Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Certainly! Below is a C program that generates a bitmap (.bmp) representation of the Italian flag and outputs it to `stdout`. The Italian flag consists of three vertical stripes: green on the left, white in the middle, and red on the right. Here's the code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) // Ensure no padding is added to the structures typedef struct { uint16_t bfType; // Magic identifier uint32_t bfSize; // File size in bytes uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to the start of pixel data } BITMAPFILEHEADER; typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Bits per pixel (24 bits for RGB) uint32_t biCompression; // Compression type (0 = none) uint32_t biSizeImage; // Image size (can be 0 for uncompressed) int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the palette (0 for 2^n) uint32_t biClrImportant; // Important colors (0 = all) } BITMAPINFOHEADER; #pragma pack(pop) void write_bmp_header(FILE *fp, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; // Set the BMP file header bfh.bfType = 0x4D42; // "BM" bfh.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + (width * height * 3); bfh.bfReserved1 = 0; bfh.bfReserved2 = 0; bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Set the BMP info header bih.biSize = sizeof(BITMAPINFOHEADER); bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; // 24 bits for RGB bih.biCompression = 0; // No compression bih.biSizeImage = 0; // Can be 0 for uncompressed bih.biXPelsPerMeter = 2835; // 72 DPI bih.biYPelsPerMeter = 2835; // 72 DPI bih.biClrUsed = 0; // 0 means use all colors bih.biClrImportant = 0; // All colors are important // Write the headers to the file fwrite(&bfh, sizeof(BITMAPFILEHEADER), 1, fp); fwrite(&bih, sizeof(BITMAPINFOHEADER), 1, fp); } void write_pixel_data(FILE *fp, int width, int height) { uint8_t green[3] = {0, 255, 0}; // Green uint8_t white[3] = {255, 255, 255}; // White uint8_t red[3] = {255, 0, 0}; // Red for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { fwrite(green, sizeof(uint8_t), 3, fp); // Write green } else if (x < 2 * width / 3) { fwrite(white, sizeof(uint8_t), 3, fp); // Write white } else { fwrite(red, sizeof(uint8_t), 3, fp); // Write red } } } } int main() { int width = 300; // Width of the flag int height = 200; // Height of the flag // Write BMP header and pixel data to stdout write_bmp_header(stdout, width, height); write_pixel_data(stdout, width, height); return 0; } |
1 2 | gcc -o italian_flag italian_flag.c ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) // Ensure no padding is added to the structures typedef struct { uint16_t bfType; // Magic identifier uint32_t bfSize; // File size in bytes uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to the start of pixel data } BITMAPFILEHEADER; typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Bits per pixel (24 bits for RGB) uint32_t biCompression; // Compression type (0 = none) uint32_t biSizeImage; // Image size (can be 0 for uncompressed) int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the palette (0 for 2^n) uint32_t biClrImportant; // Important colors (0 = all) } BITMAPINFOHEADER; #pragma pack(pop) void write_bmp_header(FILE *fp, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; // Set the BMP file header bfh.bfType = 0x4D42; // "BM" bfh.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + (width * height * 3); bfh.bfReserved1 = 0; bfh.bfReserved2 = 0; bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Set the BMP info header bih.biSize = sizeof(BITMAPINFOHEADER); bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; // 24 bits for RGB bih.biCompression = 0; // No compression bih.biSizeImage = 0; // Can be 0 for uncompressed bih.biXPelsPerMeter = 2835; // 72 DPI bih.biYPelsPerMeter = 2835; // 72 DPI bih.biClrUsed = 0; // 0 means use all colors bih.biClrImportant = 0; // All colors are important // Write the headers to the file fwrite(&bfh, sizeof(BITMAPFILEHEADER), 1, fp); fwrite(&bih, sizeof(BITMAPINFOHEADER), 1, fp); } void write_pixel_data(FILE *fp, int width, int height) { uint8_t green[3] = {0, 255, 0}; // Green uint8_t white[3] = {255, 255, 255}; // White uint8_t red[3] = {255, 0, 0}; // Red for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { fwrite(green, sizeof(uint8_t), 3, fp); // Write green } else if (x < 2 * width / 3) { fwrite(white, sizeof(uint8_t), 3, fp); // Write white } else { fwrite(red, sizeof(uint8_t), 3, fp); // Write red } } } } int main() { int width = 300; // Width of the flag int height = 200; // Height of the flag // Write BMP header and pixel data to stdout write_bmp_header(stdout, width, height); write_pixel_data(stdout, width, height); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) // Ensure no padding is added to the structures typedef struct { uint16_t bfType; // Magic identifier uint32_t bfSize; // File size in bytes uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to the start of pixel data } BITMAPFILEHEADER; typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Bits per pixel (24 bits for RGB) uint32_t biCompression; // Compression type (0 = none) uint32_t biSizeImage; // Image size (can be 0 for uncompressed) int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the palette (0 for 2^n) uint32_t biClrImportant; // Important colors (0 = all) } BITMAPINFOHEADER; #pragma pack(pop) void write_bmp_header(FILE *fp, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; // Set the BMP file header bfh.bfType = 0x4D42; // "BM" bfh.