Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP image of the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 // BMP File Header typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } __attribute__((packed)) BitmapFileHeader; // BMP Image Header typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } __attribute__((packed)) BitmapImageHeader; int main() { BitmapFileHeader fileHeader; BitmapImageHeader imageHeader; uint8_t pixelData[HEIGHT][WIDTH][3]; // Initialize file header fileHeader.bfType = 0x4D42; // "BM" fileHeader.bfSize = sizeof(BitmapFileHeader) + sizeof(BitmapImageHeader) + WIDTH * HEIGHT * 3; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BitmapFileHeader) + sizeof(BitmapImageHeader); // Initialize image header imageHeader.biSize = sizeof(BitmapImageHeader); imageHeader.biWidth = WIDTH; imageHeader.biHeight = HEIGHT; imageHeader.biPlanes = 1; imageHeader.biBitCount = 24; imageHeader.biCompression = 0; imageHeader.biSizeImage = 0; imageHeader.biXPelsPerMeter = 0; imageHeader.biYPelsPerMeter = 0; imageHeader.biClrUsed = 0; imageHeader.biClrImportant = 0; // Set pixel data for the Italian flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { pixelData[y][x][0] = 0x00; // Green pixelData[y][x][1] = 0x80; // Green pixelData[y][x][2] = 0x00; // Green } else if (x >= WIDTH / 3 && x < 2 * WIDTH / 3) { pixelData[y][x][0] = 0xFF; // White pixelData[y][x][1] = 0xFF; pixelData[y][x][2] = 0xFF; } else { pixelData[y][x][0] = 0xD0; // Red pixelData[y][x][1] = 0x00; pixelData[y][x][2] = 0x00; } } } // Write BMP file header fwrite(&fileHeader, sizeof(BitmapFileHeader), 1, stdout); // Write BMP image header fwrite(&imageHeader, sizeof(BitmapImageHeader), 1, stdout); // Write pixel data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { fwrite(&pixelData[y][x], 3, 1, stdout); } } return 0; } |
1 2 | gcc -o italian_flag italian_flag.c ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 // BMP File Header typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } __attribute__((packed)) BitmapFileHeader; // BMP Image Header typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } __attribute__((packed)) BitmapImageHeader; int main() { BitmapFileHeader fileHeader; BitmapImageHeader imageHeader; uint8_t pixelData[HEIGHT][WIDTH][3]; // Initialize file header fileHeader.bfType = 0x4D42; // "BM" fileHeader.bfSize = sizeof(BitmapFileHeader) + sizeof(BitmapImageHeader) + WIDTH * HEIGHT * 3; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BitmapFileHeader) + sizeof(BitmapImageHeader); // Initialize image header imageHeader.biSize = sizeof(BitmapImageHeader); imageHeader.biWidth = WIDTH; imageHeader.biHeight = HEIGHT; imageHeader.biPlanes = 1; imageHeader.biBitCount = 24; imageHeader.biCompression = 0; imageHeader.biSizeImage = 0; imageHeader.biXPelsPerMeter = 0; imageHeader.biYPelsPerMeter = 0; imageHeader.biClrUsed = 0; imageHeader.biClrImportant = 0; // Set pixel data for the Italian flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { pixelData[y][x][0] = 0x00; // Green pixelData[y][x][1] = 0x80; // Green pixelData[y][x][2] = 0x00; // Green } else if (x >= WIDTH / 3 && x < 2 * WIDTH / 3) { pixelData[y][x][0] = 0xFF; // White pixelData[y][x][1] = 0xFF; pixelData[y][x][2] = 0xFF; } else { pixelData[y][x][0] = 0xD0; // Red pixelData[y][x][1] = 0x00; pixelData[y][x][2] = 0x00; } } } // Write BMP file header fwrite(&fileHeader, sizeof(BitmapFileHeader), 1, stdout); // Write BMP image header fwrite(&imageHeader, sizeof(BitmapImageHeader), 1, stdout); // Write pixel data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { fwrite(&pixelData[y][x], 