Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP file representing the Italian flag (vertical stripes of green, white, and red) and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; // Magic identifier "BM" uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data in bytes } BMPHeader; typedef struct { uint32_t size; // Header size in bytes int32_t width; // Width of image int32_t height; // Height of image uint16_t planes; // Number of color planes uint16_t bits; // Bits per pixel uint32_t compression; // Compression type uint32_t imagesize; // Image size in bytes int32_t xresolution; // Pixels per meter int32_t yresolution; // Pixels per meter uint32_t ncolors; // Number of colors uint32_t importantcolors; // Important colors } BMPInfoHeader; #pragma pack(pop) int main() { // Define flag dimensions const int width = 300; // Width of the flag const int height = 200; // Height of the flag const int stripe_width = width / 3; // Width of each stripe // Initialize headers BMPHeader header = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + width * height * 3, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits = 24, // 24-bit color .compression = 0, .imagesize = width * height * 3, .xresolution = 2835, // 72 DPI .yresolution = 2835, // 72 DPI .ncolors = 0, .importantcolors = 0 }; // Write headers to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Define colors (BGR format) const unsigned char green[] = {0, 146, 70}; // Italian flag green const unsigned char white[] = {255, 255, 255}; // White const unsigned char red[] = {41, 33, 205}; // Italian flag red // Generate and write pixel data // BMP stores pixels bottom-to-top, left-to-right for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { const unsigned char* color; if (x < stripe_width) { color = green; } else if (x < stripe_width * 2) { color = white; } else { color = red; } fwrite(color, 1, 3, stdout); } // BMP rows must be padded to multiple of 4 bytes int padding = (width * 3) % 4; if (padding) { const unsigned char pad[3] = {0, 0, 0}; fwrite(pad, 1, padding, stdout); } } return 0; } |
1 2 | gcc italianflag.c -o italianflag ./italianflag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; // Magic identifier "BM" uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data in bytes } BMPHeader; typedef struct { uint32_t size; // Header size in bytes int32_t width; // Width of image int32_t height; // Height of image uint16_t planes; // Number of color planes uint16_t bits; // Bits per pixel uint32_t compression; // Compression type uint32_t imagesize; // Image size in bytes int32_t xresolution; // Pixels per meter int32_t yresolution; // Pixels per meter uint32_t ncolors; // Number of colors uint32_t importantcolors; // Important colors } BMPInfoHeader; #pragma pack(pop) int main() { // Define flag dimensions const int width = 300; // Width of the flag const int height = 200; // Height of the flag const int stripe_width = width / 3; // Width of each stripe // Initialize headers BMPHeader header = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + width * height * 3, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits = 24, // 24-bit color .compression = 0, .imagesize = width * height * 3, .xresolution = 2835, // 72 DPI .yresolution = 2835, // 72 DPI .ncolors = 0, .importantcolors = 0 }; // Write headers to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Define colors (BGR format) const unsigned char green[] = {0, 146, 70}; // Italian flag green const unsigned char white[] = {255, 255, 255}; // White const unsigned char red[] = {41, 33, 205}; // Italian flag red // Generate and write pixel data // BMP stores pixels bottom-to-top, left-to-right for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { const unsigned char* color; if (x < stripe_width) { color = green; } else if (x < stripe_width * 2) { color = white; } else { color = red; } fwrite(color, 1, 3, stdout); } // BMP rows must be padded to multiple of 4 bytes int padding = (width * 3) % 4; if (padding) { const unsigned char pad[3] = {0, 0, 0}; fwrite(pad, 1, padding, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; // Magic identifier "BM" uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data in bytes } BMPHeader; typedef struct { uint32_t size; // Header size in bytes int32_t width; // Width of image int32_t height; // Height of image uint16_t planes; // Number of color planes uint16_t bits; // Bits per pixel uint32_t compression; // Compression type uint32_t imagesize; // Image size in bytes int32_t xresolution; // Pixels per meter int32_t yresolution; // Pixels per meter uint32_t ncolors; // Number of colors uint32_t importantcolors; // Important colors } BMPInfoHeader; #pragma pack(pop) int main() { // Define flag dimensions const int width = 300; // Width of the flag const int height = 200; // Height of the flag const int stripe_width = width / 3; // Width of each stripe // Initialize headers BMPHeader header = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + width * height * 3, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits = 24, // 24-bit color .compression = 0, .imagesize = width * height * 3, .xresolution = 2835, // 72 DPI .yresolution = 2835, // 72 DPI .ncolors = 0, .importantcolors = 0 }; // Write headers to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Define colors (BGR format) const unsigned char green[] = {0, 146, 70}; // Italian flag green const unsigned char white[] = {255, 255, 255}; // White const unsigned char red[] = {41, 33, 205}; // Italian flag red // Generate and write pixel data // BMP stores pixels bottom-to-top, left-to-right for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { const unsigned char* color; if (x < stripe_width) { color = green; } else if (x < stripe_width * 2) { color = white; } else { color = red; } fwrite(color, 1, 3, stdout); } // BMP rows must be padded to multiple of 4 bytes int padding = (width * 3) % 4; if (padding) { const unsigned char pad[3] = {0, 0, 0}; fwrite(pad, 1, padding, stdout); } } return 0; } |
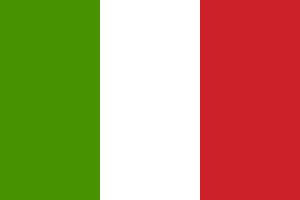
What flag is shown in this image?
