Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; int height = 200; // BMP header unsigned char header[54] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 24, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; // Calculate file size and image data size int filesize = 54 + width * height * 3; int datasize = width * height * 3; // Update header with file size and image data size header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; header[34] = datasize & 0xFF; header[35] = (datasize >> 8) & 0xFF; header[36] = (datasize >> 16) & 0xFF; header[37] = (datasize >> 24) & 0xFF; header[18] = width & 0xFF; header[19] = (width >> 8) & 0xFF; header[20] = (width >> 16) & 0xFF; header[21] = (width >> 24) & 0xFF; header[22] = height & 0xFF; header[23] = (height >> 8) & 0xFF; header[24] = (height >> 16) & 0xFF; header[25] = (height >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB pixel; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green pixel.r = 0; pixel.g = 146; pixel.b = 70; } else if (x < 2 * width / 3) { // White pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { // Red pixel.r = 206; pixel.g = 43; pixel.b = 55; } fwrite(&pixel, 1, 3, stdout); } // Padding (if needed) - make each row a multiple of 4 bytes int padding = (4 - (width * 3) % 4) % 4; for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
1 2 3 4 | if (fwrite(&pixel, 1, 3, stdout) != 3) { fprintf(stderr, "Error writing pixel data\n"); return 1; // Indicate an error } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; int height = 200; // BMP header unsigned char header[54] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 24, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; // Calculate file size and image data size int filesize = 54 + width * height * 3; int datasize = width * height * 3; // Update header with file size and image data size header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; header[34] = datasize & 0xFF; header[35] = (datasize >> 8) & 0xFF; header[36] = (datasize >> 16) & 0xFF; header[37] = (datasize >> 24) & 0xFF; header[18] = width & 0xFF; header[19] = (width >> 8) & 0xFF; header[20] = (width >> 16) & 0xFF; header[21] = (width >> 24) & 0xFF; header[22] = height & 0xFF; header[23] = (height >> 8) & 0xFF; header[24] = (height >> 16) & 0xFF; header[25] = (height >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB pixel; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green pixel.r = 0; pixel.g = 146; pixel.b = 70; } else if (x < 2 * width / 3) { // White pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { // Red pixel.r = 206; pixel.g = 43; pixel.b = 55; } fwrite(&pixel, 1, 3, stdout); } // Padding (if needed) - make each row a multiple of 4 bytes int padding = (4 - (width * 3) % 4) % 4; for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; int height = 200; // BMP header unsigned char header[54] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0, 40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 24, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; // Calculate file size and image data size int filesize = 54 + width * height * 3; int datasize = width * height * 3; // Update header with file size and image data size header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; header[34] = datasize & 0xFF; header[35] = (datasize >> 8) & 0xFF; header[36] = (datasize >> 16) & 0xFF; header[37] = (datasize >> 24) & 0xFF; header[18] = width & 0xFF; header[19] = (width >> 8) & 0xFF; header[20] = (width >> 16) & 0xFF; header[21] = (width >> 24) & 0xFF; header[22] = height & 0xFF; header[23] = (height >> 8) & 0xFF; header[24] = (height >> 16) & 0xFF; header[25] = (height >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB pixel; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green pixel.r = 0; pixel.g = 146; pixel.b = 70; } else if (x < 2 * width / 3) { // White pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { // Red pixel.r = 206; pixel.g = 43; pixel.b = 55; } fwrite(&pixel, 1, 3, stdout); } // Padding (if needed) - make each row a multiple of 4 bytes int padding = (4 - (width * 3) % 4) % 4; for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
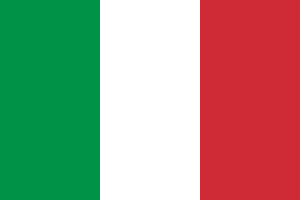
What flag is shown in this image?