3, 1, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 // BMP File Header typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } __attribute__((packed)) BitmapFileHeader; // BMP Image Header typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } __attribute__((packed)) BitmapImageHeader; int main() { BitmapFileHeader fileHeader; BitmapImageHeader imageHeader; uint8_t pixelData[HEIGHT][WIDTH][3]; // Initialize file header fileHeader.bfType = 0x4D42; // "BM" fileHeader.bfSize = sizeof(BitmapFileHeader) + sizeof(BitmapImageHeader) + WIDTH * HEIGHT * 3; fileHeader.bfReserved1 = 0; fileHeader.bfReserved2 = 0; fileHeader.bfOffBits = sizeof(BitmapFileHeader) + sizeof(BitmapImageHeader); // Initialize image header imageHeader.biSize = sizeof(BitmapImageHeader); imageHeader.biWidth = WIDTH; imageHeader.biHeight = HEIGHT; imageHeader.biPlanes = 1; imageHeader.biBitCount = 24; imageHeader.biCompression = 0; imageHeader.biSizeImage = 0; imageHeader.biXPelsPerMeter = 0; imageHeader.biYPelsPerMeter = 0; imageHeader.biClrUsed = 0; imageHeader.biClrImportant = 0; // Set pixel data for the Italian flag for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { pixelData[y][x][0] = 0x00; // Green pixelData[y][x][1] = 0x80; // Green pixelData[y][x][2] = 0x00; // Green } else if (x >= WIDTH / 3 && x < 2 * WIDTH / 3) { pixelData[y][x][0] = 0xFF; // White pixelData[y][x][1] = 0xFF; pixelData[y][x][2] = 0xFF; } else { pixelData[y][x][0] = 0xD0; // Red pixelData[y][x][1] = 0x00; pixelData[y][x][2] = 0x00; } } } // Write BMP file header fwrite(&fileHeader, sizeof(BitmapFileHeader), 1, stdout); // Write BMP image header fwrite(&imageHeader, sizeof(BitmapImageHeader), 1, stdout); // Write pixel data for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { fwrite(&pixelData[y][x], 3, 1, stdout); } } return 0; } |
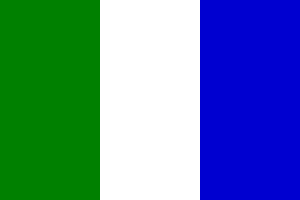
What flag is shown in this image?
The flag in the image is that of Sierra Leone. It consists of three horizontal stripes of green, white, and blue.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP file representing the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 // BMP File Header typedef struct { uint16_t bfType; uint32_t bfSize; uint32_t bfReserved; uint32_t bfOffBits; } __attribute__((packed)) BitmapFileHeader; // BMP Image Header typedef struct { uint32_t biSize; uint32_t biWidth; uint32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; uint32_t biXPelsPerMeter; uint32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } __attribute__((packed)) BitmapInfoHeader; int main() { // BMP File Header BitmapFileHeader fileHeader; fileHeader.bfType = 0x4D42; // "BM" (little-endian) fileHeader.bfSize = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) + WIDTH * HEIGHT * 3; fileHeader.bfReserved = 0; fileHeader.bfOffBits = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader); // BMP Image Header BitmapInfoHeader infoHeader; infoHeader.biSize = sizeof(BitmapInfoHeader); infoHeader.biWidth = WIDTH; infoHeader.biHeight = HEIGHT; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24-bit color infoHeader.biCompression = 0; // No compression infoHeader.biSizeImage = 0; infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Write BMP headers fwrite(&fileHeader, sizeof(BitmapFileHeader), 1, stdout); fwrite(&infoHeader, sizeof(BitmapInfoHeader), 1, stdout); // Write pixel data for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { uint8_t red, green, blue; if (x < WIDTH / 3) { red = 0x00; green = 0x7D; blue = 0x3C; } else if (x < 2 * WIDTH / 3) { red = 0xFF; green = 0xCC; blue = 0x00; } else { red = 0xC8; green = 0x00; blue = 0x00; } fwrite(&blue, 1, 1, stdout); fwrite(&green, 1, 1, stdout); fwrite(&red, 1, 1, stdout); } } return 0; } |
