Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Certainly! Here's a C program that generates a BMP file representing the Italian flag and outputs it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // DIB header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } __attribute__((packed)) DIBHeader; // RGB color typedef struct { uint8_t blue; uint8_t green; uint8_t red; } __attribute__((packed)) RGBColor; void writeHeaders(BMPHeader *bmpHeader, DIBHeader *dibHeader) { bmpHeader->type = 0x4D42; // "BM" bmpHeader->size = sizeof(BMPHeader) + sizeof(DIBHeader) + (WIDTH * HEIGHT * 3); bmpHeader->reserved1 = 0; bmpHeader->reserved2 = 0; bmpHeader->offset = sizeof(BMPHeader) + sizeof(DIBHeader); dibHeader->size = sizeof(DIBHeader); dibHeader->width = WIDTH; dibHeader->height = HEIGHT; dibHeader->planes = 1; dibHeader->bitsPerPixel = 24; dibHeader->compression = 0; dibHeader->imageSize = WIDTH * HEIGHT * 3; dibHeader->xPixelsPerMeter = 2835; dibHeader->yPixelsPerMeter = 2835; dibHeader->colorsUsed = 0; dibHeader->colorsImportant = 0; fwrite(bmpHeader, sizeof(BMPHeader), 1, stdout); fwrite(dibHeader, sizeof(DIBHeader), 1, stdout); } void writePixel(RGBColor color) { fwrite(&color, sizeof(RGBColor), 1, stdout); } int main() { BMPHeader bmpHeader; DIBHeader dibHeader; RGBColor green = {0, 146, 70}; // Italian flag green RGBColor white = {255, 255, 255}; RGBColor red = {0, 0, 140}; // Italian flag red writeHeaders(&bmpHeader, &dibHeader); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { writePixel(green); } else if (x < 2 * WIDTH / 3) { writePixel(white); } else { writePixel(red); } } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // DIB header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } __attribute__((packed)) DIBHeader; // RGB color typedef struct { uint8_t blue; uint8_t green; uint8_t red; } __attribute__((packed)) RGBColor; void writeHeaders(BMPHeader *bmpHeader, DIBHeader *dibHeader) { bmpHeader->type = 0x4D42; // "BM" bmpHeader->size = sizeof(BMPHeader) + sizeof(DIBHeader) + (WIDTH * HEIGHT * 3); bmpHeader->reserved1 = 0; bmpHeader->reserved2 = 0; bmpHeader->offset = sizeof(BMPHeader) + sizeof(DIBHeader); dibHeader->size = sizeof(DIBHeader); dibHeader->width = WIDTH; dibHeader->height = HEIGHT; dibHeader->planes = 1; dibHeader->bitsPerPixel = 24; dibHeader->compression = 0; dibHeader->imageSize = WIDTH * HEIGHT * 3; dibHeader->xPixelsPerMeter = 2835; dibHeader->yPixelsPerMeter = 2835; dibHeader->colorsUsed = 0; dibHeader->colorsImportant = 0; fwrite(bmpHeader, sizeof(BMPHeader), 1, stdout); fwrite(dibHeader, sizeof(DIBHeader), 1, stdout); } void writePixel(RGBColor color) { fwrite(&color, sizeof(RGBColor), 1, stdout); } int main() { BMPHeader bmpHeader; DIBHeader dibHeader; RGBColor green = {0, 146, 70}; // Italian flag green RGBColor white = {255, 255, 255}; RGBColor red = {0, 0, 140}; // Italian flag red writeHeaders(&bmpHeader, &dibHeader); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { writePixel(green); } else if (x < 2 * WIDTH / 3) { writePixel(white); } else { writePixel(red); } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // DIB header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } __attribute__((packed)) DIBHeader; // RGB color typedef struct { uint8_t blue; uint8_t green; uint8_t red; } __attribute__((packed)) RGBColor; void writeHeaders(BMPHeader *bmpHeader, DIBHeader *dibHeader) { bmpHeader->type = 0x4D42; // "BM" bmpHeader->size = sizeof(BMPHeader) + sizeof(DIBHeader) + (WIDTH * HEIGHT * 3); bmpHeader->reserved1 = 0; bmpHeader->reserved2 = 0; bmpHeader->offset = sizeof(BMPHeader) + sizeof(DIBHeader); dibHeader->size = sizeof(DIBHeader); dibHeader->width = WIDTH; dibHeader->height = HEIGHT; dibHeader->planes = 1; dibHeader->bitsPerPixel = 24; dibHeader->compression = 0; dibHeader->imageSize = WIDTH * HEIGHT * 3; dibHeader->xPixelsPerMeter = 2835; dibHeader->yPixelsPerMeter = 2835; dibHeader->colorsUsed = 0; dibHeader->colorsImportant = 0; fwrite(bmpHeader, sizeof(BMPHeader), 1, stdout); fwrite(dibHeader, sizeof(DIBHeader), 1, stdout); } void writePixel(RGBColor color) { fwrite(&color, sizeof(RGBColor), 1, stdout); } int main() { BMPHeader bmpHeader; DIBHeader dibHeader; RGBColor green = {0, 146, 70}; // Italian flag green RGBColor white = {255, 255, 255}; RGBColor red = {0, 0, 140}; // Italian flag red writeHeaders(&bmpHeader, &dibHeader); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { writePixel(green); } else if (x < 2 * WIDTH / 3) { writePixel(white); } else { writePixel(red); } } } return 0; } |
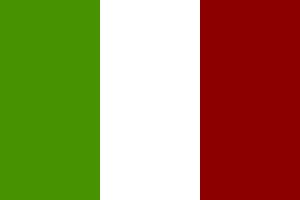
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three vertical stripes of equal width, with green on the hoist side, white in the middle, and red on the fly side.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP file representing the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // BMP info header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } __attribute__((packed)) BMPInfoHeader; // RGB color typedef struct { uint8_t blue; uint8_t green; uint8_t red; } __attribute__((packed)) RGBColor; void writeHeaders(FILE* fp, int width, int height) { BMPHeader header = { .type = 0x4D42, // "BM" .size = 54 + width * height * 3, .reserved1 = 0, .reserved2 = 0, .offset = 54 }; BMPInfoHeader infoHeader = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bitsPerPixel = 24, .compression = 0, .imageSize = width * height * 3, .xPixelsPerMeter = 2835, .yPixelsPerMeter = 2835, .colorsUsed = 0, .colorsImportant = 0 }; fwrite(&header, sizeof(header), 1, fp); fwrite(&infoHeader, sizeof(infoHeader), 1, fp); } int main() { FILE* fp = stdout; writeHeaders(fp, WIDTH, HEIGHT); RGBColor green = {0, 146, 70}; RGBColor white = {255, 255, 255}; RGBColor red = {206, 43, 55}; for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { RGBColor* color; if (x < WIDTH / 3) { color = &green; } else if (x < 2 * WIDTH / 3) { color = &white; } else { color = &red; } fwrite(color, sizeof(RGBColor), 1, fp); } } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // BMP info header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } __attribute__((packed)) BMPInfoHeader; // RGB color typedef struct { uint8_t blue; uint8_t green; uint8_t red; } __attribute__((packed)) RGBColor; void writeHeaders(FILE* fp, int width, int height) { BMPHeader header = { .type = 0x4D42, // "BM" .size = 54 + width * height * 3, .reserved1 = 0, .reserved2 = 0, .offset = 54 }; BMPInfoHeader infoHeader = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bitsPerPixel = 24, .compression = 0, .imageSize = width * height * 3, .xPixelsPerMeter = 2835, .yPixelsPerMeter = 2835, .colorsUsed = 0, .colorsImportant = 0 }; fwrite(&header, sizeof(header), 1, fp); fwrite(&infoHeader, sizeof(infoHeader), 1, fp); } int main() { FILE* fp = stdout; writeHeaders(fp, WIDTH, HEIGHT); RGBColor green = {0, 146, 70}; RGBColor white = {255, 255, 255}; RGBColor red = {206, 43, 55}; for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { RGBColor* color; if (x < WIDTH / 3) { color = &green; } else if (x < 2 * WIDTH / 3) { color = &white; } else { color = &red; } fwrite(color, sizeof(RGBColor), 