Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates an Italian flag as a 24-bit bitmap image and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | #include <stdio.h> #include <stdint.h> // Function to write a 2-byte value (little-endian) void write_u16(uint16_t value) { putchar(value & 0xFF); putchar((value >> 8) & 0xFF); } // Function to write a 4-byte value (little-endian) void write_u32(uint32_t value) { putchar(value & 0xFF); putchar((value >> 8) & 0xFF); putchar((value >> 16) & 0xFF); putchar((value >> 24) & 0xFF); } int main() { // Flag dimensions const int width = 300; const int height = 200; // Colors (in BGR format) const uint32_t green = 0x00008000; // Dark green const uint32_t white = 0x00FFFFFF; // White const uint32_t red = 0x000000FF; // Red // BMP Header putchar('B'); putchar('M'); // File size (calculated) write_u32(54 + width * height * 3); // File header + pixel data write_u16(0); // Reserved write_u16(0); // Reserved write_u32(54); // Pixel data offset // DIB Header write_u32(40); // DIB header size write_u32(width); // Width write_u32(height); // Height write_u16(1); // Color planes write_u16(24); // Bits per pixel write_u32(0); // Compression method write_u32(width * height * 3); // Image size write_u32(0); // Horizontal resolution write_u32(0); // Vertical resolution write_u32(0); // Color palette write_u32(0); // Important colors // Pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint32_t color; // Divide flag into three equal vertical stripes if (x < width / 3) { color = green; } else if (x < 2 * width / 3) { color = white; } else { color = red; } // Write BGR pixel putchar(color & 0xFF); putchar((color >> 8) & 0xFF); putchar((color >> 16) & 0xFF); } } return 0; } |
1 2 | gcc -o italian_flag italian_flag.c |
1 2 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | #include <stdio.h> #include <stdint.h> // Function to write a 2-byte value (little-endian) void write_u16(uint16_t value) { putchar(value & 0xFF); putchar((value >> 8) & 0xFF); } // Function to write a 4-byte value (little-endian) void write_u32(uint32_t value) { putchar(value & 0xFF); putchar((value >> 8) & 0xFF); putchar((value >> 16) & 0xFF); putchar((value >> 24) & 0xFF); } int main() { // Flag dimensions const int width = 300; const int height = 200; // Colors (in BGR format) const uint32_t green = 0x00008000; // Dark green const uint32_t white = 0x00FFFFFF; // White const uint32_t red = 0x000000FF; // Red // BMP Header putchar('B'); putchar('M'); // File size (calculated) write_u32(54 + width * height * 3); // File header + pixel data write_u16(0); // Reserved write_u16(0); // Reserved write_u32(54); // Pixel data offset // DIB Header write_u32(40); // DIB header size write_u32(width); // Width write_u32(height); // Height write_u16(1); // Color planes write_u16(24); // Bits per pixel write_u32(0); // Compression method write_u32(width * height * 3); // Image size write_u32(0); // Horizontal resolution write_u32(0); // Vertical resolution write_u32(0); // Color palette write_u32(0); // Important colors // Pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint32_t color; // Divide flag into three equal vertical stripes if (x < width / 3) { color = green; } else if (x < 2 * width / 3) { color = white; } else { color = red; } // Write BGR pixel putchar(color & 0xFF); putchar((color >> 8) & 0xFF); putchar((color >> 16) & 0xFF); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | #include <stdio.h> #include <stdint.h> // Function to write a 2-byte value (little-endian) void write_u16(uint16_t value) { putchar(value & 0xFF); putchar((value >> 8) & 0xFF); } // Function to write a 4-byte value (little-endian) void write_u32(uint32_t value) { putchar(value & 0xFF); putchar((value >> 8) & 0xFF); putchar((value >> 16) & 0xFF); putchar((value >> 24) & 0xFF); } int main() { // Flag dimensions const int width = 300; const int height = 200; // Colors (in BGR format) const uint32_t green = 0x00008000; // Dark green const uint32_t white = 0x00FFFFFF; // White const uint32_t red = 0x000000FF; // Red // BMP Header putchar('B'); putchar('M'); // File size (calculated) write_u32(54 + width * height * 3); // File header + pixel data write_u16(0); // Reserved write_u16(0); // Reserved write_u32(54); // Pixel data offset // DIB Header write_u32(40); // DIB header size write_u32(width); // Width write_u32(height); // Height write_u16(1); // Color planes write_u16(24); // Bits per pixel write_u32(0); // Compression method write_u32(width * height * 3); // Image size write_u32(0); // Horizontal resolution write_u32(0); // Vertical resolution write_u32(0); // Color palette write_u32(0); // Important colors // Pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint32_t color; // Divide flag into three equal vertical stripes if (x < width / 3) { color = green; } else if (x < 2 * width / 3) { color = white; } else { color = red; } // Write BGR pixel putchar(color & 0xFF); putchar((color >> 8) & 0xFF); putchar((color >> 16) & 0xFF); } } return 0; } |
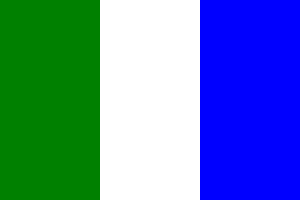
What flag is shown in this image?
