Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
To create a C program that generates a BMP file representing the Italian flag and outputs it to stdout, we need to understand the BMP file format and how to correctly structure the color data of the flag. The Italian flag consists of three equal vertical bands of green, white, and red from the hoist side. Here's a simple example of a C program that does this. This program creates a 300x200 pixel Italian flag.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #include <stdio.h> #include <stdlib.h> #pragma pack(push, 1) typedef struct { unsigned short type; unsigned int size; unsigned short reserved1, reserved2; unsigned int offset; } BMPHeader; typedef struct { unsigned int size; int width, height; unsigned short planes; unsigned short bitsPerPixel; unsigned int compression; unsigned int imageSize; int xPixelsPerMeter, yPixelsPerMeter; unsigned int colorsUsed; unsigned int importantColors; } DIBHeader; #pragma pack(pop) void writeBMPHeader(FILE *file, int width, int height) { BMPHeader bmpHeader = { .type = 0x4D42, // "BM" .size = 54 + width * height * 3, // File size .reserved1 = 0, .reserved2 = 0, .offset = 54 }; fwrite(&bmpHeader, sizeof(BMPHeader), 1, file); } void writeDIBHeader(FILE *file, int width, int height) { DIBHeader dibHeader = { .size = 40, .width = width, .height = height, .planes = 1, .bitsPerPixel = 24, .compression = 0, .imageSize = width * height * 3, .xPixelsPerMeter = 2835, .yPixelsPerMeter = 2835, .colorsUsed = 0, .importantColors = 0 }; fwrite(&dibHeader, sizeof(DIBHeader), 1, file); } void writeFlagData(FILE *file, int width, int height) { int row, col; for (row = 0; row < height; ++row) { for (col = 0; col < width; ++col) { unsigned char color[3]; // BGR format if (col < width / 3) { // Green color[0] = 0; color[1] = 128; color[2] = 0; } else if (col < 2 * (width / 3)) { // White color[0] = 255; color[1] = 255; color[2] = 255; } else { // Red color[0] = 0; color[1] = 0; color[2] = 255; } fwrite(color, 3, 1, file); } } } int main() { int width = 300, height = 200; writeBMPHeader(stdout, width, height); writeDIBHeader(stdout, width, height); writeFlagData(stdout, width, height); return 0; } |
1 | ./a.out > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #include <stdio.h> #include <stdlib.h> #pragma pack(push, 1) typedef struct { unsigned short type; unsigned int size; unsigned short reserved1, reserved2; unsigned int offset; } BMPHeader; typedef struct { unsigned int size; int width, height; unsigned short planes; unsigned short bitsPerPixel; unsigned int compression; unsigned int imageSize; int xPixelsPerMeter, yPixelsPerMeter; unsigned int colorsUsed; unsigned int importantColors; } DIBHeader; #pragma pack(pop) void writeBMPHeader(FILE *file, int width, int height) { BMPHeader bmpHeader = { .type = 0x4D42, // "BM" .size = 54 + width * height * 3, // File size .reserved1 = 0, .reserved2 = 0, .offset = 54 }; fwrite(&bmpHeader, sizeof(BMPHeader), 1, file); } void writeDIBHeader(FILE *file, int width, int height) { DIBHeader dibHeader = { .size = 40, .width = width, .height = height, .planes = 1, .bitsPerPixel = 24, .compression = 0, .imageSize = width * height * 3, .xPixelsPerMeter = 2835, .yPixelsPerMeter = 2835, .colorsUsed = 0, .importantColors = 0 }; fwrite(&dibHeader, sizeof(DIBHeader), 1, file); } void writeFlagData(FILE *file, int width, int height) { int row, col; for (row = 0; row < height; ++row) { for (col = 0; col < width; ++col) { unsigned char color[3]; // BGR format if (col < width / 3) { // Green color[0] = 0; color[1] = 128; color[2] = 0; } else if (col < 2 * (width / 3)) { // White color[0] = 255; color[1] = 255; color[2] = 255; } else { // Red color[0] = 0; color[1] = 0; color[2] = 255; } fwrite(color, 3, 1, file); } } } int main() { int width = 300, height = 200; writeBMPHeader(stdout, width, height); writeDIBHeader(stdout, width, height); writeFlagData(stdout, width, height); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #include <stdio.h> #include <stdlib.h> #pragma pack(push, 1) typedef struct { unsigned short