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + (width * height * 3); bfh.bfReserved1 = 0; bfh.bfReserved2 = 0; bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Set the BMP info header bih.biSize = sizeof(BITMAPINFOHEADER); bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; // 24 bits for RGB bih.biCompression = 0; // No compression bih.biSizeImage = 0; // Can be 0 for uncompressed bih.biXPelsPerMeter = 2835; // 72 DPI bih.biYPelsPerMeter = 2835; // 72 DPI bih.biClrUsed = 0; // 0 means use all colors bih.biClrImportant = 0; // All colors are important // Write the headers to the file fwrite(&bfh, sizeof(BITMAPFILEHEADER), 1, fp); fwrite(&bih, sizeof(BITMAPINFOHEADER), 1, fp); } void write_pixel_data(FILE *fp, int width, int height) { uint8_t green[3] = {0, 255, 0}; // Green uint8_t white[3] = {255, 255, 255}; // White uint8_t red[3] = {255, 0, 0}; // Red for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { fwrite(green, sizeof(uint8_t), 3, fp); // Write green } else if (x < 2 * width / 3) { fwrite(white, sizeof(uint8_t), 3, fp); // Write white } else { fwrite(red, sizeof(uint8_t), 3, fp); // Write red } } } } int main() { int width = 300; // Width of the flag int height = 200; // Height of the flag // Write BMP header and pixel data to stdout write_bmp_header(stdout, width, height); write_pixel_data(stdout, width, height); return 0; } |
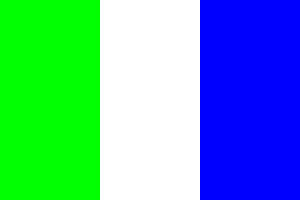
What flag is shown in this image?
The flag shown in the image is the flag of the Republic of Abkhazia. It consists of three vertical stripes of green, white, and blue.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
To create a C program that generates a BMP image of the Italian flag and outputs it to `stdout`, we need to adhere to the BMP file format. The Italian flag consists of three vertical stripes: green on the left, white in the middle, and red on the right. Here's a simple C program that accomplishes this task:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 | #include <stdio.h> #include <stdint.h> #include <string.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; // Magic number for BMP files uint32_t bfSize; // Size of the BMP file uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to start of pixel data } BITMAPFILEHEADER; typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Compression method (0 = none) uint32_t biSizeImage; // Size of the image data (can be 0 for uncompressed) int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the palette uint32_t biClrImportant; // Important colors (usually ignored) } BITMAPINFOHEADER; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) void writeBMPHeader(FILE *f, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; // Initialize headers memset(&bfh, 0, sizeof(bfh)); memset(&bih, 0, sizeof(bih)); bfh.bfType = 0x4D42; // 'BM' bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); bfh.bfSize = bfh.bfOffBits + (width * height * sizeof(RGB)); bfh.bfReserved1 = 0; bfh.bfReserved2 = 0; bih.biSize = sizeof(BITMAPINFOHEADER); bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; // 24 bits per pixel bih.biCompression = 0; // No compression bih.biSizeImage = 0; // Can be 0 for uncompressed bih.biXPelsPerMeter = 0; // Not important bih.biYPelsPerMeter = 0; // Not important bih.biClrUsed = 0; // All colors are important bih.biClrImportant = 0; // All colors are important fwrite(&bfh, sizeof(bfh), 1, f); fwrite(&bih, sizeof(bih), 1, f); } void writePixelData(FILE *f, int width, int height) { RGB colors[3] = { {0, 128, 0}, // Green {255, 255, 255}, // White {255, 0, 0} // Red }; for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { // Determine which color to use based on the x position int color_index = x / (width / 3); fwrite(&colors[color_index], sizeof(RGB), 1, f); } } } int main() { const int width = 300; // Width of the BMP const int height = 200; // Height of the BMP // Write the BMP header and pixel data to stdout writeBMPHeader(stdout, width, height); writePixelData(stdout, width, height); return 0; } |
