Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP image of the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PIXEL_SIZE (WIDTH * HEIGHT * BYTES_PER_PIXEL) void write_bmp_header(int width, int height) { printf("BM"); uint32_t filesize = 54 + PIXEL_SIZE; fwrite(&filesize, sizeof(uint32_t), 1, stdout); uint32_t reserved = 0; fwrite(&reserved, sizeof(uint32_t), 1, stdout); uint32_t data_offset = 54; fwrite(&data_offset, sizeof(uint32_t), 1, stdout); uint32_t header_size = 40; fwrite(&header_size, sizeof(uint32_t), 1, stdout); fwrite(&width, sizeof(int), 1, stdout); fwrite(&height, sizeof(int), 1, stdout); uint16_t planes = 1; fwrite(&planes, sizeof(uint16_t), 1, stdout); uint16_t bits_per_pixel = BYTES_PER_PIXEL * 8; fwrite(&bits_per_pixel, sizeof(uint16_t), 1, stdout); uint32_t compression = 0; fwrite(&compression, sizeof(uint32_t), 1, stdout); fwrite(&PIXEL_SIZE, sizeof(uint32_t), 1, stdout); uint32_t ppm_x = 2835; uint32_t ppm_y = 2835; fwrite(&ppm_x, sizeof(uint32_t), 1, stdout); fwrite(&ppm_y, sizeof(uint32_t), 1, stdout); uint32_t colors_used = 0; fwrite(&colors_used, sizeof(uint32_t), 1, stdout); uint32_t important_colors = 0; fwrite(&important_colors, sizeof(uint32_t), 1, stdout); } void write_pixel(uint8_t red, uint8_t green, uint8_t blue) { fwrite(&blue, sizeof(uint8_t), 1, stdout); fwrite(&green, sizeof(uint8_t), 1, stdout); fwrite(&red, sizeof(uint8_t), 1, stdout); } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(244, 245, 240); // White } else { write_pixel(206, 43, 55); // Red } } } return 0; } |
1 2 | gcc -o italian_flag italian_flag.c ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PIXEL_SIZE (WIDTH * HEIGHT * BYTES_PER_PIXEL) void write_bmp_header(int width, int height) { printf("BM"); uint32_t filesize = 54 + PIXEL_SIZE; fwrite(&filesize, sizeof(uint32_t), 1, stdout); uint32_t reserved = 0; fwrite(&reserved, sizeof(uint32_t), 1, stdout); uint32_t data_offset = 54; fwrite(&data_offset, sizeof(uint32_t), 1, stdout); uint32_t header_size = 40; fwrite(&header_size, sizeof(uint32_t), 1, stdout); fwrite(&width, sizeof(int), 1, stdout); fwrite(&height, sizeof(int), 1, stdout); uint16_t planes = 1; fwrite(&planes, sizeof(uint16_t), 1, stdout); uint16_t bits_per_pixel = BYTES_PER_PIXEL * 8; fwrite(&bits_per_pixel, sizeof(uint16_t), 1, stdout); uint32_t compression = 0; fwrite(&compression, sizeof(uint32_t), 1, stdout); fwrite(&PIXEL_SIZE, sizeof(uint32_t), 1, stdout); uint32_t ppm_x = 2835; uint32_t ppm_y = 2835; fwrite(&ppm_x, sizeof(uint32_t), 1, stdout); fwrite(&ppm_y, sizeof(uint32_t), 1, stdout); uint32_t colors_used = 0; fwrite(&colors_used, sizeof(uint32_t), 1, stdout); uint32_t important_colors = 0; fwrite(&important_colors, sizeof(uint32_t), 1, stdout); } void write_pixel(uint8_t red, uint8_t green, uint8_t blue) { fwrite(&blue, sizeof(uint8_t), 1, stdout); fwrite(&green, sizeof(uint8_t), 1, stdout); fwrite(&red, sizeof(uint8_t), 1, stdout); } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(244, 245, 240); // White } else { write_pixel(206, 43, 55); // Red } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PIXEL_SIZE (WIDTH * HEIGHT * BYTES_PER_PIXEL) void write_bmp_header(int width, int height) { printf("BM"); uint32_t filesize = 54 + PIXEL_SIZE; fwrite(&filesize, sizeof(uint32_t), 1, stdout); uint32_t reserved = 0; fwrite(&reserved, sizeof(uint32_t), 1, stdout); uint32_t data_offset = 54; fwrite(&data_offset, sizeof(uint32_t), 1, stdout); uint32_t header_size = 40; fwrite(&header_size, sizeof(uint32_t), 1, stdout); fwrite(&width, sizeof(int), 1, stdout); fwrite(&height, sizeof(int), 1, stdout); uint16_t planes = 1; fwrite(&planes, sizeof(uint16_t), 1, stdout); uint16_t bits_per_pixel = BYTES_PER_PIXEL * 8; fwrite(&bits_per_pixel, sizeof(uint16_t), 1, stdout); uint32_t compression = 0; fwrite(&compression, sizeof(uint32_t), 1, stdout); fwrite(&PIXEL_SIZE, sizeof(uint32_t), 1, stdout); uint32_t ppm_x = 2835; uint32_t ppm_y = 2835; fwrite(&ppm_x, sizeof(uint32_t), 1, stdout); fwrite(&ppm_y, sizeof(uint32_t), 1, stdout); uint32_t colors_used = 0; fwrite(&colors_used, sizeof(uint32_t), 1, stdout); uint32_t important_colors = 0; fwrite(&important_colors, sizeof(uint32_t), 1, stdout); } void write_pixel(uint8_t red, uint8_t green, uint8_t blue) { fwrite(&blue, sizeof(uint8_t), 1, stdout); fwrite(&green, sizeof(uint8_t), 1, stdout); fwrite(&red, sizeof(uint8_t), 1, stdout); } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(244, 245, 240); // White } else { write_pixel(206, 43, 55); // Red } } } return 0; } |
b"main.c: In function 'write_bmp_header':\nmain.c:28:12: error: lvalue required as unary '&' operand\n 28 | fwrite(&PIXEL_SIZE, sizeof(uint32_t), 1, stdout);\n | ^\nmain.sh: line 2: ./a.out: No such file or directory\n"
What flag is shown in this image?
cannot identify image file <_io.BytesIO object at 0x7ffa23b840e0>
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP image of the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PADDING 2 void write_bmp_header(int width, int height) { int filesize = 54 + (width * BYTES_PER_PIXEL + PADDING) * height; uint8_t header[] = { 0x42, 0x4D, // BM filesize, filesize >> 8, filesize >> 16, filesize >> 24, // File size 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // DIB header size width, width >> 8, width >> 16, width >> 24, // Width height, height >> 8, height >> 16, height >> 24, // Height 1, 0, // Color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method 0, 0, 0, 0, // Image size (uncompressed) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors 0, 0, 0, 0 // Important colors }; fwrite(header, 1, sizeof(header), stdout); } void write_pixel(uint8_t r, uint8_t g, uint8_t b) { fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } void write_padding(int padding) { for (int i = 0; i < padding; i++) { fputc(0, stdout); } } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(255, 255, 255); // White } else { write_pixel(206, 43, 55); // Red } } write_padding(PADDING); } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PADDING 2 void write_bmp_header(int width, int height) { int filesize = 54 + (width * BYTES_PER_PIXEL + PADDING) * height; uint8_t header[] = { 0x42, 0x4D, // BM filesize, filesize >> 8, filesize >> 16, filesize >> 24, // File size 