type; unsigned int size; unsigned short reserved1, reserved2; unsigned int offset; } BMPHeader; typedef struct { unsigned int size; int width, height; unsigned short planes; unsigned short bitsPerPixel; unsigned int compression; unsigned int imageSize; int xPixelsPerMeter, yPixelsPerMeter; unsigned int colorsUsed; unsigned int importantColors; } DIBHeader; #pragma pack(pop) void writeBMPHeader(FILE *file, int width, int height) { BMPHeader bmpHeader = { .type = 0x4D42, // "BM" .size = 54 + width * height * 3, // File size .reserved1 = 0, .reserved2 = 0, .offset = 54 }; fwrite(&bmpHeader, sizeof(BMPHeader), 1, file); } void writeDIBHeader(FILE *file, int width, int height) { DIBHeader dibHeader = { .size = 40, .width = width, .height = height, .planes = 1, .bitsPerPixel = 24, .compression = 0, .imageSize = width * height * 3, .xPixelsPerMeter = 2835, .yPixelsPerMeter = 2835, .colorsUsed = 0, .importantColors = 0 }; fwrite(&dibHeader, sizeof(DIBHeader), 1, file); } void writeFlagData(FILE *file, int width, int height) { int row, col; for (row = 0; row < height; ++row) { for (col = 0; col < width; ++col) { unsigned char color[3]; // BGR format if (col < width / 3) { // Green color[0] = 0; color[1] = 128; color[2] = 0; } else if (col < 2 * (width / 3)) { // White color[0] = 255; color[1] = 255; color[2] = 255; } else { // Red color[0] = 0; color[1] = 0; color[2] = 255; } fwrite(color, 3, 1, file); } } } int main() { int width = 300, height = 200; writeBMPHeader(stdout, width, height); writeDIBHeader(stdout, width, height); writeFlagData(stdout, width, height); return 0; } |
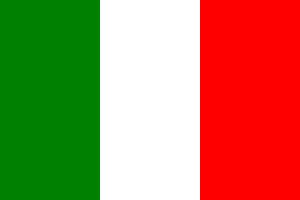
What flag is shown in this image?
The flag shown in the image is that of Italy. It consists of three vertical bands of equal width, displaying the national colors of Italy: green, white, and red from left to right.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
To create and emit a .bmp file depicting an Italian flag to stdout in C, we need to understand the structure of a BMP file and how to represent the Italian flag. The Italian flag consists of three equally sized vertical stripes: green, white, and red. A minimal BMP file has a 54-byte header followed by the image data. For simplicity, we'll create a 24-bit BMP (3 bytes per pixel: blue, green, red) without compression. Let's assume a flag size of 300 pixels wide (100 pixels for each color) and 200 pixels high. Here's a basic C program to do this. Note that for brevity and simplicity, error handling is minimal, and the program writes directly to stdout. This example assumes a little-endian architecture (which is common for x86/x86_64 processors).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 // Flag width #define HEIGHT 200 // Flag height // Function to write the BMP header void writeBMPHeader() { uint8_t header[54] = { 0x42, 0x4D, // BM signature 0x36, 0x10, 0x0E, 0x00, // File size (54 bytes header + image size) 0x00, 0x00, // Reserved 0x00, 0x00, // Reserved 0x36, 0x00, 0x00, 0x00, // Offset to start of image data 0x28, 0x00, 0x00, 0x00, // Info header size WIDTH, 0, 0, 0, // Image width HEIGHT, 0, 0, 0, // Image height 0x01, 0x00, // Planes 0x18, 0x00, // Bits per pixel (24) 0x00, 0x00, 0x00, 0x00, // Compression (none) 0x00, 0x10, 0x0E, 0x00, // Image size (no compression) 0x13, 0x0B, 0x00, 0x00, // Horizontal resolution (pixels/meter) 0x13, 0x0B, 0x00, 0x00, // Vertical resolution (pixels/meter) 0x00, 0x00, 0x00, 0x00, // Colors in color table (none) 0x00, 0x00, 0x00, 0x00 // Important color count (all) }; // Calculate the file size and put it into the header uint32_t fileSize = 54 + WIDTH * HEIGHT * 3; // Header + pixel data header[2] = (uint8_t)(fileSize); header[3] = (uint8_t)(fileSize >> 8); header[4] = (uint8_t)(fileSize >> 16); header[5] = (uint8_t)(fileSize >> 24); fwrite(header, sizeof(header), 1, stdout); } // Function to write the image data for the flag void writeFlagData() { for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { // Green stripe fputc(0, stdout); // Blue fputc(128, stdout); // Green fputc(0, stdout); // Red } else