1 2 | gcc -o italian_flag italian_flag.c ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 | #include <stdio.h> #include <stdint.h> #include <string.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; // Magic number for BMP files uint32_t bfSize; // Size of the BMP file uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to start of pixel data } BITMAPFILEHEADER; typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Compression method (0 = none) uint32_t biSizeImage; // Size of the image data (can be 0 for uncompressed) int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the palette uint32_t biClrImportant; // Important colors (usually ignored) } BITMAPINFOHEADER; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) void writeBMPHeader(FILE *f, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; // Initialize headers memset(&bfh, 0, sizeof(bfh)); memset(&bih, 0, sizeof(bih)); bfh.bfType = 0x4D42; // 'BM' bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); bfh.bfSize = bfh.bfOffBits + (width * height * sizeof(RGB)); bfh.bfReserved1 = 0; bfh.bfReserved2 = 0; bih.biSize = sizeof(BITMAPINFOHEADER); bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; // 24 bits per pixel bih.biCompression = 0; // No compression bih.biSizeImage = 0; // Can be 0 for uncompressed bih.biXPelsPerMeter = 0; // Not important bih.biYPelsPerMeter = 0; // Not important bih.biClrUsed = 0; // All colors are important bih.biClrImportant = 0; // All colors are important fwrite(&bfh, sizeof(bfh), 1, f); fwrite(&bih, sizeof(bih), 1, f); } void writePixelData(FILE *f, int width, int height) { RGB colors[3] = { {0, 128, 0}, // Green {255, 255, 255}, // White {255, 0, 0} // Red }; for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { // Determine which color to use based on the x position int color_index = x / (width / 3); fwrite(&colors[color_index], sizeof(RGB), 1, f); } } } int main() { const int width = 300; // Width of the BMP const int height = 200; // Height of the BMP // Write the BMP header and pixel data to stdout writeBMPHeader(stdout, width, height); writePixelData(stdout, width, height); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 | #include <stdio.h> #include <stdint.h> #include <string.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; // Magic number for BMP files uint32_t bfSize; // Size of the BMP file uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to start of pixel data } BITMAPFILEHEADER; typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Compression method (0 = none) uint32_t biSizeImage; // Size of the image data (can be 0 for uncompressed) int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the palette uint32_t biClrImportant; // Important colors (usually ignored) } BITMAPINFOHEADER; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) void writeBMPHeader(FILE *f, int width, int height) { BITMAPFILEHEADER bfh; BITMAPINFOHEADER bih; // Initialize headers memset(&bfh, 0, sizeof(bfh)); memset(&bih, 0, sizeof(bih)); bfh.bfType = 0x4D42; // 'BM' bfh.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); bfh.bfSize = bfh.bfOffBits + (width * height * sizeof(RGB)); bfh.bfReserved1 = 0; bfh.bfReserved2 = 0; bih.biSize = sizeof(BITMAPINFOHEADER); bih.biWidth = width; bih.biHeight = height; bih.biPlanes = 1; bih.biBitCount = 24; // 24 bits per pixel bih.biCompression = 0; // No compression bih.biSizeImage = 0; // Can be 0 for uncompressed bih.biXPelsPerMeter = 0; // Not important bih.biYPelsPerMeter = 0; // Not important bih.biClrUsed = 0; // All colors are important bih.biClrImportant = 0; // All colors are important fwrite(&bfh, sizeof(bfh), 1, f); fwrite(&bih, sizeof(bih), 1, f); } void writePixelData(FILE *f, int width, int height) { RGB colors[3] = { {0, 128, 0}, // Green {255, 255, 255}, // White {255, 0, 0} // Red }; for (int y = 0; y < height; ++y) { for (int x = 0; x < width; ++x) { // Determine which color to use based on the x position int color_index = x / (width / 3); fwrite(&colors[color_index], sizeof(RGB), 1, f); } } } int main() { const int width = 300; // Width of the BMP const int height = 200; // Height of the BMP // Write the BMP header and pixel data to stdout writeBMPHeader(stdout, width, height); writePixelData(stdout, width, height); return 0; } |
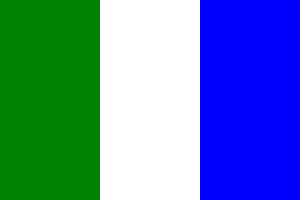
What flag is shown in this image?
The flag shown in the image is the flag of the Ivory Coast (Côte d'Ivoire). It consists of three vertical stripes of green, white, and blue.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Creating a BMP file that represents the Italian flag in C involves understanding the BMP file format and how to manipulate pixel data. The Italian flag consists of three vertical stripes: green, white, and red. The following C program generates a BMP file of the Italian flag and writes it to standard output (stdout). Here's the complete code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 | #include <stdio.h> #include <stdint.h> #include <stdlib.