Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Certainly! Below is a C program that generates a BMP image of the Italian flag and outputs it to `stdout`. You can redirect the output to a file to create the BMP image. ### Italian Flag Overview: The Italian flag consists of three vertical stripes of equal width: 1. **Green** on the left 2. **White** in the center 3. **Red** on the right ### How to Use the Program 1. **Compile the Program:**
1 2 | gcc italian_flag.c -o italian_flag |
1 2 | ./italian_flag > italian_flag.bmp |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 | #include <stdio.h> #include <stdint.h> #include <stdlib.h> // Ensure no padding in structures #pragma pack(push, 1) // BMP file header (14 bytes) typedef struct { uint16_t bfType; // File type ("BM") uint32_t bfSize; // Size of the file in bytes uint16_t bfReserved1; // Reserved, must be zero uint16_t bfReserved2; // Reserved, must be zero uint32_t bfOffBits; // Offset to start of pixel data } BITMAPFILEHEADER; // BMP info header (40 bytes) typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Compression type (0 = uncompressed) uint32_t biSizeImage; // Image size (can be 0 for uncompressed) int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the palette uint32_t biClrImportant; // Important colors } BITMAPINFOHEADER; #pragma pack(pop) int main() { // Image dimensions const int width = 300; const int height = 200; // Define BMP headers BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; // Calculate padding (each row must be a multiple of 4 bytes) int rowSize = (3 * width + 3) & (~3); int padding = rowSize - (3 * width); // Total pixel data size int pixelDataSize = rowSize * height; // Fill the file header fileHeader.bfType = 0x4D42; // 'BM' fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + pixelDataSize; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Fill the info header infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24-bit bitmap infoHeader.biCompression = 0; // BI_RGB, no compression infoHeader.biSizeImage = pixelDataSize; infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Write headers to stdout fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout); fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout); // Generate and write pixel data // BMP stores pixels from bottom to top for(int y = 0; y < height; y++) { for(int x = 0; x < width; x++) { unsigned char r, g, b; // Determine which third the current pixel is in if(x < width / 3) { // Green stripe b = 0; g = 128; // Dark green r = 0; } else if(x < 2 * width / 3) { // White stripe b = 255; g = 255; r = 255; } else { // Red stripe b = 0; g = 0; r = 128; // Dark red } // Write in BGR format fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } // Write padding unsigned char pad = 0; for(int p = 0; p < padding; p++) { fwrite(&pad, 1, 1, stdout); } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 | #include <stdio.h> #include <stdint.h> #include <stdlib.h> // Ensure no padding in structures #pragma pack(push, 1) // BMP file header (14 bytes) typedef struct { uint16_t bfType; // File type ("BM") uint32_t bfSize; // Size of the file in bytes uint16_t bfReserved1; // Reserved, must be zero uint16_t bfReserved2; // Reserved, must be zero uint32_t bfOffBits; // Offset to start of pixel data } BITMAPFILEHEADER; // BMP info header (40 bytes) typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Compression type (0 = uncompressed) uint32_t biSizeImage; // Image size (can be 0 for uncompressed) int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the palette uint32_t biClrImportant; // Important colors } BITMAPINFOHEADER; #pragma pack(pop) int main() { // Image dimensions const int width = 300; const int height = 200; // Define BMP headers BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; // Calculate padding (each row must be a multiple of 4 bytes) int rowSize = (3 * width + 3) & (~3); int padding = rowSize - (3 * width); // Total pixel data size int pixelDataSize = rowSize * height; // Fill the file header fileHeader.bfType = 0x4D42; // 'BM' fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + pixelDataSize; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Fill the info header infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24-bit bitmap infoHeader.biCompression = 0; // BI_RGB, no compression infoHeader.biSizeImage = pixelDataSize; infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Write headers to stdout fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout); fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout); // Generate and write pixel data // BMP stores pixels from bottom to top for(int y = 0; y < height; y++) { for(int x = 0; x < width; x++) { unsigned char r, g, b; // Determine which third the current pixel is in if(x < width / 3) { // Green stripe b = 0; g = 128; // Dark green r = 0; } else if(x < 2 * width / 3) { // White stripe b = 255; g = 255; r = 255; } else { // Red stripe b = 0; g = 0; r = 128; // Dark red } // Write in BGR format fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } // Write padding unsigned char pad = 0; for(int p = 0; p < padding; p++) { fwrite(&pad, 1, 1, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 | #include <stdio.h> #include <stdint.h> #include <stdlib.h> // Ensure no padding in structures #pragma pack(push, 1) // BMP file header (14 bytes) typedef struct { uint16_t bfType; // File type ("BM") uint32_t bfSize; // Size of the file in bytes uint16_t bfReserved1; // Reserved, must be zero uint16_t bfReserved2; // Reserved, must be zero uint32_t bfOffBits; // Offset to start of pixel data } BITMAPFILEHEADER; // BMP info header (40 bytes) typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Compression type (0 = uncompressed) uint32_t biSizeImage; // Image size (can be 0 for uncompressed) int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the palette uint32_t biClrImportant; // Important colors } BITMAPINFOHEADER; #pragma pack(pop) int main() { // Image dimensions const int width = 300; const int height = 200; // Define BMP headers BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; // Calculate padding (each row must be a multiple of 4 bytes) int rowSize = (3 * width + 3) & (~3); int padding = rowSize - (3 * width); // Total pixel data size int pixelDataSize = rowSize * height; // Fill the file header fileHeader.bfType = 0x4D42; // 'BM' fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + pixelDataSize; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Fill the info header infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24-bit bitmap infoHeader.biCompression = 0; // BI_RGB, no compression infoHeader.biSizeImage = pixelDataSize; infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Write headers to stdout fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout); fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout); // Generate and write pixel data // BMP stores pixels from bottom to top for(int y = 0; y < height; y++) { for(int x = 0; x < width; x++) { unsigned char r, g, b; // Determine which third the current pixel is in if(x < width / 3) { // Green stripe b = 0; g = 128; // Dark green r = 0; } else if(x < 2 * width / 3) { // White stripe b = 255; g = 255; r = 255; } else { // Red stripe b = 0; g = 0; r = 128; // Dark red } // Write in BGR format fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } // Write padding unsigned char pad = 0; for(int p = 0; p < padding; p++) { fwrite(&pad, 1, 1, stdout); } } return 0; } |
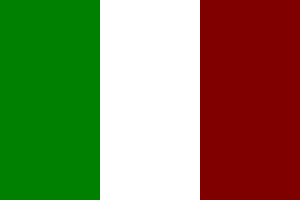
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three vertical stripes of equal width, with green on the hoist side, white in the middle, and red on the fly side.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Certainly! Below is a C program that generates a BMP image of the Italian flag and writes it to `stdout`. You can compile and run this program, redirecting the output to create a `.bmp` file. ### How It Works 1. **BMP File Structure**: - **BITMAPFILEHEADER**: Contains general information about the BMP file. - **BITMAPINFOHEADER**: Contains detailed information about the image, such as width, height, and color format. - **Pixel Data**: The actual image data, with each pixel represented in BGR format. 2. **Image Specifications**: - **Width**: 300 pixels - **Height**: 200 pixels - **Color Depth**: 24 bits per pixel (BGR) - **Stripe Division**: The flag has three vertical stripes (green, white, red), each occupying one-third of the width. 3. **Output**: - The program writes the BMP headers and pixel data directly to `stdout`. - To create the BMP file, redirect the output to a file, e.g., `./italian_flag > italian_flag.bmp`. ### C Program