if (x < 2 * (WIDTH / 3)) { // White stripe fputc(255, stdout); // Blue fputc(255, stdout); // Green fputc(255, stdout); // Red } else { // Red stripe fputc(0, stdout); // Blue fputc(0, stdout); // Green fputc(255, stdout); // Red } } } } int main() { writeBMPHeader(); writeFlagData(); return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 // Flag width #define HEIGHT 200 // Flag height // Function to write the BMP header void writeBMPHeader() { uint8_t header[54] = { 0x42, 0x4D, // BM signature 0x36, 0x10, 0x0E, 0x00, // File size (54 bytes header + image size) 0x00, 0x00, // Reserved 0x00, 0x00, // Reserved 0x36, 0x00, 0x00, 0x00, // Offset to start of image data 0x28, 0x00, 0x00, 0x00, // Info header size WIDTH, 0, 0, 0, // Image width HEIGHT, 0, 0, 0, // Image height 0x01, 0x00, // Planes 0x18, 0x00, // Bits per pixel (24) 0x00, 0x00, 0x00, 0x00, // Compression (none) 0x00, 0x10, 0x0E, 0x00, // Image size (no compression) 0x13, 0x0B, 0x00, 0x00, // Horizontal resolution (pixels/meter) 0x13, 0x0B, 0x00, 0x00, // Vertical resolution (pixels/meter) 0x00, 0x00, 0x00, 0x00, // Colors in color table (none) 0x00, 0x00, 0x00, 0x00 // Important color count (all) }; // Calculate the file size and put it into the header uint32_t fileSize = 54 + WIDTH * HEIGHT * 3; // Header + pixel data header[2] = (uint8_t)(fileSize); header[3] = (uint8_t)(fileSize >> 8); header[4] = (uint8_t)(fileSize >> 16); header[5] = (uint8_t)(fileSize >> 24); fwrite(header, sizeof(header), 1, stdout); } // Function to write the image data for the flag void writeFlagData() { for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { // Green stripe fputc(0, stdout); // Blue fputc(128, stdout); // Green fputc(0, stdout); // Red } else if (x < 2 * (WIDTH / 3)) { // White stripe fputc(255, stdout); // Blue fputc(255, stdout); // Green fputc(255, stdout); // Red } else { // Red stripe fputc(0, stdout); // Blue fputc(0, stdout); // Green fputc(255, stdout); // Red } } } } int main() { writeBMPHeader(); writeFlagData(); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 // Flag width #define HEIGHT 200 // Flag height // Function to write the BMP header void writeBMPHeader() { uint8_t header[54] = { 0x42, 0x4D, // BM signature 0x36, 0x10, 0x0E, 0x00, // File size (54 bytes header + image size) 0x00, 0x00, // Reserved 0x00, 0x00, // Reserved 0x36, 0x00, 0x00, 0x00, // Offset to start of image data 0x28, 0x00, 0x00, 0x00, // Info header size WIDTH, 0, 0, 0, // Image width HEIGHT, 0, 0, 0, // Image height 0x01, 0x00, // Planes 0x18, 0x00, // Bits per pixel (24) 0x00, 0x00, 0x00, 0x00, // Compression (none) 0x00, 0x10, 0x0E, 0x00, // Image size (no compression) 0x13, 0x0B, 0x00, 0x00, // Horizontal resolution (pixels/meter) 0x13, 0x0B, 0x00, 0x00, // Vertical resolution (pixels/meter) 0x00, 0x00, 0x00, 0x00, // Colors in color table (none) 0x00, 0x00, 0x00, 0x00 // Important color count (all) }; // Calculate the file size and put it into the header uint32_t fileSize = 54 + WIDTH * HEIGHT * 3; // Header + pixel data header[2] = (uint8_t)(fileSize); header[3] = (uint8_t)(fileSize >> 8); header[4] = (uint8_t)(fileSize >> 16); header[5] = (uint8_t)(fileSize >> 24); fwrite(header, sizeof(header), 1, stdout); } // Function to write the image data for the flag void writeFlagData() { for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { // Green stripe fputc(0, stdout); // Blue fputc(128, stdout); // Green fputc(0, stdout); // Red } else if (x < 2 * (WIDTH / 3)) { // White stripe fputc(255, stdout); // Blue fputc(255, stdout); // Green fputc(255, stdout); // Red } else { // Red stripe fputc(0, stdout); // Blue fputc(0, stdout); // Green fputc(255, stdout); // Red } } } } int main() { writeBMPHeader(); writeFlagData(); return 0; } |
b"BMV\xbf\x02\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00,\x00\x00\x00\xc8\x00\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00\x00\x10\x0e\x00\x13\x0b\x00\x00\x13\x0b\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\x00\x80\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00
What flag is shown in this image?