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; // BMP file type uint32_t bfSize; // Size of the BMP file in bytes uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to start of pixel data } BMPHeader; typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Compression method uint32_t biSizeImage; // Size of the image data int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the color palette uint32_t biClrImportant; // Important colors } BMPInfoHeader; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) #define WIDTH 300 #define HEIGHT 200 void writeBMPHeader(FILE *fp) { BMPHeader bmpHeader; BMPInfoHeader bmpInfoHeader; // Initialize BMP header bmpHeader.bfType = 0x4D42; // 'BM' bmpHeader.bfOffBits = sizeof(BMPHeader) + sizeof(BMPInfoHeader); bmpHeader.bfSize = bmpHeader.bfOffBits + (WIDTH * HEIGHT * sizeof(RGB)); bmpHeader.bfReserved1 = 0; bmpHeader.bfReserved2 = 0; // Initialize BMP info header bmpInfoHeader.biSize = sizeof(BMPInfoHeader); bmpInfoHeader.biWidth = WIDTH; bmpInfoHeader.biHeight = HEIGHT; bmpInfoHeader.biPlanes = 1; bmpInfoHeader.biBitCount = 24; // 24 bits per pixel (RGB) bmpInfoHeader.biCompression = 0; // No compression bmpInfoHeader.biSizeImage = WIDTH * HEIGHT * sizeof(RGB); bmpInfoHeader.biXPelsPerMeter = 0; bmpInfoHeader.biYPelsPerMeter = 0; bmpInfoHeader.biClrUsed = 0; bmpInfoHeader.biClrImportant = 0; // Write headers to file fwrite(&bmpHeader, sizeof(BMPHeader), 1, fp); fwrite(&bmpInfoHeader, sizeof(BMPInfoHeader), 1, fp); } void writeItalianFlag(FILE *fp) { RGB pixel; // Write pixel data for the Italian flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { // Green pixel.red = 0; pixel.green = 146; // RGB for green pixel.blue = 70; } else if (x < 2 * WIDTH / 3) { // White pixel.red = 255; pixel.green = 255; pixel.blue = 255; } else { // Red pixel.red = 206; // RGB for red pixel.green = 17; pixel.blue = 38; } fwrite(&pixel, sizeof(RGB), 1, fp); } } } int main() { // Open stdout as a binary file FILE *fp = stdout; // Write BMP headers writeBMPHeader(fp); // Write Italian flag pixel data writeItalianFlag(fp); return 0; } |
1 2 | gcc -o italian_flag italian_flag.c ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 | #include <stdio.h> #include <stdint.h> #include <stdlib.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; // BMP file type uint32_t bfSize; // Size of the BMP file in bytes uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to start of pixel data } BMPHeader; typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Compression method uint32_t biSizeImage; // Size of the image data int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the color palette uint32_t biClrImportant; // Important colors } BMPInfoHeader; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) #define WIDTH 300 #define HEIGHT 200 void writeBMPHeader(FILE *fp) { BMPHeader bmpHeader; BMPInfoHeader bmpInfoHeader; // Initialize BMP header bmpHeader.bfType = 0x4D42; // 'BM' bmpHeader.bfOffBits = sizeof(BMPHeader) + sizeof(BMPInfoHeader); bmpHeader.bfSize = bmpHeader.bfOffBits + (WIDTH * HEIGHT * sizeof(RGB)); bmpHeader.bfReserved1 = 0; bmpHeader.bfReserved2 = 0; // Initialize BMP info header bmpInfoHeader.biSize = sizeof(BMPInfoHeader); bmpInfoHeader.biWidth = WIDTH; bmpInfoHeader.biHeight = HEIGHT; bmpInfoHeader.biPlanes = 1; bmpInfoHeader.biBitCount = 24; // 24 bits per pixel (RGB) bmpInfoHeader.biCompression = 0; // No compression bmpInfoHeader.biSizeImage = WIDTH * HEIGHT * sizeof(RGB); bmpInfoHeader.biXPelsPerMeter = 0; bmpInfoHeader.biYPelsPerMeter = 0; bmpInfoHeader.biClrUsed = 0; bmpInfoHeader.biClrImportant = 0; // Write headers to file fwrite(&bmpHeader, sizeof(BMPHeader), 1, fp); fwrite(&bmpInfoHeader, sizeof(BMPInfoHeader), 1, fp); } void writeItalianFlag(FILE *fp) { RGB pixel; // Write pixel data for the Italian flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { // Green pixel.red = 0; pixel.green = 146; // RGB for green pixel.blue = 70; } else if (x < 2 * WIDTH / 3) { // White pixel.red = 255; pixel.green = 255; pixel.blue = 255; } else { // Red pixel.red = 206; // RGB for red pixel.green = 17; pixel.blue = 38; } fwrite(&pixel, sizeof(RGB), 1, fp); } } } int main() { // Open stdout as a binary file FILE *fp = stdout; // Write BMP headers writeBMPHeader(fp); // Write Italian flag pixel data writeItalianFlag(fp); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 | #include <stdio.h> #include <stdint.h> #include <stdlib.