Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> typedef struct { uint8_t blue; uint8_t green; uint8_t red; } pixel_t; typedef struct { uint32_t filesize; uint32_t reserved; uint32_t data_offset; uint32_t header_size; int32_t width; int32_t height; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; int32_t x_resolution; int32_t y_resolution; uint32_t num_colors; uint32_t important_colors; } bmp_header_t; int main() { const int width = 640; const int height = 480; const int num_pixels = width * height; pixel_t *pixels = malloc(num_pixels * sizeof(pixel_t)); for (int i = 0; i < height; i++) { for (int j = 0; j < width; j++) { if (i < height/3) { pixels[i * width + j] = (pixel_t){0, 0, 255}; } else if (i < 2*height/3) { pixels[i * width + j] = (pixel_t){255, 255, 255}; } else { pixels[i * width + j] = (pixel_t){255, 0, 0}; } } } bmp_header_t header = { .filesize = sizeof(bmp_header_t) + num_pixels * sizeof(pixel_t), .reserved = 0, .data_offset = sizeof(bmp_header_t), .header_size = sizeof(bmp_header_t), .width = width, .height = height, .num_planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = num_pixels * sizeof(pixel_t), .x_resolution = 0, .y_resolution = 0, .num_colors = 0, .important_colors = 0, }; fwrite(&header, sizeof(bmp_header_t), 1, stdout); fwrite(pixels, sizeof(pixel_t), num_pixels, stdout); free(pixels); return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> typedef struct { uint8_t blue; uint8_t green; uint8_t red; } pixel_t; typedef struct { uint32_t filesize; uint32_t reserved; uint32_t data_offset; uint32_t header_size; int32_t width; int32_t height; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; int32_t x_resolution; int32_t y_resolution; uint32_t num_colors; uint32_t important_colors; } bmp_header_t; int main() { const int width = 640; const int height = 480; const int num_pixels = width * height; pixel_t *pixels = malloc(num_pixels * sizeof(pixel_t)); for (int i = 0; i < height; i++) { for (int j = 0; j < width; j++) { if (i < height/3) { pixels[i * width + j] = (pixel_t){0, 0, 255}; } else if (i < 2*height/3) { pixels[i * width + j] = (pixel_t){255, 255, 255}; } else { pixels[i * width + j] = (pixel_t){255, 0, 0}; } } } bmp_header_t header = { .filesize = sizeof(bmp_header_t) + num_pixels * sizeof(pixel_t), .reserved = 0, .data_offset = sizeof(bmp_header_t), .header_size = sizeof(bmp_header_t), .width = width, .height = height, .num_planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = num_pixels * sizeof(pixel_t), .x_resolution = 0, .y_resolution = 0, .num_colors = 0, .important_colors = 0, }; fwrite(&header, sizeof(bmp_header_t), 1, stdout); fwrite(pixels, sizeof(pixel_t), num_pixels, stdout); free(pixels); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 | #include <stdio.h> #include <stdlib.h> #include <stdint.h> typedef struct { uint8_t blue; uint8_t green; uint8_t red; } pixel_t; typedef struct { uint32_t filesize; uint32_t reserved; uint32_t data_offset; uint32_t header_size; int32_t width; int32_t height; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; int32_t x_resolution; int32_t y_resolution; uint32_t num_colors; uint32_t important_colors; } bmp_header_t; int main() { const int width = 640; const int height = 480; const int num_pixels = width * height; pixel_t *pixels = malloc(num_pixels * sizeof(pixel_t)); for (int i = 0; i < height; i++) { for (int j = 0; j < width; j++) { if (i < height/3) { pixels[i * width + j] = (pixel_t){0, 0, 255}; } else if (i < 2*height/3) { pixels[i * width + j] = (pixel_t){255, 255, 255}; } else { pixels[i * width + j] = (pixel_t){255, 0, 0}; } } } bmp_header_t header = { .filesize = sizeof(bmp_header_t) + num_pixels * sizeof(pixel_t), .reserved = 0, .data_offset = sizeof(bmp_header_t), .header_size = sizeof(bmp_header_t), .width = width, .height = height, .num_planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = num_pixels * sizeof(pixel_t), .x_resolution = 0, .y_resolution = 0, .num_colors = 0, .important_colors = 0, }; fwrite(&header, sizeof(bmp_header_t), 1, stdout); fwrite(pixels, sizeof(pixel_t), num_pixels, stdout); free(pixels); return 0; } |
b'4\x10\x0e\x00\x00\x00\x00\x004\x00\x00\x004\x00\x00\x00\x80\x02\x00\x00\xe0\x01\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00\x00\x10\x0e\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x0
What flag is shown in this image?