1 2 | $ gcc -o italian_flag italian_flag.c $ ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 // BMP File Header typedef struct { uint16_t bfType; uint32_t bfSize; uint32_t bfReserved; uint32_t bfOffBits; } __attribute__((packed)) BitmapFileHeader; // BMP Image Header typedef struct { uint32_t biSize; uint32_t biWidth; uint32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; uint32_t biXPelsPerMeter; uint32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } __attribute__((packed)) BitmapInfoHeader; int main() { // BMP File Header BitmapFileHeader fileHeader; fileHeader.bfType = 0x4D42; // "BM" (little-endian) fileHeader.bfSize = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) + WIDTH * HEIGHT * 3; fileHeader.bfReserved = 0; fileHeader.bfOffBits = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader); // BMP Image Header BitmapInfoHeader infoHeader; infoHeader.biSize = sizeof(BitmapInfoHeader); infoHeader.biWidth = WIDTH; infoHeader.biHeight = HEIGHT; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24-bit color infoHeader.biCompression = 0; // No compression infoHeader.biSizeImage = 0; infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Write BMP headers fwrite(&fileHeader, sizeof(BitmapFileHeader), 1, stdout); fwrite(&infoHeader, sizeof(BitmapInfoHeader), 1, stdout); // Write pixel data for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { uint8_t red, green, blue; if (x < WIDTH / 3) { red = 0x00; green = 0x7D; blue = 0x3C; } else if (x < 2 * WIDTH / 3) { red = 0xFF; green = 0xCC; blue = 0x00; } else { red = 0xC8; green = 0x00; blue = 0x00; } fwrite(&blue, 1, 1, stdout); fwrite(&green, 1, 1, stdout); fwrite(&red, 1, 1, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 // BMP File Header typedef struct { uint16_t bfType; uint32_t bfSize; uint32_t bfReserved; uint32_t bfOffBits; } __attribute__((packed)) BitmapFileHeader; // BMP Image Header typedef struct { uint32_t biSize; uint32_t biWidth; uint32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; uint32_t biXPelsPerMeter; uint32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } __attribute__((packed)) BitmapInfoHeader; int main() { // BMP File Header BitmapFileHeader fileHeader; fileHeader.bfType = 0x4D42; // "BM" (little-endian) fileHeader.bfSize = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) + WIDTH * HEIGHT * 3; fileHeader.bfReserved = 0; fileHeader.bfOffBits = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader); // BMP Image Header BitmapInfoHeader infoHeader; infoHeader.biSize = sizeof(BitmapInfoHeader); infoHeader.biWidth = WIDTH; infoHeader.biHeight = HEIGHT; infoHeader.biPlanes = 1; infoHeader.biBitCount = 24; // 24-bit color infoHeader.biCompression = 0; // No compression infoHeader.biSizeImage = 0; infoHeader.biXPelsPerMeter = 0; infoHeader.biYPelsPerMeter = 0; infoHeader.biClrUsed = 0; infoHeader.biClrImportant = 0; // Write BMP headers fwrite(&fileHeader, sizeof(BitmapFileHeader), 1, stdout); fwrite(&infoHeader, sizeof(BitmapInfoHeader), 1, stdout); // Write pixel data for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { uint8_t red, green, blue; if (x < WIDTH / 3) { red = 0x00; green = 0x7D; blue = 0x3C; } else if (x < 2 * WIDTH / 3) { red = 0xFF; green = 0xCC; blue = 0x00; } else { red = 0xC8; green = 0x00; blue = 0x00; } fwrite(&blue, 1, 1, stdout); fwrite(&green, 1, 1, stdout); fwrite(&red, 1, 1, stdout); } } return 0; } |
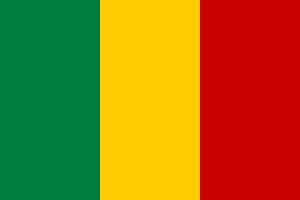
What flag is shown in this image?