1, fp); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // BMP info header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } __attribute__((packed)) BMPInfoHeader; // RGB color typedef struct { uint8_t blue; uint8_t green; uint8_t red; } __attribute__((packed)) RGBColor; void writeHeaders(FILE* fp, int width, int height) { BMPHeader header = { .type = 0x4D42, // "BM" .size = 54 + width * height * 3, .reserved1 = 0, .reserved2 = 0, .offset = 54 }; BMPInfoHeader infoHeader = { .size = sizeof(BMPInfoHeader), .width = width, .height = height, .planes = 1, .bitsPerPixel = 24, .compression = 0, .imageSize = width * height * 3, .xPixelsPerMeter = 2835, .yPixelsPerMeter = 2835, .colorsUsed = 0, .colorsImportant = 0 }; fwrite(&header, sizeof(header), 1, fp); fwrite(&infoHeader, sizeof(infoHeader), 1, fp); } int main() { FILE* fp = stdout; writeHeaders(fp, WIDTH, HEIGHT); RGBColor green = {0, 146, 70}; RGBColor white = {255, 255, 255}; RGBColor red = {206, 43, 55}; for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { RGBColor* color; if (x < WIDTH / 3) { color = &green; } else if (x < 2 * WIDTH / 3) { color = &white; } else { color = &red; } fwrite(color, sizeof(RGBColor), 1, fp); } } return 0; } |
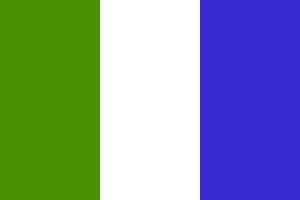
What flag is shown in this image?
The flag shown in the image is the flag of the Nigerian Army. It consists of three vertical stripes of green, white, and blue.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP image of the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width_px; int32_t height_px; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size_bytes; int32_t x_resolution_ppm; int32_t y_resolution_ppm; uint32_t num_colors; uint32_t important_colors; } BMPHeader; #pragma pack(pop) void write_header(BMPHeader *header) { header->type = 0x4D42; // BM header->size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3; header->reserved1 = 0; header->reserved2 = 0; header->offset = sizeof(BMPHeader); header->dib_header_size = 40; header->width_px = WIDTH; header->height_px = HEIGHT; header->num_planes = 1; header->bits_per_pixel = 24; header->compression = 0; header->image_size_bytes = WIDTH * HEIGHT * 3; header->x_resolution_ppm = 2835; header->y_resolution_ppm = 2835; header->num_colors = 0; header->important_colors = 0; fwrite(header, sizeof(BMPHeader), 1, stdout); } void write_pixel(uint8_t r, uint8_t g, uint8_t b) { fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } int main() { BMPHeader header; write_header(&header); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(255, 255, 255); // White } else { write_pixel(206, 43, 55); // Red } } } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width_px; int32_t height_px; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size_bytes; int32_t x_resolution_ppm; int32_t y_resolution_ppm; uint32_t num_colors; uint32_t important_colors; } BMPHeader; #pragma pack(pop) void write_header(BMPHeader *header) { header->type = 0x4D42; // BM header->size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3; header->reserved1 = 0; header->reserved2 = 0; header->offset = sizeof(BMPHeader); header->dib_header_size = 40; header->width_px = WIDTH; header->height_px = HEIGHT; header->num_planes = 1; header->bits_per_pixel = 24; header->compression = 0; header->image_size_bytes = WIDTH * HEIGHT * 3; header->x_resolution_ppm = 2835; header->y_resolution_ppm = 2835; header->num_colors = 0; header->important_colors = 0; fwrite(header, sizeof(BMPHeader), 1, stdout); } void write_pixel(uint8_t r, uint8_t