Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Below is one complete C program that generates a 24‑bit BMP file of an Italian flag (with vertical green, white, and red stripes) and writes it to stdout. You can compile it with a C compiler (for example, using gcc) and redirect its output to a file to obtain the BMP image. Below is the full source code: ------------------------------------------------------------ /* italian_flag.c * * This program creates a 24-bit BMP image of the Italian flag * (three vertical stripes: green, white, red) and writes it to stdout. * * Compile with: * gcc italian_flag.c -o italian_flag * * Run and redirect output: * ./italian_flag > italian_flag.bmp */ #include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> int main(void) { // Image dimensions const int width = 300; // width in pixels. const int height = 200; // height in pixels. // Each pixel uses 3 bytes (B, G, R). BMP rows are padded to a multiple of 4 bytes. int rowBytes = width * 3; // Calculate padding per row (if any) int padding = (4 - (rowBytes % 4)) % 4; int rowSizePadded = rowBytes + padding; // Total file size: header (54 bytes) + pixel data. const int fileHeaderSize = 14; const int dibHeaderSize = 40; int pixelDataSize = rowSizePadded * height; int fileSize = fileHeaderSize + dibHeaderSize + pixelDataSize; // Create and fill the 54-byte BMP header. unsigned char header[54]; memset(header, 0, sizeof(header)); // BMP file header (14 bytes) header[0] = 'B'; header[1] = 'M'; // Write file size (bytes 2-5, little-endian) header[2] = (unsigned char)( fileSize & 0xFF ); header[3] = (unsigned char)((fileSize >> 8) & 0xFF ); header[4] = (unsigned char)((fileSize >> 16) & 0xFF ); header[5] = (unsigned char)((fileSize >> 24) & 0xFF ); // Reserved bytes (6-9) remain zero. // Pixel data offset (start address of pixel array): header size = 54 bytes. header[10] = 54; // little-endian 54 = 0x36, rest are 0. // DIB header (BITMAPINFOHEADER, 40 bytes, from byte 14 to 53) // DIB header size header[14] = 40; // header size = 40 bytes. // Image width (bytes 18-21) header[18] = (unsigned char)( width & 0xFF ); header[19] = (unsigned char)((width >> 8) & 0xFF ); header[20] = (unsigned char)((width >> 16) & 0xFF ); header[21] = (unsigned char)((width >> 24) & 0xFF ); // Image height (bytes 22-25). (Positive height means bottom-up bitmap.) header[22] = (unsigned char)( height & 0xFF ); header[23] = (unsigned char)((height >> 8) & 0xFF ); header[24] = (unsigned char)((height >> 16) & 0xFF ); header[25] = (unsigned char)((height >> 24) & 0xFF ); // Planes (2 bytes, bytes 26-27); must be 1. header[26] = 1; // Bits per pixel (2 bytes, bytes 28-29): 24 for 24-bit bitmap. header[28] = 24; // Compression (bytes 30-33): 0 = no compression. // Image size (bytes 34-37): can be 0 for BI_RGB; however, we fill in our pixelDataSize. header[34] = (unsigned char)( pixelDataSize & 0xFF ); header[35] = (unsigned char)((pixelDataSize >> 8) & 0xFF ); header[36] = (unsigned char)((pixelDataSize >> 16) & 0xFF ); header[37] = (unsigned char)((pixelDataSize >> 24) & 0xFF ); // The rest (resolution and color palette) can be left as 0. // Write header to stdout. if (fwrite(header, 1, sizeof(header), stdout) != sizeof(header)) { fprintf(stderr, "Error writing BMP header.\n"); return EXIT_FAILURE; } // Define colors in BGR order // Italian flag: left stripe green, middle stripe white, right stripe red. // Green: (R,G,B) = (0, 255, 0) => bytes: 0x00, 0xFF, 0x00. // White: (255,255,255) => 0xFF, 0xFF, 0xFF. // Red: (255, 0, 0) => 0x00, 0x00, 0xFF. // For each row, BMP format requires writing from left-to-right, but rows from bottom to top. for (int y = height - 1; y >= 0; y--) { // For each pixel in the row for (int x = 0; x < width; x++) { unsigned char blue, green, red; if (x < width / 3) { // Left third: green stripe. blue = 0x00; green = 0xFF; red = 0x00; } else if (x < 2 * (width / 3)) { // Middle third: white stripe. blue = 0xFF; green = 0xFF; red = 0xFF; } else { // Right third: red stripe. blue = 0x00; green = 0x00; red = 0xFF; } fputc(blue, stdout); fputc(green, stdout); fputc(red, stdout); } // Write any row padding bytes (if needed). for (int p = 0; p < padding; p++) { fputc(0x00, stdout); } } fflush(stdout); return EXIT_SUCCESS; } ------------------------------------------------------------ Explanation: 1. The program sets a fixed image size (300×200 pixels). Adjust width and height as desired. 2. It computes the proper padded row size (each row’s size in bytes is rounded up to be a multiple of 4, though for 300 pixels × 3 bytes = 900 bytes, no extra padding is needed). 3. It builds the BMP file header (14 bytes) and the DIB header (40 bytes) in little‑endian format. 4. It then writes the pixel data starting from the bottom row (BMP’s bottom‑up order). Each pixel is output in BGR order. 5. The left third of each row is painted green, the middle third white, and the right third red. To produce the BMP file, compile and run as follows: gcc italian_flag.c -o italian_flag ./italian_flag > italian_flag.bmp Then view italian_flag.bmp with any image viewer that supports BMP files. Enjoy your Italian flag BMP!