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; // BMP file type uint32_t bfSize; // Size of the BMP file in bytes uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to start of pixel data } BMPHeader; typedef struct { uint32_t biSize; // Size of this header (40 bytes) int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Compression method uint32_t biSizeImage; // Size of the image data int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the color palette uint32_t biClrImportant; // Important colors } BMPInfoHeader; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } RGB; #pragma pack(pop) #define WIDTH 300 #define HEIGHT 200 void writeBMPHeader(FILE *fp) { BMPHeader bmpHeader; BMPInfoHeader bmpInfoHeader; // Initialize BMP header bmpHeader.bfType = 0x4D42; // 'BM' bmpHeader.bfOffBits = sizeof(BMPHeader) + sizeof(BMPInfoHeader); bmpHeader.bfSize = bmpHeader.bfOffBits + (WIDTH * HEIGHT * sizeof(RGB)); bmpHeader.bfReserved1 = 0; bmpHeader.bfReserved2 = 0; // Initialize BMP info header bmpInfoHeader.biSize = sizeof(BMPInfoHeader); bmpInfoHeader.biWidth = WIDTH; bmpInfoHeader.biHeight = HEIGHT; bmpInfoHeader.biPlanes = 1; bmpInfoHeader.biBitCount = 24; // 24 bits per pixel (RGB) bmpInfoHeader.biCompression = 0; // No compression bmpInfoHeader.biSizeImage = WIDTH * HEIGHT * sizeof(RGB); bmpInfoHeader.biXPelsPerMeter = 0; bmpInfoHeader.biYPelsPerMeter = 0; bmpInfoHeader.biClrUsed = 0; bmpInfoHeader.biClrImportant = 0; // Write headers to file fwrite(&bmpHeader, sizeof(BMPHeader), 1, fp); fwrite(&bmpInfoHeader, sizeof(BMPInfoHeader), 1, fp); } void writeItalianFlag(FILE *fp) { RGB pixel; // Write pixel data for the Italian flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { // Green pixel.red = 0; pixel.green = 146; // RGB for green pixel.blue = 70; } else if (x < 2 * WIDTH / 3) { // White pixel.red = 255; pixel.green = 255; pixel.blue = 255; } else { // Red pixel.red = 206; // RGB for red pixel.green = 17; pixel.blue = 38; } fwrite(&pixel, sizeof(RGB), 1, fp); } } } int main() { // Open stdout as a binary file FILE *fp = stdout; // Write BMP headers writeBMPHeader(fp); // Write Italian flag pixel data writeItalianFlag(fp); return 0; } |
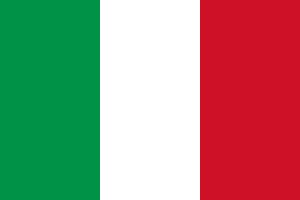
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three vertical stripes of equal width, with green on the hoist side, white in the middle, and red on the fly side.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
To create a BMP file that depicts the Italian flag using C, we need to follow the BMP file format specifications. The Italian flag consists of three vertical stripes in green, white, and red. We'll generate a 24-bit BMP file and output it to standard output (stdout). Here's a simple C program that does just that:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> // BMP file header size #define BMP_FILE_HEADER_SIZE 14 #define BMP_INFO_HEADER_SIZE 40 // BMP dimensions #define WIDTH 300 #define HEIGHT 200 // Function to write a 24-bit pixel void write_pixel(FILE *fp, uint8_t r, uint8_t g, uint8_t b) { fputc(b, fp); // Blue fputc(g, fp); // Green fputc(r, fp); // Red } int main() { // BMP headers uint8_t bmp_file_header[BMP_FILE_HEADER_SIZE] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (to be filled later) 0, 0, // Reserved1 0, 0, // Reserved2 BMP_FILE_HEADER_SIZE + BMP_INFO_HEADER_SIZE // Offset to pixel data }; uint8_t bmp_info_header[BMP_INFO_HEADER_SIZE] = { BMP_INFO_HEADER_SIZE, // Header size WIDTH & 0xFF, (WIDTH >> 8) & 0xFF, (WIDTH >> 16) & 0xFF, (WIDTH >> 24) & 0xFF, // Width HEIGHT & 0xFF, (HEIGHT >> 8) & 0xFF, (HEIGHT >> 16) & 0xFF, (HEIGHT >> 24) & 0xFF, // Height 1, // Planes 24, // Bits per pixel 0, // Compression 0, 0, 0, 0, // Size of raw bitmap data (to be filled later) 0, 0, 0, 0, // Print resolution (pixels per meter) 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Important colors }; // Calculate the sizes to fill in the headers int row_padded = (WIDTH * 3 + 3) & (~3); // Row size padded to 4 bytes int size_data = row_padded * HEIGHT; // Size of the pixel data int file_size = BMP_FILE_HEADER_SIZE + BMP_INFO_HEADER_SIZE + size_data; // Fill in the file size bmp_file_header[2] = (file_size) & 0xFF; bmp_file_header[3] = (file_size >> 8) & 0xFF; bmp_file_header[4] = (file_size >> 16) & 0xFF; bmp_file_header[5] = (file_size >> 24) & 0xFF; // Fill in the size of the raw bitmap data bmp_info_header[34] = (size_data) & 0xFF; bmp_info_header[35] = (size_data >> 8) & 0xFF; bmp_info_header[36] = (size_data >> 16) & 0xFF; bmp_info_header[37] = (size_data >> 24) & 0xFF; // Write the headers to stdout fwrite(bmp_file_header, sizeof(uint8_t), BMP_FILE_HEADER_SIZE, stdout); fwrite(bmp_info_header, sizeof(uint8_t), BMP_INFO_HEADER_SIZE, stdout); // Write the pixel data for the Italian flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { // Left stripe - Green write_pixel(stdout, 0, 155, 70); // RGB value for green } else if (x < 2 * WIDTH / 3) { // Middle stripe - White write_pixel(stdout, 255, 255, 255); // RGB value for white } else { // Right stripe - Red write_pixel(stdout, 186, 12, 47); // RGB value for red } } // Padding for each row for (int p = 0; p < (row_padded - WIDTH * 3); p++) { fputc(0, stdout); } } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> // BMP file header size #define BMP_FILE_HEADER_SIZE 14 #define BMP_INFO_HEADER_SIZE 40 // BMP dimensions #define WIDTH 300 #define HEIGHT 200 // Function to write a 24-bit pixel void write_pixel(FILE *fp, uint8_t r, uint8_t g, uint8_t b) { fputc(b, fp); // Blue fputc(g, fp); // Green fputc(r, fp); // Red } int main() { // BMP headers uint8_t