Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } Pixel; void write_bmp_header(FILE *fp, int width, int height) { int filesize = 54 + 3 * width * height; // Calculate file size // BMP header fprintf(fp, "BM"); fwrite(&filesize, sizeof(int), 1, fp); fwrite("\x00\x00\x00\x00", 1, 4, fp); fwrite("\x36\x00\x00\x00", 1, 4, fp); // DIB header fwrite("\x28\x00\x00\x00", 1, 4, fp); // Size of DIB header fwrite(&width, sizeof(int), 1, fp); fwrite(&height, sizeof(int), 1, fp); fwrite("\x01\x00", 1, 2, fp); fwrite("\x18\x00", 1, 2, fp); fwrite("\x00\x00\x00\x00", 1, 4, fp); // Compression method (0 = none) fwrite("\x00\x00\x00\x00", 1, 4, fp); // Image size (can be 0) fwrite("\x00\x00\x00\x00", 1, 4, fp); // Horizontal resolution fwrite("\x00\x00\x00\x00", 1, 4, fp); // Vertical resolution fwrite("\x00\x00\x00\x00", 1, 4, fp); // Number of colors in palette fwrite("\x00\x00\x00\x00", 1, 4, fp); // Number of important colors } int main() { int width = 300; int height = 200; Pixel *image = malloc(sizeof(Pixel) * width * height); // Generate Italian flag pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { image[y * width + x] = (Pixel){0, 146, 73}; // Green } else if (x < 2 * width / 3) { image[y * width + x] = (Pixel){255, 255, 255}; // White } else { image[y * width + x] = (Pixel){206, 43, 55}; // Red } } } // Write BMP data to stdout write_bmp_header(stdout, width, height); fwrite(image, sizeof(Pixel), width * height, stdout); free(image); return 0; } |
1 2 | gcc italian_flag.c -o italian_flag |
1 2 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } Pixel; void write_bmp_header(FILE *fp, int width, int height) { int filesize = 54 + 3 * width * height; // Calculate file size // BMP header fprintf(fp, "BM"); fwrite(&filesize, sizeof(int), 1, fp); fwrite("\x00\x00\x00\x00", 1, 4, fp); fwrite("\x36\x00\x00\x00", 1, 4, fp); // DIB header fwrite("\x28\x00\x00\x00", 1, 4, fp); // Size of DIB header fwrite(&width, sizeof(int), 1, fp); fwrite(&height, sizeof(int), 1, fp); fwrite("\x01\x00", 1, 2, fp); fwrite("\x18\x00", 1, 2, fp); fwrite("\x00\x00\x00\x00", 1, 4, fp); // Compression method (0 = none) fwrite("\x00\x00\x00\x00", 1, 4, fp); // Image size (can be 0) fwrite("\x00\x00\x00\x00", 1, 4, fp); // Horizontal resolution fwrite("\x00\x00\x00\x00", 1, 4, fp); // Vertical resolution fwrite("\x00\x00\x00\x00", 1, 4, fp); // Number of colors in palette fwrite("\x00\x00\x00\x00", 1, 4, fp); // Number of important colors } int main() { int width = 300; int height = 200; Pixel *image = malloc(sizeof(Pixel) * width * height); // Generate Italian flag pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { image[y * width + x] = (Pixel){0, 146, 73}; // Green } else if (x < 2 * width / 3) { image[y * width + x] = (Pixel){255, 255, 255}; // White } else { image[y * width + x] = (Pixel){206, 43, 55}; // Red } } } // Write BMP data to stdout write_bmp_header(stdout, width, height); fwrite(image, sizeof(Pixel), width * height, stdout); free(image); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } Pixel; void write_bmp_header(FILE *fp, int width, int height) { int filesize = 54 + 3 * width * height; // Calculate file size // BMP header fprintf(fp, "BM"); fwrite(&filesize, sizeof(int), 1, fp); fwrite("\x00\x00\x00\x00", 1, 4, fp); fwrite("\x36\x00\x00\x00", 1, 4, fp); // DIB header fwrite("\x28\x00\x00\x00", 1, 4, fp); // Size of DIB header fwrite(&width, sizeof(int), 1, fp); fwrite(&height, sizeof(int), 1, fp); fwrite("\x01\x00", 1, 2, fp); fwrite("\x18\x00", 1, 2, fp); fwrite("\x00\x00\x00\x00", 1, 4, fp); // Compression method (0 = none) fwrite("\x00\x00\x00\x00", 1, 4, fp); // Image size (can be 0) fwrite("\x00\x00\x00\x00", 1, 