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 | /* italian_flag.c * * This program creates a 24-bit BMP image of the Italian flag * (three vertical stripes: green, white, red) and writes it to stdout. * * Compile with: * gcc italian_flag.c -o italian_flag * * Run and redirect output: * ./italian_flag > italian_flag.bmp */ #include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> int main(void) { // Image dimensions const int width = 300; // width in pixels. const int height = 200; // height in pixels. // Each pixel uses 3 bytes (B, G, R). BMP rows are padded to a multiple of 4 bytes. int rowBytes = width * 3; // Calculate padding per row (if any) int padding = (4 - (rowBytes % 4)) % 4; int rowSizePadded = rowBytes + padding; // Total file size: header (54 bytes) + pixel data. const int fileHeaderSize = 14; const int dibHeaderSize = 40; int pixelDataSize = rowSizePadded * height; int fileSize = fileHeaderSize + dibHeaderSize + pixelDataSize; // Create and fill the 54-byte BMP header. unsigned char header[54]; memset(header, 0, sizeof(header)); // BMP file header (14 bytes) header[0] = 'B'; header[1] = 'M'; // Write file size (bytes 2-5, little-endian) header[2] = (unsigned char)( fileSize & 0xFF ); header[3] = (unsigned char)((fileSize >> 8) & 0xFF ); header[4] = (unsigned char)((fileSize >> 16) & 0xFF ); header[5] = (unsigned char)((fileSize >> 24) & 0xFF ); // Reserved bytes (6-9) remain zero. // Pixel data offset (start address of pixel array): header size = 54 bytes. header[10] = 54; // little-endian 54 = 0x36, rest are 0. // DIB header (BITMAPINFOHEADER, 40 bytes, from byte 14 to 53) // DIB header size header[14] = 40; // header size = 40 bytes. // Image width (bytes 18-21) header[18] = (unsigned char)( width & 0xFF ); header[19] = (unsigned char)((width >> 8) & 0xFF ); header[20] = (unsigned char)((width >> 16) & 0xFF ); header[21] = (unsigned char)((width >> 24) & 0xFF ); // Image height (bytes 22-25). (Positive height means bottom-up bitmap.) header[22] = (unsigned char)( height & 0xFF ); header[23] = (unsigned char)((height >> 8) & 0xFF ); header[24] = (unsigned char)((height >> 16) & 0xFF ); header[25] = (unsigned char)((height >> 24) & 0xFF ); // Planes (2 bytes, bytes 26-27); must be 1. header[26] = 1; // Bits per pixel (2 bytes, bytes 28-29): 24 for 24-bit bitmap. header[28] = 24; // Compression (bytes 30-33): 0 = no compression. // Image size (bytes 34-37): can be 0 for BI_RGB; however, we fill in our pixelDataSize. header[34] = (unsigned char)( pixelDataSize & 0xFF ); header[35] = (unsigned char)((pixelDataSize >> 8) & 0xFF ); header[36] = (unsigned char)((pixelDataSize >> 16) & 0xFF ); header[37] = (unsigned char)((pixelDataSize >> 24) & 0xFF ); // The rest (resolution and color palette) can be left as 0. // Write header to stdout. if (fwrite(header, 1, sizeof(header), stdout) != sizeof(header)) { fprintf(stderr, "Error writing BMP header.\n"); return EXIT_FAILURE; } // Define colors in BGR order // Italian flag: left stripe green, middle stripe white, right stripe red. // Green: (R,G,B) = (0, 255, 0) => bytes: 0x00, 0xFF, 0x00. // White: (255,255,255) => 0xFF, 0xFF, 0xFF. // Red: (255, 0, 0) => 0x00, 0x00, 0xFF. // For each row, BMP format requires writing from left-to-right, but rows from bottom to top. for (int y = height - 1; y >= 0; y--) { // For each pixel in the row for (int x = 0; x < width; x++) { unsigned char blue, green, red; if (x < width / 3) { // Left third: green stripe. blue = 0x00; green = 0xFF; red = 0x00; } else if (x < 2 * (width / 3)) { // Middle third: white stripe. blue = 0xFF; green = 0xFF; red = 0xFF; } else { // Right third: red stripe. blue = 0x00; green = 0x00; red = 0xFF; } fputc(blue, stdout); fputc(green, stdout); fputc(red, stdout); } // Write any row padding bytes (if needed). for (int p = 0; p < padding; p++) { fputc(0x00, stdout); } } fflush(stdout); return EXIT_SUCCESS; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 | /* italian_flag.c * * This program creates a 24-bit BMP image of the Italian flag * (three vertical stripes: green, white, red) and writes it to stdout. * * Compile with: * gcc italian_flag.c -o italian_flag * * Run and redirect output: * ./italian_flag > italian_flag.bmp */ #include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> int main(void) { // Image dimensions const int width = 300; // width in pixels. const int height = 200; // height in pixels. // Each pixel uses 3 bytes (B, G, R). BMP rows are padded to a multiple of 4 bytes. int rowBytes = width * 3; // Calculate padding per row (if any) int padding = (4 - (rowBytes % 4)) % 4; int rowSizePadded = rowBytes + padding; // Total file size: header (54 bytes) + pixel data. const int fileHeaderSize = 14; const int dibHeaderSize = 40; int pixelDataSize = rowSizePadded * height; int fileSize = fileHeaderSize + dibHeaderSize + pixelDataSize; // Create and fill the 54-byte BMP header. unsigned char header[54]; memset(header, 0, sizeof(header)); // BMP file header (14 bytes) header[0] = 'B'; header[1] = 'M'; // Write file size (bytes 2-5, little-endian) header[2] = (unsigned char)( fileSize & 0xFF ); header[3] = (unsigned char)((fileSize >> 8) & 0xFF ); header[4] = (unsigned char)((fileSize >> 16) & 0xFF ); header[5] = (unsigned char)((fileSize >> 24) & 0xFF ); // Reserved bytes (6-9) remain zero. // Pixel data offset (start address of pixel array): header size = 54 bytes. header[10] = 54; // little-endian 54 = 0x36, rest are 0. // DIB header (BITMAPINFOHEADER, 40 bytes, from byte 14 to 53) // DIB header size header[14] = 40; // header size = 40 bytes. // Image width (bytes 18-21) header[18] = (unsigned char)( width & 0xFF ); header[19] = (unsigned char)((width >> 8) & 0xFF ); header[20] = (unsigned char)((width >> 16) & 0xFF ); header[21] = (unsigned char)((width >> 24) & 0xFF ); // Image height (bytes 22-25). (Positive height means bottom-up bitmap.) header[22] = (unsigned char)( height & 0xFF ); header[23] = (unsigned char)((height >> 8) & 0xFF ); header[24] = (unsigned char)((height >> 16) & 0xFF ); header[25] = (unsigned char)((height >> 24) & 0xFF ); // Planes (2 bytes, bytes 26-27); must be 1. header[26] = 1; // Bits per pixel (2 bytes, bytes 28-29): 24 for 24-bit bitmap. header[28] = 24; // Compression (bytes 30-33): 0 = no compression. // Image size (bytes 34-37): can be 0 for BI_RGB; however, we fill in our pixelDataSize. header[34] = (unsigned char)( pixelDataSize & 0xFF ); header[35] = (unsigned char)((pixelDataSize >> 8) & 0xFF ); header[36] = (unsigned char)((pixelDataSize >> 16) & 0xFF ); header[37] = (unsigned char)((pixelDataSize >> 24) & 0xFF ); // The rest (resolution and color palette) can be left as 0. // Write header to stdout. if (fwrite(header, 1, sizeof(header), stdout) != sizeof(header)) { fprintf(stderr, "Error writing BMP header.