_t filesize; uint16_t reserved1; uint16_t reserved2; uint32_t data_offset; } BMPHeader; typedef struct { uint32_t header_size; int32_t width; int32_t height; uint16_t planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; int32_t x_pixels_per_meter; int32_t y_pixels_per_meter; uint32_t colors_used; uint32_t important_colors; } BMPInfoHeader; #pragma pack(pop) // Flag dimensions #define FLAG_WIDTH 250 #define FLAG_HEIGHT 150 #define STRIPE_HEIGHT (FLAG_HEIGHT / 13) #define UNION_WIDTH (FLAG_WIDTH * 2/5) #define UNION_HEIGHT (STRIPE_HEIGHT * 7) // Colors #define RED (uint8_t[]){0, 0, 255} #define WHITE (uint8_t[]){255, 255, 255} #define BLUE (uint8_t[]){128, 0, 0} void write_pixel(uint8_t* color) { fwrite(color, 1, 3, stdout); } int main() { // Create BMP headers BMPHeader bmp_header = { .signature = 0x4D42, .filesize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (FLAG_WIDTH * FLAG_HEIGHT * 3), .data_offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader bmp_info = { .header_size = sizeof(BMPInfoHeader), .width = FLAG_WIDTH, .height = FLAG_HEIGHT, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = FLAG_WIDTH * FLAG_HEIGHT * 3 }; // Write headers fwrite(&bmp_header, sizeof(BMPHeader), 1, stdout); fwrite(&bmp_info, sizeof(BMPInfoHeader), 1, stdout); // Draw flag for (int y = 0; y < FLAG_HEIGHT; y++) { for (int x = 0; x < FLAG_WIDTH; x++) { // Blue union if (x < UNION_WIDTH && y < UNION_HEIGHT) { write_pixel(BLUE); } // Red and white stripes else { int stripe = y / STRIPE_HEIGHT; if (stripe % 2 == 0) { write_pixel(RED); } else { write_pixel(WHITE); } } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 | #include <stdio.h> #include <stdint.h> // BMP Header structures #pragma pack(push, 1) typedef struct { uint16_t signature; uint32_t filesize; uint16_t reserved1; uint16_t reserved2; uint32_t data_offset; } BMPHeader; typedef struct { uint32_t header_size; int32_t width; int32_t height; uint16_t planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; int32_t x_pixels_per_meter; int32_t y_pixels_per_meter; uint32_t colors_used; uint32_t important_colors; } BMPInfoHeader; #pragma pack(pop) // Flag dimensions #define FLAG_WIDTH 250 #define FLAG_HEIGHT 150 #define STRIPE_HEIGHT (FLAG_HEIGHT / 13) #define UNION_WIDTH (FLAG_WIDTH * 2/5) #define UNION_HEIGHT (STRIPE_HEIGHT * 7) // Colors #define RED (uint8_t[]){0, 0, 255} #define WHITE (uint8_t[]){255, 255, 255} #define BLUE (uint8_t[]){128, 0, 0} void write_pixel(uint8_t* color) { fwrite(color, 1, 3, stdout); } int main() { // Create BMP headers BMPHeader bmp_header = { .signature = 0x4D42, .filesize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (FLAG_WIDTH * FLAG_HEIGHT * 3), .data_offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader bmp_info = { .header_size = sizeof(BMPInfoHeader), .width = FLAG_WIDTH, .height = FLAG_HEIGHT, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = FLAG_WIDTH * FLAG_HEIGHT * 3 }; // Write headers fwrite(&bmp_header, sizeof(BMPHeader), 1, stdout); fwrite(&bmp_info, sizeof(BMPInfoHeader), 1, stdout); // Draw flag for (int y = 0; y < FLAG_HEIGHT; y++) { for (int x = 0; x < FLAG_WIDTH; x++) { // Blue union if (x < UNION_WIDTH && y < UNION_HEIGHT) { write_pixel(BLUE); } // Red and white stripes else { int stripe = y / STRIPE_HEIGHT; if (stripe % 2 == 0) { write_pixel(RED); } else { write_pixel(WHITE); } } } } return 0; } |
b'BM\xaa\xb7\x01\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\xfa\x00\x00\x00\x96\x00\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00t\xb7\x01\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\
What flag is shown in this image?
image file is truncated (452 bytes not processed)
Testing if the previous output contains the string America
: False
Testing if the previous output contains the string USA
: False
Testing if the previous output contains the string United States
: False