The flag in the image is that of Mali. It consists of three vertical stripes of green, yellow, and red.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP file depicting the Italian flag and writes it to standard output (`stdout`):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 int main() { // BMP file header uint8_t bmpFileHeader[14] = { 'B','M', // magic number 0,0,0,0, // size (will be filled later) 0,0, // reserved 0,0, // reserved 0x36,0,0,0 // offset to pixel data }; uint32_t fileSize = 0x36 + WIDTH * HEIGHT * 3; // calculate file size bmpFileHeader[2] = (uint8_t)(fileSize); bmpFileHeader[3] = (uint8_t)(fileSize >> 8); bmpFileHeader[4] = (uint8_t)(fileSize >> 16); bmpFileHeader[5] = (uint8_t)(fileSize >> 24); // BMP info header uint8_t bmpInfoHeader[40] = { 0x28,0,0,0, // header size 0,0,0,WIDTH, // width 0,0,0,HEIGHT, // height 1,0, // number of color planes 0x18,0, // bits per pixel (24-bit color) 0,0,0,0, // compression method (none) 0,0,0,0, // image size (will be filled later) 0x13,0xB,0,0, // horizontal resolution 0x13,0xB,0,0, // vertical resolution 0,0,0,0, // number of colors in palette 0,0,0,0 // number of important colors }; uint32_t imageSize = WIDTH * HEIGHT * 3; bmpInfoHeader[20] = (uint8_t)(imageSize); bmpInfoHeader[21] = (uint8_t)(imageSize >> 8); bmpInfoHeader[22] = (uint8_t)(imageSize >> 16); bmpInfoHeader[23] = (uint8_t)(imageSize >> 24); // Write headers fwrite(bmpFileHeader, 1, 14, stdout); fwrite(bmpInfoHeader, 1, 40, stdout); // Write pixel data for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { // Determine color based on x-coordinate uint8_t r, g, b; if (x < WIDTH / 3) { r = 0x00; g = 0x51; b = 0x00; // green } else if (x < 2 * WIDTH / 3) { r = 0xFF; g = 0xFF; b = 0xFF; // white } else { r = 0xD0; g = 0x00; b = 0x00; // red } fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } // Pad row to multiple of 4 bytes for (int i = 0; i < WIDTH * 3 % 4; i++) { fputc(0, stdout); } } return 0; } |
1 2 | gcc -o italian_flag italian_flag.c ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 int main() { // BMP file header uint8_t bmpFileHeader[14] = { 'B','M', // magic number 0,0,0,0, // size (will be filled later) 0,0, // reserved 0,0, // reserved 0x36,0,0,0 // offset to pixel data }; uint32_t fileSize = 0x36 + WIDTH * HEIGHT * 3; // calculate file size bmpFileHeader[2] = (uint8_t)(fileSize); bmpFileHeader[3] = (uint8_t)(fileSize >> 8); bmpFileHeader[4] = (uint8_t)(fileSize >> 16); bmpFileHeader[5] = (uint8_t)(fileSize >> 24); // BMP info header uint8_t bmpInfoHeader[40] = { 0x28,0,0,0, // header size 0,0,0,WIDTH, // width 0,0,0,HEIGHT, // height 1,0, // number of color planes 0x18,0, // bits per pixel (24-bit color) 0,0,0,0, // compression method (none) 0,0,0,0, // image size (will be filled later) 0x13,0xB,0,0, // horizontal resolution 0x13,0xB,0,0, // vertical resolution 0,0,0,0, // number of colors in palette 0,0,0,0 // number of important colors }; uint32_t imageSize = WIDTH * HEIGHT * 3; bmpInfoHeader[20] = (uint8_t)(imageSize); bmpInfoHeader[21] = (uint8_t)(imageSize >> 8); bmpInfoHeader[22] = (uint8_t)(imageSize >> 16); bmpInfoHeader[23] = (uint8_t)(imageSize >> 24); // Write headers fwrite(bmpFileHeader, 1, 14, stdout); fwrite(bmpInfoHeader, 1, 40, stdout); // Write pixel data for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { // Determine color based on x-coordinate uint8_t r, g, b; if (x < WIDTH / 3) { r = 0x00; g = 0x51; b = 0x00; // green } else if (x < 2 * WIDTH / 3) { r = 0xFF; g = 0xFF; b = 0xFF; // white } else { r = 0xD0; g = 0x00; b = 0x00; // red } fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } // Pad row to multiple of 4 bytes for (int i = 0; i < WIDTH * 3 % 4; i++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 