The flag shown in the image is the flag of the Ivory Coast (Côte d'Ivoire). It consists of three vertical stripes of green, white, and blue.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates an Italian flag as a .bmp file and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 | #include <stdio.h> #include <stdint.h> // Function to write a 24-bit BMP file of an Italian flag void write_italian_flag(int width, int height) { // BMP file header uint16_t bfType = 0x4D42; // "BM" uint32_t bfSize = 54 + width * height * 3; // File size uint16_t bfReserved1 = 0; uint16_t bfReserved2 = 0; uint32_t bfOffBits = 54; // Offset to pixel data // BMP info header uint32_t biSize = 40; uint32_t biWidth = width; uint32_t biHeight = height; uint16_t biPlanes = 1; uint16_t biBitCount = 24; uint32_t biCompression = 0; uint32_t biSizeImage = width * height * 3; uint32_t biXPelsPerMeter = 0; uint32_t biYPelsPerMeter = 0; uint32_t biClrUsed = 0; uint32_t biClrImportant = 0; // Write file header fwrite(&bfType, 2, 1, stdout); fwrite(&bfSize, 4, 1, stdout); fwrite(&bfReserved1, 2, 1, stdout); fwrite(&bfReserved2, 2, 1, stdout); fwrite(&bfOffBits, 4, 1, stdout); // Write info header fwrite(&biSize, 4, 1, stdout); fwrite(&biWidth, 4, 1, stdout); fwrite(&biHeight, 4, 1, stdout); fwrite(&biPlanes, 2, 1, stdout); fwrite(&biBitCount, 2, 1, stdout); fwrite(&biCompression, 4, 1, stdout); fwrite(&biSizeImage, 4, 1, stdout); fwrite(&biXPelsPerMeter, 4, 1, stdout); fwrite(&biYPelsPerMeter, 4, 1, stdout); fwrite(&biClrUsed, 4, 1, stdout); fwrite(&biClrImportant, 4, 1, stdout); // Write pixel data (Italian flag colors) for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t r, g, b; // Divide flag into three equal vertical sections if (x < width / 3) { // Green section r = 0; g = 140; b = 0; } else if (x < 2 * width / 3) { // White section r = g = b = 255; } else { // Red section r = 206; g = 0; b = 0; } // Write BGR pixel data (BMP stores colors in BGR order) fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } } } int main() { // Create a 300x200 pixel Italian flag write_italian_flag(300, 200); return 0; } |
1 2 | gcc italian_flag.c -o italian_flag |
1 2 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 | #include <stdio.h> #include <stdint.h> // Function to write a 24-bit BMP file of an Italian flag void write_italian_flag(int width, int height) { // BMP file header uint16_t bfType = 0x4D42; // "BM" uint32_t bfSize = 54 + width * height * 3; // File size uint16_t bfReserved1 = 0; uint16_t bfReserved2 = 0; uint32_t bfOffBits = 54; // Offset to pixel data // BMP info header uint32_t biSize = 40; uint32_t biWidth = width; uint32_t biHeight = height; uint16_t biPlanes = 1; uint16_t biBitCount = 24; uint32_t biCompression = 0; uint32_t biSizeImage = width * height * 3; uint32_t biXPelsPerMeter = 0; uint32_t biYPelsPerMeter = 0; uint32_t biClrUsed = 0; uint32_t biClrImportant = 0; // Write file header fwrite(&bfType, 2, 1, stdout); fwrite(&bfSize, 4, 1, stdout); fwrite(&bfReserved1, 2, 