The flag shown is the national flag of Italy.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; int height = 200; int stripe_width = width / 3; // BMP header unsigned char header[54] = { 'B', 'M', // Magic number 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (unsigned char)(width & 0xFF), (unsigned char)((width >> 8) & 0xFF), 0, 0, // Width (unsigned char)(height & 0xFF), (unsigned char)((height >> 8) & 0xFF), 0, 0, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (none) 0, 0, 0, 0, // Image size (can be 0 for uncompressed) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; // Calculate file size and update header int fileSize = 54 + width * height * 3; header[2] = fileSize & 0xFF; header[3] = (fileSize >> 8) & 0xFF; header[4] = (fileSize >> 16) & 0xFF; header[5] = (fileSize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); // Pixel data (bottom-up, left-to-right) for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { RGB pixel; if (x < stripe_width) { // Green pixel.r = 0; pixel.g = 128; // Adjust for a darker green pixel.b = 0; } else if (x < 2 * stripe_width) { // White pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { // Red pixel.r = 255; pixel.g = 0; pixel.b = 0; } fwrite(&pixel, 1, 3, stdout); } // Padding (for 4-byte alignment of rows) int padding = (4 - (width * 3) % 4) % 4; for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; int height = 200; int stripe_width = width / 3; // BMP header unsigned char header[54] = { 'B', 'M', // Magic number 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (unsigned char)(width & 0xFF), (unsigned char)((width >> 8) & 0xFF), 0, 0, // Width (unsigned char)(height & 0xFF), (unsigned char)((height >> 8) & 0xFF), 0, 0, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (none) 0, 0, 0, 0, // Image size (can be 0 for uncompressed) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; // Calculate file size and update header int fileSize = 54 + width * height * 3; header[2] = fileSize & 0xFF; header[3] = (fileSize >> 8) & 0xFF; header[4] = (fileSize >> 16) & 0xFF; header[5] = (fileSize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); // Pixel data (bottom-up, left-to-right) for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { RGB pixel; if (x < stripe_width) { // Green pixel.r = 0; pixel.g = 128; // Adjust for a darker green pixel.b = 0; } else if (x < 2 * stripe_width) { // White pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { // Red pixel.r = 255; pixel.g = 0; pixel.b = 0; } fwrite(&pixel, 1, 3, stdout); } // Padding (for 4-byte alignment of rows) int padding = (4 - (width * 3) % 4) % 4; for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; int height = 200; int stripe_width = width / 3; // BMP header unsigned char header[54] = { 'B', 'M', // Magic number 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (unsigned char)(width & 0xFF), (unsigned char)((width >> 8) & 0xFF), 0, 0, // Width (unsigned char)(height & 0xFF), (unsigned char)((height >> 8) & 0xFF), 0, 0, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (none) 0, 0, 0, 0, // Image size (can be 0 for uncompressed) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; // Calculate file size and update header int fileSize = 54 + width * height * 3; header[2] = fileSize & 0xFF; header[3] = (fileSize >> 8) & 0xFF; header[4] = (fileSize >> 16) & 0xFF; header[5] = (fileSize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); // Pixel data (bottom-up, left-to-right) for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { RGB pixel; if (x < stripe_width) { // Green pixel.r = 0; pixel.g = 128; // Adjust for a darker green pixel.b = 0; } else if (x < 2 * stripe_width) { // White pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { // Red pixel.r = 255; pixel.g = 0; pixel.b = 0; } fwrite(&pixel, 1, 3, stdout); } // Padding (for 4-byte alignment of rows) int padding = (4 - (width * 3) % 4) % 4; for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
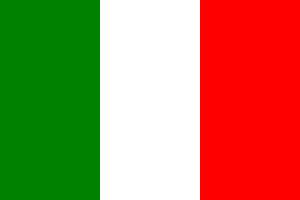
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three vertical stripes of green, white, and red.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; int height = 200; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (unsigned char)(width & 0xFF), (unsigned char)((width >> 8) & 0xFF), 0, 0, // Width (unsigned char)(height & 0xFF), (unsigned char)((height >> 8) & 0xFF), 0, 0, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB = 0) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution (pixels/meter) 0, 0, 0, 0, // Vertical resolution (pixels/meter) 0, 0, 0, 0, // Number of colors in palette (0 for 2^24) 0, 0, 0, 0 // Number of important colors (0 means all) }; // Calculate file size and update header int filesize = 54 + width * height * 3; // 3 bytes per pixel (RGB) header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB pixel; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green pixel.r = 0; pixel.g = 146; pixel.b = 70; } else if (x < 2 * width / 3) { // White pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { // Red pixel.r = 206; pixel.g = 43; pixel.b = 55; } fwrite(&pixel, 1, 3, stdout); // Write pixel data } // Padding (BMP rows must be multiples of 4 bytes) int padding = (4 - (width * 3) % 4) % 4; for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag ./italian_flag > italian_flag.bmp // Redirect output to a file |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; int height = 200; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (unsigned char)(width & 0xFF), (unsigned char)((width >> 8) & 0xFF), 0, 0, // Width (unsigned char)(height & 0xFF), (unsigned char)((height >> 8) & 0xFF), 0, 0, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB = 0) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution (pixels/meter) 0, 0, 0, 0, // Vertical resolution (pixels/meter) 0, 0, 0, 0, // Number of colors in palette (0 for 2^24) 0, 0, 0, 0 // Number of important colors (0 means all) }; // Calculate file size and update header int filesize = 54 + width * height * 3; // 3 bytes per pixel (RGB) header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB pixel; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green pixel.r = 0; pixel.g = 146; pixel.b = 70; } else if (x < 2 * width / 3) { // White pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { // Red pixel.r = 206; pixel.g = 43; pixel.b = 55; } fwrite(&pixel, 1, 3, stdout); // Write pixel data } // Padding (BMP rows must be multiples of 4 bytes) int padding = (4 - (width * 3) % 4) % 4; for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; int height = 200; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (unsigned char)(width & 0xFF), (unsigned char)((width >> 8) & 0xFF), 0, 0, // Width (unsigned char)(height & 0xFF), (unsigned char)((height >> 8) & 0xFF), 0, 0, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB = 0) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution (pixels/meter) 0, 0, 0, 0, // Vertical resolution (pixels/meter) 0, 0, 0, 0, // Number of colors in palette (0 for 2^24) 0, 0, 0, 0 // Number of important colors (0 means all) }; // Calculate file size and update header int filesize = 54 + width * height * 3; // 3 bytes per pixel (RGB) header[2] = filesize & 0xFF; header[3] = (filesize >> 8) & 0xFF; header[4] = (filesize >> 16) & 0xFF; header[5] = (filesize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB pixel; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green pixel.r = 0; pixel.g = 146; pixel.b = 70; } else if (x < 2 * width / 3) { // White pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { // Red pixel.r = 206; pixel.g = 43; pixel.b = 55; } fwrite(&pixel, 1, 3, stdout); // Write pixel data } // Padding (BMP rows must be multiples of 4 bytes) int padding = (4 - (width * 3) % 4) % 4; for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
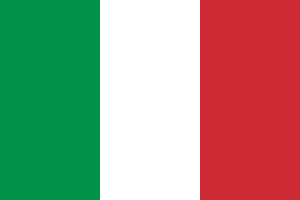
What flag is shown in this image?
The flag shown is the national flag of Italy.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | #include <stdio.h> #include <stdlib.h> // BMP file header structure typedef struct { unsigned short bfType; // Magic number for BMP files (BM) unsigned int bfSize; // File size in bytes unsigned short bfReserved1; // Reserved (usually 0) unsigned short bfReserved2; // Reserved (usually 0) unsigned int bfOffBits; // Offset to pixel data from beginning of file } BITMAPFILEHEADER; // BMP info header structure typedef struct { unsigned int biSize; // Size of this structure int biWidth; // Image width in pixels int biHeight; // Image height in pixels unsigned short biPlanes; // Number of color planes (must be 1) unsigned short biBitCount; // Bits per pixel (e.g., 24 for 24-bit RGB) unsigned int biCompression; // Compression type (0 for no compression) unsigned int biSizeImage; // Size of image data in bytes int biXPelsPerMeter; // Horizontal resolution in pixels per meter int biYPelsPerMeter; // Vertical resolution in pixels per meter unsigned int biClrUsed; // Number of colors in the color palette (0 for all) unsigned int biClrImportant; // Number of important colors (0 for all) } BITMAPINFOHEADER; int main() { int width = 300; // Image width int height = 200; // Image height int padding = (4 - (width * 3) % 4) % 4; // Calculate padding for each row BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; // Fill in file header fileHeader.bfType = 0x4D42; // BM fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + (width * 3 + padding) * height; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Fill in info header infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24-bit RGB infoHeader.biCompression = 0; // No compression infoHeader.biSizeImage = (width * 3 + padding) * height; infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Write headers to stdout fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout); fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout); unsigned char pixel[3]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green pixel[0] = 0; pixel[1] = 146; // Slightly darker green for better visibility pixel[2] = 70; } else if (x < 2 * width / 3) { // White pixel[0] = 255; pixel[1] = 255; pixel[2] = 255; } else { // Red pixel[0] = 206; // Slightly darker red pixel[1] = 43; pixel[2] = 55; } fwrite(pixel, 3, 1, stdout); } // Padding for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag |
1 2 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | #include <stdio.h> #include <stdlib.h> // BMP file header structure typedef struct { unsigned short bfType; // Magic number for BMP files (BM) unsigned int bfSize; // File size in bytes unsigned short bfReserved1; // Reserved (usually 0) unsigned short bfReserved2; // Reserved (usually 0) unsigned int bfOffBits; // Offset to pixel data from beginning of file } BITMAPFILEHEADER; // BMP info header structure typedef struct { unsigned int biSize; // Size of this structure int biWidth; // Image width in pixels int biHeight; // Image height in pixels unsigned short biPlanes; // Number of color planes (must be 1) unsigned short biBitCount; // Bits per pixel (e.g., 24 for 24-bit RGB) unsigned int biCompression; // Compression type (0 for no compression) unsigned int biSizeImage; // Size of image data in bytes int biXPelsPerMeter; // Horizontal resolution in pixels per meter int biYPelsPerMeter; // Vertical resolution in pixels per meter unsigned int biClrUsed; // Number of colors in the color palette (0 for all) unsigned int biClrImportant; // Number of important colors (0 for all) } BITMAPINFOHEADER; int main() { int width = 300; // Image width int height = 200; // Image height int padding = (4 - (width * 3) % 4) % 4; // Calculate padding for each row BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; // Fill in file header fileHeader.bfType = 0x4D42; // BM fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + (width * 3 + padding) * height; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Fill in info header infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24-bit RGB infoHeader.biCompression = 0; // No compression infoHeader.biSizeImage = (width * 3 + padding) * height; infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Write headers to stdout fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout); fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout); unsigned char pixel[3]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green pixel[0] = 0; pixel[1] = 146; // Slightly darker green for better visibility pixel[2] = 70; } else if (x < 2 * width / 3) { // White pixel[0] = 255; pixel[1] = 255; pixel[2] = 255; } else { // Red pixel[0] = 206; // Slightly darker red pixel[1] = 43; pixel[2] = 55; } fwrite(pixel, 3, 1, stdout); } // Padding for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | #include <stdio.h> #include <stdlib.h> // BMP file header structure typedef struct { unsigned short bfType; // Magic number for BMP files (BM) unsigned int bfSize; // File size in bytes unsigned short bfReserved1; // Reserved (usually 0) unsigned short bfReserved2; // Reserved (usually 0) unsigned int bfOffBits; // Offset to pixel data from beginning of file } BITMAPFILEHEADER; // BMP info header structure typedef struct { unsigned int biSize; // Size of this structure int biWidth; // Image width in pixels int biHeight; // Image height in pixels unsigned short biPlanes; // Number of color planes (must be 1) unsigned short biBitCount; // Bits per pixel (e.g., 24 for 24-bit RGB) unsigned int biCompression; // Compression type (0 for no compression) unsigned int biSizeImage; // Size of image data in bytes int biXPelsPerMeter; // Horizontal resolution in pixels per meter int biYPelsPerMeter; // Vertical resolution in pixels per meter unsigned int biClrUsed; // Number of colors in the color palette (0 for all) unsigned int biClrImportant; // Number of important colors (0 for all) } BITMAPINFOHEADER; int main() { int width = 300; // Image width int height = 200; // Image height int padding = (4 - (width * 3) % 4) % 4; // Calculate padding for each row BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; // Fill in file header fileHeader.bfType = 0x4D42; // BM fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + (width * 3 + padding) * height; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); // Fill in info header infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24-bit RGB infoHeader.biCompression = 0; // No compression infoHeader.biSizeImage = (width * 3 + padding) * height; infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Write headers to stdout fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout); fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout); unsigned char pixel[3]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green pixel[0] = 0; pixel[1] = 146; // Slightly darker green for better visibility pixel[2] = 70; } else if (x < 2 * width / 3) { // White pixel[0] = 255; pixel[1] = 255; pixel[2] = 255; } else { // Red pixel[0] = 206; // Slightly darker red pixel[1] = 43; pixel[2] = 55; } fwrite(pixel, 3, 1, stdout); } // Padding for (int i = 0; i < padding; i++) { fputc(0, stdout); } } return 0; } |
b'BM\x00\x00X\xbf\x02\x00\x00\x00\x00\x008\x00\x00\x00(\x00\x00\x00,\x01\x00\x00\xc8\x00\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00 \xbf\x02\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\xce+7\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\x00\x92F\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xf
What flag is shown in this image?