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // DIB header size width, width >> 8, width >> 16, width >> 24, // Width height, height >> 8, height >> 16, height >> 24, // Height 1, 0, // Color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method 0, 0, 0, 0, // Image size (uncompressed) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors 0, 0, 0, 0 // Important colors }; fwrite(header, 1, sizeof(header), stdout); } void write_pixel(uint8_t r, uint8_t g, uint8_t b) { fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } void write_padding(int padding) { for (int i = 0; i < padding; i++) { fputc(0, stdout); } } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(255, 255, 255); // White } else { write_pixel(206, 43, 55); // Red } } write_padding(PADDING); } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PADDING 2 void write_bmp_header(int width, int height) { int filesize = 54 + (width * BYTES_PER_PIXEL + PADDING) * height; uint8_t header[] = { 0x42, 0x4D, // BM filesize, filesize >> 8, filesize >> 16, filesize >> 24, // File size 0, 0, 0, 0, // Reserved 54, 0, 0, 0, // Offset to pixel data 40, 0, 0, 0, // DIB header size width, width >> 8, width >> 16, width >> 24, // Width height, height >> 8, height >> 16, height >> 24, // Height 1, 0, // Color planes 24, 0, // Bits per pixel 0, 0, 0, 0, // Compression method 0, 0, 0, 0, // Image size (uncompressed) 0, 0, 0, 0, // Horizontal resolution 0, 0, 0, 0, // Vertical resolution 0, 0, 0, 0, // Number of colors 0, 0, 0, 0 // Important colors }; fwrite(header, 1, sizeof(header), stdout); } void write_pixel(uint8_t r, uint8_t g, uint8_t b) { fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } void write_padding(int padding) { for (int i = 0; i < padding; i++) { fputc(0, stdout); } } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(255, 255, 255); // White } else { write_pixel(206, 43, 55); // Red } } write_padding(PADDING); } return 0; } |
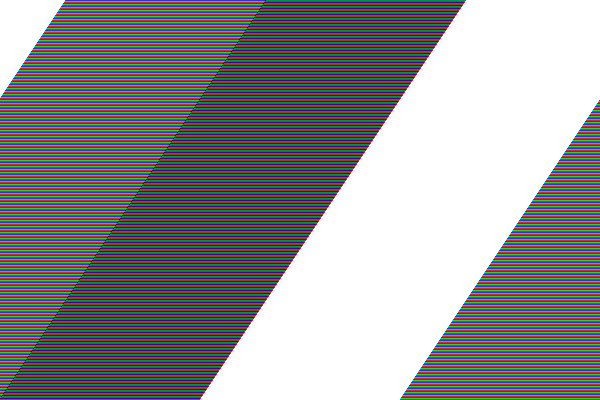
What flag is shown in this image?
The image appears to be a digital or stylized representation of a flag, but the design has been altered with a moiré pattern effect, which makes it difficult to identify the flag accurately. The moiré pattern is created by overlaying repetitive details such as lines or dots, which can create an interference pattern. Due to this effect, I cannot provide a definitive answer about which flag is depicted. If you can provide a clearer image or more context, I might be able to assist you further.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP image of the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PADDING 0 void write_bmp_header(int width, int height) { int bitmap_size = (width * BYTES_PER_PIXEL + PADDING) * height; int file_size = 54 + bitmap_size; putchar('B'); putchar('M'); fwrite(&file_size, sizeof(int), 1, stdout); fwrite("\0\0\0\0", 1, 4, stdout); putchar(54); putchar(0); putchar(0); putchar(0); putchar(40); putchar(0); putchar(0); putchar(0); fwrite(&width, sizeof(int), 1, stdout); fwrite(&height, sizeof(int), 1, stdout); putchar(1); putchar(0); putchar(24); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); fwrite(&bitmap_size, sizeof(int), 1, stdout); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); } void write_pixel(uint8_t red, uint8_t green, uint8_t blue) { putchar(blue); putchar(green); putchar(red); } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(244, 245, 240); // White } else { write_pixel(206, 43, 55); // Red } } for (int p = 0; p < PADDING; p++) { putchar(0); } } return 0; } |