The flag shown in the image is the national flag of Italy.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP file representing the Italian flag (vertical stripes of green, white, and red) and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; // Magic identifier "BM" uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data in bytes } BMPHeader; typedef struct { uint32_t size; // Header size in bytes int32_t width; // Width of image int32_t height; // Height of image uint16_t planes; // Number of color planes uint16_t bits; // Bits per pixel uint32_t compression; // Compression type uint32_t imagesize; // Image size in bytes int32_t xresolution; // Pixels per meter int32_t yresolution; // Pixels per meter uint32_t ncolors; // Number of colors uint32_t importantcolors; // Important colors } BMPInfoHeader; #pragma pack(pop) int main() { // Define flag dimensions const int width = 300; // Width of the flag const int height = 200; // Height of the flag const int padding = (4 - (width * 3) % 4) % 4; // Padding bytes for each row // Create headers BMPHeader header = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (width * 3 + padding) * height, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader infoHeader = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits = 24, .compression = 0, .imagesize = (width * 3 + padding) * height, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .importantcolors = 0 }; // Write headers to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(&infoHeader, sizeof(infoHeader), 1, stdout); // Write pixel data // BMP stores pixels bottom-to-top, left-to-right for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { unsigned char pixel[3]; // Determine color based on x position (Italian flag: green, white, red) if (x < width / 3) { // Green pixel[0] = 0; // Blue pixel[1] = 146; // Green pixel[2] = 70; // Red } else if (x < 2 * width / 3) { // White pixel[0] = 255; // Blue pixel[1] = 255; // Green pixel[2] = 255; // Red } else { // Red pixel[0] = 0; // Blue pixel[1] = 0; // Green pixel[2] = 255; // Red } fwrite(pixel, 1, 3, stdout); } // Write padding bytes const unsigned char pad = 0; for (int p = 0; p < padding; p++) { fwrite(&pad, 1, 1, stdout); } } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag ./italian_flag > flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; // Magic identifier "BM" uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data in bytes } BMPHeader; typedef struct { uint32_t size; // Header size in bytes int32_t width; // Width of image int32_t height; // Height of image uint16_t planes; // Number of color planes uint16_t bits; // Bits per pixel uint32_t compression; // Compression type uint32_t imagesize; // Image size in bytes int32_t xresolution; // Pixels per meter int32_t yresolution; // Pixels per meter uint32_t ncolors; // Number of colors uint32_t importantcolors; // Important colors } BMPInfoHeader; #pragma pack(pop) int main() { // Define flag dimensions const int width = 300; // Width of the flag const int height = 200; // Height of the flag const int padding = (4 - (width * 3) % 4) % 4; // Padding bytes for each row // Create headers BMPHeader header = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (width * 3 + padding) * height, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader infoHeader = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits = 24, .compression = 0, .imagesize = (width * 3 + padding) * height, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .importantcolors = 0 }; // Write headers to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(&infoHeader, sizeof(infoHeader), 1, stdout); // Write pixel data // BMP stores pixels bottom-to-top, left-to-right for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { unsigned char pixel[3]; // Determine color