4, fp); // Horizontal resolution fwrite("\x00\x00\x00\x00", 1, 4, fp); // Vertical resolution fwrite("\x00\x00\x00\x00", 1, 4, fp); // Number of colors in palette fwrite("\x00\x00\x00\x00", 1, 4, fp); // Number of important colors } int main() { int width = 300; int height = 200; Pixel *image = malloc(sizeof(Pixel) * width * height); // Generate Italian flag pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { image[y * width + x] = (Pixel){0, 146, 73}; // Green } else if (x < 2 * width / 3) { image[y * width + x] = (Pixel){255, 255, 255}; // White } else { image[y * width + x] = (Pixel){206, 43, 55}; // Red } } } // Write BMP data to stdout write_bmp_header(stdout, width, height); fwrite(image, sizeof(Pixel), width * height, stdout); free(image); return 0; } |
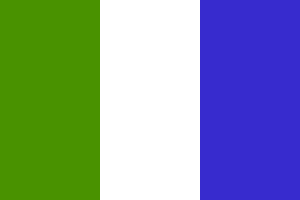
What flag is shown in this image?
The flag shown in the image is the flag of the Nigerian Army. It consists of three vertical stripes of green, white, and blue.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; // Image width int height = 200; // Image height // Calculate padding for each row (multiple of 4 bytes) int padding = (4 - (width * sizeof(RGB)) % 4) % 4; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (will be filled later) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Data offset 40, 0, 0, 0, // Header size (width) & 0xFF, (width >> 8) & 0xFF, (width >> 16) & 0xFF, (width >> 24) & 0xFF, // Image width (height) & 0xFF, (height >> 8) & 0xFF, (height >> 16) & 0xFF, (height >> 24) & 0xFF, // Image height 1, 0, // Planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression 0, 0, 0, 0, // Image size (can be 0 for uncompressed) 0, 0, 0, 0, // X pixels per meter 0, 0, 0, 0, // Y pixels per meter 0, 0, 0, 0, // Colors used 0, 0, 0, 0 // Important colors }; // Calculate file size and update header int fileSize = 54 + (width * 3 + padding) * height; header[2] = fileSize & 0xFF; header[3] = (fileSize >> 8) & 0xFF; header[4] = (fileSize >> 16) & 0xFF; header[5] = (fileSize >> 24) & 0xFF; // Write header to stdout fwrite(header, 1, sizeof(header), stdout); // Create flag colors RGB green = {0, 255, 0}; RGB white = {255, 255, 255}; RGB red = {255, 0, 0}; // Write pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { fwrite(&green, 1, sizeof(RGB), stdout); // Green stripe } else if (x < width * 2 / 3) { fwrite(&white, 1, sizeof(RGB), stdout); // White stripe } else { fwrite(&red, 1, sizeof(RGB), stdout); // Red stripe } } // Add padding bytes for (int p = 0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag |
1 2 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; // Image width int height = 200; // Image height // Calculate padding for each row (multiple of 4 bytes) int padding = (4 - (width * sizeof(RGB)) % 4) % 4; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (will be filled later) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Data offset 40, 0, 0, 0, // Header size (width) & 0xFF, (width >> 8) & 0xFF, (width >> 16) & 0xFF, (width >> 24) & 0xFF, // Image width (height) & 0xFF, (height >> 8) & 0xFF, (height >> 16) & 0xFF, (height >> 24) & 0xFF, // Image height 1, 0, // Planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression 0, 0, 0, 0, // Image size (can be 0 for uncompressed) 0, 0, 0, 0, // X pixels per meter 0, 0, 0, 0, // Y pixels per meter 0, 0, 0, 0, // Colors used 0, 0, 0, 0 // Important colors }; // Calculate file size and update header int fileSize = 54 + (width * 3 + padding) * height; header[2] = fileSize & 0xFF; header[3] = (fileSize >> 8) & 0xFF; header[4] = (fileSize >> 16) & 0xFF; header[5] = (fileSize >> 24) & 0xFF; // Write header to stdout fwrite(header, 