cannot identify image file <_io.BytesIO object at 0x7f6879cb32c0>
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 | #include <stdio.h> #include <stdlib.h> int main() { // Define the dimensions of the flag int width = 640; int height = 480; // Create a buffer to store the image data unsigned char *buffer = malloc(width * height * 3); // Set the background color to white for (int i = 0; i < width * height * 3; i++) { buffer[i] = 255; } // Draw the green stripe for (int i = 0; i < width * height / 3; i++) { buffer[(i * 3) + 1] = 0; } // Draw the white stripe for (int i = width * height / 3; i < width * height * 2 / 3; i++) { buffer[(i * 3) + 2] = 255; } // Draw the red stripe for (int i = width * height * 2 / 3; i < width * height; i++) { buffer[(i * 3) + 0] = 255; } // Write the image data to a .bmp file FILE *fp = fopen("italian_flag.bmp", "wb"); fprintf(fp, "BM"); fprintf(fp, "%c%c%c%c", 0, 0, 0, 54); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 40); fprintf(fp, "%c%c%c%c", 0, 0, 0, width); fprintf(fp, "%c%c%c%c", 0, 0, 0, height); fprintf(fp, "%c%c", 0, 1); fprintf(fp, "%c%c", 0, 24); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); for (int i = 0; i < width * height; i++) { fprintf(fp, "%c%c%c", buffer[(i * 3) + 2], buffer[(i * 3) + 1], buffer[(i * 3) + 0]); } fclose(fp); return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 | #include <stdio.h> #include <stdlib.h> int main() { // Define the dimensions of the flag int width = 640; int height = 480; // Create a buffer to store the image data unsigned char *buffer = malloc(width * height * 3); // Set the background color to white for (int i = 0; i < width * height * 3; i++) { buffer[i] = 255; } // Draw the green stripe for (int i = 0; i < width * height / 3; i++) { buffer[(i * 3) + 1] = 0; } // Draw the white stripe for (int i = width * height / 3; i < width * height * 2 / 3; i++) { buffer[(i * 3) + 2] = 255; } // Draw the red stripe for (int i = width * height * 2 / 3; i < width * height; i++) { buffer[(i * 3) + 0] = 255; } // Write the image data to a .bmp file FILE *fp = fopen("italian_flag.bmp", "wb"); fprintf(fp, "BM"); fprintf(fp, "%c%c%c%c", 0, 0, 0, 54); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 40); fprintf(fp, "%c%c%c%c", 0, 0, 0, width); fprintf(fp, "%c%c%c%c", 0, 0, 0, height); fprintf(fp, "%c%c", 0, 1); fprintf(fp, "%c%c", 0, 24); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); for (int i = 0; i < width * height; i++) { fprintf(fp, "%c%c%c", buffer[(i * 3) + 2], buffer[(i * 3) + 1], buffer[(i * 3) + 0]); } fclose(fp); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 | #include <stdio.h> #include <stdlib.h> int main() { // Define the dimensions of the flag int width = 640; int height = 480; // Create a buffer to store the image data unsigned char *buffer = malloc(width * height * 3); // Set the background color to white for (int i = 0; i < width * height * 3; i++) { buffer[i] = 255; } // Draw the green stripe for (int i = 0; i < width * height / 3; i++) { buffer[(i * 3) + 1] = 0; } // Draw the white stripe for (int i = width * height / 3; i < width * height * 2 / 3; i++) { buffer[(i * 3) + 2] = 255; } // Draw the red stripe for (int i = width * height * 2 / 3; i < width * height; i++) { buffer[(i * 3) + 0] = 255; } // Write the image data to a .bmp file FILE *fp = fopen("italian_flag.bmp", "wb"); fprintf(fp, "BM"); fprintf(fp, "%c%c%c%c", 0, 0, 0, 54); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 40); fprintf(fp, "%c%c%c%c", 0, 0, 0, width); fprintf(fp, "%c%c%c%c", 0, 0, 0, height); fprintf(fp, "%c%c", 0, 1); fprintf(fp, "%c%c", 0, 24); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); fprintf(fp, "%c%c%c%c", 0, 0, 0, 0); for (int i = 0; i < width * height; i++) { fprintf(fp, "%c%c%c", buffer[(i * 3) + 2], buffer[(i * 3) + 1], buffer[(i * 3) + 0]); } fclose(fp); return 0; } |
b''
What flag is shown in this image?