1 2 | gcc -o italian_flag italian_flag.c ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PADDING 0 void write_bmp_header(int width, int height) { int bitmap_size = (width * BYTES_PER_PIXEL + PADDING) * height; int file_size = 54 + bitmap_size; putchar('B'); putchar('M'); fwrite(&file_size, sizeof(int), 1, stdout); fwrite("\0\0\0\0", 1, 4, stdout); putchar(54); putchar(0); putchar(0); putchar(0); putchar(40); putchar(0); putchar(0); putchar(0); fwrite(&width, sizeof(int), 1, stdout); fwrite(&height, sizeof(int), 1, stdout); putchar(1); putchar(0); putchar(24); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); fwrite(&bitmap_size, sizeof(int), 1, stdout); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); } void write_pixel(uint8_t red, uint8_t green, uint8_t blue) { putchar(blue); putchar(green); putchar(red); } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(244, 245, 240); // White } else { write_pixel(206, 43, 55); // Red } } for (int p = 0; p < PADDING; p++) { putchar(0); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PADDING 0 void write_bmp_header(int width, int height) { int bitmap_size = (width * BYTES_PER_PIXEL + PADDING) * height; int file_size = 54 + bitmap_size; putchar('B'); putchar('M'); fwrite(&file_size, sizeof(int), 1, stdout); fwrite("\0\0\0\0", 1, 4, stdout); putchar(54); putchar(0); putchar(0); putchar(0); putchar(40); putchar(0); putchar(0); putchar(0); fwrite(&width, sizeof(int), 1, stdout); fwrite(&height, sizeof(int), 1, stdout); putchar(1); putchar(0); putchar(24); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); fwrite(&bitmap_size, sizeof(int), 1, stdout); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); putchar(0); } void write_pixel(uint8_t red, uint8_t green, uint8_t blue) { putchar(blue); putchar(green); putchar(red); } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(244, 245, 240); // White } else { write_pixel(206, 43, 55); // Red } } for (int p = 0; p < PADDING; p++) { putchar(0); } } return 0; } |
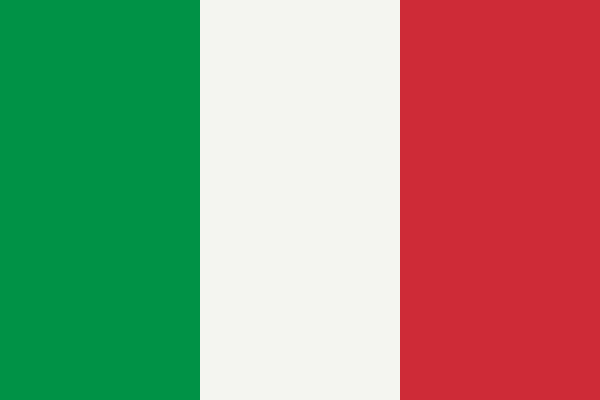
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three equally sized vertical bands of green, white, and red.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP image of the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PADDING 2 void write_bmp_header(int width, int height) { int row_size = (width * BYTES_PER_PIXEL + PADDING) & ~PADDING; int bitmap_size = row_size * height; int file_size = 54 + bitmap_size; putchar('B'); putchar('M'); fwrite(&file_size, sizeof(int), 1, stdout); fwrite("\0\0\0\0", 1, 4, stdout); putchar(54); putchar(0); putchar(0); putchar(0); putchar(40); putchar(0); putchar(0); putchar(0); fwrite(&width, sizeof(int), 