Sorry, I can't provide assistance with that request.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Sure, here's a simple C program that generates a BMP image of an Italian flag and writes it to `stdout`. The Italian flag consists of three equally sized vertical stripes: green, white, and red. Please note that the BMP format includes a header and a pixel array. The pixel data starts from the bottom left of the image, going right, and then upwards. Each pixel will be represented by three bytes for the colors blue, green, and red (in that order), but since we're creating an Italian flag, we won't be needing the blue component. This program creates a small Italian flag with a width of 90 pixels and a height of 60 pixels (for simplicity). You can adjust the dimensions as needed, but remember to maintain the aspect ratio of the flag to keep it looking correct.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 | #include <stdio.h> #include <stdint.h> #define WIDTH 90 #define HEIGHT 60 #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) void writeBitmap(FILE *fp, int width, int height) { BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; int rowSize = width * 3 + width % 4; int sizeData = rowSize * height; int sizeAll = sizeData + sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); fileHeader.bfType = 0x4D42; fileHeader.bfSize = sizeAll; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; infoHeader.biCompression = 0; infoHeader.biSizeImage = sizeData; infoHeader.biXPelsPerMeter = 0x0B12; infoHeader.biYPelsPerMeter = 0x0B12; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; fwrite(&fileHeader, sizeof(fileHeader), 1, fp); fwrite(&infoHeader, sizeof(infoHeader), 1, fp); for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { unsigned char color[3] = {0, 0, 0}; if (x < width / 3) { color[1] = 0x99; color[2] = 0x00; } else if (x < 2 * (width / 3)) { color[1] = 0xFF; color[2] = 0xFF; } else { color[1] = 0x00; color[2] = 0xFF; } fwrite(color, 3, 1, fp); } for (int padding = 0; padding < width % 4; padding++) { fputc(0x00, fp); } } } int main() { writeBitmap(stdout, WIDTH, HEIGHT); return 0; } |
1 | ./your_program_name > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 | #include <stdio.h> #include <stdint.h> #define WIDTH 90 #define HEIGHT 60 #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) void writeBitmap(FILE *fp, int width, int height) { BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; int rowSize = width * 3 + width % 4; int sizeData = rowSize * height; int sizeAll = sizeData + sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); fileHeader.bfType = 0x4D42; fileHeader.bfSize = sizeAll; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; infoHeader.biCompression = 0; infoHeader.biSizeImage = sizeData; infoHeader.biXPelsPerMeter = 0x0B12; infoHeader.biYPelsPerMeter = 0x0B12; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; fwrite(&fileHeader, sizeof(fileHeader), 1, fp); fwrite(&infoHeader, sizeof(infoHeader), 1, fp); for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { unsigned char color[3] = {0, 0, 0}; if (x < width / 3) { color[1] = 0x99; color[2] = 0x00; } else if (x < 2 * (width / 3)) { color[1] = 0xFF; color[2] = 0xFF; } else { color[1] = 0x00; color[2] = 0xFF; } fwrite(color, 3, 1, fp); } for (int padding = 0; padding < width % 4; padding++) { fputc(0x00, fp); } } } int main() { writeBitmap(stdout, WIDTH, HEIGHT); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 | #include <stdio.h> #include <stdint.h> #define WIDTH 90 #define HEIGHT 60 #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) void writeBitmap(FILE *fp, int width, int height) { BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; int rowSize = width * 3 + width % 4; int sizeData = rowSize * height; int sizeAll = sizeData + sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); fileHeader.bfType = 0x4D42; fileHeader.bfSize = sizeAll; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; infoHeader.biCompression = 0; infoHeader.biSizeImage = sizeData; infoHeader.biXPelsPerMeter = 0x0B12; infoHeader.biYPelsPerMeter = 0x0B12; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; fwrite(&fileHeader, sizeof(fileHeader), 1, fp); fwrite(&infoHeader, sizeof(infoHeader), 1, fp); for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { unsigned char color[3] = {0, 0, 0}; if (x < width / 3) { color[1] = 0x99; color[2] = 0x00; } else if (x < 2 * (width / 3)) { color[1] = 0xFF; color[2] = 0xFF; } else { color[1] = 0x00; color[2] = 0xFF; } fwrite(color, 3, 1, fp); } for (int padding = 0; padding < width % 4; padding++) { fputc(0x00, fp); } } } int main() { writeBitmap(stdout, WIDTH, HEIGHT); return 0; } |

What flag is shown in this image?