cannot identify image file <_io.BytesIO object at 0x7f9bc44b45e0>
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | #include <stdio.h> int main() { // Header printf("BM"); // Magic number printf("%c%c%c%c", 54, 0, 0, 0); // File size (dummy) printf("%c%c", 0, 0); // Reserved (always 0) printf("%c%c", 54, 0); // Offset to image data // DIB header printf("%c%c%c%c", 40, 0, 0, 0); // DIB header size printf("%c%c", 22, 0); // Width printf("%c%c", 11, 0); // Height (negative indicates top-down) printf("%c%c", 1, 0); // Number of color planes printf("%c%c", 24, 0); // Bits per pixel printf("%c%c%c%c", 0, 0, 0, 0); // Compression (none) printf("%c%c%c%c", 0, 0, 0, 0); // Image size (dummy) printf("%c%c", 0, 0); // Horizontal resolution (dummy) printf("%c%c", 0, 0); // Vertical resolution (dummy) printf("%c%c%c%c", 0, 0, 0, 0); // Number of colors in palette (0 indicates 2^n) printf("%c%c%c%c", 0, 0, 0, 0); // Number of important colors (0 indicates all) // Pixel data for (int i = 0; i < 11; i++) { for (int j = 0; j < 22; j++) { if (j < 8) { printf("%c%c%c", 0, 255, 0); // Green } else if (j < 16) { printf("%c%c%c", 255, 255, 255); // White } else { printf("%c%c%c", 255, 0, 0); // Red } } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | #include <stdio.h> int main() { // Header printf("BM"); // Magic number printf("%c%c%c%c", 54, 0, 0, 0); // File size (dummy) printf("%c%c", 0, 0); // Reserved (always 0) printf("%c%c", 54, 0); // Offset to image data // DIB header printf("%c%c%c%c", 40, 0, 0, 0); // DIB header size printf("%c%c", 22, 0); // Width printf("%c%c", 11, 0); // Height (negative indicates top-down) printf("%c%c", 1, 0); // Number of color planes printf("%c%c", 24, 0); // Bits per pixel printf("%c%c%c%c", 0, 0, 0, 0); // Compression (none) printf("%c%c%c%c", 0, 0, 0, 0); // Image size (dummy) printf("%c%c", 0, 0); // Horizontal resolution (dummy) printf("%c%c", 0, 0); // Vertical resolution (dummy) printf("%c%c%c%c", 0, 0, 0, 0); // Number of colors in palette (0 indicates 2^n) printf("%c%c%c%c", 0, 0, 0, 0); // Number of important colors (0 indicates all) // Pixel data for (int i = 0; i < 11; i++) { for (int j = 0; j < 22; j++) { if (j < 8) { printf("%c%c%c", 0, 255, 0); // Green } else if (j < 16) { printf("%c%c%c", 255, 255, 255); // White } else { printf("%c%c%c", 255, 0, 0); // Red } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | #include <stdio.h> int main() { // Header printf("BM"); // Magic number printf("%c%c%c%c", 54, 0, 0, 0); // File size (dummy) printf("%c%c", 0, 0); // Reserved (always 0) printf("%c%c", 54, 0); // Offset to image data // DIB header printf("%c%c%c%c", 40, 0, 0, 0); // DIB header size printf("%c%c", 22, 0); // Width printf("%c%c", 11, 0); // Height (negative indicates top-down) printf("%c%c", 1, 0); // Number of color planes printf("%c%c", 24, 0); // Bits per pixel printf("%c%c%c%c", 0, 0, 0, 0); // Compression (none) printf("%c%c%c%c", 0, 0, 0, 0); // Image size (dummy) printf("%c%c", 0, 0); // Horizontal resolution (dummy) printf("%c%c", 0, 0); // Vertical resolution (dummy) printf("%c%c%c%c", 0, 0, 0, 0); // Number of colors in palette (0 indicates 2^n) printf("%c%c%c%c", 0, 0, 0, 0); // Number of important colors (0 indicates all) // Pixel data for (int i = 0; i < 11; i++) { for (int j = 0; j < 22; j++) { if (j < 8) { printf("%c%c%c", 0, 255, 0); // Green } else if (j < 16) { printf("%c%c%c", 255, 255, 255); // White } else { printf("%c%c%c", 255, 0, 0); // Red } } } return 0; } |
b'BM6\x00\x00\x00\x00\x006\x00(\x00\x00\x00\x16\x00\x0b\x00\x01\x00\x18\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00\xff\x00\x00'
What flag is shown in this image?
Truncated File Read
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | #include <stdio.h> int main() { int n, i, j, count = 0; scanf("%d", &n); int arr[n]; for (i = 0; i < n; i++) { scanf("%d", &arr[i]); } for (i = 0; i < n; i++) { for (j = i + 1; j < n; j++) { if (arr[i] == arr[j]) { count++; break; } } } printf("%d", count); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | #include <stdio.h> int main() { int n, i, j, count = 0; scanf("%d", &n); int arr[n]; for (i = 0; i < n; i++) { scanf("%d", &arr[i]); } for (i = 0; i < n; i++) { for (j = i + 1; j < n; j++) { if (arr[i] == arr[j]) { count++; break; } } } printf("%d", count); return 0; } |
b'main.sh: line 2: 16 Segmentation fault (core dumped) ./a.out\n'
What flag is shown in this image?