g, uint8_t b) { fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } int main() { BMPHeader header; write_header(&header); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(255, 255, 255); // White } else { write_pixel(206, 43, 55); // Red } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP header structure #pragma pack(push, 1) typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; uint32_t dib_header_size; int32_t width_px; int32_t height_px; uint16_t num_planes; uint16_t bits_per_pixel; uint32_t compression; uint32_t image_size_bytes; int32_t x_resolution_ppm; int32_t y_resolution_ppm; uint32_t num_colors; uint32_t important_colors; } BMPHeader; #pragma pack(pop) void write_header(BMPHeader *header) { header->type = 0x4D42; // BM header->size = sizeof(BMPHeader) + WIDTH * HEIGHT * 3; header->reserved1 = 0; header->reserved2 = 0; header->offset = sizeof(BMPHeader); header->dib_header_size = 40; header->width_px = WIDTH; header->height_px = HEIGHT; header->num_planes = 1; header->bits_per_pixel = 24; header->compression = 0; header->image_size_bytes = WIDTH * HEIGHT * 3; header->x_resolution_ppm = 2835; header->y_resolution_ppm = 2835; header->num_colors = 0; header->important_colors = 0; fwrite(header, sizeof(BMPHeader), 1, stdout); } void write_pixel(uint8_t r, uint8_t g, uint8_t b) { fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } int main() { BMPHeader header; write_header(&header); for (int y = 0; y < HEIGHT; y++) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(255, 255, 255); // White } else { write_pixel(206, 43, 55); // Red } } } return 0; } |
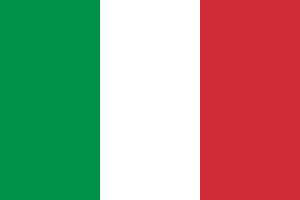
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three vertical stripes of equal width, with green on the hoist side, white in the middle, and red on the fly side.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP file representing the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // DIB header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } __attribute__((packed)) DIBHeader; void writeHeader(BMPHeader *header, DIBHeader *dibHeader) { fwrite(header, sizeof(BMPHeader), 1, stdout); fwrite(dibHeader, sizeof(DIBHeader), 1, stdout); } void writePixel(uint8_t r, uint8_t g, uint8_t b) { fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } int main() { BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + sizeof(DIBHeader) + 3 * WIDTH * HEIGHT, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(DIBHeader) }; DIBHeader dibHeader = { .size = sizeof(DIBHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bitsPerPixel = 24, .compression = 0, .imageSize = 3 * WIDTH * HEIGHT, .xPixelsPerMeter = 2835, .yPixelsPerMeter = 2835, .colorsUsed = 0, .colorsImportant = 0 }; writeHeader(&header, &dibHeader); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { writePixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { writePixel(255, 255, 255); // White } else { writePixel(206, 43, 55); // Red } } // Pad row to multiple of 4 bytes for (int i = 0; i < (WIDTH * 3) % 4; i++) { fputc(0, stdout); } } return 0; } |