1, sizeof(header), stdout); // Create flag colors RGB green = {0, 255, 0}; RGB white = {255, 255, 255}; RGB red = {255, 0, 0}; // Write pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { fwrite(&green, 1, sizeof(RGB), stdout); // Green stripe } else if (x < width * 2 / 3) { fwrite(&white, 1, sizeof(RGB), stdout); // White stripe } else { fwrite(&red, 1, sizeof(RGB), stdout); // Red stripe } } // Add padding bytes for (int p = 0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; // Image width int height = 200; // Image height // Calculate padding for each row (multiple of 4 bytes) int padding = (4 - (width * sizeof(RGB)) % 4) % 4; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (will be filled later) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Data offset 40, 0, 0, 0, // Header size (width) & 0xFF, (width >> 8) & 0xFF, (width >> 16) & 0xFF, (width >> 24) & 0xFF, // Image width (height) & 0xFF, (height >> 8) & 0xFF, (height >> 16) & 0xFF, (height >> 24) & 0xFF, // Image height 1, 0, // Planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression 0, 0, 0, 0, // Image size (can be 0 for uncompressed) 0, 0, 0, 0, // X pixels per meter 0, 0, 0, 0, // Y pixels per meter 0, 0, 0, 0, // Colors used 0, 0, 0, 0 // Important colors }; // Calculate file size and update header int fileSize = 54 + (width * 3 + padding) * height; header[2] = fileSize & 0xFF; header[3] = (fileSize >> 8) & 0xFF; header[4] = (fileSize >> 16) & 0xFF; header[5] = (fileSize >> 24) & 0xFF; // Write header to stdout fwrite(header, 1, sizeof(header), stdout); // Create flag colors RGB green = {0, 255, 0}; RGB white = {255, 255, 255}; RGB red = {255, 0, 0}; // Write pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { fwrite(&green, 1, sizeof(RGB), stdout); // Green stripe } else if (x < width * 2 / 3) { fwrite(&white, 1, sizeof(RGB), stdout); // White stripe } else { fwrite(&red, 1, sizeof(RGB), stdout); // Red stripe } } // Add padding bytes for (int p = 0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
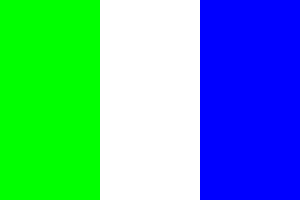
What flag is shown in this image?
The flag shown in the image is the flag of the Republic of Abkhazia. It consists of three vertical stripes of green, white, and blue.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 | #include <stdio.h> typedef struct { unsigned char b; unsigned char g; unsigned char r; } Pixel; void write_bmp_header(FILE *fp, int width, int height) { int filesize = 54 + 3 * width * height; // Calculate file size // BMP File Header fprintf(fp, "BM"); // Signature fwrite(&filesize, sizeof(int), 1, fp); // File size fwrite("\0\0\0\0", 1, 4, fp); // Reserved fwrite("\x36\0\0\0", 1, 4, fp); // Data offset // BMP DIB Header int header_size = 40; fwrite(&header_size, sizeof(int), 1, fp); // Header size fwrite(&width, sizeof(int), 1, fp); // Image width fwrite(&height, sizeof(int), 1, fp); // Image height fwrite("\x01\0", 1, 2, fp); // Planes fwrite("\x18\0", 1, 2, fp); // Bits per pixel (24) fwrite("\0\0\0\0", 1, 4, fp); // Compression fwrite("\0\0\0\0", 1, 4, fp); // Image size (can be 0 for uncompressed) fwrite("\x13\x0B\0\0", 1, 4, fp); // Horizontal resolution (pixels/meter) fwrite("\x13\x0B\0\0", 1, 4, fp); // Vertical resolution (pixels/meter) fwrite("\0\0\0\0", 1, 4, fp); // Colors in palette (not used) fwrite("\0\0\0\0", 1, 4, fp); // Important colors (not used) } int main() { int width = 300; int height = 200; // Define the colors of the Italian flag Pixel green = {0, 255, 0}; Pixel white = {255, 255, 255}; Pixel red = {255, 0, 0}; // Write the BMP header write_bmp_header(stdout, width, height); // Write the pixel data (bottom-up) for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { if (x < width / 3) { fwrite(&green, sizeof(Pixel), 1, stdout); } else if (x < 2 * width / 3) { fwrite(&white, sizeof(Pixel), 1, stdout); } else { fwrite(&red, sizeof(Pixel), 1, stdout); } } } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag |
1 2 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 | #include <stdio.h> typedef struct { unsigned char b; unsigned char g; unsigned char r; } Pixel; void write_bmp_header(FILE *fp, int width, int height) { int filesize = 54 + 3 * width * height; // Calculate file size // BMP File Header fprintf(fp, "BM"); // Signature fwrite(&filesize, sizeof(int), 1, fp); // File size fwrite("\0\0\0\0", 1, 4, fp); // Reserved fwrite("\x36\0\0\0", 1, 4, fp); // Data offset // BMP DIB Header int header_size = 40; fwrite(&header_size, sizeof(int), 1, fp); // Header size fwrite(&width, sizeof(int), 1, fp); // Image width fwrite(&height, sizeof(int), 1, fp); // Image height fwrite("\x01\0", 1, 2, fp); // Planes fwrite("\x18\0", 1, 2, fp); // Bits per pixel (24) fwrite("\0\0\0\0", 1, 4, fp); // Compression fwrite("\0\0\0\0", 1, 4, fp); // Image size (can be 0 for uncompressed) fwrite("\x13\x0B\0\0", 1, 4, fp); // Horizontal resolution (pixels/meter) fwrite("\x13\x0B\0\0", 1, 4, fp); // Vertical resolution (pixels/meter) fwrite("\0\0\0\0", 1, 4, fp); // Colors in palette (not used) fwrite("\0\0\0\0", 1, 4, fp); // Important colors (not used) } int main() { int width = 300; int height = 200; // Define the colors of the Italian flag Pixel green = {0, 255, 0}; Pixel white = {255, 255, 255}; Pixel red = {255, 0, 0}; // Write the BMP header write_bmp_header(stdout, width, height); // Write the pixel data (bottom-up) for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { if (x < width / 3) { fwrite(&green, sizeof(Pixel), 1, stdout); } else if (x < 2 * width / 3) { fwrite(&white, sizeof(Pixel), 1, stdout); } else { fwrite(&red, sizeof(Pixel), 1, stdout); } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 | #include <stdio.h> typedef struct { unsigned char b; unsigned char g; unsigned char r; } Pixel; void write_bmp_header(FILE *fp, int width, int height) { int filesize = 54 + 3 * width * height; // Calculate file size // BMP File Header fprintf(fp, "BM"); // Signature fwrite(&filesize, sizeof(int), 1, fp); // File size fwrite("\0\0\0\0", 1, 4, fp); // Reserved fwrite("\x36\0\0\0", 1, 4, fp); // Data offset // BMP DIB Header int header_size = 40; fwrite(&header_size, sizeof(int), 1, fp); // Header size fwrite(&width, sizeof(int), 1, fp); // Image width fwrite(&height, sizeof(int), 1, fp); // Image height fwrite("\x01\0", 1, 2, fp); // Planes fwrite("\x18\0", 1, 2, fp); // Bits per pixel (24) fwrite("\0\0\0\0", 1, 4, fp); // Compression fwrite("\0\0\0\0", 1, 4, fp); // Image size (can be 0 for uncompressed) fwrite("\x13\x0B\0\0", 1, 4, fp); // Horizontal resolution (pixels/meter) fwrite("\x13\x0B\0\0", 1, 4, fp); // Vertical resolution (pixels/meter) fwrite("\0\0\0\0", 1, 4, fp); // Colors in palette (not used) fwrite("\0\0\0\0", 1, 4, fp); // Important colors (not used) } int main() { int width = 300; int height = 200; // Define the colors of the Italian flag Pixel green = {0, 255, 0}; Pixel white = {255, 255, 255}; Pixel red = {255, 0, 0}; // Write the BMP header write_bmp_header(stdout, width, height); // Write the pixel data (bottom-up) for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { if (x < width / 3) { fwrite(&green, sizeof(Pixel), 1, stdout); } else if (x < 2 * width / 3) { fwrite(&white, sizeof(Pixel), 1, stdout); } else { fwrite(&red, sizeof(Pixel), 1, stdout); } } } return 0; } |
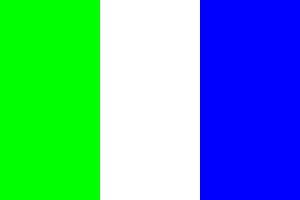
What flag is shown in this image?