1, stdout); fwrite(&bfReserved2, 2, 1, stdout); fwrite(&bfOffBits, 4, 1, stdout); // Write info header fwrite(&biSize, 4, 1, stdout); fwrite(&biWidth, 4, 1, stdout); fwrite(&biHeight, 4, 1, stdout); fwrite(&biPlanes, 2, 1, stdout); fwrite(&biBitCount, 2, 1, stdout); fwrite(&biCompression, 4, 1, stdout); fwrite(&biSizeImage, 4, 1, stdout); fwrite(&biXPelsPerMeter, 4, 1, stdout); fwrite(&biYPelsPerMeter, 4, 1, stdout); fwrite(&biClrUsed, 4, 1, stdout); fwrite(&biClrImportant, 4, 1, stdout); // Write pixel data (Italian flag colors) for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t r, g, b; // Divide flag into three equal vertical sections if (x < width / 3) { // Green section r = 0; g = 140; b = 0; } else if (x < 2 * width / 3) { // White section r = g = b = 255; } else { // Red section r = 206; g = 0; b = 0; } // Write BGR pixel data (BMP stores colors in BGR order) fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } } } int main() { // Create a 300x200 pixel Italian flag write_italian_flag(300, 200); return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 | #include <stdio.h> #include <stdint.h> // Function to write a 24-bit BMP file of an Italian flag void write_italian_flag(int width, int height) { // BMP file header uint16_t bfType = 0x4D42; // "BM" uint32_t bfSize = 54 + width * height * 3; // File size uint16_t bfReserved1 = 0; uint16_t bfReserved2 = 0; uint32_t bfOffBits = 54; // Offset to pixel data // BMP info header uint32_t biSize = 40; uint32_t biWidth = width; uint32_t biHeight = height; uint16_t biPlanes = 1; uint16_t biBitCount = 24; uint32_t biCompression = 0; uint32_t biSizeImage = width * height * 3; uint32_t biXPelsPerMeter = 0; uint32_t biYPelsPerMeter = 0; uint32_t biClrUsed = 0; uint32_t biClrImportant = 0; // Write file header fwrite(&bfType, 2, 1, stdout); fwrite(&bfSize, 4, 1, stdout); fwrite(&bfReserved1, 2, 1, stdout); fwrite(&bfReserved2, 2, 1, stdout); fwrite(&bfOffBits, 4, 1, stdout); // Write info header fwrite(&biSize, 4, 1, stdout); fwrite(&biWidth, 4, 1, stdout); fwrite(&biHeight, 4, 1, stdout); fwrite(&biPlanes, 2, 1, stdout); fwrite(&biBitCount, 2, 1, stdout); fwrite(&biCompression, 4, 1, stdout); fwrite(&biSizeImage, 4, 1, stdout); fwrite(&biXPelsPerMeter, 4, 1, stdout); fwrite(&biYPelsPerMeter, 4, 1, stdout); fwrite(&biClrUsed, 4, 1, stdout); fwrite(&biClrImportant, 4, 1, stdout); // Write pixel data (Italian flag colors) for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t r, g, b; // Divide flag into three equal vertical sections if (x < width / 3) { // Green section r = 0; g = 140; b = 0; } else if (x < 2 * width / 3) { // White section r = g = b = 255; } else { // Red section r = 206; g = 0; b = 0; } // Write BGR pixel data (BMP stores colors in BGR order) fwrite(&b, 1, 1, stdout); fwrite(&g, 1, 1, stdout); fwrite(&r, 1, 1, stdout); } } } int main() { // Create a 300x200 pixel Italian flag write_italian_flag(300, 200); return 0; } |
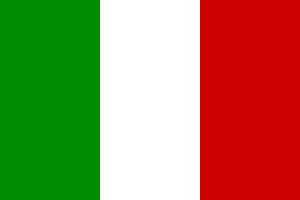
What flag is shown in this image?