\n"); return EXIT_FAILURE; } // Define colors in BGR order // Italian flag: left stripe green, middle stripe white, right stripe red. // Green: (R,G,B) = (0, 255, 0) => bytes: 0x00, 0xFF, 0x00. // White: (255,255,255) => 0xFF, 0xFF, 0xFF. // Red: (255, 0, 0) => 0x00, 0x00, 0xFF. // For each row, BMP format requires writing from left-to-right, but rows from bottom to top. for (int y = height - 1; y >= 0; y--) { // For each pixel in the row for (int x = 0; x < width; x++) { unsigned char blue, green, red; if (x < width / 3) { // Left third: green stripe. blue = 0x00; green = 0xFF; red = 0x00; } else if (x < 2 * (width / 3)) { // Middle third: white stripe. blue = 0xFF; green = 0xFF; red = 0xFF; } else { // Right third: red stripe. blue = 0x00; green = 0x00; red = 0xFF; } fputc(blue, stdout); fputc(green, stdout); fputc(red, stdout); } // Write any row padding bytes (if needed). for (int p = 0; p < padding; p++) { fputc(0x00, stdout); } } fflush(stdout); return EXIT_SUCCESS; } |
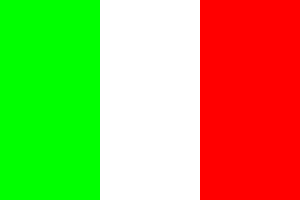
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three vertical stripes of green, white, and red.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Below is one example in C. This program builds a 300×200, 24‐bit BMP image whose three vertical thirds are green, white, and red (left to right) representing the Italian flag. When run the program writes the BMP file (including header and pixel data) to standard output. Note that on some systems you may need to redirect stdout to a file (for example, using “./flag > flag.bmp”) and then open the file in an image viewer. -------------------------------------------------- #include <stdio.h> #include <stdint.h> int main(void) { // Image dimensions const int width = 300; const int height = 200; // Each pixel is 3 bytes (B, G, R). Each row must be padded to a multiple of 4 bytes. // For width=300, row size = 300*3 = 900, which is already a multiple of 4. const int rowSize = width * 3; const int pixelDataSize = rowSize * height; // BMP file header (14 bytes) + DIB header (40 bytes) const int fileHeaderSize = 14; const int infoHeaderSize = 40; const int fileSize = fileHeaderSize + infoHeaderSize + pixelDataSize; // --- Build the BMP file header (14 bytes) --- unsigned char fileHeader[14] = {0}; // Signature "BM" fileHeader[0] = 'B'; fileHeader[1] = 'M'; // File size in little endian fileHeader[2] = (unsigned char)(fileSize & 0xFF); fileHeader[3] = (unsigned char)((fileSize >> 8) & 0xFF); fileHeader[4] = (unsigned char)((fileSize >> 16) & 0xFF); fileHeader[5] = (unsigned char)((fileSize >> 24) & 0xFF); // Reserved fields (4 bytes) stay 0. // Offset to start of pixel data (header + info header) fileHeader[10] = fileHeaderSize + infoHeaderSize; // --- Build the BMP info header (40 bytes, BITMAPINFOHEADER) --- unsigned char infoHeader[40] = {0}; infoHeader[0] = infoHeaderSize; // Header size (40 bytes) // Image width in pixels (little endian) infoHeader[4] = (unsigned char)(width & 0xFF); infoHeader[5] = (unsigned char)((width >> 8) & 0xFF); infoHeader[6] = (unsigned char)((width >> 16) & 0xFF); infoHeader[7] = (unsigned char)((width >> 24) & 0xFF); // Image height in pixels (little endian). Positive height = bottom-up. infoHeader[8] = (unsigned char)(height & 0xFF); infoHeader[9] = (unsigned char)((height >> 8) & 0xFF); infoHeader[10] = (unsigned char)((height >> 16) & 0xFF); infoHeader[11] = (unsigned char)((height >> 24) & 0xFF); // Planes (always 1) infoHeader[12] = 1; // Bits per pixel (24 for 24-bit BMP) infoHeader[14] = 24; // The remaining fields (compression, image size, resolution, colors used, etc.) // can be left as zero for an uncompressed BMP. // Write the headers to stdout. fwrite(fileHeader, 1, fileHeaderSize, stdout); fwrite(infoHeader, 