bmp_file_header[BMP_FILE_HEADER_SIZE] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (to be filled later) 0, 0, // Reserved1 0, 0, // Reserved2 BMP_FILE_HEADER_SIZE + BMP_INFO_HEADER_SIZE // Offset to pixel data }; uint8_t bmp_info_header[BMP_INFO_HEADER_SIZE] = { BMP_INFO_HEADER_SIZE, // Header size WIDTH & 0xFF, (WIDTH >> 8) & 0xFF, (WIDTH >> 16) & 0xFF, (WIDTH >> 24) & 0xFF, // Width HEIGHT & 0xFF, (HEIGHT >> 8) & 0xFF, (HEIGHT >> 16) & 0xFF, (HEIGHT >> 24) & 0xFF, // Height 1, // Planes 24, // Bits per pixel 0, // Compression 0, 0, 0, 0, // Size of raw bitmap data (to be filled later) 0, 0, 0, 0, // Print resolution (pixels per meter) 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Important colors }; // Calculate the sizes to fill in the headers int row_padded = (WIDTH * 3 + 3) & (~3); // Row size padded to 4 bytes int size_data = row_padded * HEIGHT; // Size of the pixel data int file_size = BMP_FILE_HEADER_SIZE + BMP_INFO_HEADER_SIZE + size_data; // Fill in the file size bmp_file_header[2] = (file_size) & 0xFF; bmp_file_header[3] = (file_size >> 8) & 0xFF; bmp_file_header[4] = (file_size >> 16) & 0xFF; bmp_file_header[5] = (file_size >> 24) & 0xFF; // Fill in the size of the raw bitmap data bmp_info_header[34] = (size_data) & 0xFF; bmp_info_header[35] = (size_data >> 8) & 0xFF; bmp_info_header[36] = (size_data >> 16) & 0xFF; bmp_info_header[37] = (size_data >> 24) & 0xFF; // Write the headers to stdout fwrite(bmp_file_header, sizeof(uint8_t), BMP_FILE_HEADER_SIZE, stdout); fwrite(bmp_info_header, sizeof(uint8_t), BMP_INFO_HEADER_SIZE, stdout); // Write the pixel data for the Italian flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { // Left stripe - Green write_pixel(stdout, 0, 155, 70); // RGB value for green } else if (x < 2 * WIDTH / 3) { // Middle stripe - White write_pixel(stdout, 255, 255, 255); // RGB value for white } else { // Right stripe - Red write_pixel(stdout, 186, 12, 47); // RGB value for red } } // Padding for each row for (int p = 0; p < (row_padded - WIDTH * 3); p++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> // BMP file header size #define BMP_FILE_HEADER_SIZE 14 #define BMP_INFO_HEADER_SIZE 40 // BMP dimensions #define WIDTH 300 #define HEIGHT 200 // Function to write a 24-bit pixel void write_pixel(FILE *fp, uint8_t r, uint8_t g, uint8_t b) { fputc(b, fp); // Blue fputc(g, fp); // Green fputc(r, fp); // Red } int main() { // BMP headers uint8_t bmp_file_header[BMP_FILE_HEADER_SIZE] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (to be filled later) 0, 0, // Reserved1 0, 0, // Reserved2 BMP_FILE_HEADER_SIZE + BMP_INFO_HEADER_SIZE // Offset to pixel data }; uint8_t bmp_info_header[BMP_INFO_HEADER_SIZE] = { BMP_INFO_HEADER_SIZE, // Header size WIDTH & 0xFF, (WIDTH >> 8) & 0xFF, (WIDTH >> 16) & 0xFF, (WIDTH >> 24) & 0xFF, // Width HEIGHT & 0xFF, (HEIGHT >> 8) & 0xFF, (HEIGHT >> 16) & 0xFF, (HEIGHT >> 24) & 0xFF, // Height 1, // Planes 24, // Bits per pixel 0, // Compression 0, 0, 0, 0, // Size of raw bitmap data (to be filled later) 0, 0, 0, 0, // Print resolution (pixels per meter) 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Important colors }; // Calculate the sizes to fill in the headers int row_padded = (WIDTH * 3 + 3) & (~3); // Row size padded to 4 bytes int size_data = row_padded * HEIGHT; // Size of the pixel data int file_size = BMP_FILE_HEADER_SIZE + BMP_INFO_HEADER_SIZE + size_data; // Fill in the file size bmp_file_header[2] = (file_size) & 0xFF; bmp_file_header[3] = (file_size >> 8) & 0xFF; bmp_file_header[4] = (file_size >> 16) & 0xFF; bmp_file_header[5] = (file_size >> 24) & 0xFF; // Fill in the size of the raw bitmap data bmp_info_header[34] = (size_data) & 0xFF; bmp_info_header[35] = (size_data >> 8) & 0xFF; bmp_info_header[36] = (size_data >> 16) & 0xFF; bmp_info_header[37] = (size_data >> 24) & 0xFF; // Write the headers to stdout fwrite(bmp_file_header, sizeof(uint8_t), BMP_FILE_HEADER_SIZE, stdout); fwrite(bmp_info_header, sizeof(uint8_t), BMP_INFO_HEADER_SIZE, stdout); // Write the pixel data for the Italian flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { // Left stripe - Green write_pixel(stdout, 0, 155, 70); // RGB value for green } else if (x < 2 * WIDTH / 3) { // Middle stripe - White write_pixel(stdout, 255, 255, 255); // RGB value for white } else { // Right stripe - Red write_pixel(stdout, 186, 12, 47); // RGB value for red } } // Padding for each row for (int p = 0; p < (row_padded - WIDTH * 3); p++) { fputc(0, stdout); } } return 0; } |
b'BMV\xbf\x02\x00\x00\x00\x00\x006\x00\x00\x00(,\x01\x00\x00\xc8\x00\x00\x00\x01\x18\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00 \xbf\x02\x00\x00\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xbaF\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xbaF\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xba/\x0c\xbaF\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\x9b\x00F\
What flag is shown in this image?