The flag shown is the national flag of Italy.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a BMP image of the Italian flag and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | #include <stdio.h> #include <stdint.h> // Function to write a multi-byte integer in little-endian format void write_le(uint8_t* buf, uint32_t val, int bytes) { for (int i = 0; i < bytes; i++) { buf[i] = val & 0xFF; val >>= 8; } } int main() { // Flag dimensions const int width = 300; const int height = 200; // Colors (in BGR format for BMP) const uint8_t green[3] = {0, 128, 0}; // Left stripe const uint8_t white[3] = {255, 255, 255}; // Middle stripe const uint8_t red[3] = {0, 0, 255}; // Right stripe // BMP header uint8_t header[54] = {0}; header[0] = 'B'; header[1] = 'M'; // File size calculation int row_size = width * 3; int padding = (4 - (row_size % 4)) % 4; int file_size = 54 + (row_size + padding) * height; write_le(header + 2, file_size, 4); // File size header[10] = 54; // Pixel data offset header[14] = 40; // DIB header size write_le(header + 18, width, 4); // Width write_le(header + 22, height, 4); // Height header[26] = 1; // Planes header[28] = 24; // Bits per pixel // Write header to stdout fwrite(header, 1, 54, stdout); // Generate flag pixels for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t pixel[3]; if (x < width / 3) { // Green stripe (left) pixel[0] = green[0]; pixel[1] = green[1]; pixel[2] = green[2]; } else if (x < 2 * width / 3) { // White stripe (middle) pixel[0] = white[0]; pixel[1] = white[1]; pixel[2] = white[2]; } else { // Red stripe (right) pixel[0] = red[0]; pixel[1] = red[1]; pixel[2] = red[2]; } fwrite(pixel, 1, 3, stdout); } // Add padding bytes if needed uint8_t padding_byte[3] = {0}; for (int p = 0; p < padding; p++) { fwrite(padding_byte, 1, 1, stdout); } } return 0; } |
1 2 3 4 5 6 7 8 9 10 | # Compile the program gcc -o italian_flag italian_flag.c # Generate the BMP file ./italian_flag > italian_flag.bmp # Or directly pipe to an image viewer or file ./italian_flag | display # ImageMagick # or ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | #include <stdio.h> #include <stdint.h> // Function to write a multi-byte integer in little-endian format void write_le(uint8_t* buf, uint32_t val, int bytes) { for (int i = 0; i < bytes; i++) { buf[i] = val & 0xFF; val >>= 8; } } int main() { // Flag dimensions const int width = 300; const int height = 200; // Colors (in BGR format for BMP) const uint8_t green[3] = {0, 128, 0}; // Left stripe const uint8_t white[3] = {255, 255, 255}; // Middle stripe const uint8_t red[3] = {0, 0, 255}; // Right stripe // BMP header uint8_t header[54] = {0}; header[0] = 'B'; header[1] = 'M'; // File size calculation int row_size = width * 3; int padding = (4 - (row_size % 4)) % 4; int file_size = 54 + (row_size + padding) * height; write_le(header + 2, file_size, 4); // File size header[10] = 54; // Pixel data offset header[14] = 40; // DIB header size write_le(header + 18, width, 4); // Width write_le(header + 22, height, 4); // Height header[26] = 1; // Planes header[28] = 24; // Bits per pixel // Write header to stdout fwrite(header, 1, 54, stdout); // Generate flag pixels for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t pixel[3]; if (x < width / 3) { // Green stripe (left) pixel[0] = green[0]; pixel[1] = green[1]; pixel[2] = green[2]; } else if (x < 2 * width / 3) { // White stripe (middle) pixel[0] = white[0]; pixel[1] = white[1]; pixel[2] = white[2]; } else { // Red stripe (right) pixel[0] = red[0]; pixel[1] = red[1]; pixel[2] = red[2]; } fwrite(pixel, 1, 3, stdout); } // Add padding bytes if needed uint8_t padding_byte[3] = {0}; for (int p = 0; p < padding; p++) { fwrite(padding_byte, 1, 1, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | #include <stdio.h> #include <stdint.h> // Function to write a multi-byte