Truncated File Read
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; int height = 200; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (unsigned char)(width & 0xFF), (unsigned char)((width >> 8) & 0xFF), 0, 0, // Width (unsigned char)(height & 0xFF), (unsigned char)((height >> 8) & 0xFF), 0, 0, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB = 0) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; int imageSize = width * height * 3; int fileSize = imageSize + 54; // Update file size in header header[2] = fileSize & 0xFF; header[3] = (fileSize >> 8) & 0xFF; header[4] = (fileSize >> 16) & 0xFF; header[5] = (fileSize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB pixel; int padding = (4 - (width * 3) % 4) % 4; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green pixel.r = 0; pixel.g = 146; // Slightly darker green for better visibility pixel.b = 70; } else if (x < 2 * width / 3) { // White pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { // Red pixel.r = 206; // Slightly darker red for better visibility pixel.g = 43; pixel.b = 55; } fwrite(&pixel, 1, 3, stdout); } // Padding for(int p=0; p<padding; p++){ fputc(0, stdout); } } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag |
1 2 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; int height = 200; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (unsigned char)(width & 0xFF), (unsigned char)((width >> 8) & 0xFF), 0, 0, // Width (unsigned char)(height & 0xFF), (unsigned char)((height >> 8) & 0xFF), 0, 0, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB = 0) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; int imageSize = width * height * 3; int fileSize = imageSize + 54; // Update file size in header header[2] = fileSize & 0xFF; header[3] = (fileSize >> 8) & 0xFF; header[4] = (fileSize >> 16) & 0xFF; header[5] = (fileSize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB pixel; int padding = (4 - (width * 3) % 4) % 4; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green pixel.r = 0; pixel.g = 146; // Slightly darker green for better visibility pixel.b = 70; } else if (x < 2 * width / 3) { // White pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { // Red pixel.r = 206; // Slightly darker red for better visibility pixel.g = 43; pixel.b = 55; } fwrite(&pixel, 1, 3, stdout); } // Padding for(int p=0; p<padding; p++){ fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | #include <stdio.h> #include <stdlib.h> typedef struct { unsigned char b, g, r; } RGB; int main() { int width = 300; int height = 200; // BMP header unsigned char header[54] = { 'B', 'M', // Signature 0, 0, 0, 0, // File size (placeholder) 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // Size of DIB header (unsigned char)(width & 0xFF), (unsigned char)((width >> 8) & 0xFF), 0, 0, // Width (unsigned char)(height & 0xFF), (unsigned char)((height >> 8) & 0xFF), 0, 0, // Height 1, 0, // Number of color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method (BI_RGB = 0) 0, 0, 0, 0, // Image size (can be 0 for BI_RGB) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors in palette 0, 0, 0, 0 // Number of important colors }; int imageSize = width * height * 3; int fileSize = imageSize + 54; // Update file size in header header[2] = fileSize & 0xFF; header[3] = (fileSize >> 8) & 0xFF; header[4] = (fileSize >> 16) & 0xFF; header[5] = (fileSize >> 24) & 0xFF; fwrite(header, 1, 54, stdout); RGB pixel; int padding = (4 - (width * 3) % 4) % 4; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green pixel.r = 0; pixel.g = 146; // Slightly darker green for better visibility pixel.b = 70; } else if (x < 2 * width / 3) { // White pixel.r = 255; pixel.g = 255; pixel.b = 255; } else { // Red pixel.r = 206; // Slightly darker red for better visibility pixel.g = 43; pixel.b = 55; } fwrite(&pixel, 1, 3, stdout); } // Padding for(int p=0; p<padding; p++){ fputc(0, stdout); } } return 0; } |
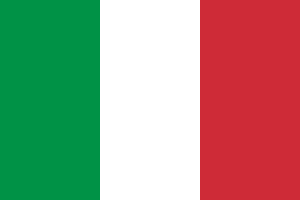
What flag is shown in this image?
The flag shown is the national flag of Italy.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True