Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP image of the Italian flag and writes it to stdout. The Italian flag consists of three vertical stripes: green, white, and red.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) void write_bmp_header(FILE *f, int width, int height) { BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; int rowSize = (3 * width + 3) & (~3); int imageSize = rowSize * height; fileHeader.bfType = 0x4D42; fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + imageSize; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; infoHeader.biCompression = 0; infoHeader.biSizeImage = imageSize; infoHeader.biXPelsPerMeter = 2835; infoHeader.biYPelsPerMeter = 2835; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; fwrite(&fileHeader, sizeof(fileHeader), 1, f); fwrite(&infoHeader, sizeof(infoHeader), 1, f); } void write_pixel(FILE *f, uint8_t r, uint8_t g, uint8_t b) { fwrite(&b, 1, 1, f); fwrite(&g, 1, 1, f); fwrite(&r, 1, 1, f); } int main() { int width = 300; int height = 200; int stripeWidth = width / 3; write_bmp_header(stdout, width, height); for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < stripeWidth) { write_pixel(stdout, 0, 146, 70); // Green } else if (x < 2 * stripeWidth) { write_pixel(stdout, 255, 255, 255); // White } else { write_pixel(stdout, 206, 43, 55); // Red } } // Padding for 4-byte alignment for (int p = 0; p < (4 - (width * 3) % 4) % 4; p++) { fputc(0, stdout); } } return 0; } |
1 | gcc -o italian_flag italian_flag.c |
1 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) void write_bmp_header(FILE *f, int width, int height) { BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; int rowSize = (3 * width + 3) & (~3); int imageSize = rowSize * height; fileHeader.bfType = 0x4D42; fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + imageSize; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; infoHeader.biCompression = 0; infoHeader.biSizeImage = imageSize; infoHeader.biXPelsPerMeter = 2835; infoHeader.biYPelsPerMeter = 2835; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; fwrite(&fileHeader, sizeof(fileHeader), 1, f); fwrite(&infoHeader, sizeof(infoHeader), 1, f); } void write_pixel(FILE *f, uint8_t r, uint8_t g, uint8_t b) { fwrite(&b, 1, 1, f); fwrite(&g, 1, 1, f); fwrite(&r, 1, 1, f); } int main() { int width = 300; int height = 200; int stripeWidth = width / 3; write_bmp_header(stdout, width, height); for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < stripeWidth) { write_pixel(stdout, 0, 146, 70); // Green } else if (x < 2 * stripeWidth) { write_pixel(stdout, 255, 255, 255); // White } else { write_pixel(stdout, 206, 43, 55); // Red } } // Padding for 4-byte alignment for (int p = 0; p < (4 - (width * 3) % 4) % 4; p++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) void write_bmp_header(FILE *f, int width, int height) { BITMAPFILEHEADER fileHeader; BITMAPINFOHEADER infoHeader; int rowSize = (3 * width + 3) & (~3); int imageSize = rowSize * height; fileHeader.bfType = 0x4D42; fileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + imageSize; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER); infoHeader.biSize = sizeof(BITMAPINFOHEADER); infoHeader.biWidth = width; infoHeader.biHeight = height; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; infoHeader.biCompression = 0; infoHeader.biSizeImage = imageSize; infoHeader.biXPelsPerMeter = 2835; infoHeader.biYPelsPerMeter = 2835; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; fwrite(&fileHeader, sizeof(fileHeader), 1, f); fwrite(&infoHeader, sizeof(infoHeader), 1, f); } void write_pixel(FILE *f, uint8_t r, uint8_t g, uint8_t b) { fwrite(&b, 1, 1, f); fwrite(&g, 1, 1, f); fwrite(&r, 1, 1, f); } int main() { int width = 300; int height = 200; int stripeWidth = width / 3; write_bmp_header(stdout, width, height); for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < stripeWidth) { write_pixel(stdout, 0, 146, 70); // Green } else if (x < 2 * stripeWidth) { write_pixel(stdout, 255, 255, 255); // White } else { write_pixel(stdout, 206, 43, 55); // Red } } // Padding for 4-byte alignment for (int p = 0; p < (4 - (width * 3) % 4) % 4; p++) { fputc(0, stdout); } } return 0; } |
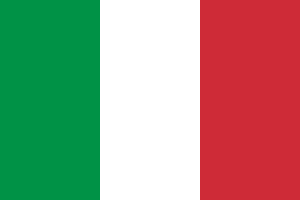
What flag is shown in this image?
The flag shown is the national flag of Italy.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True