integer in little-endian format void write_le(uint8_t* buf, uint32_t val, int bytes) { for (int i = 0; i < bytes; i++) { buf[i] = val & 0xFF; val >>= 8; } } int main() { // Flag dimensions const int width = 300; const int height = 200; // Colors (in BGR format for BMP) const uint8_t green[3] = {0, 128, 0}; // Left stripe const uint8_t white[3] = {255, 255, 255}; // Middle stripe const uint8_t red[3] = {0, 0, 255}; // Right stripe // BMP header uint8_t header[54] = {0}; header[0] = 'B'; header[1] = 'M'; // File size calculation int row_size = width * 3; int padding = (4 - (row_size % 4)) % 4; int file_size = 54 + (row_size + padding) * height; write_le(header + 2, file_size, 4); // File size header[10] = 54; // Pixel data offset header[14] = 40; // DIB header size write_le(header + 18, width, 4); // Width write_le(header + 22, height, 4); // Height header[26] = 1; // Planes header[28] = 24; // Bits per pixel // Write header to stdout fwrite(header, 1, 54, stdout); // Generate flag pixels for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t pixel[3]; if (x < width / 3) { // Green stripe (left) pixel[0] = green[0]; pixel[1] = green[1]; pixel[2] = green[2]; } else if (x < 2 * width / 3) { // White stripe (middle) pixel[0] = white[0]; pixel[1] = white[1]; pixel[2] = white[2]; } else { // Red stripe (right) pixel[0] = red[0]; pixel[1] = red[1]; pixel[2] = red[2]; } fwrite(pixel, 1, 3, stdout); } // Add padding bytes if needed uint8_t padding_byte[3] = {0}; for (int p = 0; p < padding; p++) { fwrite(padding_byte, 1, 1, stdout); } } return 0; } |
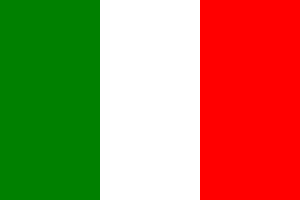
What flag is shown in this image?
The flag shown in the image is the national flag of Italy. It consists of three vertical stripes of green, white, and red.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a simple Italian flag (green, white, and red vertical stripes) as a bitmap image and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 | #include <stdio.h> #include <stdint.h> // Color definitions (BGR format for BMP) #define GREEN 0x00, 0x80, 0x00 #define WHITE 0xFF, 0xFF, 0xFF #define RED 0x00, 0x00, 0xFF // Function to write a byte void write_byte(uint8_t byte) { fputc(byte, stdout); } // Function to write a multi-byte integer (little-endian) void write_int(uint32_t value) { write_byte(value & 0xFF); write_byte((value >> 8) & 0xFF); write_byte((value >> 16) & 0xFF); write_byte((value >> 24) & 0xFF); } // Function to write a short integer (little-endian) void write_short(uint16_t value) { write_byte(value & 0xFF); write_byte((value >> 8) & 0xFF); } int main() { // Flag dimensions const int width = 300; const int height = 200; // BMP Header write_byte('B'); write_byte('M'); // File size (calculated) write_int(14 + 40 + (width * height * 3)); // Reserved write_short(0); write_short(0); // Pixel array offset write_int(14 + 40); // DIB Header write_int(40); // Header size write_int(width); // Width write_int(height); // Height write_short(1); // Planes write_short(24); // Bits per pixel write_int(0); // Compression write_int(width * height * 3); // Image size write_int(0); // Horizontal resolution write_int(0); // Vertical resolution write_int(0); // Color palette write_int(0); // Important colors // Pixel data (BGR format) for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green stripe write_byte(GREEN); } else if (x < 2 * width / 3) { // White stripe write_byte(WHITE); } else { // Red stripe write_byte(RED); } } } return 0; } |
1 | gcc -o italian_flag italian_flag.c |