1 2 | gcc italian_flag.c -o italian_flag ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // DIB header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } __attribute__((packed)) DIBHeader; void writeHeader(BMPHeader *header, DIBHeader *dibHeader) { fwrite(header, sizeof(BMPHeader), 1, stdout); fwrite(dibHeader, sizeof(DIBHeader), 1, stdout); } void writePixel(uint8_t r, uint8_t g, uint8_t b) { fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } int main() { BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + sizeof(DIBHeader) + 3 * WIDTH * HEIGHT, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(DIBHeader) }; DIBHeader dibHeader = { .size = sizeof(DIBHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bitsPerPixel = 24, .compression = 0, .imageSize = 3 * WIDTH * HEIGHT, .xPixelsPerMeter = 2835, .yPixelsPerMeter = 2835, .colorsUsed = 0, .colorsImportant = 0 }; writeHeader(&header, &dibHeader); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { writePixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { writePixel(255, 255, 255); // White } else { writePixel(206, 43, 55); // Red } } // Pad row to multiple of 4 bytes for (int i = 0; i < (WIDTH * 3) % 4; i++) { fputc(0, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // DIB header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bitsPerPixel; uint32_t compression; uint32_t imageSize; int32_t xPixelsPerMeter; int32_t yPixelsPerMeter; uint32_t colorsUsed; uint32_t colorsImportant; } __attribute__((packed)) DIBHeader; void writeHeader(BMPHeader *header, DIBHeader *dibHeader) { fwrite(header, sizeof(BMPHeader), 1, stdout); fwrite(dibHeader, sizeof(DIBHeader), 1, stdout); } void writePixel(uint8_t r, uint8_t g, uint8_t b) { fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } int main() { BMPHeader header = { .type = 0x4D42, .size = sizeof(BMPHeader) + sizeof(DIBHeader) + 3 * WIDTH * HEIGHT, .reserved1 = 0, .reserved2 = 0, .offset = sizeof(BMPHeader) + sizeof(DIBHeader) }; DIBHeader dibHeader = { .size = sizeof(DIBHeader), .width = WIDTH, .height = HEIGHT, .planes = 1, .bitsPerPixel = 24, .compression = 0, .imageSize = 3 * WIDTH * HEIGHT, .xPixelsPerMeter = 2835, .yPixelsPerMeter = 2835, .colorsUsed = 0, .colorsImportant = 0 }; writeHeader(&header, &dibHeader); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { writePixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { writePixel(255, 255, 255); // White } else { writePixel(206, 43, 55); // Red } } // Pad row to multiple of 4 bytes for (int i = 0; i < (WIDTH * 3) % 4; i++) { fputc(0, stdout); } } return 0; } |
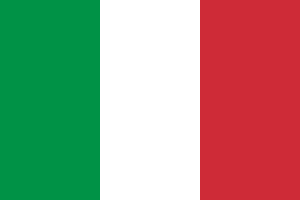
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three vertical stripes of equal width, with green on the hoist side, white in the middle, and red on the fly side.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Certainly! Here's a C program that generates a BMP file representing the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // DIB header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bpp; uint32_t compression; uint32_t image_size; int32_t x_ppm; int32_t y_ppm; uint32_t colors_used; uint32_t important_colors; } __attribute__((packed)) DIBHeader; void write_headers(BMPHeader *bmp_header, DIBHeader *dib_header) { bmp_header->type = 0x4D42; // "BM" bmp_header->size = sizeof(BMPHeader) + sizeof(DIBHeader) + WIDTH * HEIGHT * 3; bmp_header->reserved1 = 0; bmp_header->reserved2 = 0; bmp_header->offset = sizeof(BMPHeader) + sizeof(DIBHeader); dib_header->size = sizeof(DIBHeader); dib_header->width = WIDTH; dib_header->height = HEIGHT; dib_header->planes = 1; dib_header->bpp = 24; dib_header->compression = 0; dib_header->image_size = WIDTH * HEIGHT * 3; dib_header->x_ppm = 2835; dib_header->y_ppm = 2835; dib_header->colors_used = 0; dib_header->important_colors = 0; fwrite(bmp_header, sizeof(BMPHeader), 1, stdout); fwrite(dib_header, sizeof(DIBHeader), 1, stdout); } void write_pixel(uint8_t r, uint8_t g, uint8_t b) { fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } int main() { BMPHeader