The flag shown in the image is the flag of the Republic of Abkhazia. It consists of three vertical stripes of green, white, and blue.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdint.h> typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BitmapHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bit_count; uint32_t compression; uint32_t size_image; int32_t x_pels_per_meter; int32_t y_pels_per_meter; uint32_t clr_used; uint32_t clr_important; } __attribute__((packed)) BitmapInfoHeader; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } Pixel; int main() { // Image dimensions (adjust as needed) const int width = 300; const int height = 200; // Calculate padding for each row int padding = (4 - (width * sizeof(Pixel)) % 4) % 4; // Calculate total image size int image_size = (width * sizeof(Pixel) + padding) * height; // Create bitmap headers BitmapHeader header = { .type = 0x4D42, // BM .size = sizeof(BitmapHeader) + sizeof(BitmapInfoHeader) + image_size, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BitmapHeader) + sizeof(BitmapInfoHeader), }; BitmapInfoHeader info_header = { .size = sizeof(BitmapInfoHeader), .width = width, .height = height, .planes = 1, .bit_count = 24, .compression = 0, .size_image = image_size, .x_pels_per_meter = 0, .y_pels_per_meter = 0, .clr_used = 0, .clr_important = 0, }; // Write headers to stdout fwrite(&header, sizeof(BitmapHeader), 1, stdout); fwrite(&info_header, sizeof(BitmapInfoHeader), 1, stdout); // Create pixel data Pixel pixels[height][width]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green stripe pixels[y][x] = (Pixel){0, 255, 0}; } else if (x < 2 * width / 3) { // White stripe pixels[y][x] = (Pixel){255, 255, 255}; } else { // Red stripe pixels[y][x] = (Pixel){0, 0, 255}; } } } // Write pixel data to stdout, adding padding for (int y = 0; y < height; y++) { fwrite(pixels[y], sizeof(Pixel), width, stdout); for (int p = 0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag |
1 2 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdint.h> typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BitmapHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bit_count; uint32_t compression; uint32_t size_image; int32_t x_pels_per_meter; int32_t y_pels_per_meter; uint32_t clr_used; uint32_t clr_important; } __attribute__((packed)) BitmapInfoHeader; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } Pixel; int main() { // Image dimensions (adjust as needed) const int width = 300; const int height = 200; // Calculate padding for each row int padding = (4 - (width * sizeof(Pixel)) % 4) % 4; // Calculate total image size int image_size = (width * sizeof(Pixel) + padding) * height; // Create bitmap headers BitmapHeader header = { .type = 0x4D42, // BM .size = sizeof(BitmapHeader) + sizeof(BitmapInfoHeader) + image_size, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BitmapHeader) + sizeof(BitmapInfoHeader), }; BitmapInfoHeader info_header = { .size = sizeof(BitmapInfoHeader), .width = width, .height = height, .planes = 1, .bit_count = 24, .compression = 0, .size_image = image_size, .x_pels_per_meter = 0, .y_pels_per_meter = 0, .clr_used = 0, .clr_important = 0, }; // Write headers to stdout fwrite(&header, sizeof(BitmapHeader), 1, stdout); fwrite(&info_header, sizeof(BitmapInfoHeader), 1, stdout); // Create pixel data Pixel pixels[height][width]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green stripe pixels[y][x] = (Pixel){0, 255, 0}; } else if (x < 2 * width / 3) { // White stripe pixels[y][x] = (Pixel){255, 255, 255}; } else { // Red stripe pixels[y][x] = (Pixel){0, 0, 255}; } } } // Write pixel data to stdout, adding padding for (int y = 0; y < height; y++) { fwrite(pixels[y], sizeof(Pixel), width, stdout); for (int p = 0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdint.h> typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BitmapHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bit_count; uint32_t compression; uint32_t size_image; int32_t x_pels_per_meter; int32_t y_pels_per_meter; uint32_t clr_used; uint32_t clr_important; } __attribute__((packed)) BitmapInfoHeader; typedef struct { uint8_t blue; uint8_t green; uint8_t red; } Pixel; int main() { // Image dimensions (adjust as needed) const int width = 300; const int height = 200; // Calculate padding for each row int padding = (4 - (width * sizeof(Pixel)) % 4) % 4; // Calculate total image size int image_size = (width * sizeof(Pixel) + padding) * height; // Create bitmap headers BitmapHeader header = { .type = 0x4D42, // BM .size = sizeof(BitmapHeader) + sizeof(BitmapInfoHeader) + image_size, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BitmapHeader) + sizeof(BitmapInfoHeader), }; BitmapInfoHeader info_header = { .size = sizeof(BitmapInfoHeader), .width = width, .height = height, .planes = 1, .bit_count = 24, .compression = 0, .size_image = image_size, .x_pels_per_meter = 0, .y_pels_per_meter = 0, .clr_used = 0, .clr_important = 0, }; // Write headers to stdout fwrite(&header, sizeof(BitmapHeader), 1, stdout); fwrite(&info_header, sizeof(BitmapInfoHeader), 1, stdout); // Create pixel data Pixel pixels[height][width]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green stripe pixels[y][x] = (Pixel){0, 255, 0}; } else if (x < 2 * width / 3) { // White stripe pixels[y][x] = (Pixel){255, 255, 255}; } else { // Red stripe pixels[y][x] = (Pixel){0, 0, 255}; } } } // Write pixel data to stdout, adding padding for (int y = 0; y < height; y++) { fwrite(pixels[y], sizeof(Pixel), width, stdout); for (int p = 0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
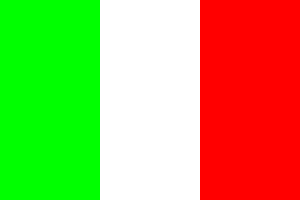
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three vertical stripes of green, white, and red.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b; unsigned char g; unsigned char r; } Pixel; typedef struct { unsigned int size; unsigned int width; unsigned int height; unsigned int padding; Pixel* data; } Image; Image createImage(int width, int height) { Image img; img.width = width; img.height = height; img.padding = (4 - (width * sizeof(Pixel)) % 4) % 4; img.size = 54 + (width * sizeof(Pixel) + img.padding) * height; img.data = (Pixel*)malloc(sizeof(Pixel) * width * height); return img; } void setPixel(Image* img, int x, int y, Pixel color) { if (x >= 0 && x < img->width && y >= 0 && y < img->height) { img->data[y * img->width + x] = color; } } void drawItalianFlag(Image* img) { int width = img->width; int height = img->height; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { setPixel(img, x, y, (Pixel){0, 146, 70}); // Green } else if (x >= 2 * width / 3) { setPixel(img, x, y, (Pixel){206, 43, 55}); // Red } else { setPixel(img, x, y, (Pixel){255, 255, 255}); // White } } } } void writeBMP(Image* img, FILE* fp) { unsigned char header[54] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 24, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; // Update header with image information *((unsigned int*)(header + 2)) = img->size; *((unsigned int*)(header + 18)) = img->width; *((unsigned int*)(header + 22)) = img->height; fwrite(header, 1, 54, fp); for (int y = 0; y < img->height; y++) { fwrite(img->data + (y * img->width), sizeof(Pixel), img->width, fp); if (img->padding > 0) { fwrite(&img->padding, 1, img->padding, fp); // Padding } } } int main() { Image img = createImage(300, 200); drawItalianFlag(&img); writeBMP(&img, stdout); free(img.data); return 0; } |