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 | #include <stdio.h> #include <stdint.h> #include <stdlib.h> // Ensure no padding #pragma pack(push, 1) typedef struct { uint16_t bfType; // Signature "BM" uint32_t bfSize; // File size in bytes uint16_t bfReserved1; // Reserved, must be 0 uint16_t bfReserved2; // Reserved, must be 0 uint32_t bfOffBits; // Offset to pixel data } BITMAPFILEHEADER; typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of bitmap in pixels int32_t biHeight; // Height of bitmap in pixels uint16_t biPlanes; // Number of color planes, must be 1 uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Compression type (0 = uncompressed) uint32_t biSizeImage; // Image size (can be 0 for uncompressed) int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the palette uint32_t biClrImportant; // Important colors } BITMAPINFOHEADER; #pragma pack(pop) int main() { // Image dimensions const int width = 300; const int height = 200; const int bytesPerPixel = 3; // 24-bit BMP const int paddingPerRow = (4 - (width * bytesPerPixel) % 4) % 4; const int pixelDataSize = (bytesPerPixel * width + paddingPerRow) * height; const int fileSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + pixelDataSize; // Initialize BMP file header BITMAPFILEHEADER fileHeader; fileHeader.bfType = 0x4D42; // "BM" fileHeader.bfSize = fileSize; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Initialize BMP info header BITMAPINFOHEADER infoHeader; infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; // Positive for bottom-up bitmap infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24-bit bitmap infoHeader.biCompression = 0; // BI_RGB, no compression infoHeader.biSizeImage = pixelDataSize; infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Write headers to stdout if (fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout) != 1) { fprintf(stderr, "Error writing BMP file header.\n"); return EXIT_FAILURE; } if (fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout) != 1) { fprintf(stderr, "Error writing BMP info header.\n"); return EXIT_FAILURE; } // Define colors in BGR uint8_t green[3] = {0x00, 0x80, 0x00}; // Dark green uint8_t white[3] = {0xFF, 0xFF, 0xFF}; uint8_t red[3] = {0x00, 0x00, 0xFF}; // Red in BGR // Calculate stripe width int stripeWidth = width / 3; // Generate pixel data (BMP stores from bottom to top) for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t *color; if (x < stripeWidth) { color = green; } else if (x < 2 * stripeWidth) { color = white; } else { color = red; } if (fwrite(color, 1, 3, stdout) != 3) { fprintf(stderr, "Error writing pixel data.\n"); return EXIT_FAILURE; } } // Write padding uint8_t padding[3] = {0, 0, 0}; if (paddingPerRow > 0) { if (fwrite(padding, 1, paddingPerRow, stdout) != paddingPerRow) { fprintf(stderr, "Error writing padding.\n"); return EXIT_FAILURE; } } } return EXIT_SUCCESS; } |
1 2 | gcc -o italian_flag italian_flag.c |
1 2 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 | #include <stdio.h> #include <stdint.h> #include <stdlib.h> // Ensure no padding #pragma pack(push, 1) typedef struct { uint16_t bfType; // Signature "BM" uint32_t bfSize; // File size in bytes uint16_t bfReserved1; // Reserved, must be 0 uint16_t bfReserved2; // Reserved, must be 0 uint32_t bfOffBits; // Offset to pixel data } BITMAPFILEHEADER; typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of bitmap in pixels int32_t biHeight; // Height of bitmap in pixels uint16_t biPlanes; // Number of color planes, must be 1 uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Compression type (0 = uncompressed) uint32_t biSizeImage; // Image size (can be 0 for uncompressed) int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the palette uint32_t biClrImportant; // Important colors } BITMAPINFOHEADER; #pragma pack(pop) int main() { // Image dimensions const int width = 300; const int height = 200; const int bytesPerPixel = 3; // 24-bit BMP const int paddingPerRow = (4 - (width * bytesPerPixel) % 4) % 4; const int pixelDataSize = (bytesPerPixel * width + paddingPerRow) * height; const int fileSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + pixelDataSize; // Initialize BMP file header BITMAPFILEHEADER fileHeader; fileHeader.bfType = 0x4D42; // "BM" fileHeader.bfSize = fileSize; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Initialize BMP info header BITMAPINFOHEADER infoHeader; infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; // Positive for bottom-up bitmap infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24-bit bitmap infoHeader.biCompression = 0; // BI_RGB, no compression infoHeader.biSizeImage = pixelDataSize; infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Write headers to stdout if (fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout) != 1) { fprintf(stderr, "Error writing BMP file header.