Unsupported BMP header type (76840)
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Creating a BMP file in C to represent the Italian flag involves generating the correct header and pixel data. The Italian flag consists of three vertical stripes: green on the left, white in the middle, and red on the right. Below is a C program that generates a BMP image of the Italian flag and writes it to standard output.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 | #include <stdio.h> #include <stdint.h> #include <string.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; // BMP file type uint32_t bfSize; // Size of the BMP file uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to start of pixel data } BMPHeader; typedef struct { uint32_t biSize; // Size of this header int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Type of compression to use uint32_t biSizeImage; // Size of the image data int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the color palette uint32_t biClrImportant; // Number of important colors } BMPInfoHeader; #pragma pack(pop) void write_bmp_header(FILE *fp, int width, int height) { BMPHeader bmp_header; BMPInfoHeader bmp_info_header; // Fill BMP Header bmp_header.bfType = 0x4D42; // 'BM' bmp_header.bfSize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + width * height * 3; bmp_header.bfReserved1 = 0; bmp_header.bfReserved2 = 0; bmp_header.bfOffBits = sizeof(BMPHeader) + sizeof(BMPInfoHeader); // Fill BMP Info Header bmp_info_header.biSize = sizeof(BMPInfoHeader); bmp_info_header.biWidth = width; bmp_info_header.biHeight = height; bmp_info_header.biPlanes = 1; bmp_info_header.biBitCount = 24; // 24 bits per pixel bmp_info_header.biCompression = 0; // No compression bmp_info_header.biSizeImage = 0; // No compression, so image size can be 0 bmp_info_header.biXPelsPerMeter = 0; bmp_info_header.biYPelsPerMeter = 0; bmp_info_header.biClrUsed = 0; bmp_info_header.biClrImportant = 0; // Write headers fwrite(&bmp_header, sizeof(BMPHeader), 1, fp); fwrite(&bmp_info_header, sizeof(BMPInfoHeader), 1, fp); } void write_pixel_data(FILE *fp, int width, int height) { int row_size = (3 * width + 3) & ~3; // Rows must be aligned to 4-byte boundary uint8_t green[3] = {0, 255, 0}; // Green color uint8_t white[3] = {255, 255, 255}; // White color uint8_t red[3] = {255, 0, 0}; // Red color for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t *color; if (x < width / 3) { color = green; } else if (x < 2 * width / 3) { color = white; } else { color = red; } fwrite(color, 1, 3, fp); // Write pixel color } // Fill the rest of the row to meet the row size requirement uint8_t padding[3] = {0, 0, 0}; fwrite(padding, 1, row_size - (width * 3), fp); } } int main() { int width = 300; // Width of the flag int height = 200; // Height of the flag // Write to stdout write_bmp_header(stdout, width, height); write_pixel_data(stdout, width, height); return 0; } |
1 2 | gcc -o italian_flag italian_flag.c ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 | #include <stdio.h> #include <stdint.h> #include <string.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; // BMP file type uint32_t bfSize; // Size of the BMP file uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to start of pixel data } BMPHeader; typedef struct { uint32_t biSize; // Size of this header int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Type of compression to use uint32_t biSizeImage; // Size of the image data int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the color palette uint32_t biClrImportant; // Number of important colors } BMPInfoHeader; #pragma pack(pop) void write_bmp_header(FILE *fp, int width, int height) { BMPHeader bmp_header; BMPInfoHeader bmp_info_header; // Fill BMP Header bmp_header.bfType = 0x4D42; // 'BM' bmp_header.bfSize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + width * height * 3; bmp_header.bfReserved1 = 0; bmp_header.bfReserved2 = 0; bmp_header.bfOffBits = sizeof(BMPHeader) + sizeof(BMPInfoHeader); // Fill BMP Info Header bmp_info_header.biSize = sizeof(BMPInfoHeader); bmp_info_header.biWidth = width; bmp_info_header.biHeight = height; bmp_info_header.biPlanes = 1; bmp_info_header.biBitCount = 24; // 24 bits per pixel bmp_info_header.biCompression = 0; // No compression