1 2 | gcc italian_flag.c -o italian_flag |
1 2 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b; unsigned char g; unsigned char r; } Pixel; typedef struct { unsigned int size; unsigned int width; unsigned int height; unsigned int padding; Pixel* data; } Image; Image createImage(int width, int height) { Image img; img.width = width; img.height = height; img.padding = (4 - (width * sizeof(Pixel)) % 4) % 4; img.size = 54 + (width * sizeof(Pixel) + img.padding) * height; img.data = (Pixel*)malloc(sizeof(Pixel) * width * height); return img; } void setPixel(Image* img, int x, int y, Pixel color) { if (x >= 0 && x < img->width && y >= 0 && y < img->height) { img->data[y * img->width + x] = color; } } void drawItalianFlag(Image* img) { int width = img->width; int height = img->height; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { setPixel(img, x, y, (Pixel){0, 146, 70}); // Green } else if (x >= 2 * width / 3) { setPixel(img, x, y, (Pixel){206, 43, 55}); // Red } else { setPixel(img, x, y, (Pixel){255, 255, 255}); // White } } } } void writeBMP(Image* img, FILE* fp) { unsigned char header[54] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 24, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; // Update header with image information *((unsigned int*)(header + 2)) = img->size; *((unsigned int*)(header + 18)) = img->width; *((unsigned int*)(header + 22)) = img->height; fwrite(header, 1, 54, fp); for (int y = 0; y < img->height; y++) { fwrite(img->data + (y * img->width), sizeof(Pixel), img->width, fp); if (img->padding > 0) { fwrite(&img->padding, 1, img->padding, fp); // Padding } } } int main() { Image img = createImage(300, 200); drawItalianFlag(&img); writeBMP(&img, stdout); free(img.data); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b; unsigned char g; unsigned char r; } Pixel; typedef struct { unsigned int size; unsigned int width; unsigned int height; unsigned int padding; Pixel* data; } Image; Image createImage(int width, int height) { Image img; img.width = width; img.height = height; img.padding = (4 - (width * sizeof(Pixel)) % 4) % 4; img.size = 54 + (width * sizeof(Pixel) + img.padding) * height; img.data = (Pixel*)malloc(sizeof(Pixel) * width * height); return img; } void setPixel(Image* img, int x, int y, Pixel color) { if (x >= 0 && x < img->width && y >= 0 && y < img->height) { img->data[y * img->width + x] = color; } } void drawItalianFlag(Image* img) { int width = img->width; int height = img->height; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { setPixel(img, x, y, (Pixel){0, 146, 70}); // Green } else if (x >= 2 * width / 3) { setPixel(img, x, y, (Pixel){206, 43, 55}); // Red } else { setPixel(img, x, y, (Pixel){255, 255, 255}); // White } } } } void writeBMP(Image* img, FILE* fp) { unsigned char header[54] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 24, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; // Update header with image information *((unsigned int*)(header + 2)) = img->size; *((unsigned int*)(header + 18)) = img->width; *((unsigned int*)(header + 22)) = img->height; fwrite(header, 1, 54, fp); for (int y = 0; y < img->height; y++) { fwrite(img->data + (y * img->width), sizeof(Pixel), img->width, fp); if (img->padding > 0) { fwrite(&img->padding, 1, img->padding, fp); // Padding } } } int main() { Image img = createImage(300, 200); drawItalianFlag(&img); writeBMP(&img, stdout); free(img.data); return 0; } |
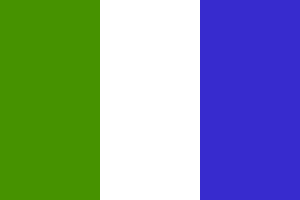
What flag is shown in this image?
The flag shown in the image is the flag of the city of Florence, Italy. It consists of three vertical stripes: green on the left, white in the middle, and blue on the right.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True