cannot identify image file <_io.BytesIO object at 0x7ff2ad0a0a40>
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 | #include <stdio.h> #include <stdlib.h> #pragma pack(1) typedef struct { unsigned char signature[2]; unsigned int file_size; unsigned int reserved; unsigned int data_offset; unsigned int header_size; int width; int height; unsigned short planes; unsigned short bits_per_pixel; unsigned int compression; unsigned int image_size; int x_resolution; int y_resolution; unsigned int num_colors; unsigned int important_colors; } bmp_header; int main() { const int width = 300; const int height = 150; const int padding = (4 - (width * 3) % 4) % 4; bmp_header header = { .signature = {'B', 'M'}, .file_size = sizeof(bmp_header) + width * height * 3 + padding * height, .reserved = 0, .data_offset = sizeof(bmp_header), .header_size = 40, .width = width, .height = height, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = width * height * 3 + padding * height, .x_resolution = 0, .y_resolution = 0, .num_colors = 0, .important_colors = 0 }; fwrite(&header, sizeof(bmp_header), 1, stdout); for (int i = 0; i < height; i++) { for (int j = 0; j < width; j++) { unsigned char color; if (j < width / 3) { color = 0; // green } else if (j < 2 * width / 3) { color = 255; // white } else { color = 128; // red } fwrite(&color, 1, 1, stdout); fwrite(&color, 1, 1, stdout); fwrite(&color, 1, 1, stdout); } for (int j = 0; j < padding; j++) { fwrite("\0", 1, 1, stdout); } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 | #include <stdio.h> #include <stdlib.h> #pragma pack(1) typedef struct { unsigned char signature[2]; unsigned int file_size; unsigned int reserved; unsigned int data_offset; unsigned int header_size; int width; int height; unsigned short planes; unsigned short bits_per_pixel; unsigned int compression; unsigned int image_size; int x_resolution; int y_resolution; unsigned int num_colors; unsigned int important_colors; } bmp_header; int main() { const int width = 300; const int height = 150; const int padding = (4 - (width * 3) % 4) % 4; bmp_header header = { .signature = {'B', 'M'}, .file_size = sizeof(bmp_header) + width * height * 3 + padding * height, .reserved = 0, .data_offset = sizeof(bmp_header), .header_size = 40, .width = width, .height = height, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = width * height * 3 + padding * height, .x_resolution = 0, .y_resolution = 0, .num_colors = 0, .important_colors = 0 }; fwrite(&header, sizeof(bmp_header), 1, stdout); for (int i = 0; i < height; i++) { for (int j = 0; j < width; j++) { unsigned char color; if (j < width / 3) { color = 0; // green } else if (j < 2 * width / 3) { color = 255; // white } else { color = 128; // red } fwrite(&color, 1, 1, stdout); fwrite(&color, 1, 1, stdout); fwrite(&color, 1, 1, stdout); } for (int j = 0; j < padding; j++) { fwrite("\0", 1, 1, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 | #include <stdio.h> #include <stdlib.h> #pragma pack(1) typedef struct { unsigned char signature[2]; unsigned int file_size; unsigned int reserved; unsigned int data_offset; unsigned int header_size; int width; int height; unsigned short planes; unsigned short bits_per_pixel; unsigned int compression; unsigned int image_size; int x_resolution; int y_resolution; unsigned int num_colors; unsigned int important_colors; } bmp_header; int main() { const int width = 300; const int height = 150; const int padding = (4 - (width * 3) % 4) % 4; bmp_header header = { .signature = {'B', 'M'}, .file_size = sizeof(bmp_header) + width * height * 3 + padding * height, .reserved = 0, .data_offset = sizeof(bmp_header), .header_size = 40, .width = width, .height = height, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = width * height * 3 + padding * height, .x_resolution = 0, .y_resolution = 0, .num_colors = 0, .important_colors = 0 }; fwrite(&header, sizeof(bmp_header), 1, stdout); for (int i = 0; i < height; i++) { for (int j = 0; j < width; j++) { unsigned char color; if (j < width / 3) { color = 0; // green } else if (j < 2 * width / 3) { color = 255; // white } else { color = 128; // red } fwrite(&color, 1, 1, stdout); fwrite(&color, 1, 1, stdout); fwrite(&color, 1, 1, stdout); } for (int j = 0; j < padding; j++) { fwrite("\0", 1, 1, stdout); } } return 0; } |
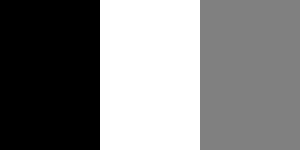
What flag is shown in this image?
The image shows a flag with three vertical stripes of equal width, colored black, white, and grey. This flag does not correspond to any official national flag. However, it resembles the design of a typical national flag, with the exception that the colors are grayscale. If this flag is intended to represent a specific group, organization, or concept, additional context would be needed to accurately identify it.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False