based on x position (Italian flag: green, white, red) if (x < width / 3) { // Green pixel[0] = 0; // Blue pixel[1] = 146; // Green pixel[2] = 70; // Red } else if (x < 2 * width / 3) { // White pixel[0] = 255; // Blue pixel[1] = 255; // Green pixel[2] = 255; // Red } else { // Red pixel[0] = 0; // Blue pixel[1] = 0; // Green pixel[2] = 255; // Red } fwrite(pixel, 1, 3, stdout); } // Write padding bytes const unsigned char pad = 0; for (int p = 0; p < padding; p++) { fwrite(&pad, 1, 1, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; // Magic identifier "BM" uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data in bytes } BMPHeader; typedef struct { uint32_t size; // Header size in bytes int32_t width; // Width of image int32_t height; // Height of image uint16_t planes; // Number of color planes uint16_t bits; // Bits per pixel uint32_t compression; // Compression type uint32_t imagesize; // Image size in bytes int32_t xresolution; // Pixels per meter int32_t yresolution; // Pixels per meter uint32_t ncolors; // Number of colors uint32_t importantcolors; // Important colors } BMPInfoHeader; #pragma pack(pop) int main() { // Define flag dimensions const int width = 300; // Width of the flag const int height = 200; // Height of the flag const int padding = (4 - (width * 3) % 4) % 4; // Padding bytes for each row // Create headers BMPHeader header = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + (width * 3 + padding) * height, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader infoHeader = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits = 24, .compression = 0, .imagesize = (width * 3 + padding) * height, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .importantcolors = 0 }; // Write headers to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(&infoHeader, sizeof(infoHeader), 1, stdout); // Write pixel data // BMP stores pixels bottom-to-top, left-to-right for (int y = height - 1; y >= 0; y--) { for (int x = 0; x < width; x++) { unsigned char pixel[3]; // Determine color based on x position (Italian flag: green, white, red) if (x < width / 3) { // Green pixel[0] = 0; // Blue pixel[1] = 146; // Green pixel[2] = 70; // Red } else if (x < 2 * width / 3) { // White pixel[0] = 255; // Blue pixel[1] = 255; // Green pixel[2] = 255; // Red } else { // Red pixel[0] = 0; // Blue pixel[1] = 0; // Green pixel[2] = 255; // Red } fwrite(pixel, 1, 3, stdout); } // Write padding bytes const unsigned char pad = 0; for (int p = 0; p < padding; p++) { fwrite(&pad, 1, 1, stdout); } } return 0; } |
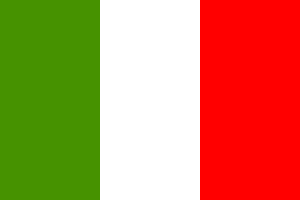
What flag is shown in this image?
The flag shown in the image is the national flag of Italy.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP file representing the Italian flag (vertical tricolor of green, white, and red) and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; int32_t x_pixels_per_m; int32_t y_pixels_per_m; uint32_t colors_used; uint32_t important_colors; } BMPInfoHeader; #pragma pack(pop) int main() { // Flag dimensions const int width = 300; // Width must be divisible by 3 for equal stripes const int height = 200; const int bytes_per_pixel = 3; const int padding = (4 - (width * bytes_per_pixel) % 4) % 4; const int row_size = width * bytes_per_pixel + padding; // Create headers BMPHeader header = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + height * row_size, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info_header = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = height * row_size, .x_pixels_per_m = 2835, .y_pixels_per_m = 2835, .colors_used = 0, .important_colors = 0 }; // Write headers fwrite(&header, sizeof(header), 1, stdout); fwrite(&info_header, sizeof(info_header), 1, stdout); // Write pixel data uint8_t padding_bytes[3] = {0, 0, 0}; uint8_t pixel[3]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { // Determine color based on x position if (x < width/3) { // Green (left stripe) pixel[0] = 0; // Blue pixel[1] = 146; // Green pixel[2] = 70; // Red } else if (x < 2*width/3) { // White (middle stripe) pixel[0] = 255; // Blue pixel[1] = 255; // Green pixel[2] = 255; // Red } else { // Red (right stripe) pixel[0] = 0; // Blue pixel[1] = 0; // Green pixel[2] = 206; // Red } fwrite(pixel, 1, 3, stdout); } // Write padding bytes if (padding > 0) { fwrite(padding_bytes, 1, padding, stdout); } } return 0; } |