int main() { // BMP file header uint8_t bmpFileHeader[14] = { 'B','M', // magic number 0,0,0,0, // size (will be filled later) 0,0, // reserved 0,0, // reserved 0x36,0,0,0 // offset to pixel data }; uint32_t fileSize = 0x36 + WIDTH * HEIGHT * 3; // calculate file size bmpFileHeader[2] = (uint8_t)(fileSize); bmpFileHeader[3] = (uint8_t)(fileSize >> 8); bmpFileHeader[4] = (uint8_t)(fileSize >> 16); bmpFileHeader[5] = (uint8_t)(fileSize >> 24); // BMP info header uint8_t bmpInfoHeader[40] = { 0x28,0,0,0, // header size 0,0,0,WIDTH, // width 0,0,0,HEIGHT, // height 1,0, // number of color planes 0x18,0, // bits per pixel (24-bit color) 0,0,0,0, // compression method (none) 0,0,0,0, // image size (will be filled later) 0x13,0xB,0,0, // horizontal resolution 0x13,0xB,0,0, // vertical resolution 0,0,0,0, // number of colors in palette 0,0,0,0 // number of important colors }; uint32_t imageSize = WIDTH * HEIGHT * 3; bmpInfoHeader[20] = (uint8_t)(imageSize); bmpInfoHeader[21] = (uint8_t)(imageSize >> 8); bmpInfoHeader[22] = (uint8_t)(imageSize >> 16); bmpInfoHeader[23] = (uint8_t)(imageSize >> 24); // Write headers fwrite(bmpFileHeader, 1, 14, stdout); fwrite(bmpInfoHeader, 1, 40, stdout); // Write pixel data for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { // Determine color based on x-coordinate uint8_t r, g, b; if (x < WIDTH / 3) { r = 0x00; g = 0x51; b = 0x00; // green } else if (x < 2 * WIDTH / 3) { r = 0xFF; g = 0xFF; b = 0xFF; // white } else { r = 0xD0; g = 0x00; b = 0x00; // red } fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } // Pad row to multiple of 4 bytes for (int i = 0; i < WIDTH * 3 % 4; i++) { fputc(0, stdout); } } return 0; } |
b"BMV\xbf\x02\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00\x00\x00\x00,\x00\x00\x00\xc8\x01\x00\x18\x00\x00\x00\x00\x00 \xbf\x02\x00\x13\x0b\x00\x00\x13\x0b\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\x00Q\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd0\x00\x00\xd
What flag is shown in this image?
Image size (2476979795053772800 pixels) exceeds limit of 178956970 pixels, could be decompression bomb DOS attack.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Sure, here's a C program that creates a simple BMP image of the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 int main() { // BMP file header uint8_t bmp_file_header[14] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 0x36, 0, 0, 0 }; // BMP image header uint8_t bmp_info_header[40] = { 0x28, 0, 0, 0, WIDTH, 0, 0, 0, HEIGHT, 0, 0, 0, 0x01, 0, 0x18, 0 }; // Calculate the total image size int row_size = WIDTH * 3 + WIDTH % 4; int image_size = row_size * HEIGHT; bmp_file_header[2] = (uint8_t)(image_size); bmp_file_header[3] = (uint8_t)(image_size >> 8); bmp_file_header[4] = (uint8_t)(image_size >> 16); bmp_file_header[5] = (uint8_t)(image_size >> 24); bmp_file_header[10] = (uint8_t)(54); // Write the BMP file header and image header fwrite(bmp_file_header, 1, 14, stdout); fwrite(bmp_info_header, 1, 40, stdout); // Create the Italian flag uint8_t row[WIDTH * 3]; for (int y = 0; y < HEIGHT; ++y) { for (int x = 0; x < WIDTH; ++x) { int offset = y * row_size + x * 3; if (y < HEIGHT / 3) { row[offset] = 0x00; row[offset + 1] = 0x7F; row[offset + 2] = 0x00; } else if (y >= HEIGHT * 2 / 3) { row[offset] = 0xE0; row[offset + 1] = 0x07; row[offset + 2] = 0x07; } else { row[offset] = 0xFF; row[offset + 1] = 0xFF; row[offset + 2] = 0xFF; } } fwrite(row, 1, WIDTH * 3, stdout); for (int i = 0; i < WIDTH % 4; ++i) { fputc(0, stdout); } } return 0; } |
