Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
**Italian Flag Generator in C** ================================ Below is a C program that generates a .bmp image of the Italian flag and outputs it to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 | #include <stdio.h> // Define the BMP file header structure typedef struct { char bfType[2]; // 'BM' (0x424d) int bfSize; // size of file in bytes short bfReserved1; // reserved, must be 0 short bfReserved2; // reserved, must be 0 int bfOffBits; // offset to pixel array in bytes } BMPFileHeader; // Define the BMP info header structure typedef struct { int biSize; // size of info header in bytes int biWidth; // width of image in pixels int biHeight; // height of image in pixels short biPlanes; // number of color planes, must be 1 short biBitCount; // number of bits per pixel, 1, 4, 8, or 24 int biCompression; // compression type (0 = none) int biSizeImage; // size of pixel array in bytes int biXPelsPerMeter; // horizontal resolution in pixels per meter int biYPelsPerMeter; // vertical resolution in pixels per meter int biClrUsed; // number of colors in color table int biClrImportant; // number of important colors } BMPInfoHeader; int main() { // Set image dimensions const int width = 300; const int height = 200; // Set colors (RGB) const unsigned char green[] = {0, 255, 0}; // green const unsigned char white[] = {255, 255, 255}; // white const unsigned char red[] = {255, 0, 0}; // red // Create BMP file header BMPFileHeader fileHeader; fileHeader.bfType[0] = 'B'; fileHeader.bfType[1] = 'M'; fileHeader.bfSize = 54 + 3 * width * height; // size of file in bytes fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = 54; // offset to pixel array in bytes // Create BMP info header BMPInfoHeader infoHeader; infoHeader.biSize = 40; // size of info header in bytes infoHeader.biWidth = width; // width of image in pixels infoHeader.biHeight = height; // height of image in pixels infoHeader.biPlanes = 1; // number of color planes, must be 1 infoHeader.biBitCount = 24; // number of bits per pixel, 1, 4, 8, or 24 infoHeader.biCompression = 0; // compression type (0 = none) infoHeader.biSizeImage = 3 * width * height; // size of pixel array in bytes infoHeader.biXPelsPerMeter = 0; // horizontal resolution in pixels per meter infoHeader.biYPelsPerMeter = 0; // vertical resolution in pixels per meter infoHeader.biClrUsed = 0; // number of colors in color table infoHeader.biClrImportant = 0; // number of important colors // Write BMP file header fwrite(&fileHeader.bfType, 1, 2, stdout); fwrite(&fileHeader.bfSize, 4, 1, stdout); fwrite(&fileHeader.bfReserved1, 2, 1, stdout); fwrite(&fileHeader.bfReserved2, 2, 1, stdout); fwrite(&fileHeader.bfOffBits, 4, 1, stdout); // Write BMP info header fwrite(&infoHeader.biSize, 4, 1, stdout); fwrite(&infoHeader.biWidth, 4, 1, stdout); fwrite(&infoHeader.biHeight, 4, 1, stdout); fwrite(&infoHeader.biPlanes, 2, 1, stdout); fwrite(&infoHeader.biBitCount, 2, 1, stdout); fwrite(&infoHeader.biCompression, 4, 1, stdout); fwrite(&infoHeader.biSizeImage, 4, 1, stdout); fwrite(&infoHeader.biXPelsPerMeter, 4, 1, stdout); fwrite(&infoHeader.biYPelsPerMeter, 4, 1, stdout); fwrite(&infoHeader.biClrUsed, 4, 1, stdout); fwrite(&infoHeader.biClrImportant, 4, 1, stdout); // Write pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // green fwrite(green, 1, 3, stdout); } else if (x < 2 * width / 3) { // white fwrite(white, 1, 3, stdout); } else { // red fwrite(red, 1, 3, stdout); } } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 | #include <stdio.h> // Define the BMP file header structure typedef struct { char bfType[2]; // 'BM' (0x424d) int bfSize; // size of file in bytes short bfReserved1; // reserved, must be 0 short bfReserved2; // reserved, must be 0 int bfOffBits; // offset to pixel array in bytes } BMPFileHeader; // Define the BMP info header structure typedef struct { int biSize; // size of info header in bytes int biWidth; // width of image in pixels int biHeight; // height of image in pixels short biPlanes; // number of color planes, must be 1 short biBitCount; // number of bits per pixel, 1, 4, 8, or 24 int biCompression; // compression type (0 = none) int biSizeImage; // size of pixel array in bytes int biXPelsPerMeter; // horizontal resolution in pixels per meter int biYPelsPerMeter; // vertical resolution in pixels per meter int biClrUsed; // number of colors in color table int biClrImportant; // number of important colors } BMPInfoHeader; int main() { // Set image dimensions const int width = 300; const int height = 200; // Set colors (RGB) const unsigned char green[] = {0, 255, 0}; // green const unsigned char white[] = {255, 255, 255}; // white const unsigned char red[] = {255, 0, 0}; // red // Create BMP file header BMPFileHeader fileHeader; fileHeader.bfType[0] = 'B'; fileHeader.bfType[1] = 'M'; fileHeader.bfSize = 54 + 3 * width * height; // size of file in bytes fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = 54; // offset to pixel array in bytes // Create BMP info header BMPInfoHeader infoHeader; infoHeader.biSize = 40; // size of info header in bytes infoHeader.biWidth = width; // width of image in