1 | gcc italian_flag.c -o italian_flag |
1 | ./italian_flag > italy.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; int32_t x_pixels_per_m; int32_t y_pixels_per_m; uint32_t colors_used; uint32_t important_colors; } BMPInfoHeader; #pragma pack(pop) int main() { // Flag dimensions const int width = 300; // Width must be divisible by 3 for equal stripes const int height = 200; const int bytes_per_pixel = 3; const int padding = (4 - (width * bytes_per_pixel) % 4) % 4; const int row_size = width * bytes_per_pixel + padding; // Create headers BMPHeader header = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + height * row_size, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info_header = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = height * row_size, .x_pixels_per_m = 2835, .y_pixels_per_m = 2835, .colors_used = 0, .important_colors = 0 }; // Write headers fwrite(&header, sizeof(header), 1, stdout); fwrite(&info_header, sizeof(info_header), 1, stdout); // Write pixel data uint8_t padding_bytes[3] = {0, 0, 0}; uint8_t pixel[3]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { // Determine color based on x position if (x < width/3) { // Green (left stripe) pixel[0] = 0; // Blue pixel[1] = 146; // Green pixel[2] = 70; // Red } else if (x < 2*width/3) { // White (middle stripe) pixel[0] = 255; // Blue pixel[1] = 255; // Green pixel[2] = 255; // Red } else { // Red (right stripe) pixel[0] = 0; // Blue pixel[1] = 0; // Green pixel[2] = 206; // Red } fwrite(pixel, 1, 3, stdout); } // Write padding bytes if (padding > 0) { fwrite(padding_bytes, 1, padding, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size; int32_t x_pixels_per_m; int32_t y_pixels_per_m; uint32_t colors_used; uint32_t important_colors; } BMPInfoHeader; #pragma pack(pop) int main() { // Flag dimensions const int width = 300; // Width must be divisible by 3 for equal stripes const int height = 200; const int bytes_per_pixel = 3; const int padding = (4 - (width * bytes_per_pixel) % 4) % 4; const int row_size = width * bytes_per_pixel + padding; // Create headers BMPHeader header = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + height * row_size, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info_header = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits_per_pixel = 24, .compression = 0, .image_size = height * row_size, .x_pixels_per_m = 2835, .y_pixels_per_m = 2835, .colors_used = 0, .important_colors = 0 }; // Write headers fwrite(&header, sizeof(header), 1, stdout); fwrite(&info_header, sizeof(info_header), 1, stdout); // Write pixel data uint8_t padding_bytes[3] = {0, 0, 0}; uint8_t pixel[3]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { // Determine color based on x position if (x < width/3) { // Green (left stripe) pixel[0] = 0; // Blue pixel[1] = 146; // Green pixel[2] = 70; // Red } else if (x < 2*width/3) { // White (middle stripe) pixel[0] = 255; // Blue pixel[1] = 255; // Green pixel[2] = 255; // Red } else { // Red (right stripe) pixel[0] = 0; // Blue pixel[1] = 0; // Green pixel[2] = 206; // Red } fwrite(pixel, 1, 3, stdout); } // Write padding bytes if (padding > 0) { fwrite(padding_bytes, 1, padding, stdout); } } return 0; } |
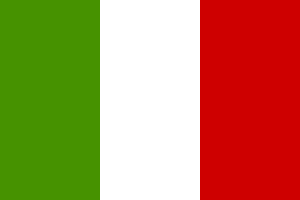
What flag is shown in this image?