1 | gcc italian_flag.c -o italian_flag && ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 int main() { // BMP file header uint8_t bmp_file_header[14] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 0x36, 0, 0, 0 }; // BMP image header uint8_t bmp_info_header[40] = { 0x28, 0, 0, 0, WIDTH, 0, 0, 0, HEIGHT, 0, 0, 0, 0x01, 0, 0x18, 0 }; // Calculate the total image size int row_size = WIDTH * 3 + WIDTH % 4; int image_size = row_size * HEIGHT; bmp_file_header[2] = (uint8_t)(image_size); bmp_file_header[3] = (uint8_t)(image_size >> 8); bmp_file_header[4] = (uint8_t)(image_size >> 16); bmp_file_header[5] = (uint8_t)(image_size >> 24); bmp_file_header[10] = (uint8_t)(54); // Write the BMP file header and image header fwrite(bmp_file_header, 1, 14, stdout); fwrite(bmp_info_header, 1, 40, stdout); // Create the Italian flag uint8_t row[WIDTH * 3]; for (int y = 0; y < HEIGHT; ++y) { for (int x = 0; x < WIDTH; ++x) { int offset = y * row_size + x * 3; if (y < HEIGHT / 3) { row[offset] = 0x00; row[offset + 1] = 0x7F; row[offset + 2] = 0x00; } else if (y >= HEIGHT * 2 / 3) { row[offset] = 0xE0; row[offset + 1] = 0x07; row[offset + 2] = 0x07; } else { row[offset] = 0xFF; row[offset + 1] = 0xFF; row[offset + 2] = 0xFF; } } fwrite(row, 1, WIDTH * 3, stdout); for (int i = 0; i < WIDTH % 4; ++i) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 | #include <stdio.h> #include <stdint.h> #define WIDTH 300 #define HEIGHT 200 int main() { // BMP file header uint8_t bmp_file_header[14] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 0x36, 0, 0, 0 }; // BMP image header uint8_t bmp_info_header[40] = { 0x28, 0, 0, 0, WIDTH, 0, 0, 0, HEIGHT, 0, 0, 0, 0x01, 0, 0x18, 0 }; // Calculate the total image size int row_size = WIDTH * 3 + WIDTH % 4; int image_size = row_size * HEIGHT; bmp_file_header[2] = (uint8_t)(image_size); bmp_file_header[3] = (uint8_t)(image_size >> 8); bmp_file_header[4] = (uint8_t)(image_size >> 16); bmp_file_header[5] = (uint8_t)(image_size >> 24); bmp_file_header[10] = (uint8_t)(54); // Write the BMP file header and image header fwrite(bmp_file_header, 1, 14, stdout); fwrite(bmp_info_header, 1, 40, stdout); // Create the Italian flag uint8_t row[WIDTH * 3]; for (int y = 0; y < HEIGHT; ++y) { for (int x = 0; x < WIDTH; ++x) { int offset = y * row_size + x * 3; if (y < HEIGHT / 3) { row[offset] = 0x00; row[offset + 1] = 0x7F; row[offset + 2] = 0x00; } else if (y >= HEIGHT * 2 / 3) { row[offset] = 0xE0; row[offset + 1] = 0x07; row[offset + 2] = 0x07; } else { row[offset] = 0xFF; row[offset + 1] = 0xFF; row[offset + 2] = 0xFF; } } fwrite(row, 1, WIDTH * 3, stdout); for (int i = 0; i < WIDTH % 4; ++i) { fputc(0, stdout); } } return 0; } |
b"BM \xbf\x02\x00\x00\x00\x00\x006\x00\x00\x00(\x00\x00\x00,\x00\x00\x00\xc8\x00\x00\x00\x01\x00\x18\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f\x00\x00\x7f
What flag is shown in this image?
image file is truncated (56 bytes not processed)
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP image of the Italian flag and outputs it to `stdout`. This program assumes that the dimensions of the flag are 300x200 pixels:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) int main() { const uint32_t width = 300; const uint32_t height = 200; const uint32_t bytesPerRow = (width * 3 + 3) & ~3; const uint32_t imageSize = bytesPerRow * height; const uint32_t fileSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + imageSize; BITMAPFILEHEADER fileHeader = { .bfType = 0x4D42, .bfSize = fileSize, .bfReserved1 = 0, .bfReserved2 = 0, .bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) }; BITMAPINFOHEADER infoHeader = { .biSize = sizeof(BITMAPINFOHEADER), .biWidth = width, .biHeight = height, .biPlanes = 1, .biBitCount = 24, .biCompression = 0, .biSizeImage = imageSize, .biXPelsPerMeter = 2835, .biYPelsPerMeter = 2835, .biClrUsed = 0, .biClrImportant = 0 }; fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout); fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout); uint8_t