1, stdout); fwrite(&height, sizeof(int), 1, stdout); putchar(1); putchar(0); putchar(24); putchar(0); fwrite("\0\0\0\0", 1, 4, stdout); fwrite(&bitmap_size, sizeof(int), 1, stdout); fwrite("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0", 1, 16, stdout); } void write_pixel(uint8_t red, uint8_t green, uint8_t blue) { putchar(blue); putchar(green); putchar(red); } void write_padding(int padding) { for (int i = 0; i < padding; i++) { putchar(0); } } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 140, 69); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(244, 245, 240); // White } else { write_pixel(206, 43, 55); // Red } } write_padding(PADDING); } return 0; } |
1 2 | gcc -o italian_flag italian_flag.c ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PADDING 2 void write_bmp_header(int width, int height) { int row_size = (width * BYTES_PER_PIXEL + PADDING) & ~PADDING; int bitmap_size = row_size * height; int file_size = 54 + bitmap_size; putchar('B'); putchar('M'); fwrite(&file_size, sizeof(int), 1, stdout); fwrite("\0\0\0\0", 1, 4, stdout); putchar(54); putchar(0); putchar(0); putchar(0); putchar(40); putchar(0); putchar(0); putchar(0); fwrite(&width, sizeof(int), 1, stdout); fwrite(&height, sizeof(int), 1, stdout); putchar(1); putchar(0); putchar(24); putchar(0); fwrite("\0\0\0\0", 1, 4, stdout); fwrite(&bitmap_size, sizeof(int), 1, stdout); fwrite("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0", 1, 16, stdout); } void write_pixel(uint8_t red, uint8_t green, uint8_t blue) { putchar(blue); putchar(green); putchar(red); } void write_padding(int padding) { for (int i = 0; i < padding; i++) { putchar(0); } } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 140, 69); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(244, 245, 240); // White } else { write_pixel(206, 43, 55); // Red } } write_padding(PADDING); } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PADDING 2 void write_bmp_header(int width, int height) { int row_size = (width * BYTES_PER_PIXEL + PADDING) & ~PADDING; int bitmap_size = row_size * height; int file_size = 54 + bitmap_size; putchar('B'); putchar('M'); fwrite(&file_size, sizeof(int), 1, stdout); fwrite("\0\0\0\0", 1, 4, stdout); putchar(54); putchar(0); putchar(0); putchar(0); putchar(40); putchar(0); putchar(0); putchar(0); fwrite(&width, sizeof(int), 1, stdout); fwrite(&height, sizeof(int), 1, stdout); putchar(1); putchar(0); putchar(24); putchar(0); fwrite("\0\0\0\0", 1, 4, stdout); fwrite(&bitmap_size, sizeof(int), 1, stdout); fwrite("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0", 1, 16, stdout); } void write_pixel(uint8_t red, uint8_t green, uint8_t blue) { putchar(blue); putchar(green); putchar(red); } void write_padding(int padding) { for (int i = 0; i < padding; i++) { putchar(0); } } int main() { write_bmp_header(WIDTH, HEIGHT); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 140, 69); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(244, 245, 240); // White } else { write_pixel(206, 43, 55); // Red } } write_padding(PADDING); } return 0; } |
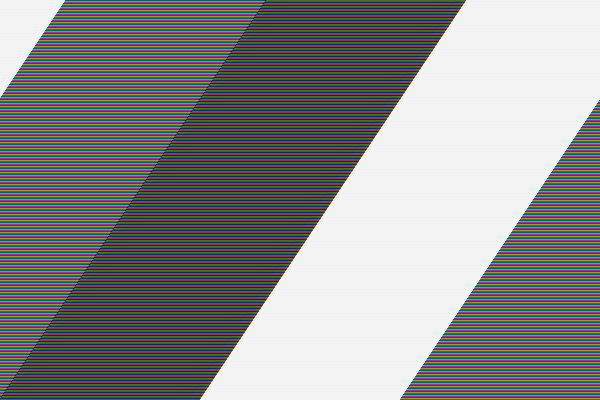
What flag is shown in this image?