The flag shown in the image is the national flag of Italy.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP file representing the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits; uint32_t compression; uint32_t imagesize; int32_t xresolution; int32_t yresolution; uint32_t ncolors; uint32_t importantcolors; } BMPInfoHeader; #pragma pack(pop) int main() { // Define flag dimensions const int width = 300; // Width of the flag const int height = 200; // Height of the flag const int stripe_width = width / 3; // Width of each color stripe // Calculate padding (BMP rows must be multiple of 4 bytes) const int padding = (4 - (width * 3) % 4) % 4; // Calculate total size const int imagesize = height * (width * 3 + padding); const int filesize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + imagesize; // Create and fill BMP header BMPHeader header = { .type = 0x4D42, // "BM" .size = filesize, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; // Create and fill BMP info header BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits = 24, .compression = 0, .imagesize = imagesize, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .importantcolors = 0 }; // Write headers to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Write pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { unsigned char r, g, b; // Determine color based on x position if (x < stripe_width) { // Green stripe r = 0; g = 146; b = 70; } else if (x < stripe_width * 2) { // White stripe r = 255; g = 255; b = 255; } else { // Red stripe r = 206; g = 43; b = 55; } // Write RGB values fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } // Add padding for (int p = 0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
1 | gcc italian_flag.c -o italian_flag |
1 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits; uint32_t compression; uint32_t imagesize; int32_t xresolution; int32_t yresolution; uint32_t ncolors; uint32_t importantcolors; } BMPInfoHeader; #pragma pack(pop) int main() { // Define flag dimensions const int width = 300; // Width of the flag const int height = 200; // Height of the flag const int stripe_width = width / 3; // Width of each color stripe // Calculate padding (BMP rows must be multiple of 4 bytes) const int padding = (4 - (width * 3) % 4) % 4; // Calculate total size const int imagesize = height * (width * 3 + padding); const int filesize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + imagesize; // Create and fill BMP header BMPHeader header = { .type = 0x4D42, // "BM" .size = filesize, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; // Create and fill BMP info header BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits = 24, .compression = 0, .imagesize = imagesize, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .importantcolors = 0 }; // Write headers to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Write pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { unsigned char r, g, b; // Determine color based on x position if (x < stripe_width) { // Green stripe r = 0; g = 146; b = 70; } else if (x < stripe_width * 2) { // White stripe r = 255; g = 255; b = 255; } else { // Red stripe r = 206; g = 43; b = 55; } // Write RGB values fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } // Add padding for (int p = 0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } BMPHeader; typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bits; uint32_t compression; uint32_t imagesize; int32_t xresolution; int32_t yresolution; uint32_t ncolors; uint32_t importantcolors; } BMPInfoHeader; #pragma pack(pop) int main() { // Define flag dimensions const int width = 300; // Width of the flag const int height = 200; // Height of the flag const int stripe_width = width / 3; // Width of each color stripe // Calculate padding (BMP rows must be multiple of 4 bytes) const int padding = (4 - (width * 3) % 4) % 4; // Calculate total size const int imagesize = height * (width * 3 + padding); const int filesize = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + imagesize; // Create and fill BMP header BMPHeader header = { .type = 0x4D42, // "BM" .size = filesize, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; // Create and fill BMP info header BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits = 24, .compression = 0, .imagesize = imagesize, .xresolution = 2835, .yresolution = 2835, .ncolors = 0, .importantcolors = 0 }; // Write headers to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Write pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { unsigned char r, g, b; // Determine color based on x position if (x < stripe_width) { // Green stripe r = 0; g = 146; b = 70; } else if (x < stripe_width * 2) { // White stripe r = 255; g = 255; b = 255; } else { // Red stripe r = 206; g = 43; b = 55; } // Write RGB values fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } // Add padding for (int p = 0; p < padding; p++) { fputc(0, stdout); } } return 0; } |
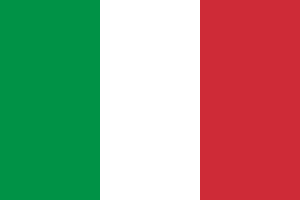
What flag is shown in this image?