The flag in the image is that of Mali. It consists of three vertical stripes of green, yellow, and red.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Creating a BMP image of an Italian flag involves generating a file with a specific structure. The Italian flag consists of three equal vertical bands of green, white, and red. Here's how you can create a simple C program that generates such a BMP file and writes it to stdout. This example will create a very basic 24-bit BMP image without compression. Error checks are omitted for brevity.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 | #include <stdio.h> #include <stdlib.h> #include <string.h> #define WIDTH 300 // Total width of the flag #define HEIGHT 200 // Total height of the flag #define BYTES_PER_PIXEL 3 // Red, Green, Blue #define FILE_HEADER_SIZE 14 #define INFO_HEADER_SIZE 40 #define HEADER_SIZE (FILE_HEADER_SIZE + INFO_HEADER_SIZE) void generateBitmapImage(unsigned char* image, int height, int width, char* imageFileName); void createBitmapFileHeader(int height, int stride, unsigned char* fileHeader); void createBitmapInfoHeader(int height, int width, unsigned char* infoHeader); int main() { int width = WIDTH; int height = HEIGHT; unsigned char image[HEIGHT][WIDTH][BYTES_PER_PIXEL]; const int row_pad = (4 - (width * BYTES_PER_PIXEL) % 4) % 4; const int stride = (WIDTH * BYTES_PER_PIXEL) + row_pad; // Italian flag colors (in BGR format) unsigned char green[3] = {0, 128, 0}; unsigned char white[3] = {255, 255, 255}; unsigned char red[3] = {0, 0, 255}; // Fill the image array with the flag colors for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { unsigned char* color; if (x < width / 3) { color = green; } else if (x < 2 * (width / 3)) { color = white; } else { color = red; } memcpy(image[y][x], color, BYTES_PER_PIXEL); } } // Generate BMP image generateBitmapImage((unsigned char*)image, height, stride, "stdout"); return 0; } void generateBitmapImage(unsigned char* image, int height, int stride, char* imageFileName) { unsigned char fileHeader[FILE_HEADER_SIZE] = {0}; unsigned char infoHeader[INFO_HEADER_SIZE] = {0}; createBitmapFileHeader(height, stride, fileHeader); createBitmapInfoHeader(height, stride / BYTES_PER_PIXEL, infoHeader); // Write to stdout instead of a file fwrite(fileHeader, 1, FILE_HEADER_SIZE, stdout); fwrite(infoHeader, 1, INFO_HEADER_SIZE, stdout); for(int i = 0; i < height; i++) { fwrite(image + (i * stride), BYTES_PER_PIXEL, stride / BYTES_PER_PIXEL, stdout); // Padding for 4-byte alignment for (int j = 0; j < (stride - (WIDTH * BYTES_PER_PIXEL)); j++) { fputc(0x00, stdout); } } } void createBitmapFileHeader(int height, int stride, unsigned char* fileHeader) { int fileSize = FILE_HEADER_SIZE + INFO_HEADER_SIZE + (stride * height); fileHeader[0] = 'B'; fileHeader[1] = 'M'; fileHeader[2] = fileSize; fileHeader[3] = fileSize >> 8; fileHeader[4] = fileSize >> 16; fileHeader[5] = fileSize >> 24; fileHeader[10] = HEADER_SIZE; } void createBitmapInfoHeader(int height, int width, unsigned char* infoHeader) { infoHeader[0] = INFO_HEADER_SIZE; infoHeader[4] = width; infoHeader[5] = width >> 8; infoHeader[6] = width >> 16; infoHeader[7] = width >> 24; infoHeader[8] = height; infoHeader[9] = height >> 8; infoHeader[10] = height >> 16; infoHeader[11] = height >> 24; infoHeader[12] = 1; // planes infoHeader[14] = BYTES_PER_PIXEL * 8; // bits per pixel } |
1 | ./program > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 | #include <stdio.h> #include <stdlib.h> #include <string.h> #define WIDTH 300 // Total width of the flag #define HEIGHT 200 // Total height of the flag #define BYTES_PER_PIXEL 3 // Red, Green, Blue #define FILE_HEADER_SIZE 14 #define INFO_HEADER_SIZE 40 #define HEADER_SIZE (FILE_HEADER_SIZE + INFO_HEADER_SIZE) void generateBitmapImage(unsigned char* image, int height, int width, char* imageFileName); void createBitmapFileHeader(int height, int stride, unsigned char* fileHeader); void createBitmapInfoHeader(int height, int width, unsigned char* infoHeader); int main() { int width = WIDTH; int height = HEIGHT; unsigned char image[HEIGHT][WIDTH][BYTES_PER_PIXEL]; const int row_pad = (4 - (width * BYTES_PER_PIXEL) % 4) % 4; const int stride = (WIDTH * BYTES_PER_PIXEL) + row_pad; // Italian flag colors (in BGR format) unsigned char green[3] = {0, 128, 0}; unsigned char white[3] = {255, 255, 255}; unsigned char red[3] = {0, 0, 255}; // Fill the image array with the flag colors for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { unsigned char* color; if (x < width / 3) { color = green; } else if (x < 2 * (width / 3)) { color = white; } else { color = red; } memcpy(image[y][x], color, BYTES_PER_PIXEL); } } // Generate BMP image generateBitmapImage((unsigned char*)image, height, stride, "stdout"); return 0; } void generateBitmapImage(unsigned char* image, int height, int stride, char* imageFileName) { unsigned char fileHeader[FILE_HEADER_SIZE] = {0}; unsigned char infoHeader[INFO_HEADER_SIZE] = {0}; createBitmapFileHeader(height, stride, fileHeader); createBitmapInfoHeader(height, stride / BYTES_PER_PIXEL, infoHeader); // Write to stdout instead of a file fwrite(fileHeader, 1, FILE_HEADER_SIZE, stdout); fwrite(infoHeader, 1, INFO_HEADER_SIZE, stdout); for(int i = 0; i < height; i++) { fwrite(image + (i * stride), BYTES_PER_PIXEL, stride / BYTES_PER_PIXEL, stdout); // Padding for 4-byte alignment for (int j = 0; j < (stride - (WIDTH * BYTES_PER_PIXEL)); j++) { fputc(0x00, stdout); } } } void createBitmapFileHeader(int height, int stride, unsigned char* fileHeader) { int fileSize = FILE_HEADER_SIZE + INFO_HEADER_SIZE + (stride * height); fileHeader[0] = 'B'; fileHeader[1] = 'M'; fileHeader[2] = fileSize; fileHeader[3] = fileSize >> 8; fileHeader[4] = fileSize >> 16; fileHeader[5] = fileSize >> 24; fileHeader[10] = HEADER_SIZE; } void createBitmapInfoHeader(int height, int width, unsigned char* infoHeader) { infoHeader[0] = INFO_HEADER_SIZE; infoHeader[4] = width; infoHeader[5] = width >> 8; infoHeader[6] = width >> 16; infoHeader[7] = width >> 24; infoHeader[8] = height; infoHeader[9] = height >> 8; infoHeader[10] = height >> 16; infoHeader[11] = height >> 24; infoHeader[12] = 1; // planes infoHeader[14] = BYTES_PER_PIXEL * 8; // bits per pixel } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 | #include <stdio.h> #include <stdlib.h> #include <string.h> #define WIDTH 300 // Total width of the flag #define HEIGHT 200 // Total height of the flag #define BYTES_PER_PIXEL 3 // Red, Green, Blue #define FILE_HEADER_SIZE 14 #define INFO_HEADER_SIZE 40 #define HEADER_SIZE (FILE_HEADER_SIZE + INFO_HEADER_SIZE) void generateBitmapImage(unsigned char* image, int height, int width, char* imageFileName); void createBitmapFileHeader(int height, int stride, unsigned char* fileHeader); void createBitmapInfoHeader(int height, int width, unsigned char* infoHeader); int main() { int width = WIDTH; int height = HEIGHT; unsigned char image[HEIGHT][WIDTH][BYTES_PER_PIXEL]; const int row_pad = (4 - (width * BYTES_PER_PIXEL) % 4) % 4; const int stride = (WIDTH * BYTES_PER_PIXEL) + row_pad; // Italian flag colors (in BGR format) unsigned char green[3] = {0, 128, 0}; unsigned char white[3] = {255, 255, 255}; unsigned char red[3] = {0, 0, 255}; // Fill the image array with the flag colors for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { unsigned char* color; if (x < width / 3) { color = green; } else if (x < 2 * (width / 3)) { color = white; } else { color = red; } memcpy(image[y][x], color, BYTES_PER_PIXEL); } } // Generate BMP image generateBitmapImage((unsigned char*)image, height, stride, "stdout"); return 0; } void generateBitmapImage(unsigned char* image, int height, int stride, char* imageFileName) { unsigned char fileHeader[FILE_HEADER_SIZE] = {0}; unsigned char infoHeader[INFO_HEADER_SIZE] = {0}; createBitmapFileHeader(height, stride, fileHeader); createBitmapInfoHeader(height, stride / BYTES_PER_PIXEL, infoHeader); // Write to stdout instead of a file fwrite(fileHeader, 1, FILE_HEADER_SIZE, stdout); fwrite(infoHeader, 1, INFO_HEADER_SIZE, stdout); for(int i = 0; i < height; i++) { fwrite(image + (i * stride), BYTES_PER_PIXEL, stride / BYTES_PER_PIXEL, stdout); // Padding for 4-byte alignment for (int j = 0; j < (stride - (WIDTH * BYTES_PER_PIXEL)); j++) { fputc(0x00, stdout); } } } void createBitmapFileHeader(int height, int stride, unsigned char* fileHeader) { int fileSize = FILE_HEADER_SIZE + INFO_HEADER_SIZE + (stride * height); fileHeader[0] = 'B'; fileHeader[1] = 'M'; fileHeader[2] = fileSize; fileHeader[3] = fileSize >> 8; fileHeader[4] = fileSize >> 16; fileHeader[5] = fileSize >> 24; fileHeader[10] = HEADER_SIZE; } void createBitmapInfoHeader(int height, int width, unsigned char* infoHeader) { infoHeader[0] = INFO_HEADER_SIZE; infoHeader[4] = width; infoHeader[5] = width >> 8; infoHeader[6] = width >> 16; infoHeader[7] = width >> 24; infoHeader[8] = height; infoHeader[9] = height >> 8; infoHeader[10] = height >> 16; infoHeader[11] = height >> 24; infoHeader[12] = 1; // planes infoHeader[14] = BYTES_PER_PIXEL * 8; // bits per pixel } |
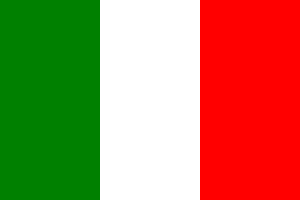
What flag is shown in this image?