1 | ./italian_flag > italian_flag.bmp |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 | #include <stdio.h> #include <stdint.h> // Color definitions (BGR format for BMP) #define GREEN 0x00, 0x80, 0x00 #define WHITE 0xFF, 0xFF, 0xFF #define RED 0x00, 0x00, 0xFF // Function to write a byte void write_byte(uint8_t byte) { fputc(byte, stdout); } // Function to write a multi-byte integer (little-endian) void write_int(uint32_t value) { write_byte(value & 0xFF); write_byte((value >> 8) & 0xFF); write_byte((value >> 16) & 0xFF); write_byte((value >> 24) & 0xFF); } // Function to write a short integer (little-endian) void write_short(uint16_t value) { write_byte(value & 0xFF); write_byte((value >> 8) & 0xFF); } int main() { // Flag dimensions const int width = 300; const int height = 200; // BMP Header write_byte('B'); write_byte('M'); // File size (calculated) write_int(14 + 40 + (width * height * 3)); // Reserved write_short(0); write_short(0); // Pixel array offset write_int(14 + 40); // DIB Header write_int(40); // Header size write_int(width); // Width write_int(height); // Height write_short(1); // Planes write_short(24); // Bits per pixel write_int(0); // Compression write_int(width * height * 3); // Image size write_int(0); // Horizontal resolution write_int(0); // Vertical resolution write_int(0); // Color palette write_int(0); // Important colors // Pixel data (BGR format) for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green stripe write_byte(GREEN); } else if (x < 2 * width / 3) { // White stripe write_byte(WHITE); } else { // Red stripe write_byte(RED); } } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 | #include <stdio.h> #include <stdint.h> // Color definitions (BGR format for BMP) #define GREEN 0x00, 0x80, 0x00 #define WHITE 0xFF, 0xFF, 0xFF #define RED 0x00, 0x00, 0xFF // Function to write a byte void write_byte(uint8_t byte) { fputc(byte, stdout); } // Function to write a multi-byte integer (little-endian) void write_int(uint32_t value) { write_byte(value & 0xFF); write_byte((value >> 8) & 0xFF); write_byte((value >> 16) & 0xFF); write_byte((value >> 24) & 0xFF); } // Function to write a short integer (little-endian) void write_short(uint16_t value) { write_byte(value & 0xFF); write_byte((value >> 8) & 0xFF); } int main() { // Flag dimensions const int width = 300; const int height = 200; // BMP Header write_byte('B'); write_byte('M'); // File size (calculated) write_int(14 + 40 + (width * height * 3)); // Reserved write_short(0); write_short(0); // Pixel array offset write_int(14 + 40); // DIB Header write_int(40); // Header size write_int(width); // Width write_int(height); // Height write_short(1); // Planes write_short(24); // Bits per pixel write_int(0); // Compression write_int(width * height * 3); // Image size write_int(0); // Horizontal resolution write_int(0); // Vertical resolution write_int(0); // Color palette write_int(0); // Important colors // Pixel data (BGR format) for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { if (x < width / 3) { // Green stripe write_byte(GREEN); } else if (x < 2 * width / 3) { // White stripe write_byte(WHITE); } else { // Red stripe write_byte(RED); } } } return 0; } |
b"main.c: In function 'main':\nmain.c:66:17: error: too many arguments to function 'write_byte'\n 66 | write_byte(GREEN);\n | ^~~~~~~~~~\nmain.c:11:6: note: declared here\n 11 | void write_byte(uint8_t byte) {\n | ^~~~~~~~~~\nmain.c:69:17: error: too many arguments to function 'write_byte'\n 69 | write_byte(WHITE);\n | ^~~~~~~~~~\nmain.c:11:6: note: declared here\n 11 | void write_byte(uint8_t byte) {\n | ^~~~~~~~~~\nmain.c:72:17: error: too many arguments to function 'write_byte'\n 72 | write_byte(RED);\n | ^~~~~~~~~~\nmain.c:11:6: note: declared here\n 11 | void write_byte(uint8_t byte) {\n | ^~~~~~~~~~\nmain.sh: line 2: ./a.out: No such file or directory\n"
What flag is shown in this image?
cannot identify image file <_io.BytesIO object at 0x7f6bd8b42f40>
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: False
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Write me a C program that emits a .bmp that's a depiction of an italian flag to stdout.