bmp_info_header.biSizeImage = 0; // No compression, so image size can be 0 bmp_info_header.biXPelsPerMeter = 0; bmp_info_header.biYPelsPerMeter = 0; bmp_info_header.biClrUsed = 0; bmp_info_header.biClrImportant = 0; // Write headers fwrite(&bmp_header, sizeof(BMPHeader), 1, fp); fwrite(&bmp_info_header, sizeof(BMPInfoHeader), 1, fp); } void write_pixel_data(FILE *fp, int width, int height) { int row_size = (3 * width + 3) & ~3; // Rows must be aligned to 4-byte boundary uint8_t green[3] = {0, 255, 0}; // Green color uint8_t white[3] = {255, 255, 255}; // White color uint8_t red[3] = {255, 0, 0}; // Red color for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t *color; if (x < width / 3) { color = green; } else if (x < 2 * width / 3) { color = white; } else { color = red; } fwrite(color, 1, 3, fp); // Write pixel color } // Fill the rest of the row to meet the row size requirement uint8_t padding[3] = {0, 0, 0}; fwrite(padding, 1, row_size - (width * 3), fp); } } int main() { int width = 300; // Width of the flag int height = 200; // Height of the flag // Write to stdout write_bmp_header(stdout, width, height); write_pixel_data(stdout, width, height); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 | #include <stdio.h> #include <stdint.h> #include <string.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; // BMP file type uint32_t bfSize; // Size of the BMP file uint16_t bfReserved1; // Reserved; must be 0 uint16_t bfReserved2; // Reserved; must be 0 uint32_t bfOffBits; // Offset to start of pixel data } BMPHeader; typedef struct { uint32_t biSize; // Size of this header int32_t biWidth; // Width of the bitmap in pixels int32_t biHeight; // Height of the bitmap in pixels uint16_t biPlanes; // Number of color planes (must be 1) uint16_t biBitCount; // Number of bits per pixel uint32_t biCompression; // Type of compression to use uint32_t biSizeImage; // Size of the image data int32_t biXPelsPerMeter; // Horizontal resolution (pixels per meter) int32_t biYPelsPerMeter; // Vertical resolution (pixels per meter) uint32_t biClrUsed; // Number of colors in the color palette uint32_t biClrImportant; // Number of important colors } BMPInfoHeader; #pragma pack(pop) void write_bmp_header(FILE *fp, int width, int height) { BMPHeader bmp_header; BMPInfoHeader bmp_info_header; // Fill BMP Header bmp_header.bfType = 0x4D42; // 'BM' bmp_header.bfSize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + width * height * 3; bmp_header.bfReserved1 = 0; bmp_header.bfReserved2 = 0; bmp_header.bfOffBits = sizeof(BMPHeader) + sizeof(BMPInfoHeader); // Fill BMP Info Header bmp_info_header.biSize = sizeof(BMPInfoHeader); bmp_info_header.biWidth = width; bmp_info_header.biHeight = height; bmp_info_header.biPlanes = 1; bmp_info_header.biBitCount = 24; // 24 bits per pixel bmp_info_header.biCompression = 0; // No compression bmp_info_header.biSizeImage = 0; // No compression, so image size can be 0 bmp_info_header.biXPelsPerMeter = 0; bmp_info_header.biYPelsPerMeter = 0; bmp_info_header.biClrUsed = 0; bmp_info_header.biClrImportant = 0; // Write headers fwrite(&bmp_header, sizeof(BMPHeader), 1, fp); fwrite(&bmp_info_header, sizeof(BMPInfoHeader), 1, fp); } void write_pixel_data(FILE *fp, int width, int height) { int row_size = (3 * width + 3) & ~3; // Rows must be aligned to 4-byte boundary uint8_t green[3] = {0, 255, 0}; // Green color uint8_t white[3] = {255, 255, 255}; // White color uint8_t red[3] = {255, 0, 0}; // Red color for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t *color; if (x < width / 3) { color = green; } else if (x < 2 * width / 3) { color = white; } else { color = red; } fwrite(color, 1, 3, fp); // Write pixel color } // Fill the rest of the row to meet the row size requirement uint8_t padding[3] = {0, 0, 0}; fwrite(padding, 1, row_size - (width * 3), fp); } } int main() { int width = 300; // Width of the flag int height = 200; // Height of the flag // Write to stdout write_bmp_header(stdout, width, height); write_pixel_data(stdout, width, height); return 0; } |
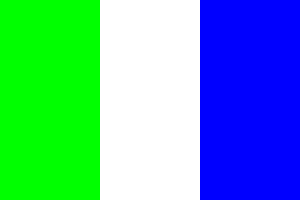
What flag is shown in this image?
The flag shown in the image is the flag of the Republic of Abkhazia. It consists of three vertical stripes of green, white, and blue.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False