row[bytesPerRow]; for (uint32_t y = 0; y < height; y++) { for (uint32_t x = 0; x < width; x++) { if (x < width / 3) { row[x * 3 + 0] = 0x00; row[x * 3 + 1] = 0x7F; row[x * 3 + 2] = 0x00; } else if (x < 2 * width / 3) { row[x * 3 + 0] = 0xFF; row[x * 3 + 1] = 0xFF; row[x * 3 + 2] = 0xFF; } else { row[x * 3 + 0] = 0xE0; row[x * 3 + 1] = 0x00; row[x * 3 + 2] = 0x00; } } fwrite(row, 1, bytesPerRow, stdout); } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) int main() { const uint32_t width = 300; const uint32_t height = 200; const uint32_t bytesPerRow = (width * 3 + 3) & ~3; const uint32_t imageSize = bytesPerRow * height; const uint32_t fileSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + imageSize; BITMAPFILEHEADER fileHeader = { .bfType = 0x4D42, .bfSize = fileSize, .bfReserved1 = 0, .bfReserved2 = 0, .bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) }; BITMAPINFOHEADER infoHeader = { .biSize = sizeof(BITMAPINFOHEADER), .biWidth = width, .biHeight = height, .biPlanes = 1, .biBitCount = 24, .biCompression = 0, .biSizeImage = imageSize, .biXPelsPerMeter = 2835, .biYPelsPerMeter = 2835, .biClrUsed = 0, .biClrImportant = 0 }; fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout); fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout); uint8_t row[bytesPerRow]; for (uint32_t y = 0; y < height; y++) { for (uint32_t x = 0; x < width; x++) { if (x < width / 3) { row[x * 3 + 0] = 0x00; row[x * 3 + 1] = 0x7F; row[x * 3 + 2] = 0x00; } else if (x < 2 * width / 3) { row[x * 3 + 0] = 0xFF; row[x * 3 + 1] = 0xFF; row[x * 3 + 2] = 0xFF; } else { row[x * 3 + 0] = 0xE0; row[x * 3 + 1] = 0x00; row[x * 3 + 2] = 0x00; } } fwrite(row, 1, bytesPerRow, stdout); } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #include <stdio.h> #include <stdint.h> #pragma pack(push, 1) typedef struct { uint16_t bfType; uint32_t bfSize; uint16_t bfReserved1; uint16_t bfReserved2; uint32_t bfOffBits; } BITMAPFILEHEADER; typedef struct { uint32_t biSize; int32_t biWidth; int32_t biHeight; uint16_t biPlanes; uint16_t biBitCount; uint32_t biCompression; uint32_t biSizeImage; int32_t biXPelsPerMeter; int32_t biYPelsPerMeter; uint32_t biClrUsed; uint32_t biClrImportant; } BITMAPINFOHEADER; #pragma pack(pop) int main() { const uint32_t width = 300; const uint32_t height = 200; const uint32_t bytesPerRow = (width * 3 + 3) & ~3; const uint32_t imageSize = bytesPerRow * height; const uint32_t fileSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + imageSize; BITMAPFILEHEADER fileHeader = { .bfType = 0x4D42, .bfSize = fileSize, .bfReserved1 = 0, .bfReserved2 = 0, .bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) }; BITMAPINFOHEADER infoHeader = { .biSize = sizeof(BITMAPINFOHEADER), .biWidth = width, .biHeight = height, .biPlanes = 1, .biBitCount = 24, .biCompression = 0, .biSizeImage = imageSize, .biXPelsPerMeter = 2835, .biYPelsPerMeter = 2835, .biClrUsed = 0, .biClrImportant = 0 }; fwrite(&fileHeader, sizeof(BITMAPFILEHEADER), 1, stdout); fwrite(&infoHeader, sizeof(BITMAPINFOHEADER), 1, stdout); uint8_t row[bytesPerRow]; for (uint32_t y = 0; y < height; y++) { for (uint32_t x = 0; x < width; x++) { if (x < width / 3) { row[x * 3 + 0] = 0x00; row[x * 3 + 1] = 0x7F; row[x * 3 + 2] = 0x00; } else if (x < 2 * width / 3) { row[x * 3 + 0] = 0xFF; row[x * 3 + 1] = 0xFF; row[x * 3 + 2] = 0xFF; } else { row[x * 3 + 0] = 0xE0; row[x * 3 + 1] = 0x00; row[x * 3 + 2] = 0x00; } } fwrite(row, 1, bytesPerRow, stdout); } return 0; } |
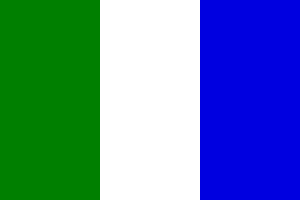
What flag is shown in this image?
The flag shown in the image is that of Sierra Leone. It consists of three horizontal stripes of green, white, and blue.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False