Here's a C program that generates a 300x200 pixel Italian flag as a .bmp file and writes it to stdout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #include <stdio.h> #include <stdint.h> // Function to write little-endian values void write_le(uint8_t* buf, uint32_t value, int size) { for (int i = 0; i < size; i++) { buf[i] = value & 0xFF; value >>= 8; } } int main() { // Flag dimensions const int width = 300; const int height = 200; // BMP header uint8_t header[54] = {0}; header[0] = 'B'; header[1] = 'M'; // File size uint32_t file_size = 54 + width * height * 3; write_le(header + 2, file_size, 4); // Reserved write_le(header + 6, 0, 4); // Pixel data offset write_le(header + 10, 54, 4); // DIB header size write_le(header + 14, 40, 4); // Image width and height write_le(header + 18, width, 4); write_le(header + 22, height, 4); // Planes write_le(header + 26, 1, 2); // Bits per pixel write_le(header + 28, 24, 2); // Write header fwrite(header, 1, 54, stdout); // Colors for the Italian flag const uint8_t green[3] = {0, 140, 70}; // Green const uint8_t white[3] = {255, 255, 255}; // White const uint8_t red[3] = {206, 41, 57}; // Red // Write pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t pixel[3]; // Divide flag into three equal vertical sections if (x < width / 3) { // Left third: Green pixel[0] = green[2]; pixel[1] = green[1]; pixel[2] = green[0]; } else if (x < 2 * width / 3) { // Middle third: White pixel[0] = white[2]; pixel[1] = white[1]; pixel[2] = white[0]; } else { // Right third: Red pixel[0] = red[2]; pixel[1] = red[1]; pixel[2] = red[0]; } fwrite(pixel, 1, 3, stdout); } } return 0; } |
I extracted the following code from that output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #include <stdio.h> #include <stdint.h> // Function to write little-endian values void write_le(uint8_t* buf, uint32_t value, int size) { for (int i = 0; i < size; i++) { buf[i] = value & 0xFF; value >>= 8; } } int main() { // Flag dimensions const int width = 300; const int height = 200; // BMP header uint8_t header[54] = {0}; header[0] = 'B'; header[1] = 'M'; // File size uint32_t file_size = 54 + width * height * 3; write_le(header + 2, file_size, 4); // Reserved write_le(header + 6, 0, 4); // Pixel data offset write_le(header + 10, 54, 4); // DIB header size write_le(header + 14, 40, 4); // Image width and height write_le(header + 18, width, 4); write_le(header + 22, height, 4); // Planes write_le(header + 26, 1, 2); // Bits per pixel write_le(header + 28, 24, 2); // Write header fwrite(header, 1, 54, stdout); // Colors for the Italian flag const uint8_t green[3] = {0, 140, 70}; // Green const uint8_t white[3] = {255, 255, 255}; // White const uint8_t red[3] = {206, 41, 57}; // Red // Write pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t pixel[3]; // Divide flag into three equal vertical sections if (x < width / 3) { // Left third: Green pixel[0] = green[2]; pixel[1] = green[1]; pixel[2] = green[0]; } else if (x < 2 * width / 3) { // Middle third: White pixel[0] = white[2]; pixel[1] = white[1]; pixel[2] = white[0]; } else { // Right third: Red pixel[0] = red[2]; pixel[1] = red[1]; pixel[2] = red[0]; } fwrite(pixel, 1, 3, stdout); } } return 0; } |
Running the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | #include <stdio.h> #include <stdint.h> // Function to write little-endian values void write_le(uint8_t* buf, uint32_t value, int size) { for (int i = 0; i < size; i++) { buf[i] = value & 0xFF; value >>= 8; } } int main() { // Flag dimensions const int width = 300; const int height = 200; // BMP header uint8_t header[54] = {0}; header[0] = 'B'; header[1] = 'M'; // File size uint32_t file_size = 54 + width * height * 3; write_le(header + 2, file_size, 4); // Reserved write_le(header + 6, 0, 4); // Pixel data offset write_le(header + 10, 54, 4); // DIB header size write_le(header + 14, 40, 4); // Image width and height write_le(header + 18, width, 4); write_le(header + 22, height, 4); // Planes write_le(header + 26, 1, 2); // Bits per pixel write_le(header + 28, 24, 2); // Write header fwrite(header, 1, 54, stdout); // Colors for the Italian flag const uint8_t green[3] = {0, 140, 70}; // Green const uint8_t white[3] = {255, 255, 255}; // White const uint8_t red[3] = {206, 41, 57}; // Red // Write pixel data for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { uint8_t pixel[3]; // Divide flag into three equal vertical sections if (x < width / 3) { // Left third: Green pixel[0] = green[2]; pixel[1] = green[1]; pixel[2] = green[0]; } else if (x < 2 * width / 3) { // Middle third: White pixel[0] = white[2]; pixel[1] = white[1]; pixel[2] = white[0]; } else { // Right third: Red pixel[0] = red[2]; pixel[1] = red[1]; pixel[2] = red[0]; } fwrite(pixel, 1, 3, stdout); } } return 0; } |
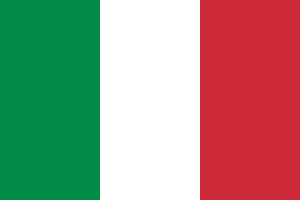
What flag is shown in this image?
This is the flag of Italy.
Testing if the previous output contains the string Italian
: False
Testing if the previous output contains the string Italy
: True