bmp_header; DIBHeader dib_header; write_headers(&bmp_header, &dib_header); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(255, 255, 255); // White } else { write_pixel(206, 43, 55); // Red } } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // DIB header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bpp; uint32_t compression; uint32_t image_size; int32_t x_ppm; int32_t y_ppm; uint32_t colors_used; uint32_t important_colors; } __attribute__((packed)) DIBHeader; void write_headers(BMPHeader *bmp_header, DIBHeader *dib_header) { bmp_header->type = 0x4D42; // "BM" bmp_header->size = sizeof(BMPHeader) + sizeof(DIBHeader) + WIDTH * HEIGHT * 3; bmp_header->reserved1 = 0; bmp_header->reserved2 = 0; bmp_header->offset = sizeof(BMPHeader) + sizeof(DIBHeader); dib_header->size = sizeof(DIBHeader); dib_header->width = WIDTH; dib_header->height = HEIGHT; dib_header->planes = 1; dib_header->bpp = 24; dib_header->compression = 0; dib_header->image_size = WIDTH * HEIGHT * 3; dib_header->x_ppm = 2835; dib_header->y_ppm = 2835; dib_header->colors_used = 0; dib_header->important_colors = 0; fwrite(bmp_header, sizeof(BMPHeader), 1, stdout); fwrite(dib_header, sizeof(DIBHeader), 1, stdout); } void write_pixel(uint8_t r, uint8_t g, uint8_t b) { fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } int main() { BMPHeader bmp_header; DIBHeader dib_header; write_headers(&bmp_header, &dib_header); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(255, 255, 255); // White } else { write_pixel(206, 43, 55); // Red } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 | #include <stdio.h> #include <stdint.h> #include <string.h> #define WIDTH 300 #define HEIGHT 200 // BMP file header typedef struct { uint16_t type; uint32_t size; uint16_t reserved1; uint16_t reserved2; uint32_t offset; } __attribute__((packed)) BMPHeader; // DIB header typedef struct { uint32_t size; int32_t width; int32_t height; uint16_t planes; uint16_t bpp; uint32_t compression; uint32_t image_size; int32_t x_ppm; int32_t y_ppm; uint32_t colors_used; uint32_t important_colors; } __attribute__((packed)) DIBHeader; void write_headers(BMPHeader *bmp_header, DIBHeader *dib_header) { bmp_header->type = 0x4D42; // "BM" bmp_header->size = sizeof(BMPHeader) + sizeof(DIBHeader) + WIDTH * HEIGHT * 3; bmp_header->reserved1 = 0; bmp_header->reserved2 = 0; bmp_header->offset = sizeof(BMPHeader) + sizeof(DIBHeader); dib_header->size = sizeof(DIBHeader); dib_header->width = WIDTH; dib_header->height = HEIGHT; dib_header->planes = 1; dib_header->bpp = 24; dib_header->compression = 0; dib_header->image_size = WIDTH * HEIGHT * 3; dib_header->x_ppm = 2835; dib_header->y_ppm = 2835; dib_header->colors_used = 0; dib_header->important_colors = 0; fwrite(bmp_header, sizeof(BMPHeader), 1, stdout); fwrite(dib_header, sizeof(DIBHeader), 1, stdout); } void write_pixel(uint8_t r, uint8_t g, uint8_t b) { fputc(b, stdout); fputc(g, stdout); fputc(r, stdout); } int main() { BMPHeader bmp_header; DIBHeader dib_header; write_headers(&bmp_header, &dib_header); for (int y = HEIGHT - 1; y >= 0; y--) { for (int x = 0; x < WIDTH; x++) { if (x < WIDTH / 3) { write_pixel(0, 146, 70); // Green } else if (x < 2 * WIDTH / 3) { write_pixel(255, 255, 255); // White } else { write_pixel(206, 43, 55); // Red } } } return 0; } |
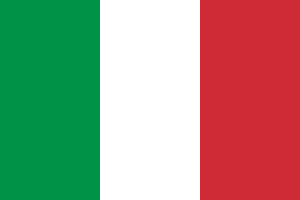
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three vertical stripes of equal width, with green on the hoist side, white in the middle, and red on the fly side.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True