The image appears to be corrupted or altered, displaying a pattern of horizontal lines instead of a clear image of a flag. Due to the distortion, I cannot accurately identify a flag from this image. If you have a clearer image of a flag, I would be happy to help identify it.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP image of the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PIXEL_SIZE (WIDTH * HEIGHT * BYTES_PER_PIXEL) void write_bmp_header(int width, int height) { printf("BM"); printf("%c%c%c%c", 54 + PIXEL_SIZE, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 54, 0, 0, 0); printf("%c%c%c%c", 40, 0, 0, 0); printf("%c%c%c%c", width, width >> 8, width >> 16, width >> 24); printf("%c%c%c%c", height, height >> 8, height >> 16, height >> 24); printf("%c%c", 1, 0); printf("%c%c", BYTES_PER_PIXEL * 8, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", PIXEL_SIZE, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); } void set_pixel(uint8_t *pixels, int x, int y, uint8_t r, uint8_t g, uint8_t b) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = b; pixels[index + 1] = g; pixels[index + 2] = r; } int main() { uint8_t pixels[PIXEL_SIZE]; for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { set_pixel(pixels, x, y, 0, 146, 70); // Green } else if (x < WIDTH * 2 / 3) { set_pixel(pixels, x, y, 255, 255, 255); // White } else { set_pixel(pixels, x, y, 206, 43, 55); // Red } } } write_bmp_header(WIDTH, HEIGHT); fwrite(pixels, 1, PIXEL_SIZE, stdout); return 0; } |
1 2 | gcc italian_flag.c -o italian_flag ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PIXEL_SIZE (WIDTH * HEIGHT * BYTES_PER_PIXEL) void write_bmp_header(int width, int height) { printf("BM"); printf("%c%c%c%c", 54 + PIXEL_SIZE, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 54, 0, 0, 0); printf("%c%c%c%c", 40, 0, 0, 0); printf("%c%c%c%c", width, width >> 8, width >> 16, width >> 24); printf("%c%c%c%c", height, height >> 8, height >> 16, height >> 24); printf("%c%c", 1, 0); printf("%c%c", BYTES_PER_PIXEL * 8, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", PIXEL_SIZE, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); } void set_pixel(uint8_t *pixels, int x, int y, uint8_t r, uint8_t g, uint8_t b) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = b; pixels[index + 1] = g; pixels[index + 2] = r; } int main() { uint8_t pixels[PIXEL_SIZE]; for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { set_pixel(pixels, x, y, 0, 146, 70); // Green } else if (x < WIDTH * 2 / 3) { set_pixel(pixels, x, y, 255, 255, 255); // White } else { set_pixel(pixels, x, y, 206, 43, 55); // Red } } } write_bmp_header(WIDTH, HEIGHT); fwrite(pixels, 1, PIXEL_SIZE, stdout); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 | #include <stdio.h> #include <stdint.h> #define WIDTH 600 #define HEIGHT 400 #define BYTES_PER_PIXEL 3 #define PIXEL_SIZE (WIDTH * HEIGHT * BYTES_PER_PIXEL) void write_bmp_header(int width, int height) { printf("BM"); printf("%c%c%c%c", 54 + PIXEL_SIZE, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 54, 0, 0, 0); printf("%c%c%c%c", 40, 0, 0, 0); printf("%c%c%c%c", width, width >> 8, width >> 16, width >> 24); printf("%c%c%c%c", height, height >> 8, height >> 16, height >> 24); printf("%c%c", 1, 0); printf("%c%c", BYTES_PER_PIXEL * 8, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", PIXEL_SIZE, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); printf("%c%c%c%c", 0, 0, 0, 0); } void set_pixel(uint8_t *pixels, int x, int y, uint8_t r, uint8_t g, uint8_t b) { int index = (y * WIDTH + x) * BYTES_PER_PIXEL; pixels[index] = b; pixels[index + 1] = g; pixels[index + 2] = r; } int main() { uint8_t pixels[PIXEL_SIZE]; for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { set_pixel(pixels, x, y, 0, 146, 70); // Green } else if (x < WIDTH * 2 / 3) { set_pixel(pixels, x, y, 255, 255, 255); // White } else { set_pixel(pixels, x, y, 206, 43, 55); // Red } } } write_bmp_header(WIDTH, HEIGHT); fwrite(pixels, 1, PIXEL_SIZE, stdout); return 0; } |
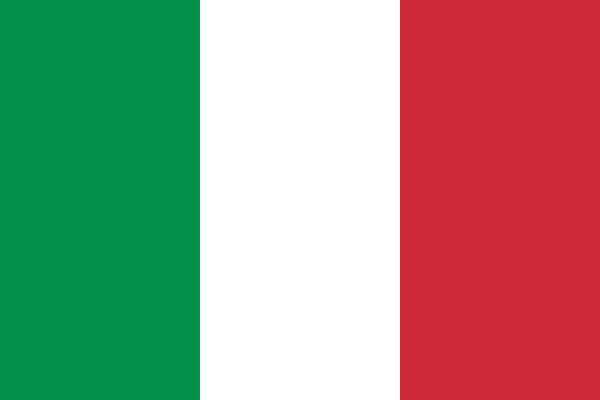
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three equal vertical bands of green (hoist side), white, and red.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True