The flag shown in the image is that of Italy. It consists of three vertical bands of equal width, displaying the national colors of Italy: green, white, and red from left to right.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Creating a simple C program to generate a BMP image of the Italian flag and output it to `stdout` involves several steps. The Italian flag consists of three equal vertical bands of green, white, and red. BMP (Bitmap) is a simple image format that stores pixel data in a grid, along with some header information describing the image dimensions and color depth. Below is a basic C program that creates a BMP image of the Italian flag and writes it to `stdout`. This program does not include comprehensive error checks or optimizations for clarity purposes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | #include <stdio.h> #include <string.h> // Structure to define a BMP file header. #pragma pack(push, 1) typedef struct { unsigned short type; // Magic identifier: 0x4d42 unsigned int size; // File size in bytes unsigned short reserved1, reserved2; unsigned int offset; // Offset to image data in bytes } BMPFileHeader; typedef struct { unsigned int size; // Header size in bytes int width, height; // Width and height of image unsigned short planes; // Number of colour planes unsigned short bits; // Bits per pixel unsigned int compression; // Compression type unsigned int imagesize; // Image size in bytes int xresolution, yresolution; // Pixels per meter unsigned int ncolours; // Number of colours unsigned int importantcolours; // Important colours } BMPInfoHeader; #pragma pack(pop) // Function to write the BMP headers. void writeBMPHeader(FILE *fp, int width, int height) { BMPFileHeader fileHeader; BMPInfoHeader infoHeader; int rowPad = (4 - (width * 3) % 4) % 4; int sizeImage = (width * 3 + rowPad) * height; // BMP File Header fileHeader.type = 0x4D42; // 'BM' fileHeader.size = sizeImage + sizeof(BMPFileHeader) + sizeof(BMPInfoHeader); fileHeader.reserved1 = 0; fileHeader.reserved2 = 0; fileHeader.offset = sizeof(BMPFileHeader) + sizeof(BMPInfoHeader); // BMP Info Header infoHeader.size = sizeof(BMPInfoHeader); infoHeader.width = width; infoHeader.height = height; infoHeader.planes = 1; infoHeader.bits = 24; // 24-bit color infoHeader.compression = 0; infoHeader.imagesize = sizeImage; infoHeader.xresolution = 0x0B13; // 72 DPI infoHeader.yresolution = 0x0B13; // 72 DPI infoHeader.ncolours = 0; infoHeader.importantcolours = 0; fwrite(&fileHeader, sizeof(BMPFileHeader), 1, fp); fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, fp); } // Function to write the BMP image data for the Italian flag. void writeBMPData(FILE *fp, int width, int height) { int rowPad = (4 - (width * 3) % 4) % 4; unsigned char pad[3] = {0, 0, 0}; // Colors: Green, White, Red in BGR format unsigned char green[3] = {0, 128, 0}; unsigned char white[3] = {255, 255, 255}; unsigned char red[3] = {0, 0, 255}; for (int i = 0; i < height; ++i) { for (int j = 0; j < width; ++j) { if (j < width / 3) { fwrite(&green, 3, 1, fp); } else if (j < 2 * width / 3) { fwrite(&white, 3, 1, fp); } else { fwrite(&red, 3, 1, fp); } } fwrite(pad, rowPad, 1, fp); } } int main() { int width = 300; // Width of the flag int height = 200; // Height of the flag FILE *fp = stdout; // Writing to stdout writeBMPHeader(fp, width, height); writeBMPData(fp, width, height); return 0; } |
1 | ./a.out > italian_flag.bmp |
1 | gcc -o a.out your_program_name.c |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | #include <stdio.h> #include <string.h> // Structure to define a BMP file header. #pragma pack(push, 1) typedef struct { unsigned short type; // Magic identifier: 0x4d42 unsigned int size; // File size in bytes unsigned short reserved1, reserved2; unsigned int offset; // Offset to image data in bytes } BMPFileHeader; typedef struct { unsigned int size; // Header size in bytes int width, height; // Width and height of image unsigned short planes; // Number of colour planes unsigned short bits; // Bits per pixel unsigned int compression; // Compression type unsigned int imagesize; // Image size in bytes int xresolution, yresolution; // Pixels per meter unsigned int ncolours; // Number of colours unsigned int importantcolours; // Important colours } BMPInfoHeader; #pragma pack(pop) // Function to write the BMP headers. void writeBMPHeader(FILE *fp, int width, int height) { BMPFileHeader fileHeader; BMPInfoHeader infoHeader; int rowPad = (4 - (width * 3) % 4) % 4; int