1, infoHeaderSize, stdout); // --- Write the pixel data --- // Note: BMP pixel data is stored bottom-up, so we iterate from the last row to the first. unsigned char pixel[3]; // For one pixel (B, G, R) for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { // Determine which stripe we are in. if (x < width / 3) { // Green stripe: (Blue=0, Green=255, Red=0) pixel[0] = 0; pixel[1] = 255; pixel[2] = 0; } else if (x < (2 * width) / 3) { // White stripe: (Blue=255, Green=255, Red=255) pixel[0] = 255; pixel[1] = 255; pixel[2] = 255; } else { // Red stripe: (Blue=0, Green=0, Red=255) pixel[0] = 0; pixel[1] = 0; pixel[2] = 255; } fwrite(pixel, 1, 3, stdout); } // No row padding is needed, as rowSize is exactly 900 bytes. } return 0; } -------------------------------------------------- How to compile and run: 1. Save this code into a file, for example, flag.c. 2. Compile it: gcc flag.c -o flag 3. Run it redirecting stdout to a .bmp file: ./flag > italian_flag.bmp You should now have a BMP image that depicts the Italian flag.
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 | #include <stdio.h> #include <stdint.h> int main(void) { // Image dimensions const int width = 300; const int height = 200; // Each pixel is 3 bytes (B, G, R). Each row must be padded to a multiple of 4 bytes. // For width=300, row size = 300*3 = 900, which is already a multiple of 4. const int rowSize = width * 3; const int pixelDataSize = rowSize * height; // BMP file header (14 bytes) + DIB header (40 bytes) const int fileHeaderSize = 14; const int infoHeaderSize = 40; const int fileSize = fileHeaderSize + infoHeaderSize + pixelDataSize; // --- Build the BMP file header (14 bytes) --- unsigned char fileHeader[14] = {0}; // Signature "BM" fileHeader[0] = 'B'; fileHeader[1] = 'M'; // File size in little endian fileHeader[2] = (unsigned char)(fileSize & 0xFF); fileHeader[3] = (unsigned char)((fileSize >> 8) & 0xFF); fileHeader[4] = (unsigned char)((fileSize >> 16) & 0xFF); fileHeader[5] = (unsigned char)((fileSize >> 24) & 0xFF); // Reserved fields (4 bytes) stay 0. // Offset to start of pixel data (header + info header) fileHeader[10] = fileHeaderSize + infoHeaderSize; // --- Build the BMP info header (40 bytes, BITMAPINFOHEADER) --- unsigned char infoHeader[40] = {0}; infoHeader[0] = infoHeaderSize; // Header size (40 bytes) // Image width in pixels (little endian) infoHeader[4] = (unsigned char)(width & 0xFF); infoHeader[5] = (unsigned char)((width >> 8) & 0xFF); infoHeader[6] = (unsigned char)((width >> 16) & 0xFF); infoHeader[7] = (unsigned char)((width >> 24) & 0xFF); // Image height in pixels (little endian). Positive height = bottom-up. infoHeader[8] = (unsigned char)(height & 0xFF); infoHeader[9] = (unsigned char)((height >> 8) & 0xFF); infoHeader[10] = (unsigned char)((height >> 16) & 0xFF); infoHeader[11] = (unsigned char)((height >> 24) & 0xFF); // Planes (always 1) infoHeader[12] = 1; // Bits per pixel (24 for 24-bit BMP) infoHeader[14] = 24; // The remaining fields (compression, image size, resolution, colors used, etc.) // can be left as zero for an uncompressed BMP. // Write the headers to stdout. fwrite(fileHeader, 1, fileHeaderSize, stdout); fwrite(infoHeader, 1, infoHeaderSize, stdout); // --- Write the pixel data --- // Note: BMP pixel data is stored bottom-up, so we iterate from the last row to the first. unsigned char pixel[3]; // For one pixel (B, G, R) for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { // Determine which stripe we are in. if (x < width / 3) { // Green stripe: (Blue=0, Green=255, Red=0) pixel[0] = 0; pixel[1] = 255; pixel[2] = 0; } else if (x < (2 * width) / 3) { // White stripe: (Blue=255, Green=255, Red=255) pixel[0] = 255; pixel[1] = 255; pixel[2] = 255; } else { // Red stripe: (Blue=0, Green=0, Red=255) pixel[0] = 0; pixel[1] = 0; pixel[2] = 255; } fwrite(pixel, 1, 3, stdout); } // No row padding is needed, as rowSize is exactly 900 bytes. } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 | #include <stdio.h> #include <stdint.h> int main(void) { // Image dimensions const int width = 300; const int height = 200; // Each pixel is 3 bytes (B, G, R). Each row must be padded to a multiple of 4 bytes. // For width=300, row size = 300*3 = 900, which is already a multiple of 4. const int rowSize = width * 3; const int pixelDataSize = rowSize * height; // BMP file header (14 bytes) + DIB header (40 bytes) const int fileHeaderSize = 14; const int infoHeaderSize = 40; const int fileSize = fileHeaderSize + infoHeaderSize + pixelDataSize; // --- Build the BMP file header (14 bytes) --- unsigned char fileHeader[14] = {0}; // Signature "BM" fileHeader[0] = 'B'; fileHeader[1] = 'M'; // File size in little endian fileHeader[2] = (unsigned char)(fileSize & 0xFF); fileHeader[3] = (unsigned char)((fileSize >> 8) & 0xFF); fileHeader[4] = (unsigned char)((fileSize >> 16) & 0xFF); fileHeader[5] = (unsigned char)((fileSize >> 24) & 0xFF); // Reserved fields (4 bytes) stay 0. // Offset to start of pixel data (header + info header) fileHeader[10] = fileHeaderSize + infoHeaderSize; // --- Build the BMP info header (40 bytes, BITMAPINFOHEADER) --- unsigned char infoHeader[40] = {0}; infoHeader[0] = infoHeaderSize; // Header size (40 bytes) // Image width in pixels (little endian) infoHeader[4] = (unsigned char)(width & 0xFF); infoHeader[5] = (unsigned char)((width >> 8) & 0xFF); infoHeader[6] = (unsigned char)((width >> 16) & 0xFF); infoHeader[7] = (unsigned char)((width >> 24) & 0xFF); // Image height in pixels (little endian). Positive height = bottom-up. infoHeader[8] = (unsigned char)(height & 0xFF); infoHeader[9] = (unsigned char)((height >> 8) & 0xFF); infoHeader[10] = (unsigned char)((height >> 16) & 0xFF); infoHeader[11] = (unsigned char)((height >> 24) & 0xFF); // Planes (always 1) infoHeader[12] = 1; // Bits per pixel (24 for 24-bit BMP) infoHeader[14] = 24; // The remaining fields (compression, image size, resolution, colors used, etc.) // can be left as zero for an uncompressed BMP. // Write the headers to stdout. fwrite(fileHeader, 1, fileHeaderSize, stdout); fwrite(infoHeader, 1, infoHeaderSize, stdout); // --- Write the pixel data --- // Note: BMP pixel data is stored bottom-up, so we iterate from the last row to the first. unsigned char pixel[3]; // For one pixel (B, G, R) for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { // Determine which stripe we are in. if (x < width / 3) { // Green stripe: (Blue=0, Green=255, Red=0) pixel[0] = 0; pixel[1] = 255; pixel[2] = 0; } else if (x < (2 * width) / 3) { // White stripe: (Blue=255, Green=255, Red=255) pixel[0] = 255; pixel[1] = 255; pixel[2] = 255; } else { // Red stripe: (Blue=0, Green=0, Red=255) pixel[0] = 0; pixel[1] = 0; pixel[2] = 255; } fwrite(pixel, 1, 3, stdout); } // No row padding is needed, as rowSize is exactly 900 bytes. } return 0; } |
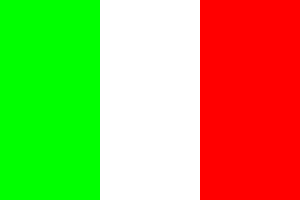
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three vertical stripes of green, white, and red.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True