The flag shown is the national flag of Italy.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP file representing the Italian flag (three vertical stripes in green, white, and red) and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; // Magic identifier "BM" uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data in bytes } BMPHeader; typedef struct { uint32_t size; // Header size in bytes int32_t width; // Width of image int32_t height; // Height of image uint16_t planes; // Number of color planes uint16_t bits; // Bits per pixel uint32_t compression; // Compression type uint32_t imagesize; // Image size in bytes int32_t xresolution; // Pixels per meter int32_t yresolution; // Pixels per meter uint32_t ncolors; // Number of colors uint32_t importantcolors; // Important colors } BMPInfoHeader; #pragma pack(pop) int main() { // Define flag dimensions const int width = 300; // Width of the flag const int height = 200; // Height of the flag const int stripe_width = width / 3; // Width of each stripe // Colors in BGR format const uint8_t green[] = {0, 146, 70}; // Italian flag green const uint8_t white[] = {255, 255, 255}; // White const uint8_t red[] = {0, 0, 205}; // Italian flag red // Calculate padding (BMP rows must be aligned to 4 bytes) const int padding = (4 - (width * 3) % 4) % 4; const int row_size = width * 3 + padding; // Setup headers BMPHeader header = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + height * row_size, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits = 24, .compression = 0, .imagesize = height * row_size, .xresolution = 2835, // 72 DPI .yresolution = 2835, // 72 DPI .ncolors = 0, .importantcolors = 0 }; // Write headers to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Write pixel data uint8_t padding_zeros[3] = {0, 0, 0}; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { const uint8_t* color; if (x < stripe_width) { color = green; } else if (x < stripe_width * 2) { color = white; } else { color = red; } fwrite(color, 3, 1, stdout); } if (padding > 0) { fwrite(padding_zeros, padding, 1, stdout); } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; // Magic identifier "BM" uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data in bytes } BMPHeader; typedef struct { uint32_t size; // Header size in bytes int32_t width; // Width of image int32_t height; // Height of image uint16_t planes; // Number of color planes uint16_t bits; // Bits per pixel uint32_t compression; // Compression type uint32_t imagesize; // Image size in bytes int32_t xresolution; // Pixels per meter int32_t yresolution; // Pixels per meter uint32_t ncolors; // Number of colors uint32_t importantcolors; // Important colors } BMPInfoHeader; #pragma pack(pop) int main() { // Define flag dimensions const int width = 300; // Width of the flag const int height = 200; // Height of the flag const int stripe_width = width / 3; // Width of each stripe // Colors in BGR format const uint8_t green[] = {0, 146, 70}; // Italian flag green const uint8_t white[] = {255, 255, 255}; // White const uint8_t red[] = {0, 0, 205}; // Italian flag red // Calculate padding (BMP rows must be aligned to 4 bytes) const int padding = (4 - (width * 3) % 4) % 4; const int row_size = width * 3 + padding; // Setup headers BMPHeader header = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + height * row_size, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits = 24, .compression = 0, .imagesize = height * row_size, .xresolution = 2835, // 72 DPI .yresolution = 2835, // 72 DPI .ncolors = 0, .importantcolors = 0 }; // Write headers to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Write pixel data uint8_t padding_zeros[3] = {0, 0, 0}; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { const uint8_t* color; if (x < stripe_width) { color = green; } else if (x < stripe_width * 2) { color = white; } else { color = red; } fwrite(color, 3, 1, stdout); } if (padding > 0) { fwrite(padding_zeros, padding, 1, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 | #include <stdio.h> #include <stdint.h> #include <string.h> // BMP header structures #pragma pack(push, 1) typedef struct { uint16_t type; // Magic identifier "BM" uint32_t size; // File size in bytes uint16_t reserved1; // Not used uint16_t reserved2; // Not used uint32_t offset; // Offset to image data in bytes } BMPHeader; typedef struct { uint32_t size; // Header size in bytes int32_t width; // Width of image int32_t height; // Height of image uint16_t planes; // Number of color planes uint16_t bits; // Bits per pixel uint32_t compression; // Compression type uint32_t imagesize; // Image size in bytes int32_t xresolution; // Pixels per meter int32_t yresolution; // Pixels per meter uint32_t ncolors; // Number of colors uint32_t importantcolors; // Important colors } BMPInfoHeader; #pragma pack(pop) int main() { // Define flag dimensions const int width = 300; // Width of the flag const int height = 200; // Height of the flag const int stripe_width = width / 3; // Width of each stripe // Colors in BGR format const uint8_t green[] = {0, 146, 70}; // Italian flag green const uint8_t white[] = {255, 255, 255}; // White const uint8_t red[] = {0, 0, 205}; // Italian flag red // Calculate padding (BMP rows must be aligned to 4 bytes) const int padding = (4 - (width * 3) % 4) % 4; const int row_size = width * 3 + padding; // Setup headers BMPHeader header = { .type = 0x4D42, // "BM" .size = sizeof(BMPHeader) + sizeof(BMPInfoHeader) + height * row_size, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(BMPInfoHeader) }; BMPInfoHeader info = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bits = 24, .compression = 0, .imagesize = height * row_size, .xresolution = 2835, // 72 DPI .yresolution = 2835, // 72 DPI .ncolors = 0, .importantcolors = 0 }; // Write headers to stdout fwrite(&header, sizeof(header), 1, stdout); fwrite(&info, sizeof(info), 1, stdout); // Write pixel data uint8_t padding_zeros[3] = {0, 0, 0}; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { const uint8_t* color; if (x < stripe_width) { color = green; } else if (x < stripe_width * 2) { color = white; } else { color = red; } fwrite(color, 3, 1, stdout); } if (padding > 0) { fwrite(padding_zeros, padding, 1, stdout); } } return 0; } |
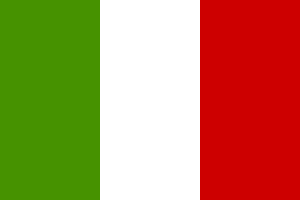
What flag is shown in this image?
The flag shown is the national flag of Italy.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True