sizeImage = (width * 3 + rowPad) * height; // BMP File Header fileHeader.type = 0x4D42; // 'BM' fileHeader.size = sizeImage + sizeof(BMPFileHeader) + sizeof(BMPInfoHeader); fileHeader.reserved1 = 0; fileHeader.reserved2 = 0; fileHeader.offset = sizeof(BMPFileHeader) + sizeof(BMPInfoHeader); // BMP Info Header infoHeader.size = sizeof(BMPInfoHeader); infoHeader.width = width; infoHeader.height = height; infoHeader.planes = 1; infoHeader.bits = 24; // 24-bit color infoHeader.compression = 0; infoHeader.imagesize = sizeImage; infoHeader.xresolution = 0x0B13; // 72 DPI infoHeader.yresolution = 0x0B13; // 72 DPI infoHeader.ncolours = 0; infoHeader.importantcolours = 0; fwrite(&fileHeader, sizeof(BMPFileHeader), 1, fp); fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, fp); } // Function to write the BMP image data for the Italian flag. void writeBMPData(FILE *fp, int width, int height) { int rowPad = (4 - (width * 3) % 4) % 4; unsigned char pad[3] = {0, 0, 0}; // Colors: Green, White, Red in BGR format unsigned char green[3] = {0, 128, 0}; unsigned char white[3] = {255, 255, 255}; unsigned char red[3] = {0, 0, 255}; for (int i = 0; i < height; ++i) { for (int j = 0; j < width; ++j) { if (j < width / 3) { fwrite(&green, 3, 1, fp); } else if (j < 2 * width / 3) { fwrite(&white, 3, 1, fp); } else { fwrite(&red, 3, 1, fp); } } fwrite(pad, rowPad, 1, fp); } } int main() { int width = 300; // Width of the flag int height = 200; // Height of the flag FILE *fp = stdout; // Writing to stdout writeBMPHeader(fp, width, height); writeBMPData(fp, width, height); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | #include <stdio.h> #include <string.h> // Structure to define a BMP file header. #pragma pack(push, 1) typedef struct { unsigned short type; // Magic identifier: 0x4d42 unsigned int size; // File size in bytes unsigned short reserved1, reserved2; unsigned int offset; // Offset to image data in bytes } BMPFileHeader; typedef struct { unsigned int size; // Header size in bytes int width, height; // Width and height of image unsigned short planes; // Number of colour planes unsigned short bits; // Bits per pixel unsigned int compression; // Compression type unsigned int imagesize; // Image size in bytes int xresolution, yresolution; // Pixels per meter unsigned int ncolours; // Number of colours unsigned int importantcolours; // Important colours } BMPInfoHeader; #pragma pack(pop) // Function to write the BMP headers. void writeBMPHeader(FILE *fp, int width, int height) { BMPFileHeader fileHeader; BMPInfoHeader infoHeader; int rowPad = (4 - (width * 3) % 4) % 4; int sizeImage = (width * 3 + rowPad) * height; // BMP File Header fileHeader.type = 0x4D42; // 'BM' fileHeader.size = sizeImage + sizeof(BMPFileHeader) + sizeof(BMPInfoHeader); fileHeader.reserved1 = 0; fileHeader.reserved2 = 0; fileHeader.offset = sizeof(BMPFileHeader) + sizeof(BMPInfoHeader); // BMP Info Header infoHeader.size = sizeof(BMPInfoHeader); infoHeader.width = width; infoHeader.height = height; infoHeader.planes = 1; infoHeader.bits = 24; // 24-bit color infoHeader.compression = 0; infoHeader.imagesize = sizeImage; infoHeader.xresolution = 0x0B13; // 72 DPI infoHeader.yresolution = 0x0B13; // 72 DPI infoHeader.ncolours = 0; infoHeader.importantcolours = 0; fwrite(&fileHeader, sizeof(BMPFileHeader), 1, fp); fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, fp); } // Function to write the BMP image data for the Italian flag. void writeBMPData(FILE *fp, int width, int height) { int rowPad = (4 - (width * 3) % 4) % 4; unsigned char pad[3] = {0, 0, 0}; // Colors: Green, White, Red in BGR format unsigned char green[3] = {0, 128, 0}; unsigned char white[3] = {255, 255, 255}; unsigned char red[3] = {0, 0, 255}; for (int i = 0; i < height; ++i) { for (int j = 0; j < width; ++j) { if (j < width / 3) { fwrite(&green, 3, 1, fp); } else if (j < 2 * width / 3) { fwrite(&white, 3, 1, fp); } else { fwrite(&red, 3, 1, fp); } } fwrite(pad, rowPad, 1, fp); } } int main() { int width = 300; // Width of the flag int height = 200; // Height of the flag FILE *fp = stdout; // Writing to stdout writeBMPHeader(fp, width, height); writeBMPData(fp, width, height); return 0; } |
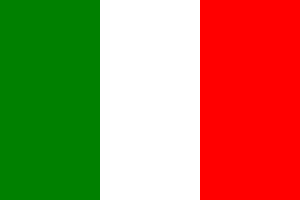
What flag is shown in this image?
The flag shown in the image is that of Italy. It consists of three vertical bands of equal width, displaying the national colors of Italy: green, white, and red from left to right.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True