\n"); return EXIT_FAILURE; } if (fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout) != 1) { fprintf(stderr, "Error writing BMP info header.\n"); return EXIT_FAILURE; } // Define colors in BGR uint8_t green[3] = {0x00, 0x80, 0x00}; // Dark green uint8_t white[3] = {0xFF, 0xFF, 0xFF}; uint8_t red[3] = {0x00, 0x00, 0xFF}; // Red in BGR // Calculate stripe width int stripeWidth = width / 3; // Generate pixel data (BMP stores from bottom to top) for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t *color; if (x < stripeWidth) { color = green; } else if (x < 2 * stripeWidth) { color = white; } else { color = red; } if (fwrite(color, 1, 3, stdout) != 3) { fprintf(stderr, "Error writing pixel data.\n"); return EXIT_FAILURE; } } // Write padding uint8_t padding[3] = {0, 0, 0}; if (paddingPerRow > 0) { if (fwrite(padding, 1, paddingPerRow, stdout) != paddingPerRow) { fprintf(stderr, "Error writing padding.\n"); return EXIT_FAILURE; } } } return EXIT_SUCCESS; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 | #include <stdio.h> #include <stdint.h> #include <stdlib.h> // Ensure no padding #pragma pack(push, 1) typedef struct { uint16_t bfType; // Signature "BM" uint32_t bfSize; // File size in bytes uint16_t bfReserved1; // Reserved, must be 0 uint16_t bfReserved2; // Reserved, must be 0 uint32_t bfOffBits; // Offset to pixel data } BITMAPFILEHEADER; typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of bitmap in pixels int32_t biHeight; // Height of bitmap in pixels uint16_t biPlanes; // Number of color planes, must be 1 uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Compression type (0 = uncompressed) uint32_t biSizeImage; // Image size (can be 0 for uncompressed) int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the palette uint32_t biClrImportant; // Important colors } BITMAPINFOHEADER; #pragma pack(pop) int main() { // Image dimensions const int width = 300; const int height = 200; const int bytesPerPixel = 3; // 24-bit BMP const int paddingPerRow = (4 - (width * bytesPerPixel) % 4) % 4; const int pixelDataSize = (bytesPerPixel * width + paddingPerRow) * height; const int fileSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + pixelDataSize; // Initialize BMP file header BITMAPFILEHEADER fileHeader; fileHeader.bfType = 0x4D42; // "BM" fileHeader.bfSize = fileSize; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Initialize BMP info header BITMAPINFOHEADER infoHeader; infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; // Positive for bottom-up bitmap infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24-bit bitmap infoHeader.biCompression = 0; // BI_RGB, no compression infoHeader.biSizeImage = pixelDataSize; infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Write headers to stdout if (fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout) != 1) { fprintf(stderr, "Error writing BMP file header.\n"); return EXIT_FAILURE; } if (fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout) != 1) { fprintf(stderr, "Error writing BMP info header.\n"); return EXIT_FAILURE; } // Define colors in BGR uint8_t green[3] = {0x00, 0x80, 0x00}; // Dark green uint8_t white[3] = {0xFF, 0xFF, 0xFF}; uint8_t red[3] = {0x00, 0x00, 0xFF}; // Red in BGR // Calculate stripe width int stripeWidth = width / 3; // Generate pixel data (BMP stores from bottom to top) for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t *color; if (x < stripeWidth) { color = green; } else if (x < 2 * stripeWidth) { color = white; } else { color = red; } if (fwrite(color, 1, 3, stdout) != 3) { fprintf(stderr, "Error writing pixel data.\n"); return EXIT_FAILURE; } } // Write padding uint8_t padding[3] = {0, 0, 0}; if (paddingPerRow > 0) { if (fwrite(padding, 1, paddingPerRow, stdout) != paddingPerRow) { fprintf(stderr, "Error writing padding.\n"); return EXIT_FAILURE; } } } return EXIT_SUCCESS; } |
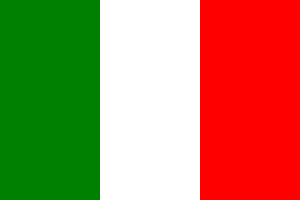
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three vertical stripes of green, white, and red.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True