pixels infoHeader.biHeight = height; // height of image in pixels infoHeader.biPlanes = 1; // number of color planes, must be 1 infoHeader.biBitCount = 24; // number of bits per pixel, 1, 4, 8, or 24 infoHeader.biCompression = 0; // compression type (0 = none) infoHeader.biSizeImage = 3 * width * height; // size of pixel array in bytes infoHeader.biXPelsPerMeter = 0; // horizontal resolution in pixels per meter infoHeader.biYPelsPerMeter = 0; // vertical resolution in pixels per meter infoHeader.biClrUsed = 0; // number of colors in color table infoHeader.biClrImportant = 0; // number of important colors // Write BMP file header fwrite(&fileHeader.bfType, 1, 2, stdout); fwrite(&fileHeader.bfSize, 4, 1, stdout); fwrite(&fileHeader.bfReserved1, 2, 1, stdout); fwrite(&fileHeader.bfReserved2, 2, 1, stdout); fwrite(&fileHeader.bfOffBits, 4, 1, stdout); // Write BMP info header fwrite(&infoHeader.biSize, 4, 1, stdout); fwrite(&infoHeader.biWidth, 4, 1, stdout); fwrite(&infoHeader.biHeight, 4, 1, stdout); fwrite(&infoHeader.biPlanes, 2, 1, stdout); fwrite(&infoHeader.biBitCount, 2, 1, stdout); fwrite(&infoHeader.biCompression, 4, 1, stdout); fwrite(&infoHeader.biSizeImage, 4, 1, stdout); fwrite(&infoHeader.biXPelsPerMeter, 4, 1, stdout); fwrite(&infoHeader.biYPelsPerMeter, 4, 1, stdout); fwrite(&infoHeader.biClrUsed, 4, 1, stdout); fwrite(&infoHeader.biClrImportant, 4, 1, stdout); // Write pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // green fwrite(green, 1, 3, stdout); } else if (x < 2 * width / 3) { // white fwrite(white, 1, 3, stdout); } else { // red fwrite(red, 1, 3, stdout); } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 | #include <stdio.h> // Define the BMP file header structure typedef struct { char bfType[2]; // 'BM' (0x424d) int bfSize; // size of file in bytes short bfReserved1; // reserved, must be 0 short bfReserved2; // reserved, must be 0 int bfOffBits; // offset to pixel array in bytes } BMPFileHeader; // Define the BMP info header structure typedef struct { int biSize; // size of info header in bytes int biWidth; // width of image in pixels int biHeight; // height of image in pixels short biPlanes; // number of color planes, must be 1 short biBitCount; // number of bits per pixel, 1, 4, 8, or 24 int biCompression; // compression type (0 = none) int biSizeImage; // size of pixel array in bytes int biXPelsPerMeter; // horizontal resolution in pixels per meter int biYPelsPerMeter; // vertical resolution in pixels per meter int biClrUsed; // number of colors in color table int biClrImportant; // number of important colors } BMPInfoHeader; int main() { // Set image dimensions const int width = 300; const int height = 200; // Set colors (RGB) const unsigned char green[] = {0, 255, 0}; // green const unsigned char white[] = {255, 255, 255}; // white const unsigned char red[] = {255, 0, 0}; // red // Create BMP file header BMPFileHeader fileHeader; fileHeader.bfType[0] = 'B'; fileHeader.bfType[1] = 'M'; fileHeader.bfSize = 54 + 3 * width * height; // size of file in bytes fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = 54; // offset to pixel array in bytes // Create BMP info header BMPInfoHeader infoHeader; infoHeader.biSize = 40; // size of info header in bytes infoHeader.biWidth = width; // width of image in pixels infoHeader.biHeight = height; // height of image in pixels infoHeader.biPlanes = 1; // number of color planes, must be 1 infoHeader.biBitCount = 24; // number of bits per pixel, 1, 4, 8, or 24 infoHeader.biCompression = 0; // compression type (0 = none) infoHeader.biSizeImage = 3 * width * height; // size of pixel array in bytes infoHeader.biXPelsPerMeter = 0; // horizontal resolution in pixels per meter infoHeader.biYPelsPerMeter = 0; // vertical resolution in pixels per meter infoHeader.biClrUsed = 0; // number of colors in color table infoHeader.biClrImportant = 0; // number of important colors // Write BMP file header fwrite(&fileHeader.bfType, 1, 2, stdout); fwrite(&fileHeader.bfSize, 4, 1, stdout); fwrite(&fileHeader.bfReserved1, 2, 1, stdout); fwrite(&fileHeader.bfReserved2, 2, 1, stdout); fwrite(&fileHeader.bfOffBits, 4, 1, stdout); // Write BMP info header fwrite(&infoHeader.biSize, 4, 1, stdout); fwrite(&infoHeader.biWidth, 4, 1, stdout); fwrite(&infoHeader.biHeight, 4, 1, stdout); fwrite(&infoHeader.biPlanes, 2, 1, stdout); fwrite(&infoHeader.biBitCount, 2, 1, stdout); fwrite(&infoHeader.biCompression, 4, 1, stdout); fwrite(&infoHeader.biSizeImage, 4, 1, stdout); fwrite(&infoHeader.biXPelsPerMeter, 4, 1, stdout); fwrite(&infoHeader.biYPelsPerMeter, 4, 1, stdout); fwrite(&infoHeader.biClrUsed, 4, 1, stdout); fwrite(&infoHeader.biClrImportant, 4, 1, stdout); // Write pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // green fwrite(green, 1, 3, stdout); } else if (x < 2 * width / 3) { // white fwrite(white, 1, 3, stdout); } else { // red fwrite(red, 1, 3, stdout); } } } return 0; } |
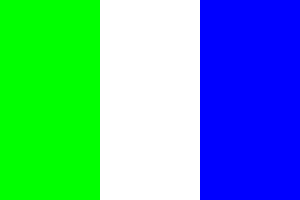
What flag is shown in this image?
The flag shown in the image is the flag of the Republic of Abkhazia. It consists of three vertical stripes of green, white, and blue.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False